PowerShell can be used to automate FTP tasks, such as uploading and downloading files, through scripting commands that leverage the FTP protocol.
Here's a simple code snippet to connect to an FTP server and upload a file:
$ftp = "ftp://example.com"
$username = "yourUsername"
$password = "yourPassword"
$file = "C:\path\to\your\file.txt"
$ftpUpload = "$ftp/file.txt"
$webclient = New-Object System.Net.WebClient
$webclient.Credentials = New-Object System.Net.NetworkCredential($username, $password)
$webclient.UploadFile($ftpUpload, "STOR", $file)
Understanding FTP
What is FTP?
File Transfer Protocol (FTP) is a standard network protocol used to transfer files between a client and a server over a computer network. It's widely utilized for uploading, downloading, and managing files on remote servers. Common use cases include transferring website files, downloading software packages, and synchronizing data between local and remote systems. It's essential to differentiate FTP from its secure counterparts, SFTP (SSH File Transfer Protocol) and FTPS (FTP Secure), which provide enhanced security features for data transmission.
How FTP Works
FTP operates on a client-server architecture where a client initiates a connection to the server to send or receive files. The protocol can function in two modes: active and passive. In active mode, the client opens a port and listens for incoming data from the server. In passive mode, the server opens a port, and the client connects to it, which is often more firewall-friendly. Understanding this can help you troubleshoot connection issues based on your network configuration.

Getting Started with PowerShell FTP
Setting Up Your Environment
Before using PowerShell for FTP operations, ensure you have PowerShell installed on your system and that you possess the necessary permissions to perform file transfers. You do not need any additional modules for basic FTP operations, as PowerShell supports FTP natively via the `.NET` framework.
Using `WebClient` for FTP Transfers
What is `WebClient`?
The `WebClient` class in PowerShell provides an easy way to send data to and receive data from Internet resources. It includes methods for uploading and downloading files, making it an ideal choice for FTP operations.
Connecting to FTP Server
To establish a connection to your FTP server, you need to create a `WebClient` object and set its credentials. Here's how you do it:
$ftpServer = "ftp://yourftpserver.com"
$username = "yourusername"
$password = "yourpassword"
$client = New-Object System.Net.WebClient
$client.Credentials = New-Object System.Net.NetworkCredential($username, $password)
With this setup, you've successfully configured the client to authenticate with your FTP server.
Uploading Files to FTP
Step-by-Step Process
To upload a file to the FTP server, specify the path of the file you want to upload and the target URL on the FTP server. Here’s an example:
$filePath = "C:\path\to\yourfile.txt"
$ftpUploadUrl = "$ftpServer/yourfile.txt"
$client.UploadFile($ftpUploadUrl, "STOR", $filePath)
Explanation of the Code
- The `$filePath` variable holds the location of your local file.
- The `$ftpUploadUrl` variable constructs the full URL where the file will be uploaded.
- The `UploadFile` method sends the file to the specified URL using the "STOR" FTP command.
In case of errors, you might encounter messages such as "Permission denied" or "File not found." Always ensure that your paths are correct and that you have the necessary permissions on the server.
Downloading Files from FTP
Step-by-Step Process
Downloading files is just as straightforward. Here’s how you can retrieve a file from your FTP server:
$ftpDownloadUrl = "$ftpServer/yourfile.txt"
$outputPath = "C:\path\to\downloadedfile.txt"
$client.DownloadFile($ftpDownloadUrl, $outputPath)
Explanation of the Code
- The `$ftpDownloadUrl` includes the URL of the file you wish to download.
- The `$outputPath` denotes where you want to save the downloaded file on your local system.
- The `DownloadFile` method performs the file retrieval operation.
Similar to uploads, if you encounter issues such as "File not found," ensure that you're pointing to the correct URL and check the server's directory structure.

Advanced PowerShell FTP Techniques
Listing Files on FTP Server
Using FTP Commands
If you need to list the files on your FTP server, you’ll utilize the `FtpWebRequest` class. Here’s a snippet demonstrating how to accomplish this:
$request = [System.Net.FtpWebRequest]::Create("$ftpServer/")
$request.Method = [System.Net.WebRequestMethods+Ftp]::ListDirectory
$request.Credentials = New-Object System.Net.NetworkCredential($username, $password)
$response = $request.GetResponse()
$reader = New-Object IO.StreamReader $response.GetResponseStream()
while(!$reader.EndOfStream) {
$line = $reader.ReadLine()
Write-Output $line
}
Explanation of the Listing Code
- You initiate an FTP request using the `Create` method.
- The method is set to `ListDirectory`, prompting the server to send back a list of files and folders in the current directory.
- The response is read line by line, outputting each file name.
When working with many files, consider implementing pagination or limiting the results if the listing is extensive.
Deleting Files from FTP
Code Snippet for Deletion
If you need to delete a file on the FTP server, here’s how you can do it:
$fileToDelete = "$ftpServer/yourfile.txt"
$request = [System.Net.FtpWebRequest]::Create($fileToDelete)
$request.Method = [System.Net.WebRequestMethods+Ftp]::DeleteFile
$request.Credentials = New-Object System.Net.NetworkCredential($username, $password)
$response = $request.GetResponse()
Important Considerations
Deleting files is a permanent action, so proceed with caution. Always ensure you are targeting the correct file and have backups if necessary. Unexpected deletions can lead to significant data loss.

Error Handling and Troubleshooting
Common Errors
When working with FTP via PowerShell, you might encounter various errors such as:
- Connection issues: This might be due to firewall settings or incorrect server addresses.
- Permission denied: Ensure your credentials have the necessary access rights.
- Timeout errors: These can result from server overload or network issues.
Best Practices for Troubleshooting
When troubleshooting FTP problems:
- Check your connection settings: Ensure the server address, username, and password are correct.
- Verify firewall rules: Ensure that the appropriate ports for FTP (usually 21) are open.
- Logs and Responses: Utilize logs and server responses to identify specific errors and adjust permissions accordingly.
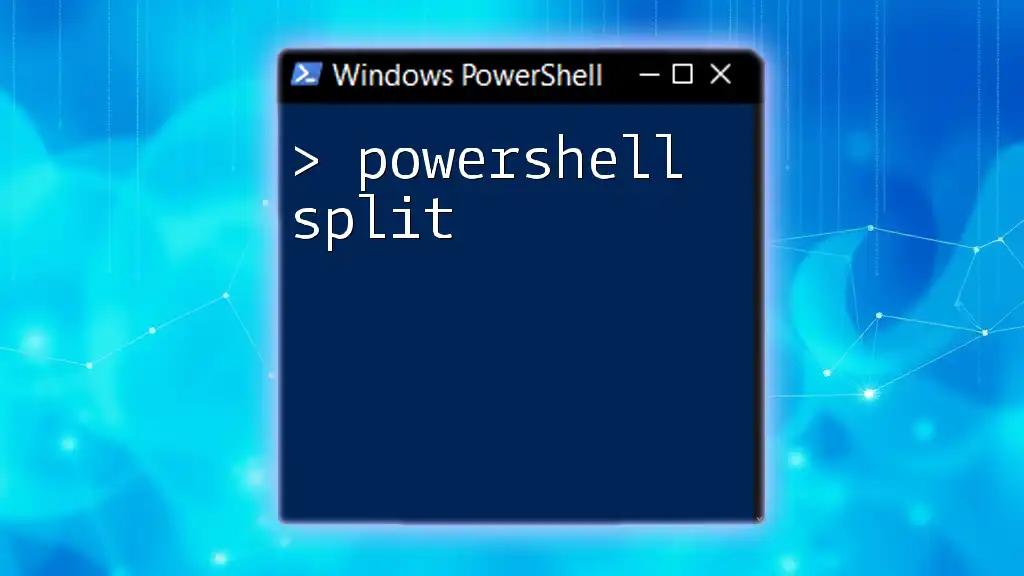
Conclusion
In this guide, we've explored the fundamental aspects of using PowerShell for FTP operations, including file uploads, downloads, and advanced commands. With these tools, you can efficiently manage file transfers from your local machine to virtually any FTP server. The simplicity and power of PowerShell make it a valuable asset for system administrators and IT professionals alike. We encourage you to practice these examples and leverage PowerShell's capabilities to enhance your file management workflows.
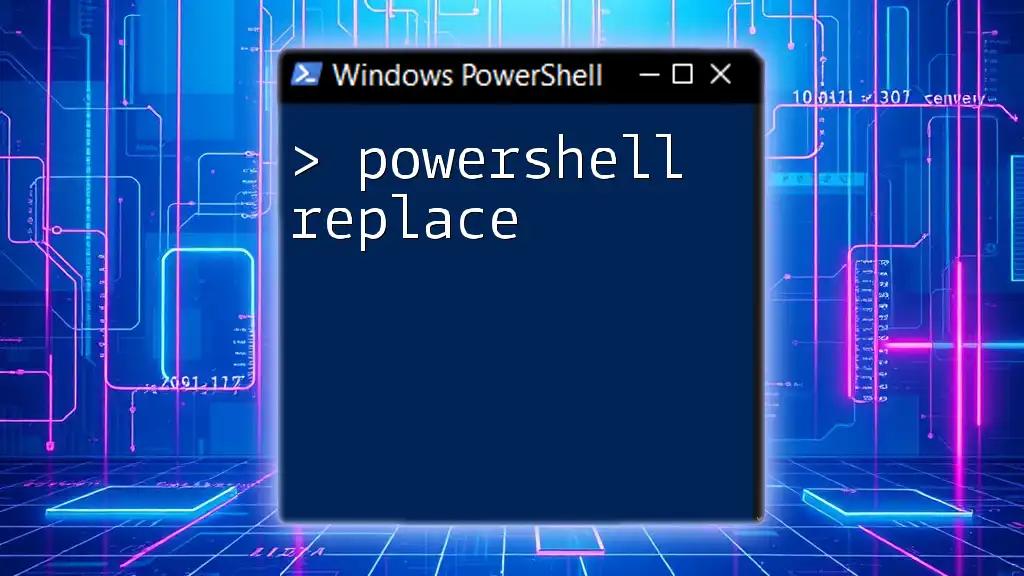
Additional Resources
For further learning, check out the official PowerShell documentation which provides in-depth insights and advanced functionalities. If you're looking for structured learning, consider online courses that delve deeper into PowerShell and networking protocols.