FTPS (FTP Secure) in PowerShell allows you to transfer files securely over the Internet using the FTPS protocol, ensuring that data is encrypted during transmission.
Here’s a basic code snippet to establish an FTPS connection using PowerShell:
$ftp = "ftps://yourftpserver.com"
$ftpUsername = "yourUsername"
$ftpPassword = "yourPassword"
$localFile = "C:\path\to\your\file.txt"
$remotePath = "/path/on/ftp/server/file.txt"
$ftpRequest = [System.Net.FtpWebRequest]::Create("$ftp$remotePath")
$ftpRequest.Method = [System.Net.WebRequestMethods+Ftp]::UploadFile
$ftpRequest.Credentials = New-Object System.Net.NetworkCredential($ftpUsername, $ftpPassword)
$ftpRequest.EnableSsl = $true
$fileContent = [System.IO.File]::ReadAllBytes($localFile)
$ftpRequest.ContentLength = $fileContent.Length
$stream = $ftpRequest.GetRequestStream()
$stream.Write($fileContent, 0, $fileContent.Length)
$stream.Close()
$response = $ftpRequest.GetResponse()
$response.Close()
Understanding FTPS
What is FTPS?
FTPS, or FTP Secure, is an extension to the commonly used File Transfer Protocol (FTP). It adds a layer of security by utilizing SSL (Secure Sockets Layer) or TLS (Transport Layer Security) encryption. The primary difference between FTPS and FTP lies in the secure connection that FTPS offers, ensuring that data is transferred securely over the network. The key benefits of using FTPS include:
- Data Encryption: Protects sensitive information during transfer.
- Authentication Options: Supports user authentication, ensuring proper access control.
- Compatibility: Utilizes existing FTP commands, making it easier to implement for users familiar with FTP.
How FTPS Works
FTPS operates through a process called the handshake. First, the client initiates a connection to the FTP server and requests a secure connection. Once the server confirms support for FTPS, they exchange SSL/TLS certificates to authenticate each other. After the handshake, both the control commands (like logging in) and data transfer (like file uploads/downloads) happen over encrypted channels.
The FTPS process involves two types of connections:
- Control Connection: Used for sending commands and receiving responses.
- Data Connection: Established for the actual file transfer.
The use of SSL/TLS ensures that both connections are secured, protecting user credentials and file contents from potential eavesdropping.
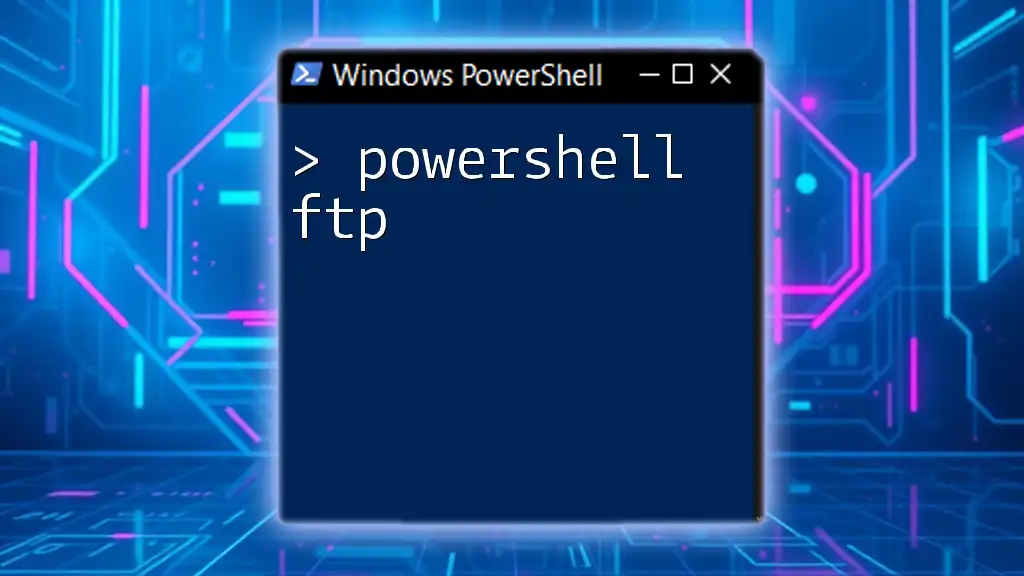
Setting Up PowerShell for FTPS
Prerequisites
Before diving into FTPS commands, ensure that your environment is prepared. You'll need PowerShell installed on your system. Additionally, consider using modules such as WinSCP or PSSFTP as they simplify FTPS tasks significantly.
Installing Required Modules
To install the PSSFTP module, execute the following command in your PowerShell console:
Install-Module -Name PSSFTP
For users opting for WinSCP, it can be downloaded from the official website, and users can reference it from their PowerShell scripts after installation.
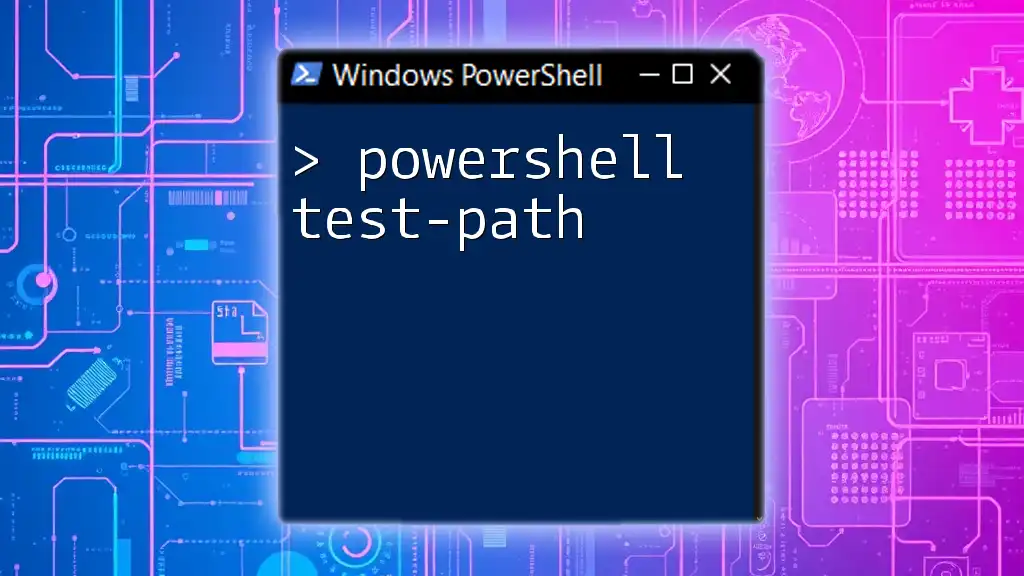
Basic FTPS Commands in PowerShell
Connecting to an FTPS Server
Connecting to an FTPS server is straightforward once you have the necessary credentials. The code snippet below demonstrates how to establish a connection using WinSCP:
$session = New-Object WinSCP.Session
$sessionOptions = New-Object WinSCP.SessionOptions -Property @{
Protocol = [WinSCP.Protocol]::Ftp
HostName = "ftp.example.com"
UserName = "username"
Password = "password"
TlsSecure = $true
}
$session.Open($sessionOptions)
This code snippet initializes a new session and specifies the connection parameters like the hostname and user credentials. Make sure to replace `ftp.example.com`, `username`, and `password` with your actual server details.
Uploading Files to an FTPS Server
Once connected, uploading files is a seamless process. Here’s a simple way to upload a file to the FTPS server:
$transferOptions = New-Object WinSCP.TransferOptions
$transferOptions.TransferMode = [WinSCP.TransferMode]::Binary
$transferOperation = $session.PutFiles("C:\local\path\file.txt", "/remote/path/", $false, $transferOptions)
This command uploads `file.txt` from your local machine to the specified remote path on your FTPS server. The `TransferMode` is set to Binary, which is recommended for file transfers to ensure no data is altered during the transfer.
Downloading Files from an FTPS Server
To download files from the server, simply use the following example code:
$session.GetFiles("/remote/path/file.txt", "C:\local\path\").Check()
This command downloads the specified `file.txt` from the remote directory to your local specified path.
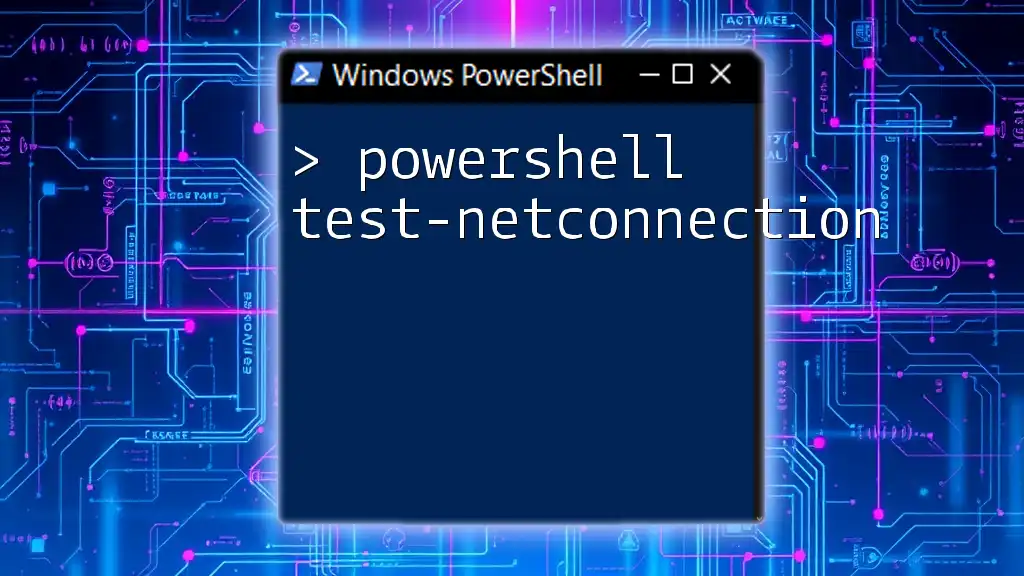
Advanced FTPS Operations
Listing Files and Directories
To list files residing on an FTPS server, use the following code snippet:
$directoryInfo = $session.ListDirectory("/remote/path/")
foreach ($fileInfo in $directoryInfo.Files) {
Write-Host $fileInfo.Name
}
This code retrieves information about files in the specified remote directory and outputs each file name. This can help you manage files effectively without needing a graphical interface.
Deleting Files on an FTPS Server
If you need to delete files or directories remotely, you can utilize the following command:
$session.RemoveFile("/remote/path/file_to_delete.txt")
Using this command, you can permanently remove `file_to_delete.txt` from the specified remote path—be cautious, as this action cannot be undone.
Error Handling and Debugging
Handling errors effectively is crucial when dealing with file transfers. Below is a simple error handling example:
try {
# FTPS command here
} catch {
Write-Host "Error encountered: $_"
}
This structure allows you to catch exceptions during operations, facilitating debugging by outputting the error message.
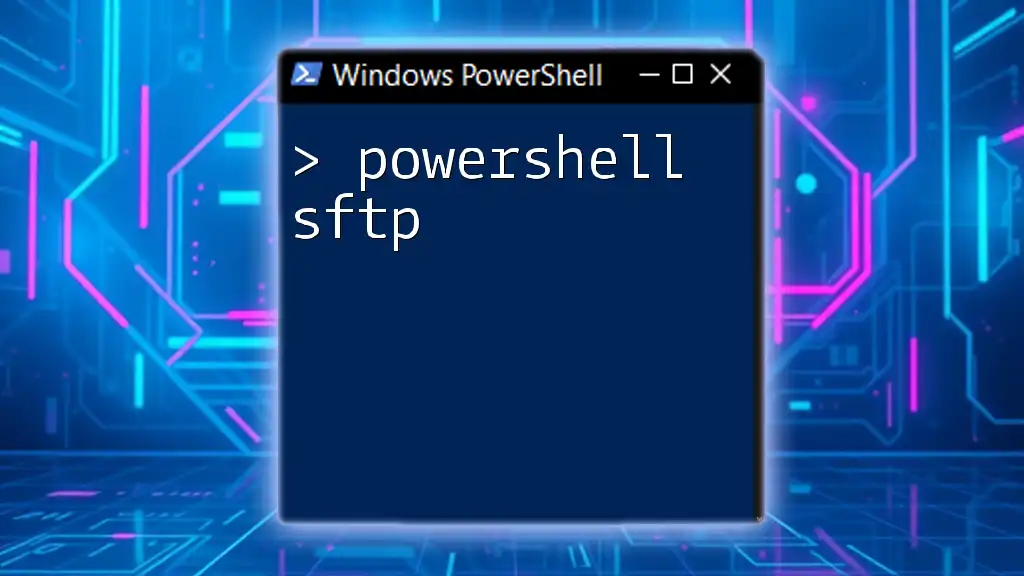
Security Best Practices
Configuring FTPS for Enhanced Security
When configuring FTPS, several best practices enhance security. Make sure to:
- Use strong passwords for user authentication.
- Regularly rotate credentials to mitigate the risk of exposure.
- Consider implementing SSL certificates from reputable authorities to prevent man-in-the-middle attacks.
Keeping Your PowerShell Environment Safe
Maintaining a safe PowerShell environment is equally important. Regular updates to PowerShell, as well as careful handling of scripts and modules, will help in minimizing vulnerabilities. You should periodically review scripts for vulnerabilities and unnecessary permissions.
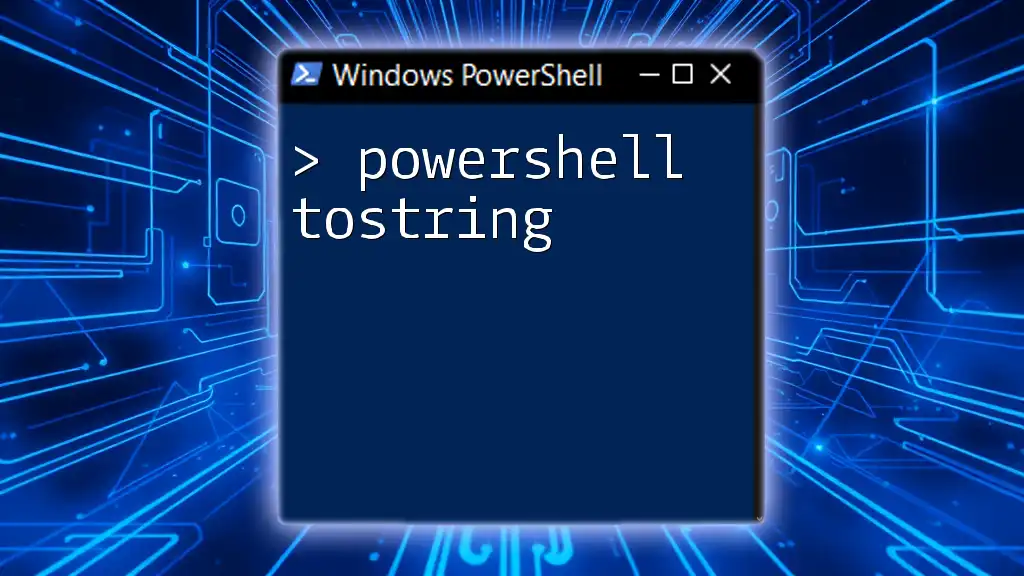
Conclusion
Utilizing PowerShell FTPS commands can significantly streamline your secure file transfer processes. With the knowledge gained in this guide, you can confidently connect to FTPS servers, upload and download files securely, and manage file operations—all while adhering to advanced security practices. Explore the example snippets provided, and leverage them to enhance your workflow in managing and transferring files securely.
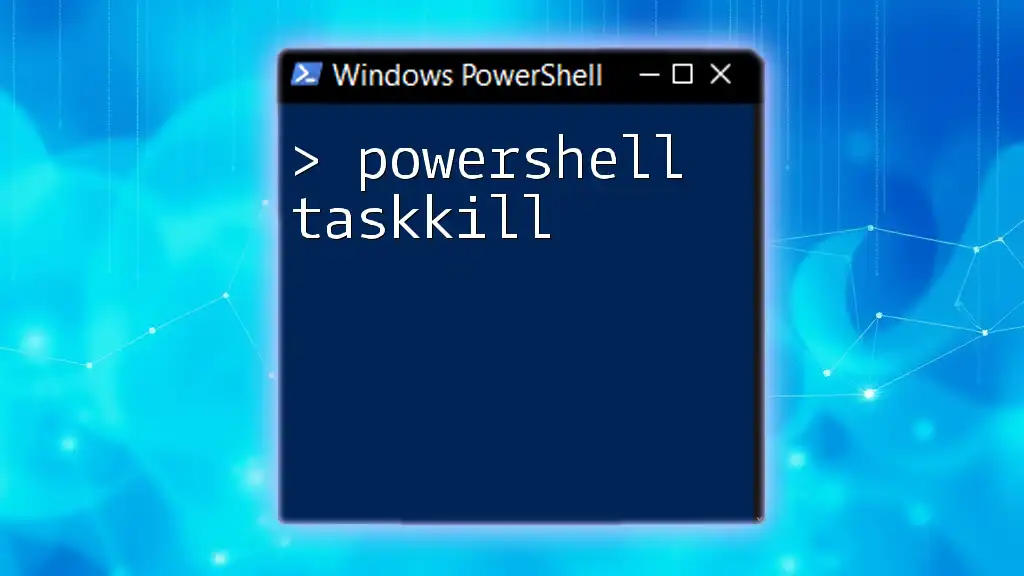
FAQs
What is the difference between FTPS and SFTP?
FTPS and SFTP are both secure file transfer protocols, but they utilize different underlying technologies. FTPS is built on FTP and uses SSL/TLS for securing connections, while SFTP (SSH File Transfer Protocol) relies on the SSH protocol for secure file transfer, making it more straightforward to configure in scenarios where only a single port needs to be opened.
Can I use PowerShell FTPS commands on any operating system?
While PowerShell is primarily associated with Windows, the FTPS commands can be executed on other platforms that support PowerShell Core, such as Linux and macOS. However, ensure compatibility with the respective modules being used.
Where can I find more resources for PowerShell and FTPS?
Consider visiting the official PowerShell documentation, joining online forums, or exploring platforms like GitHub for community contributions and additional resources related to PowerShell scripting, specifically FTPS operations.
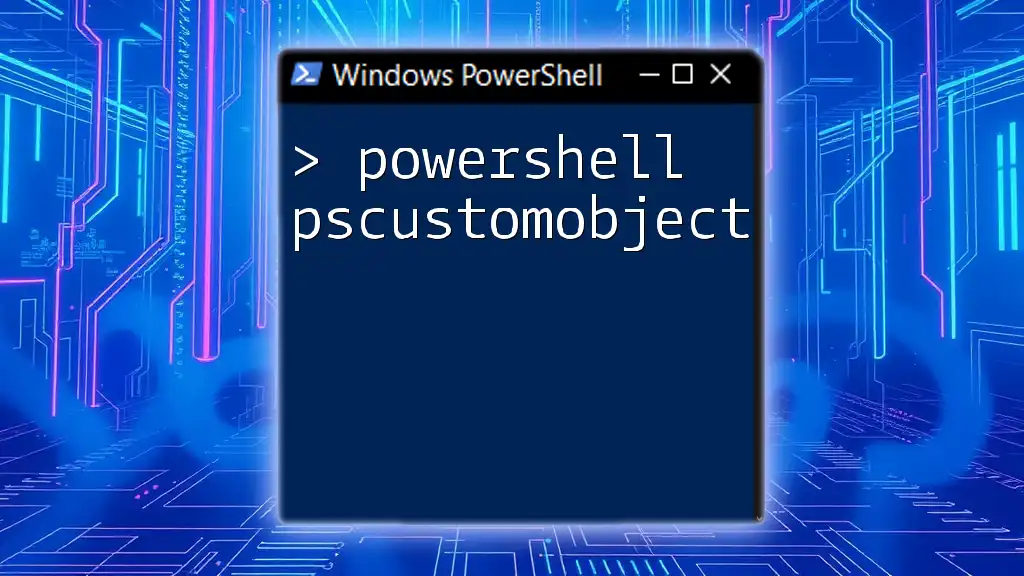
Additional Resources
For further learning, consider checking:
- PowerShell documentation for in-depth understanding and updates.
- Recommended books on PowerShell scripting which can provide insights into best practices and advanced features.
- Online tutorials, courses, and community forums where you can engage with other PowerShell users and FTPS experts for collaborative learning.
Code References
You can find the code snippets used in this article on GitHub.