In PowerShell, you can suppress error messages from commands by redirecting the error output stream to null using the following syntax:
Command-That-Might-Fail *>$null
For example, if you want to suppress errors when trying to access a non-existent file, you can use:
Get-Content 'C:\path\to\nonexistentFile.txt' *>$null
Understanding Errors in PowerShell
What are Errors?
Errors in PowerShell are issues that arise during the execution of commands or scripts. They can disrupt the flow of execution, leading to incomplete tasks or unexpected outcomes. PowerShell distinguishes between two main types of errors:
-
Terminating Errors: These stop the execution of the command or script. For example, trying to access a nonexistent file will result in a terminating error.
-
Non-Terminating Errors: These allow the command or script to continue running, even if an error occurs partway through. Many common cmdlets produce non-terminating errors.
Why Suppress Errors?
Suppressing errors can be necessary in various situations. Here are a few reasons why you might want to do so:
-
Quiet Execution: Sometimes, you don't want the output cluttered with error messages. This is especially useful in scripts meant for end-users who may not understand the underlying issues.
-
Batch Operations: When performing a batch operation on multiple files or records, errors on some items might not affect others. For example, attempting to clean up files may encounter one or two files that do not exist, but you don't want the entire process aborted.
However, it's crucial to consider the potential risks of suppressing errors. Ignoring error messages can lead to unresolved issues that could affect the integrity of your system or data.

Methods to Suppress Errors in PowerShell
Using the `-ErrorAction` Parameter
One of the most effective ways to suppress errors in PowerShell is by using the `-ErrorAction` parameter available in many cmdlets. This parameter allows you to specify how PowerShell should respond to errors that occur during command execution.
By default, PowerShell will display error messages and continue executing. You can adjust this behavior using the following options:
- SilentlyContinue: Suppresses the error message and continues execution.
- Continue: Displays the error message and continues execution.
- Stop: Terminates the command execution upon encountering an error.
Code Snippet Example:
Get-Content "nonexistentfile.txt" -ErrorAction SilentlyContinue
In this example, if "nonexistentfile.txt" does not exist, PowerShell will not display any error message but will continue executing subsequent commands.
Setting `$ErrorActionPreference`
Another powerful way to manage error handling is through the `$ErrorActionPreference` variable. This variable determines how errors are handled globally across your PowerShell session.
When you set `$ErrorActionPreference`, it affects all commands run after that assignment, unless overridden by a specific `-ErrorAction` setting.
To suppress errors globally, set it as follows:
$ErrorActionPreference = 'SilentlyContinue'
By doing this, all subsequent commands will suppress error messages, improving the overall user experience in contexts where errors are not critical.
Try-Catch-Finally Blocks
While suppressing errors can simplify script output, there are scenarios where you want to handle them more gracefully. PowerShell provides structured error handling through `Try-Catch-Finally` blocks.
Using these blocks allows you to catch and respond to errors without simply discarding them. Here’s how it works:
try {
Get-Content "nonexistentfile.txt"
} catch {
# Handle the error
Write-Host "An error occurred: $_"
}
In this example, instead of suppressing the error entirely, you can log it or notify the user when an error occurs, allowing for better debugging and system integrity.
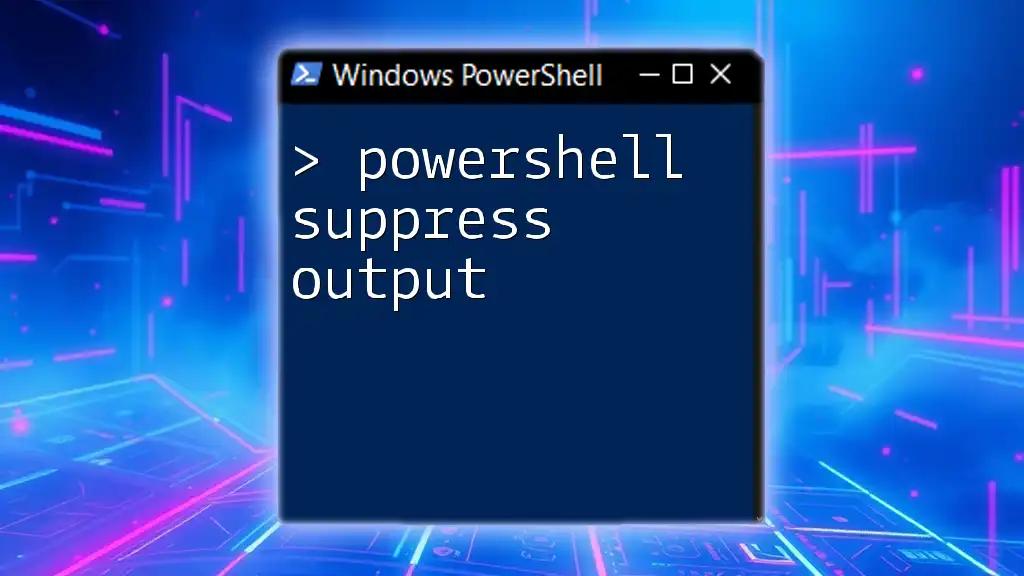
Best Practices for Suppressing Errors
Use Sparingly
While it can be tempting to suppress errors to create a cleaner output, it is vital to use this approach judiciously. Always consider the implications of ignoring warnings or errors, as it might lead to bigger issues down the line.
Log Errors for Later Review
One of the best practices involves logging errors even as you suppress them. This ensures you can monitor and address any issues without cluttering regular output.
For example, you can set your script to log errors into a file while allowing the rest of the script to run smoothly:
$ErrorActionPreference = 'Continue'
try {
Get-Content "nonexistentfile.txt"
} catch {
Add-Content "errorlog.txt" $_
}
In this scenario, even though you allow the script to proceed after an error, the details of the error will be captured in "errorlog.txt".
Testing Scripts Without Error Suppression
When developing scripts, it's essential to test them thoroughly without suppressing errors. This process helps identify potential issues early on. Use comments to disable error suppression during the testing phase, ensuring you can see all error messages.
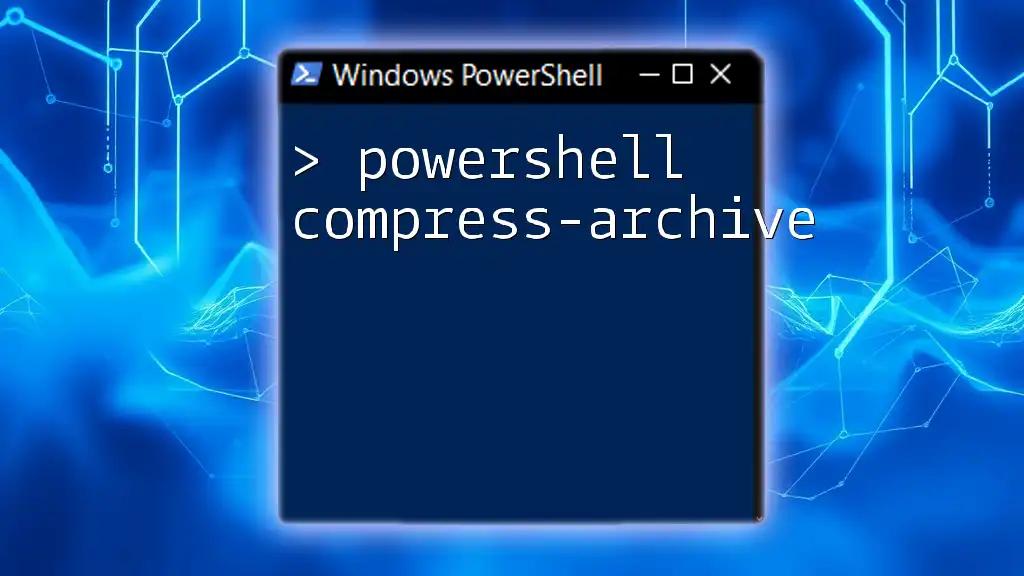
Conclusion
Understanding how to suppress errors in PowerShell is critical for anyone looking to write effective and robust scripts. By using the `-ErrorAction` parameter, setting `$ErrorActionPreference`, and implementing `Try-Catch` blocks, you can manage errors according to your requirements.
Remember to balance the need for a clean output with the importance of acknowledging potential issues. Log errors for future review, practice caution when suppressing errors, and always test your scripts thoroughly. By mastering these techniques, you'll enhance your PowerShell skills, leading to more efficient scripting and administration.
For additional resources, consider exploring PowerShell documentation on error handling and aim to enhance your skills through practical learning and hands-on experience in your future courses.