In PowerShell, you can suppress error output to the console by redirecting the error stream to `$null`, as shown in the code snippet below:
Command-That-Might-Fail 2>$null
Understanding Error Types in PowerShell
What Are Errors?
Errors in PowerShell can significantly affect how scripts and commands execute. Generally, there are two main classifications of errors: terminating and non-terminating. Understanding these error types is essential for effectively managing error output.
Types of Errors
Terminating Errors are critical failures that stop command execution immediately. For example, if an attempt is made to read from a nonexistent file, this kind of error will cause the script to halt entirely.
Example:
Get-Content "C:\nonexistent_file.txt"
This command will throw an error, effectively terminating subsequent commands unless properly managed.
On the other hand, Non-Terminating Errors allow the script to continue execution even when an error occurs. These are often used for data validation, where you want to identify issues without stopping the entire process.
Example:
Get-Content "C:\path\to\file.txt"
If the file does not exist, PowerShell will issue a non-terminating error, which means that other commands can still run, which can be useful in scenarios where one error shouldn’t stop the entire sequence.
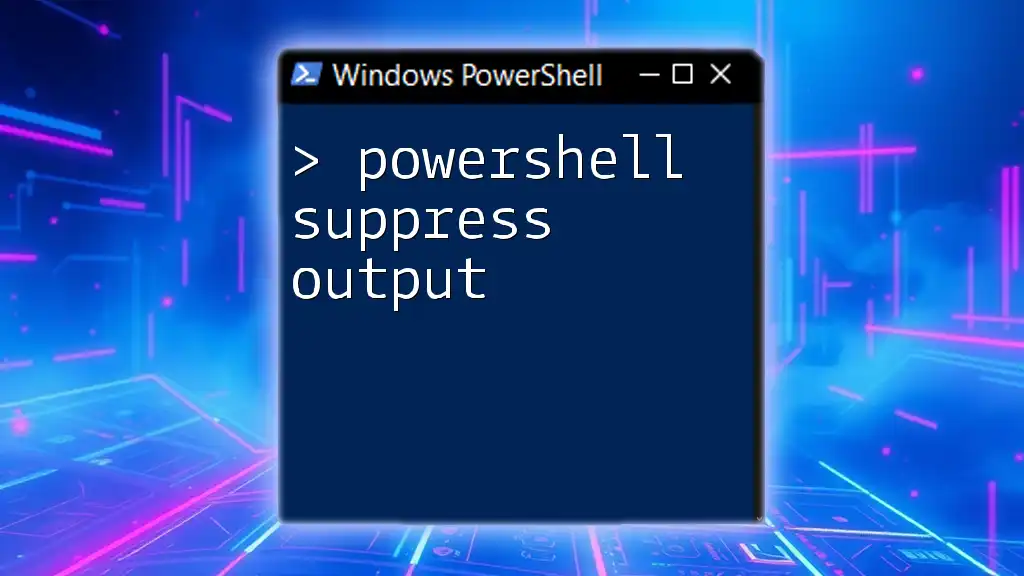
Why Suppress Error Output?
Common Scenarios for Suppressing Errors
There are numerous situations where you might want to suppress errors in PowerShell. For instance, automated scripts running in the background or system maintenance tasks often benefit from reduced console clutter. By suppressing unnecessary error messages, the output becomes clearer for end-users or system administrators.
Consequences of Unmanaged Error Output
When errors are displayed willy-nilly in the console, they can lead to confusion. Unmanaged error output can obscure important messages, making it difficult to troubleshoot or maintain scripts. This can be both frustrating and inefficient, particularly for users who are not as familiar with PowerShell.
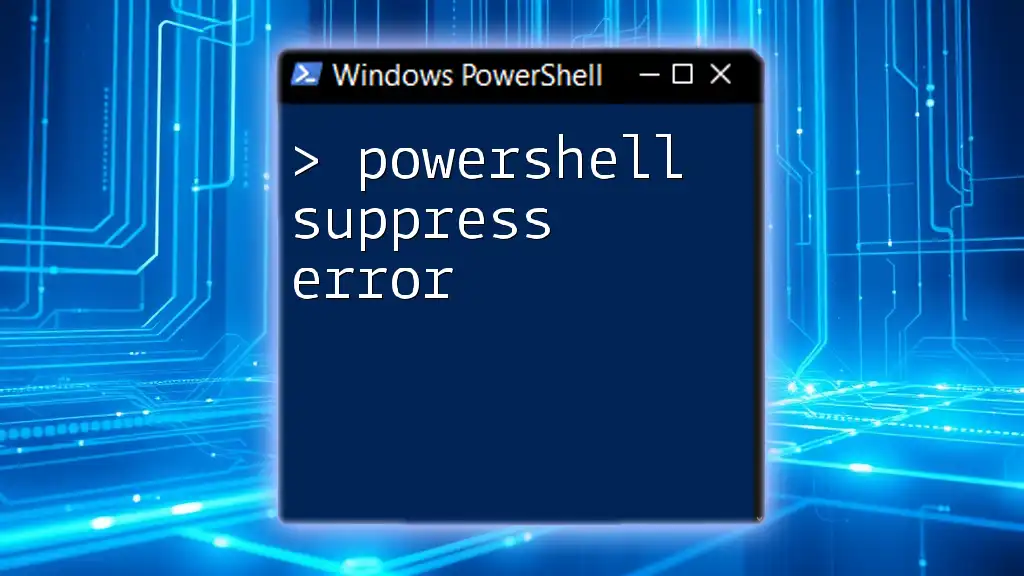
Techniques to Suppress Error Output
Using the `-ErrorAction` Parameter
One of the most effective ways to suppress error output is by employing the `-ErrorAction` parameter. This allows you to define how PowerShell responds to errors during command execution.
- SilentlyContinue: This setting suppresses all error messages.
Example:
Get-Content "C:\path\to\file.txt" -ErrorAction SilentlyContinue
In this example, if the file doesn’t exist, no error output will be shown, allowing the script to continue without interruption.
Redirecting Error Output with `2>$null`
Another straightforward method to suppress error messages is by redirecting the error stream to a null value using `2>$null`. This sends error output to a "black hole," effectively making it disappear from the console.
Example:
Get-Content "C:\nonexistent_file.txt" 2>$null
Here, even if the file does not exist and an error occurs, it won’t display anything in the console.
Using `Try` and `Catch` Blocks
PowerShell’s `try` and `catch` blocks offer a structured way to manage errors. By wrapping commands in a `try` block, you can catch errors in the `catch` block and handle them gracefully.
Example:
try {
Get-Content "C:\nonexistent_file.txt"
} catch {
# Handle the error gracefully
Write-Host "Error encountered, but the script continues."
}
This approach provides more control as you can choose to log the error, notify the user, or take corrective actions.
Suppressing Errors in Functions
When working with functions, managing error output globally can be essential. You can use the `$ErrorActionPreference` variable to dictate how errors are handled in your session or script.
Example:
$ErrorActionPreference = "SilentlyContinue"
MyFunction
Setting the `$ErrorActionPreference` to "SilentlyContinue" ensures that errors within `MyFunction` do not upset the console output.
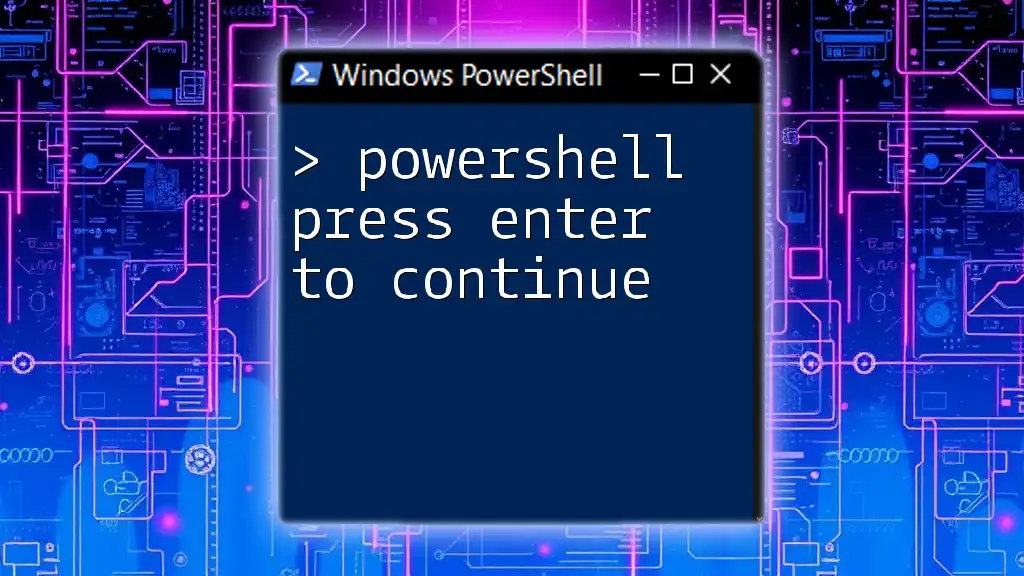
Additional Tips for Error Management
Logging Errors for Review
Rather than completely suppressing error messages, consider logging them for future reference. Maintaining a record of errors can help when troubleshooting similar issues later on.
Example:
$ErrorActionPreference = "Continue"
Get-Content "C:\nonexistent_file.txt" 2>> "C:\path\to\log.txt"
This command keeps track of any errors by appending them to `log.txt`, allowing you to review any issues without letting them clutter the immediate console output.
Best Practices for Suppressing Errors
While it may be tempting to suppress all error output, it’s crucial to discern when to do so. Always weigh the benefits of having clean output against the potential risks of missing important error messages. Aim for a balanced approach where errors that matter are captured, while non-critical ones are managed gracefully.
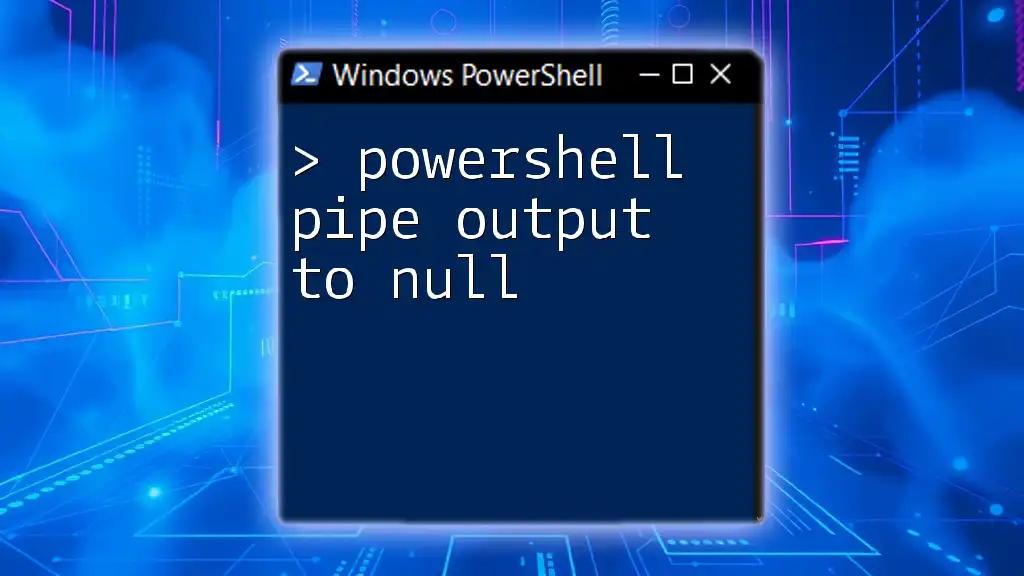
Conclusion
In summary, effectively managing error output in PowerShell is key to writing robust scripts. Utilizing techniques such as the `-ErrorAction` parameter, redirection to `$null`, and structured error handling with `try/catch` blocks can significantly enhance script clarity and performance. By adopting best practices and understanding when to suppress errors, you can make your PowerShell scripts easier to read and maintain.