In PowerShell, you can create a simple prompt that requires the user to press Enter to continue the execution of a script using the `Read-Host` cmdlet.
Read-Host -Prompt 'Press Enter to continue...'
Understanding User Input in PowerShell
What is User Input?
User input is a core concept in scripting that allows a script to receive information directly from the user. This clarity in communication is especially vital in PowerShell, where scripts often control system functions and require user actions. By utilizing prompts, scripts can be interactive rather than run in a vacuum, allowing users to make decisions directly during execution.
Common Methods of User Interaction
PowerShell provides multiple avenues for user prompts, with two of the most commonly used methods being `Read-Host` and `Get-Credential`. While `Read-Host` is instrumental for capturing simple user input, `Get-Credential` is ideal for securely asking for credentials. Understanding these input methods can greatly enhance the way scripts interact with users.
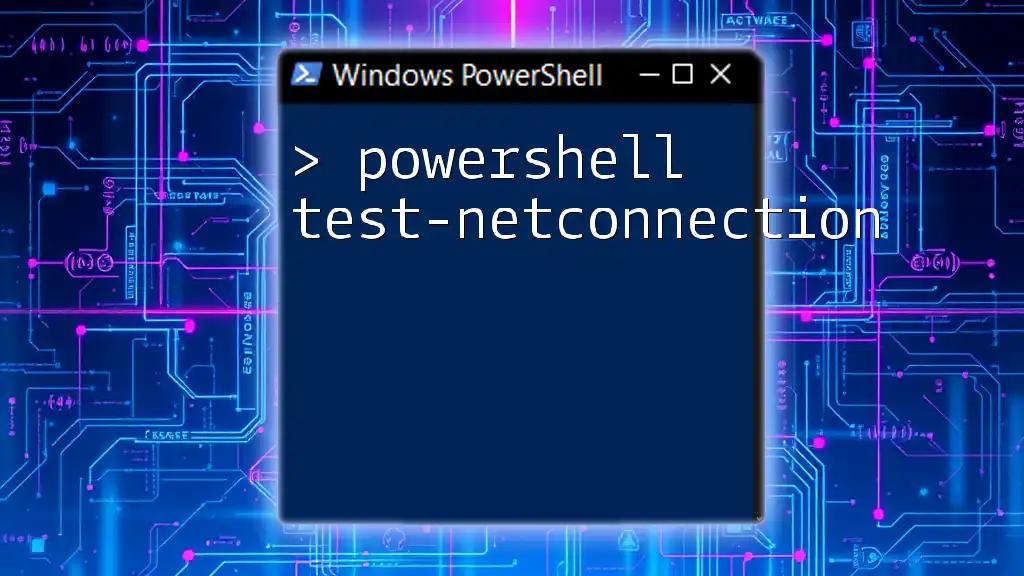
The `Press Enter to Continue` Mechanism
Why Use a "Press Enter" Pause?
Implementing a "Press Enter to Continue" mechanism is crucial for pacing the output of a script. This feature allows users to digest important information before proceeding. It creates a more pleasant user experience by avoiding overwhelming outputs and gives users the control they need to process actions, especially in scripts that perform critical system tasks.
How to Implement `Press Enter to Continue`
Basic Usage of `Read-Host`
One of the simplest methods to create a prompt that pauses execution is by using the `Read-Host` cmdlet. This allows you to inform users of what to do next while waiting for their response.
Code Snippet: Basic Example
Write-Host "Press Enter to Continue..."
$null = Read-Host
In this code, `Write-Host` displays the message, and `$null = Read-Host` waits for the user to press Enter, effectively pausing the script until the user is ready.
Enhancing the User Experience
Customizing the prompt message can make the interaction even more engaging. Providing context in your prompt can help clarify what users need to do.
Code Snippet: Custom Prompt Example
$input = Read-Host "Press Enter when you're ready to proceed"
Here, the script integrates a personalized message, guiding users more effectively through the process. Custom messages can reflect the context of what the script is doing to make the interaction meaningful.
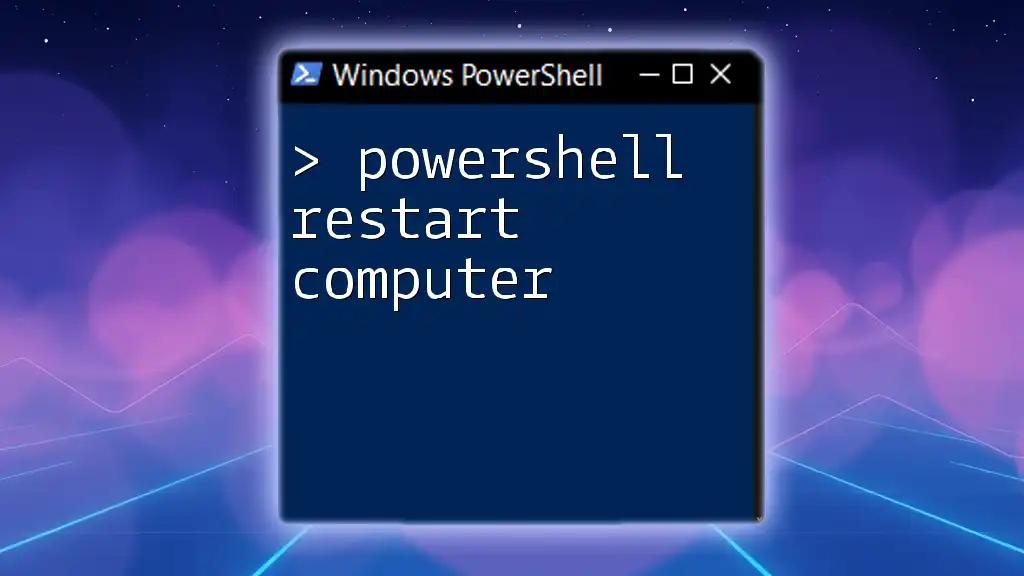
Advanced Techniques
Using Functions for Reusability
Creating a function dedicated to pauses can streamline your scripts and promote reusability across multiple scripts. This encapsulates the functionality and makes it easy to implement without repetitive code.
Code Snippet: Function Creation
function Wait-ForUser {
param(
[string]$Message = "Press Enter to Continue..."
)
Write-Host $Message
$null = Read-Host
}
With this function, you can call `Wait-ForUser` whenever you want to pause, passing a custom message as needed. This approach not only promotes cleaner code but also maintains consistency throughout your scripts.
Integrating with Workflows
In larger, multi-step scripts, it's essential to include pauses to ensure users are consciously engaged with each action being performed.
Code Snippet: Multi-Step Script Example
Write-Host "Step 1: Connecting to the server..."
# Simulated connection
Wait-ForUser
Write-Host "Step 2: Running diagnostics..."
# Simulated diagnostics
Wait-ForUser
Each step of the script incorporates a pause using the `Wait-ForUser` function, allowing users to grasp the significance of each action before proceeding. This method not only enhances clarity but also minimizes potential errors during execution.

Best Practices
When to Use "Press Enter to Continue"
The decision to implement a "Press Enter" pause should be based on the context of your script. Situations where critical operations are performed, or the results need user consent, are ideal for this feature. However, it’s vital to avoid overuse—excessive pauses can frustrate users and lead to a negative experience.
Customizing User Prompts
Effective prompts are clear and concise. Ensure that your messages are straightforward and relevant to the action being performed. Avoid jargon and focus on giving users clear guidance on what to expect next.

Troubleshooting Common Issues
Problem: Script Runs Too Fast
One of the most common complaints is that scripts run too quickly, making it difficult for users to follow along. If you notice feedback like this, integrating pauses will significantly enhance user experience and understanding.
Problem: Input Not Recognized
If you encounter issues with input not being recognized, it may be worth revisiting how you're using `Read-Host`. Ensure there are no syntax errors and that the script logic correctly interprets user inputs. Debugging tips include verifying the script flow and leveraging console outputs to catch problematic interactions.
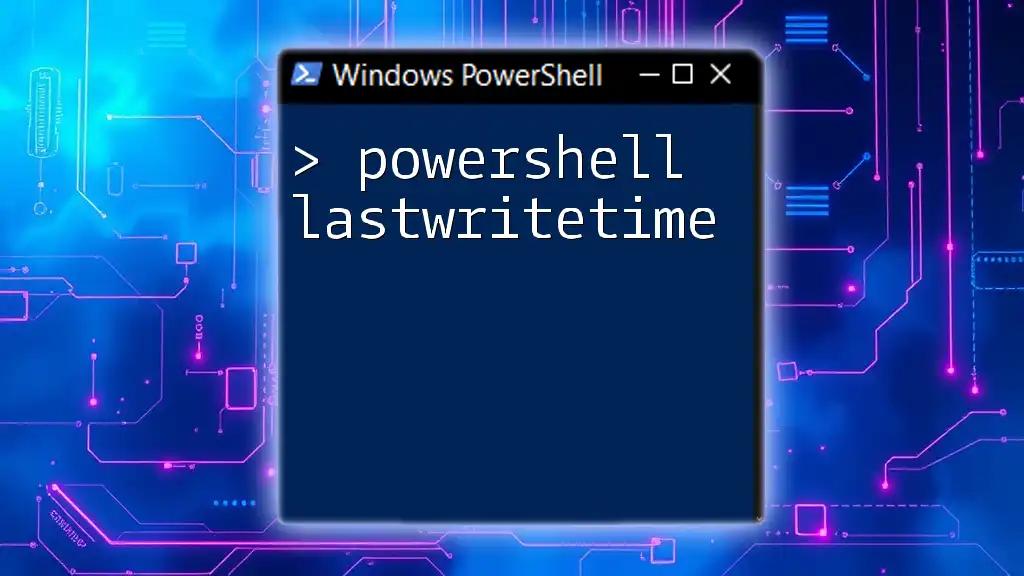
Practical Use Cases
Admin Scripts
In administrative scripts, adding a pause between operations is beneficial to confirm that actions taken are what the user intended—especially in roles with high stakes like system configurations or data migrations.
Educational Purposes
Creating interactive scripts for educational tutorials can also harness the "Press Enter" function effectively. It allows users to work through lessons at their pace, making the learning experience more engaging.
Automated Deployments
In automated deployments, requiring user consent before proceeding with significant actions can prevent unwanted outcomes. It ensures that operators are fully aware of the implications of their choices.
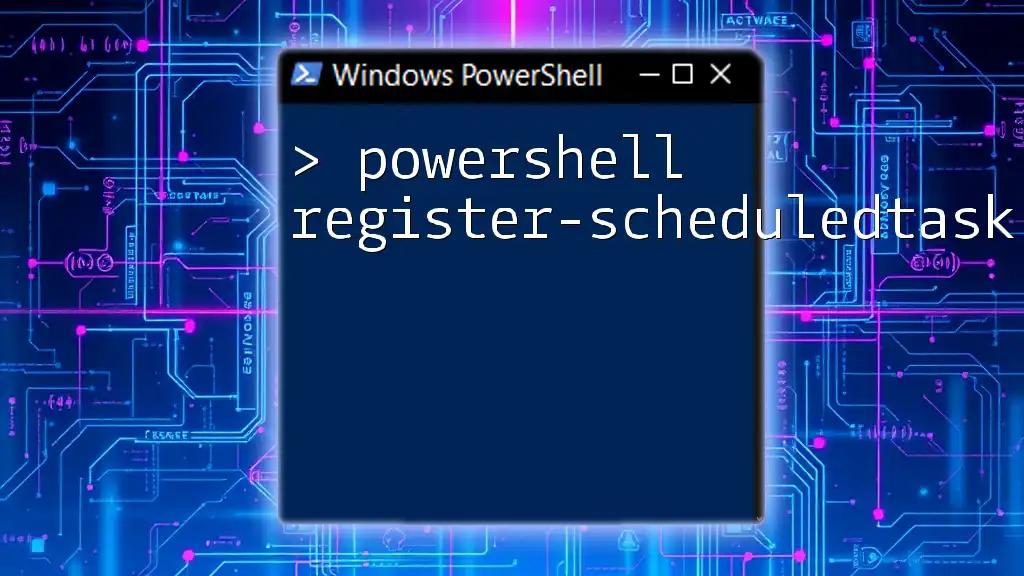
Conclusion
The "Press Enter to Continue" mechanism in PowerShell serves as an indispensable tool for creating interactive scripts that cater to user experience. By incorporating user-focused pauses, you enhance the clarity and intentionality of your scripts, making them not just more functional but also more enjoyable to work with. Whether you are crafting administrative scripts, educational resources, or automated processes, this feature can significantly augment your scripting capabilities.
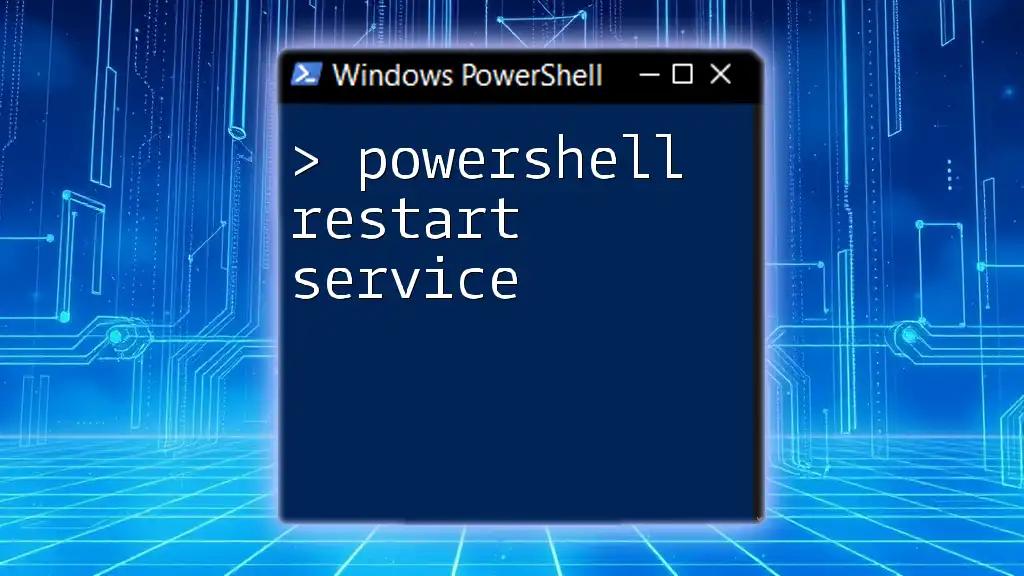
Additional Resources
Further Learning
To deepen your PowerShell knowledge, consider exploring recommended books and online courses that align with your learning style.
Community Support
Engagement with forums and user groups can provide support and spark inspiration. Connecting with like-minded individuals can foster learning opportunities and enhance your scripting proficiency.