The `ForEach-Object` cmdlet in PowerShell allows you to process each item in a pipeline, and using the `Continue` statement within it can skip the current iteration and continue with the next object in the loop.
Here’s an example code snippet demonstrating how to use `ForEach-Object` along with a `Continue` statement:
1..10 | ForEach-Object {
if ($_ -eq 5) { continue }
Write-Host "Processing number: $_"
}
Understanding ForEach-Object in PowerShell
What is ForEach-Object?
ForEach-Object is a powerful cmdlet in PowerShell that allows you to process each item in a collection, such as an array or a list, one at a time. It plays a crucial role in scripting automation by enabling the application of commands to each individual object. This cmdlet is integral to working with pipelines, making it a flexible tool for both simple and complex data manipulations.
Syntax of ForEach-Object
The basic structure of the ForEach-Object cmdlet is as follows:
$array | ForEach-Object { <script block> }
In this syntax:
- `$array`: The collection of items you wish to process.
- `<script block>`: The actions you want to perform on each item.
Parameters such as `-InputObject` allow for targeting specific collections, while `-Begin`, `-Process`, and `-End` enable segmented execution, adding even more control over data processing.
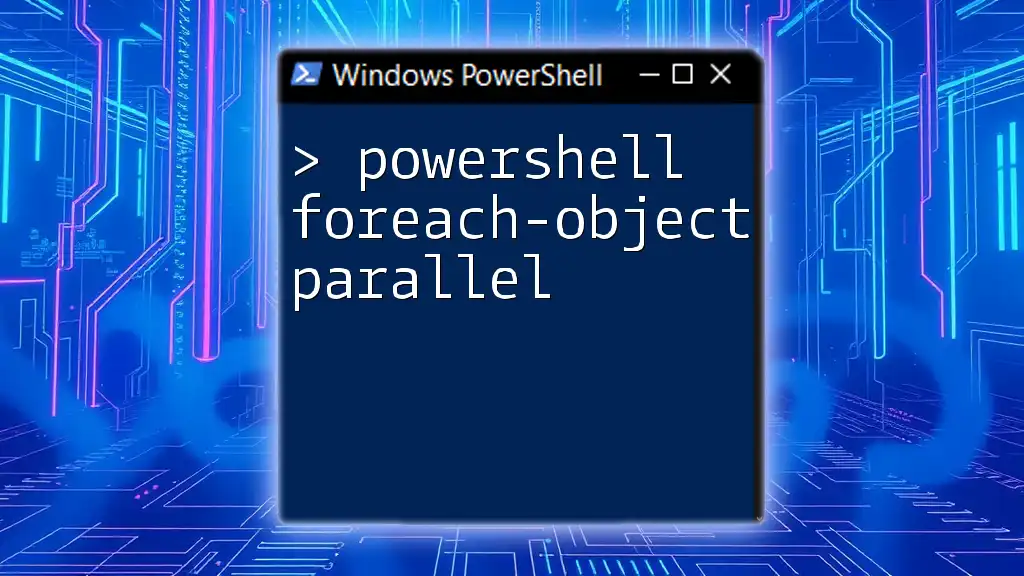
The "Continue" Statement in PowerShell
What Does "Continue" Mean?
The `Continue` keyword in PowerShell is used within loop constructs to skip the remaining statements in the current iteration and move directly to the next loop iteration. This is particularly useful in scenarios where certain conditions render further processing unnecessary or undesirable.
Using "Continue" with ForEach-Object
When utilized with ForEach-Object, the `Continue` statement provides a streamlined approach to manage item processing. It allows you to specify conditions under which certain objects should be overlooked, enhancing both efficiency and clarity in your scripts.
Practical Applications of ForEach-Object Continue
Example 1: Filtering Data
Consider a scenario in which you have a collection of user accounts, and you want to filter out any inactive accounts from processing. Here's how to apply `Continue` effectively:
$users = @("ActiveUser1", "InactiveUser1", "ActiveUser2", "InactiveUser2")
$users | ForEach-Object {
if ($_ -like "Inactive*") {
Continue
}
Write-Host "Processing $_"
}
In this example, the code checks each user. If a user’s name starts with Inactive, it uses `Continue` to skip to the next iteration, preventing any further processing of that user.
Example 2: Error Handling
Imagine a situation where you're importing data and some entries might throw errors during processing. Using `Continue` allows your script to move past problematic entries without breaking the entire operation:
$data = @("Item1", "Item2", "ErrorItem", "Item3")
$data | ForEach-Object {
try {
if ($_ -eq "ErrorItem") {
Throw "Error processing $_"
}
Write-Host "Successfully processed $_"
} catch {
Write-Host "Error encountered, skipping $_"
Continue
}
}
Here, when `ErrorItem` is encountered, the script captures it, informs the user, and uses `Continue` to skip processing that item while still allowing the other valid items to be processed.
Example 3: Speeding Up Processing
In scenarios with large data sets, enhancing performance is key. The use of `Continue` can streamline operations significantly:
$largeDataset = 1..1000
$largeDataset | ForEach-Object {
if ($_ % 2 -eq 0) {
Continue # Skip even numbers
}
Write-Host "Processing odd number $_"
}
This script focuses purely on odd numbers from a massive range, skipping unnecessary processing for even numbers and optimizing execution time.
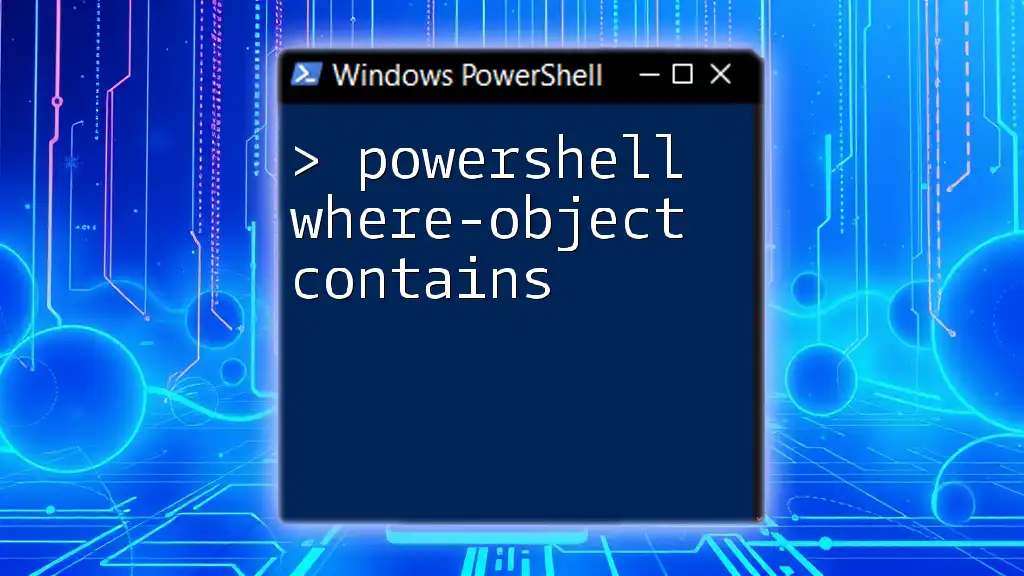
Best Practices for Using ForEach-Object and Continue
Writing Clean and Efficient Code
Ensuring readability and maintainability in your scripts is essential. Break down complex operations using comments and clear logic to indicate where.
Error Management Strategies
Using `Continue` as an error management tool can minimize disruptions in your scripts. Consider logging errors or tracking skipped items for better visibility.
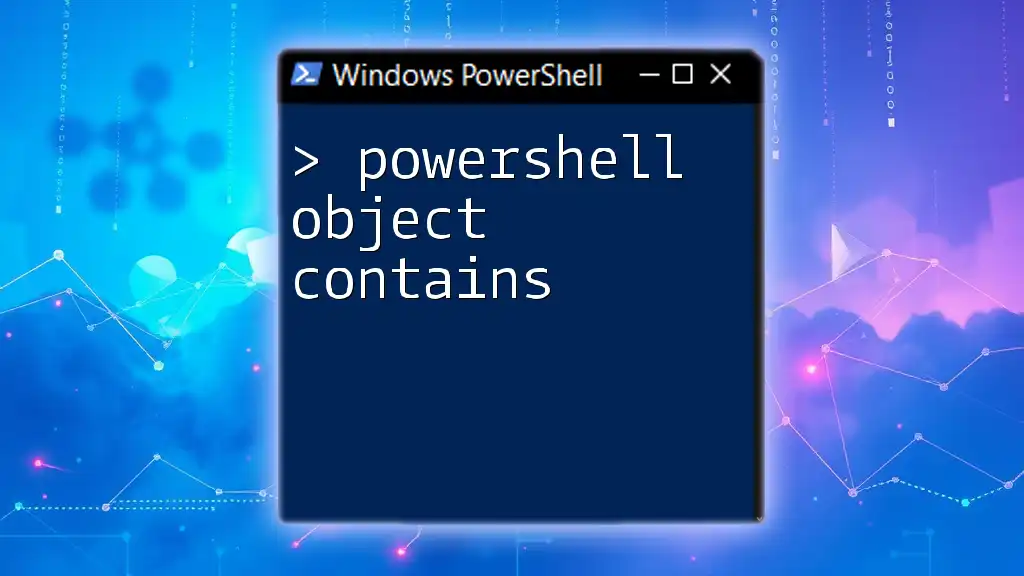
Performance Considerations
Comparing ForEach-Object vs. ForEach
While both ForEach-Object and ForEach serve similar functions, their usage context matters. ForEach-Object is typically utilized when dealing with large collections in a pipeline, while ForEach is better suited for iterating through a fixed collection in memory.
When to Choose ForEach-Object over ForEach
Choose ForEach-Object when:
- You are working with pipelines.
- You need to process data on-the-fly. Conversely, use ForEach for simpler iterations where performance is not hampered by memory constraints.
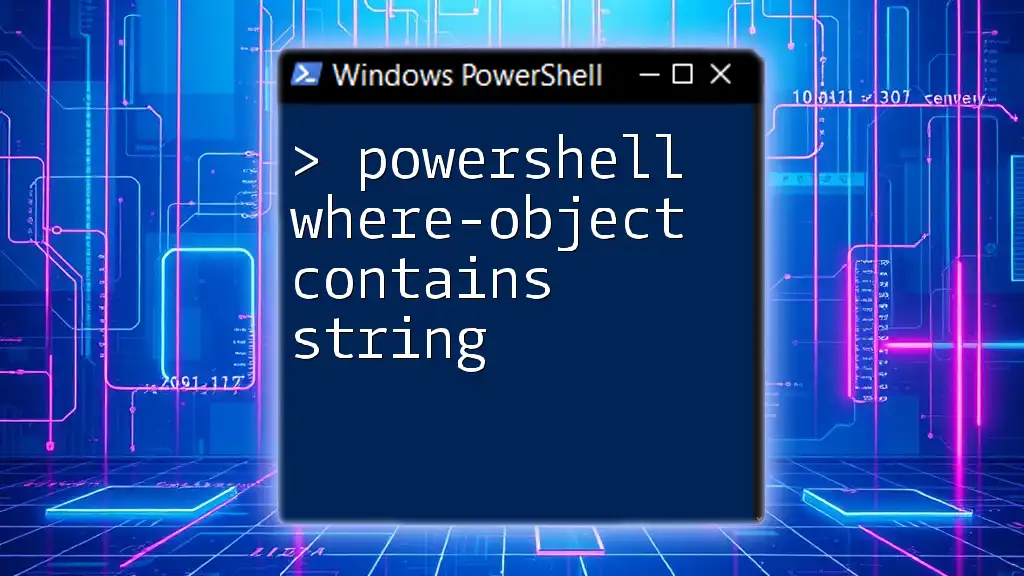
Debugging and Troubleshooting Common Issues
Common Errors with ForEach-Object
Errors often arise from object handling. Misusing the `$_` variable (which refers to the current object) can lead to runtime errors. Pay attention to where `Continue` is implemented to avoid overlooking critical runtime warnings.
How to Validate the Effectiveness of "Continue"
To ensure `Continue` operates properly, implement logging or verbose output to show which items were skipped. This validation can help confirm your conditions align with expected outcomes.
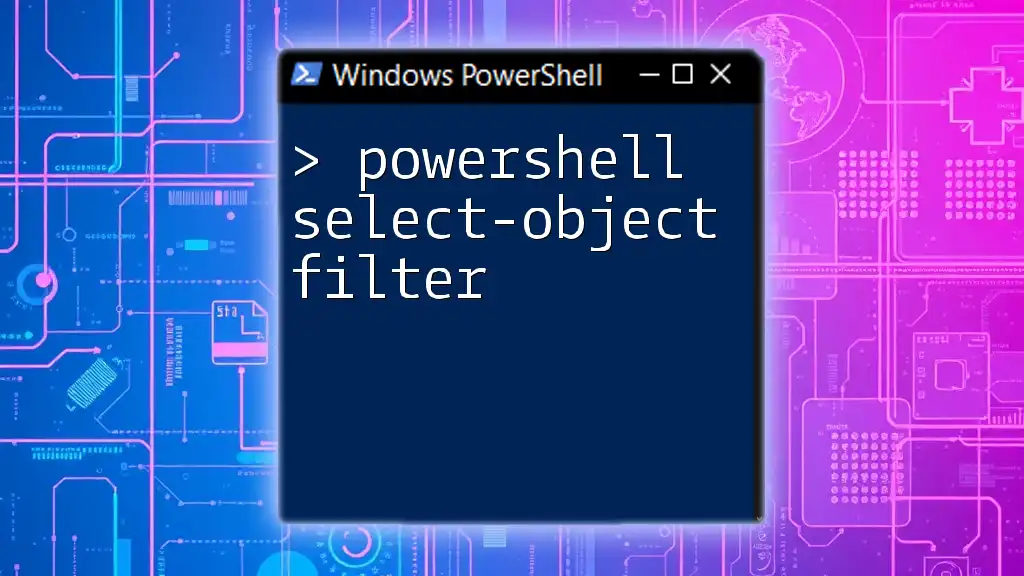
Conclusion
Using the PowerShell ForEach-Object Continue command can dramatically enhance the effectiveness and efficiency of your scripting practices. By understanding and implementing these principles, you're on your way to becoming a proficient PowerShell user.
Encouragement to Practice
Implement these strategies in your scripts to see tangible improvements. Remember, practice is crucial in mastering PowerShell, and leveraging tools like ForEach-Object and Continue is a step towards advanced automation solutions.
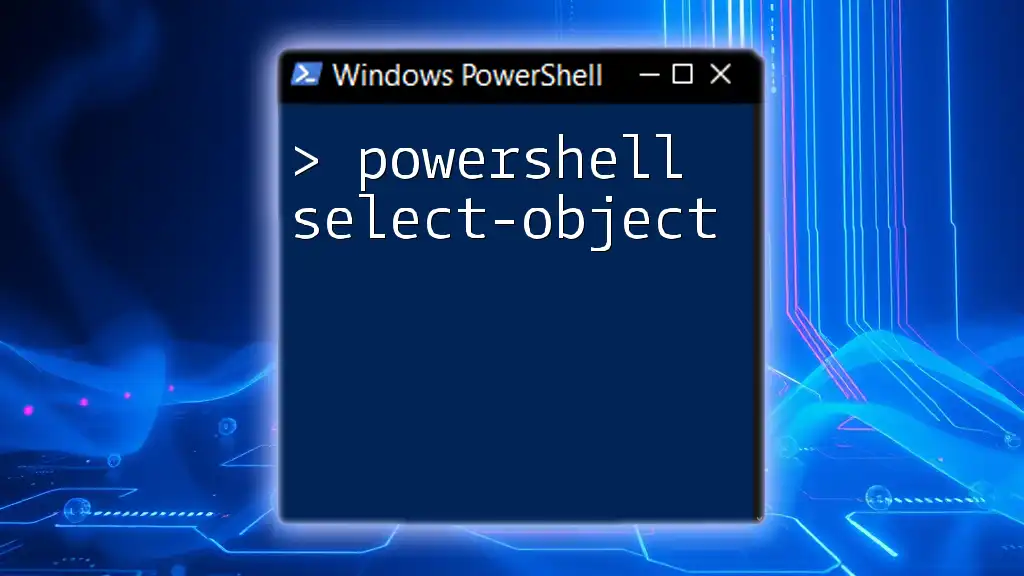
Additional Resources
Links to PowerShell Documentation
Explore official documentation at Microsoft Docs for insights into further cmdlet functionalities and best practices.
Recommended Books and Courses
Investigate books and online courses tailored towards PowerShell to deepen your understanding and skills in scripting effectively.