The `Where-Object` cmdlet in PowerShell is used to filter objects based on specific conditions, such as checking if a property contains a particular value, which can be achieved using the `-contains` operator within a script.
Here's a code snippet demonstrating its usage:
Get-Process | Where-Object { $_.Name -contains 'notepad' }
Understanding PowerShell and Where-Object
What is PowerShell?
PowerShell is a versatile task automation framework from Microsoft that combines a command-line shell and an associated scripting language. It is designed primarily for system administrators and power users to manage and automate the administration of Windows and applications. This powerful tool offers a rich set of commands and functionalities that make interacting with the Windows operating system more efficient.
The Role of Where-Object in PowerShell
The `Where-Object` cmdlet is a critical component in PowerShell that allows for the filtering of data as it flows through the pipeline. By enabling users to specify conditions that data must meet to be included in the output, `Where-Object` ensures that only the relevant information is processed.

Using Where-Object: The Basics
Syntax of Where-Object
The syntax of the `Where-Object` cmdlet is straightforward, allowing users to filter objects based on their properties. This cmdlet takes a script block as input, inside which you can define the conditions.
Here’s the basic syntax:
Where-Object { <condition> }
For example, to get all processes whose name starts with "powershell," you would use:
Get-Process | Where-Object { $_.Name -like 'powershell*' }
How Where-Object Works
When you pipe data to `Where-Object`, it processes each object in the pipeline and evaluates the specified condition within the script block. If the condition returns True, the object is included in the output; if it returns False, the object is excluded. This pipeline concept of PowerShell allows for efficient data manipulation.
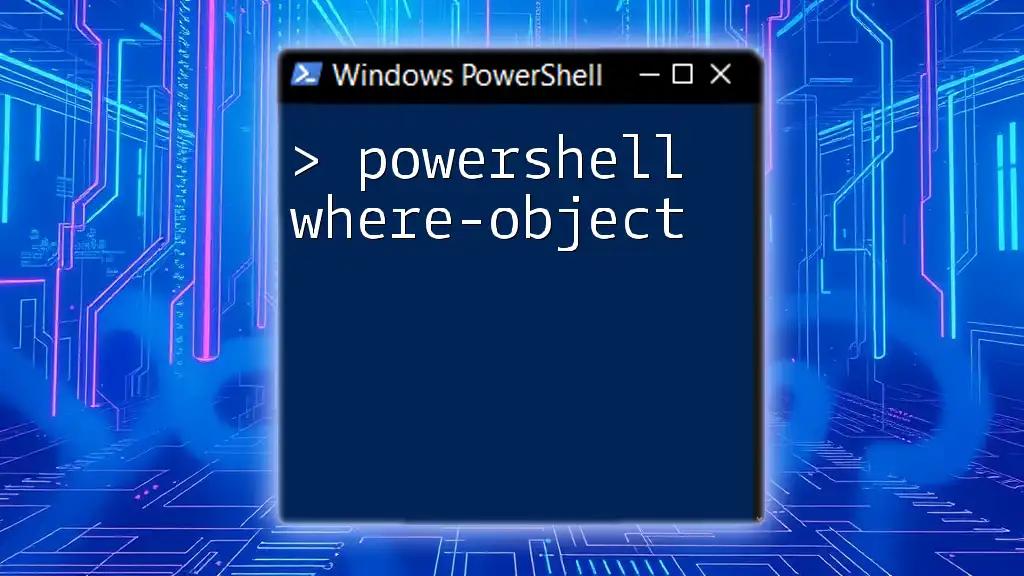
The Contains Operator in Where-Object
What Does "Contains" Mean in PowerShell?
In PowerShell, the `-contains` operator checks if an array includes a specific item. It can be incredibly useful when dealing with collections, enabling you to filter objects based on their membership within an array.
Using the Contains Operator
The `-contains` operator is often used within the `Where-Object` cmdlet to filter out items that match a particular element in a collection.
For example, if you have an array and want to find out if it contains the number `3`, you can write:
$array = 1, 2, 3, 4, 5
$array | Where-Object { $_ -contains 3 }
In this case, the output will confirm that the value `3` exists within the array.

Filtering Strings with Where-Object: The Like Operator
Overview of the Like Operator
The `-like` operator in PowerShell is used for string comparison, utilizing wildcard characters to offer flexible matching criteria. The asterisk (`*`) represents zero or more characters, while the question mark (`?`) represents a single character.
Combining Where-Object with the Like Operator
By combining `Where-Object` with the `-like` operator, users can perform powerful searches through strings. For example, if you want to filter services that include "SQL" in their display name, you can use:
Get-Service | Where-Object { $_.DisplayName -like '*SQL*' }
This command will return all services whose display names contain the substring "SQL."

Combining Conditions for Advanced Filtering
Using Multiple Criteria
PowerShell allows you to combine multiple conditions in a single `Where-Object` statement, enabling more refined data filtering. You can use logical operators like `-and`, `-or`, and `-not` to construct complex queries.
For example, to find processes that use more than `50` CPU resources and whose names begin with "powershell," your command would look like:
Get-Process | Where-Object { $_.CPU -gt 50 -and $_.Name -like 'powershell*' }
Using the Contains Operator with Arrays
You can also utilize the `-contains` operator with nested arrays to filter collections containing specific elements. For instance, if you have an array of "critical processes" and want to find those in your running processes, you could use:
$processes = Get-Process
$criticalProcesses = 'explorer', 'powershell'
$processes | Where-Object { $_.Name -in $criticalProcesses }
This command helps determine if any of the running processes are considered critical.
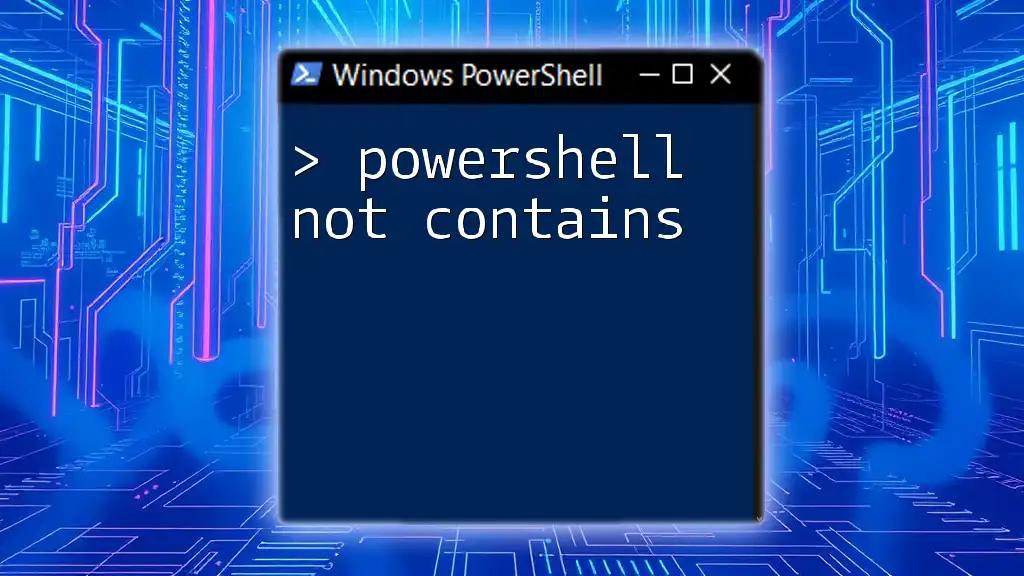
Performance Considerations
Performance Impact of Where-Object
When working with large datasets, the performance of `Where-Object` can become a consideration. Since it evaluates each object in the pipeline, efficiency may decline if many objects are being processed.
To mitigate performance issues, consider filtering data as early as possible in the pipeline, which reduces the number of objects that `Where-Object` has to evaluate. For example, filtering broad sets at the source rather than after all data is loaded offers significant performance improvements.
When to Avoid Using Where-Object
Situations arise where using `Where-Object` is not the best choice. For instance, using `Where-Object` with a complex expression on a large dataset can be slow. In such cases, consider retrieving only necessary data upfront with more specific cmdlets before filtering.
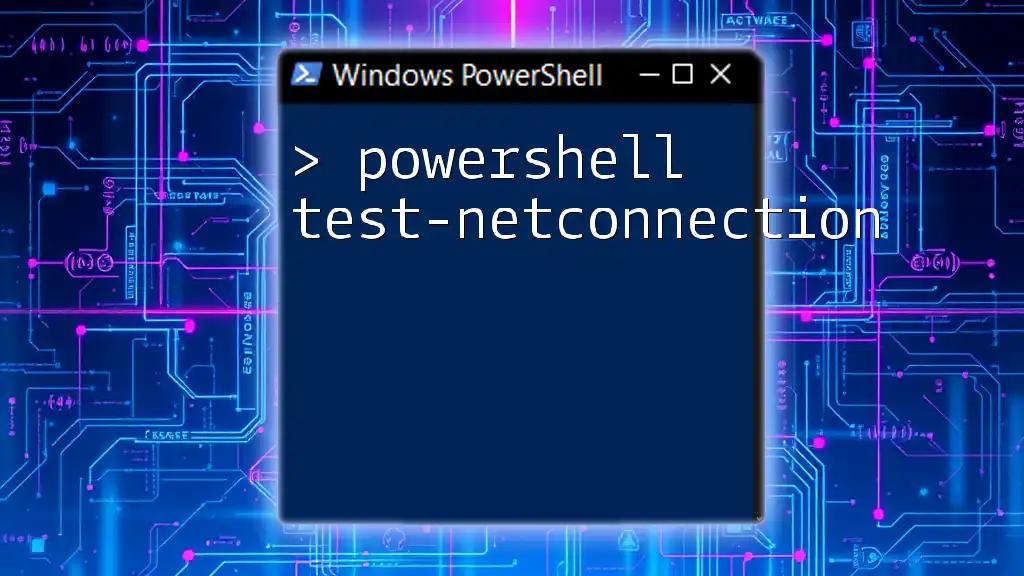
Best Practices and Common Pitfalls
Best Practices for Using Where-Object
To maximize the effectiveness of `Where-Object`, consider the following best practices:
- Use clear conditions: Ensure conditions are easy to understand.
- Comment your code: Include comments that explain complex statements.
- Limit data upfront: Retrieve only necessary objects to improve performance.
Common Pitfalls
Common mistakes include:
- Forgetting to reference the current object using `$_`, leading to unexpected results.
- Using the wrong operator for string matching, which could result in inaccurate filtering. Always debug and test your filtering commands to ensure they are functioning as intended.

Conclusion
Recap of Key Points
In this exploration of `Where-Object` and the `-contains` operator, we have seen how to filter data effectively in PowerShell. Armed with syntax, usage examples, and performance considerations, you're now equipped to leverage these commands in your daily scripting tasks.
Encouragement to Practice
To solidify your understanding, I encourage you to practice with various filtering scenarios using the examples provided. Experiment with different properties and operators, and don’t hesitate to push the boundaries of what you can accomplish with PowerShell.
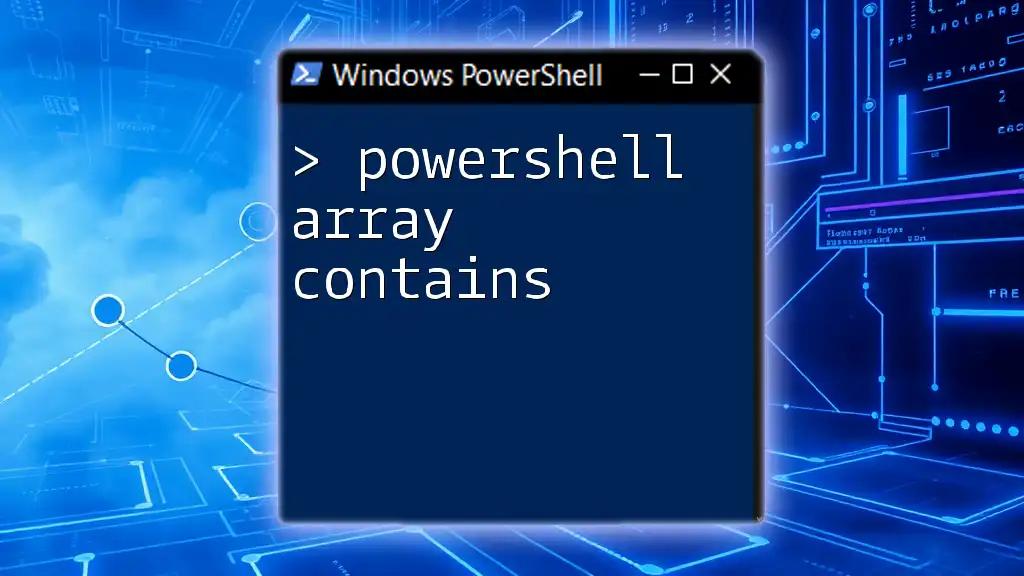
Further Reading and Resources
For further exploration into PowerShell concepts, I recommend consulting official Microsoft documentation and seeking out books or courses dedicated to advanced PowerShell usage. The learning path in PowerShell is vast, and continual learning will enhance your script-writing capacity.