In PowerShell, the `ForEach-Object` cmdlet allows you to perform an operation on each item in a collection or array of objects, making it easy to process data elements efficiently in a pipeline.
Here's a quick code snippet demonstrating its usage:
$numbers = 1..5
$numbers | ForEach-Object { Write-Host "Number: $_" }
Understanding PowerShell Objects
PowerShell objects are the foundation of the PowerShell scripting environment. Unlike plain text or unstructured data, PowerShell objects encapsulate data in a structured format, enabling you to easily manipulate and interact with it. Objects can represent a wide range of entities, including:
- Cmdlet outputs, such as results from `Get-Service` or `Get-Process`.
- Custom objects created using the `New-Object` cmdlet or `[PSCustomObject]`.
Utilizing objects allows you to access their properties and methods, making it essential for powerful scripting and automation. In PowerShell, virtually everything is an object, and understanding how to leverage these objects is crucial for effective scripting.

What is `ForEach-Object` in PowerShell?
The `ForEach-Object` cmdlet is a powerful tool that processes each item in a pipeline as it comes in. Unlike the traditional `foreach` loop, which iterates over a collection, `ForEach-Object` allows for more fluid, real-time processing of data as it flows through the pipeline. This makes it especially useful for handling streaming data.
Use Cases for `ForEach-Object`
`ForEach-Object` is commonly utilized in scenarios such as:
- Processing command output in real time.
- Executing operations that involve interacting with each object in a collection.
- Performing bulk actions without having to store intermediate results in variables.

Basic Syntax of `ForEach-Object`
Understanding the syntax of `ForEach-Object` is essential for proper implementation. The basic structure is as follows:
ForEach-Object -InputObject <input> -Process { <scriptblock> }
The key parameters of `ForEach-Object` include:
- `-InputObject`: Specifies the objects to process.
- `-Process`: Accepts a script block that defines the action for each object.
Example Code Snippet
A simple yet effective example of `ForEach-Object` in action is as follows:
Get-Process | ForEach-Object { $_.Name }
In this example, `Get-Process` retrieves the list of processes currently running, and `ForEach-Object` is used to return just the names of those processes.

Using `ForEach-Object` in PowerShell Cmdlets
Chaining Cmdlets
One of the powerful aspects of `ForEach-Object` is the ability to chain cmdlets together. This means that you can pipe the output of one cmdlet into `ForEach-Object` and perform further actions.
Get-Service | ForEach-Object { $_.Status }
Here, you fetch all services and output their status. Inside the process block, `$_` represents the current object (a service in this case), allowing you to easily access its properties.
Accessing Properties and Methods
With `ForEach-Object`, you can access any property or call any method of the object. For example, if you want to check only for services that are running:
Get-Service | ForEach-Object {
if ($_.Status -eq 'Running') {
Write-Output "$($_.Name) is running"
}
}
This process filters through services and outputs only those that are actively running.
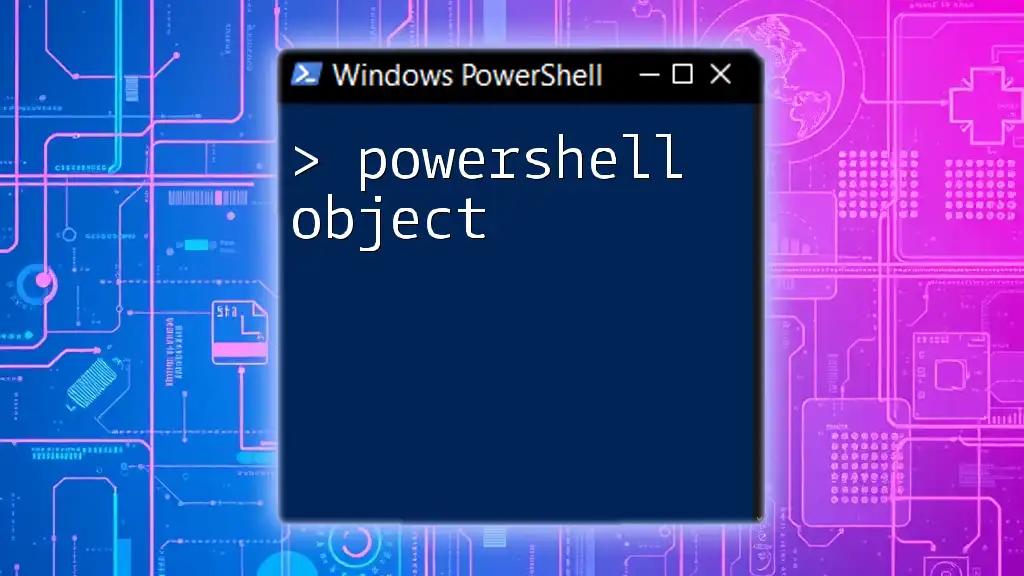
Advanced Usage of `ForEach-Object`
Utilizing ScriptBlocks
Script blocks are collections of code that can be executed together. They are beneficial when you have complex logic to apply to each object. Here’s how you can use a script block with `ForEach-Object`:
$services = Get-Service
$services | ForEach-Object {
if ($_.Status -eq 'Running') {
Write-Output "$($_.Name) is running"
}
}
In this example, you create a collection of services and use a script block to check if they are running.
Using `-Begin`, `-Process`, and `-End` Blocks
`ForEach-Object` supports additional parameters: `-Begin`, `-Process`, and `-End`. This feature allows you to define code to execute before processing, during processing, and after processing, respectively.
$array = 1..5
$array | ForEach-Object -Begin { "Start of processing:" } -Process { $_ * 2 } -End { "End of processing." }
This example starts with a message, doubles each number in the array, and ends with a completion message.
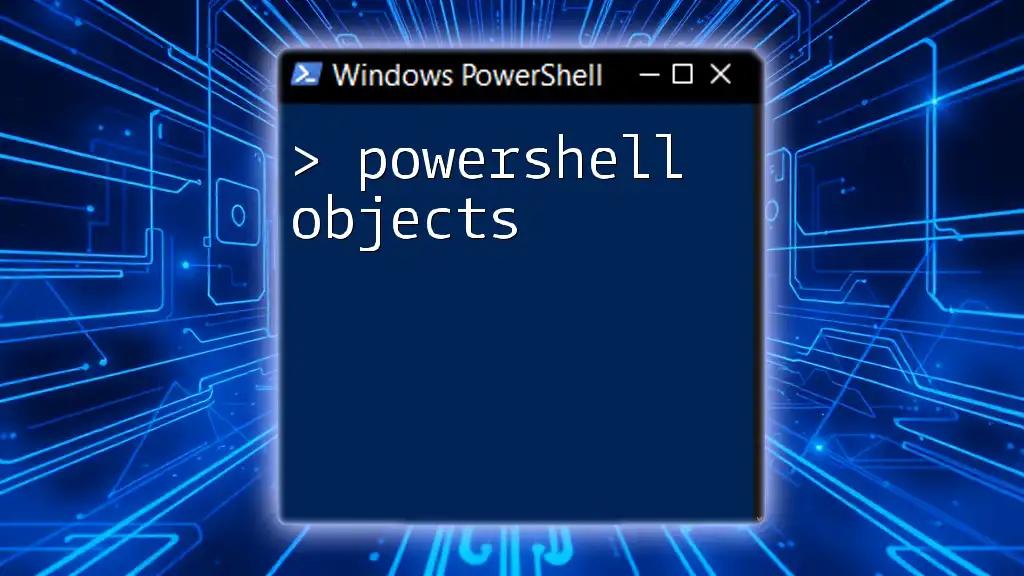
Performance Considerations
While `ForEach-Object` is versatile, it's essential to understand scenarios where it may not be the most efficient option. The traditional `foreach` loop can be more performant for large datasets where all data is available before processing. This is because `ForEach-Object` processes items one at a time as they come through the pipeline, adding overhead.
However, if you’re dealing with streaming data or want to process items on-the-fly, `ForEach-Object` is the superior choice.
Optimizing Performance
To ensure optimal performance while using `ForEach-Object`, consider the following tips:
- Only use it when necessary; opt for `foreach` when processing an array of known size.
- Limit the complexity inside the script blocks.
- Avoid long-running operations within the pipeline.

Error Handling with `ForEach-Object`
Error handling is a critical aspect of robust scripting. You can handle errors within `ForEach-Object` using try-catch blocks:
Get-Item "C:\path\to\files\*" | ForEach-Object {
try {
Remove-Item $_.FullName
} catch {
Write-Error "Failed to remove $($_.FullName): $_"
}
}
In this example, each file in the specified path is attempted to be removed, and if an error occurs, it is caught and reported without stopping the entire process. This kind of error handling is invaluable for scripts intended to run unattended.
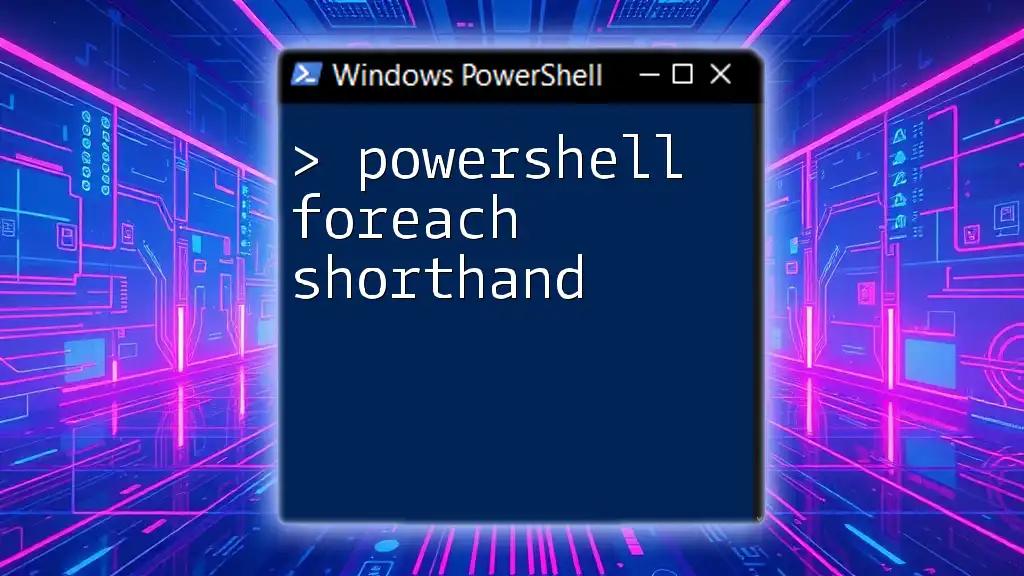
Conclusion
The PowerShell Object ForEach mechanism, specifically through the use of `ForEach-Object`, is a crucial concept for efficiently processing and manipulating data within the PowerShell environment. Recognizing how to use this cmdlet, combined with the understanding of PowerShell objects, will enhance your scripting capabilities and automate tasks effectively.
As you dive deeper into PowerShell, practicing these concepts through hands-on exercises will solidify your understanding. For further tutorials and resources, be sure to explore our website – your journey to becoming a PowerShell expert is just beginning!

Additional Resources
For those looking to expand their knowledge, refer to the official PowerShell documentation for further reading. Additional books and online courses are also excellent options to deepen your understanding of PowerShell. Joining a community forum dedicated to PowerShell can provide ongoing support as you continue to learn and experiment with new techniques.