The PowerShell command to retrieve the number of processor cores on your system can be executed using the following code snippet:
(Get-WmiObject -Class Win32_ComputerSystem).NumberOfLogicalProcessors
This command pulls the number of logical processors available, which effectively represents the cores your system has.
Understanding Processor Cores
What are Processor Cores?
Processor cores are the fundamental building blocks of modern CPUs. Each core can independently execute instructions, allowing for parallel processing and significantly increasing the computer’s performance. In simpler terms, more cores generally mean that your computer can handle more tasks at once, leading to smoother multitasking and improved performance for demanding applications.
Why Retrieve Core Information?
Retrieving core information is essential for several reasons:
- Performance Monitoring: Understanding how many cores are available helps assess your system's capacity to run multiple processes efficiently.
- Resource Management: IT professionals can utilize core data for optimizing workloads and ensuring that applications have the necessary resources to perform optimally.
- System Diagnostics: Identifying core configurations can assist in troubleshooting performance bottlenecks or when upgrading hardware.
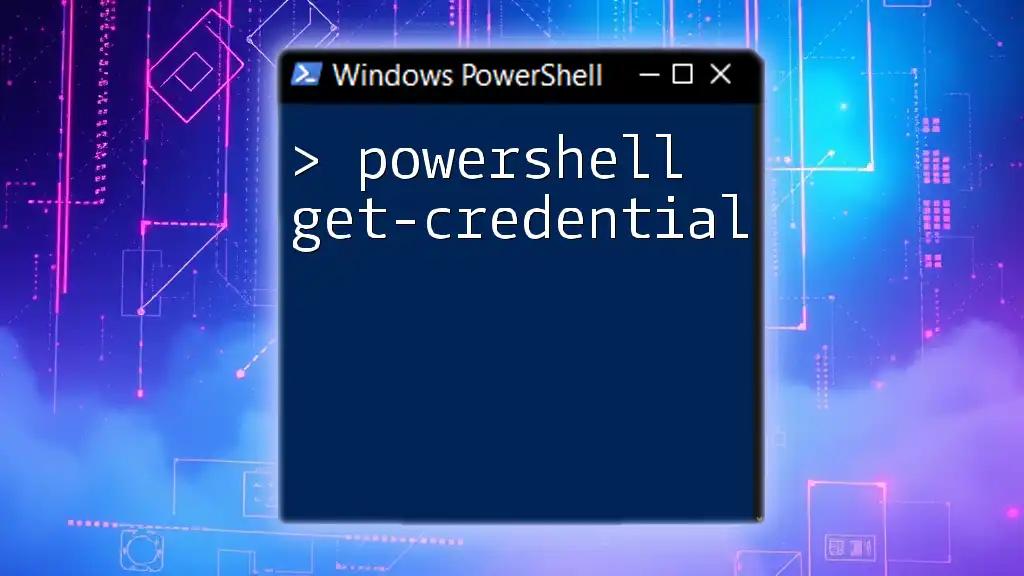
Using PowerShell to Get Processor Core Information
Basic Command Overview
In PowerShell, you can retrieve core information using commands such as `Get-WmiObject` and `Get-CimInstance`. Both commands allow access to WMI (Windows Management Instrumentation) classes, which provide detailed information about the system.
Getting Core Count Using PowerShell
Using `Get-WmiObject`:
To get the number of physical cores on your system, you can use the following command:
$cores = Get-WmiObject -Class Win32_Processor | Select-Object -Property NumberOfCores
Write-Output $cores
This command fetches details from the `Win32_Processor` class and selects the `NumberOfCores` property. The output will display the count of physical cores on your processor.
Using `Get-CimInstance`:
An alternative to `Get-WmiObject` is `Get-CimInstance`, which operates in a more efficient manner and is recommended for modern PowerShell scripts:
$cores = Get-CimInstance -ClassName Win32_Processor | Select-Object -Property NumberOfCores
Write-Output $cores
This command provides the same information but is more robust and faster, especially over network queries.
Comparison Between `Get-WmiObject` and `Get-CimInstance`: While both commands serve a similar purpose, `Get-CimInstance` is preferable because it uses the WS-Man protocol for communication, making it capable of managing remote machines more effectively.
Retrieving Logical Processor Information
In addition to physical cores, it’s important to understand logical processors, which include threads that can be run by each core. To check how many logical processors your system has, use this command:
$logicalProcessors = Get-WmiObject -Class Win32_ComputerSystem | Select-Object -Property NumberOfLogicalProcessors
Write-Output $logicalProcessors
This command pulls data from the `Win32_ComputerSystem` class, illustrating the total number of logical processors available, which is useful for assessing overall processing capabilities.
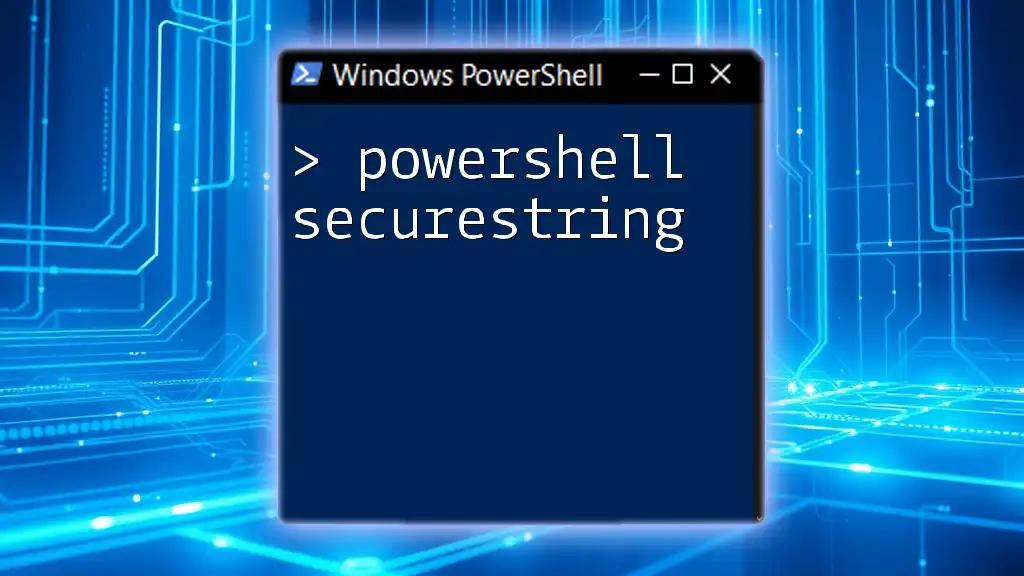
Advanced Techniques for Detailed Core Information
Exploring Processor Properties
To gain more insight into your CPU, you can retrieve comprehensive information using the following command:
Get-WmiObject -Class Win32_Processor | Format-List *
This command displays all properties associated with the CPU, allowing you to inspect important attributes like `Name`, `MaxClockSpeed`, and others. This information is crucial for understanding your processor's capabilities and limitations better.
Monitoring Core Performance
For real-time performance tracking on how effectively your cores are working, you can leverage the `Get-Counter` cmdlet. This command provides statistics on processor usage:
Get-Counter '\Processor(_Total)\% Processor Time'
The output indicates the percentage of time that the processor is actively processing data, offering valuable insights into system performance under various loads.
Filtering Specific Core Information
PowerShell allows you to filter results for specific needs. For instance, if you'd like to find processors with more than four cores, you can use the following command:
Get-WmiObject -Class Win32_Processor | Where-Object { $_.NumberOfCores -gt 4 }
This filtering helps in obtaining targeted information based on system requirements, which can be integral when preparing for upgrades or performance assessments.
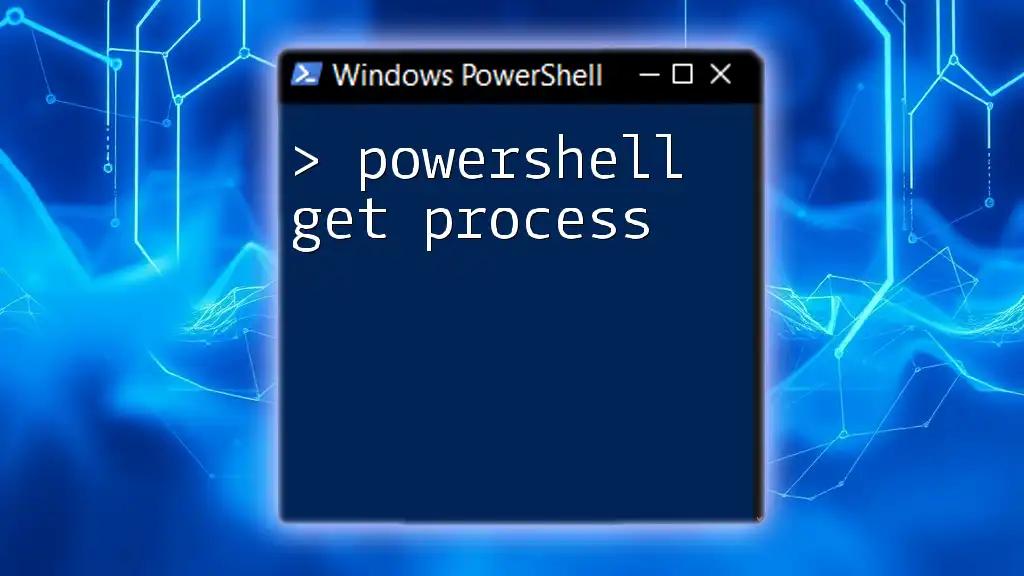
Leveraging Core Information in Scripts
Automating Core Information Retrieval
To streamline the process of retrieving core information, consider creating a reusable function. Here’s an example of such a function:
function Get-CoreInfo {
Get-CimInstance -ClassName Win32_Processor | Select-Object Name, NumberOfCores, NumberOfLogicalProcessors
}
This function allows users to easily access critical core data with a simple command.
Incorporating Core Information into System Reports
Moreover, you can automate reporting of core information. To create a CSV report documenting core data, you can use the following script:
$report = Get-CoreInfo
$report | Export-Csv -Path "C:\CoreInfoReport.csv" -NoTypeInformation
This command fetches core data using your previously defined function and exports the information to a CSV file, enabling additional analysis or sharing with team members.
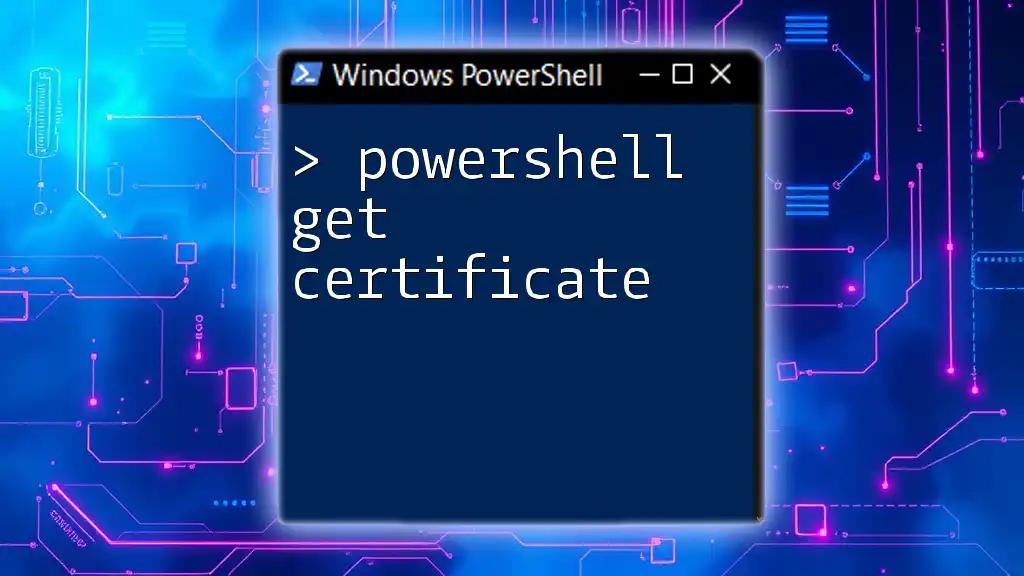
Conclusion
In summary, understanding and retrieving core information through PowerShell is vital for effective system management and performance optimization. Whether you’re monitoring resource usage, diagnosing issues, or preparing for hardware upgrades, knowing how to use PowerShell get cores effectively gives you a significant advantage. Dive deeper into PowerShell's capabilities and leverage these tools to empower your systems and enhance your productivity.