PowerShell object types define the structure and behavior of data, allowing users to interact with various data types and automate tasks effectively.
# Example of creating a simple PowerShell object
$person = New-Object PSObject -Property @{ Name = 'John'; Age = 30 }
$person
Understanding PowerShell Objects
What is an Object?
In programming, an object is a self-contained unit that encapsulates both state and behavior. State refers to its attributes (known as properties), while behavior is defined by its methods. In PowerShell, everything revolves around objects, making it essential to understand what they are and how they function. Unlike primitive data types, which represent single values (like integers or strings), objects can hold multiple values and provide functionality through methods.
Recognizing the significance of objects in PowerShell scripting is crucial, as they allow more complex data handling and manipulation. With objects, you can easily manage and interact with system resources, services, and extensive datasets in a structured manner.
The Object Model in PowerShell
PowerShell’s object model operates significantly within the .NET framework, where every component of PowerShell is an object derived from the base class `System.Object`. This relationship enables PowerShell to leverage the rich set of properties and methods provided by .NET objects, making it a powerful scripting tool.
Every object in PowerShell has properties, which store data about the object, and methods, which define actions that can be performed on the object. Understanding this relationship enables you to manipulate and interact with various types of data efficiently.
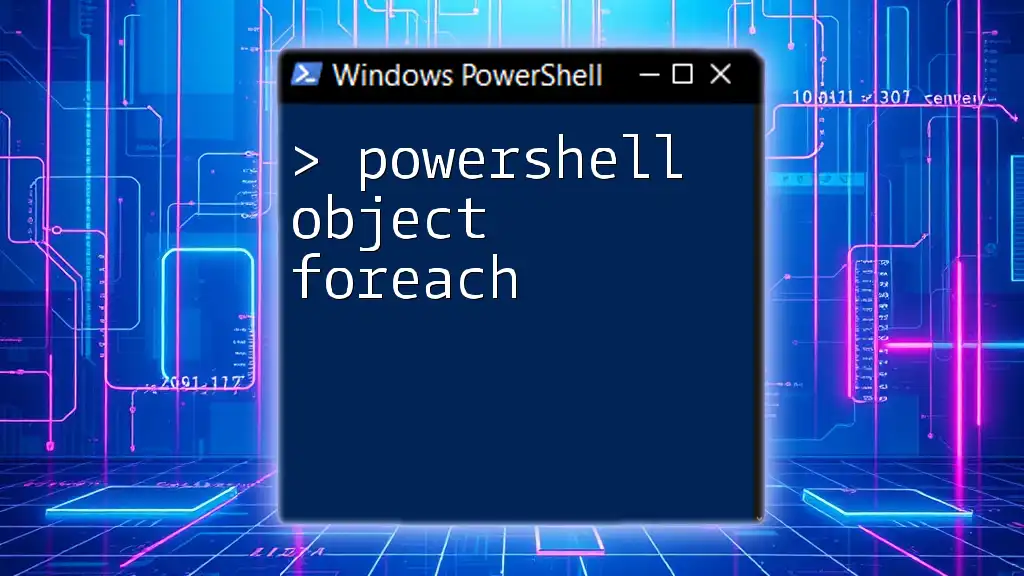
Common PowerShell Object Types
System.Object
The most basic object type in PowerShell is `System.Object`. It serves as the superclass for all objects, meaning every object you interact with in PowerShell inherits from this base type. You can create a basic object using the `New-Object` cmdlet:
$obj = New-Object System.Object
$obj
Since `System.Object` doesn’t come with any built-in properties or methods, it’s often used as a foundation for custom objects. However, it is crucial to recognize its existence as the starting point for all objects in PowerShell.
PSCustomObject
The `PSCustomObject` type is one of the most versatile and commonly used object types in PowerShell. It enables you to create custom objects that can have multiple properties defined by you. This is particularly useful for organizing complex data.
Here’s how to create a simple custom object:
$customObject = [PSCustomObject]@{
Name = "John Doe"
Age = 30
}
$customObject
Using `PSCustomObject` is beneficial because it allows for better readability and documentation of your code, providing structure and clarity to your data. With `PSCustomObject`, you can easily create collections of data where each item has the same structure.
Arrays and Collections
Arrays and collections also represent object types in PowerShell. An array is an ordered collection of items, and in PowerShell, arrays can hold multiple objects of various types.
Consider this example on creating and manipulating an array:
$array = 1, 2, 3, 4, 5
$arrayCount = $array.Count
Write-Output "Array has $arrayCount elements"
PowerShell also provides collections like Hashtables and Lists. For example, Hashtables allow you to store key-value pairs, which can be very helpful for managing configurations or settings.
Datasets and DataTables
`DataSets` and `DataTables` are powerful for managing large sets of structured data. These are particularly beneficial when you're working with relational data or require complex data operations.
Creating a `DataTable` can be done with the following code:
$dataTable = New-Object System.Data.DataTable
$dataTable.Columns.Add("ID", [int])
$dataTable.Columns.Add("Name", [string])
Utilizing `DataTables` allows you to foster advanced data manipulation and retrieval techniques, especially when interfacing with databases or processing CSV files.
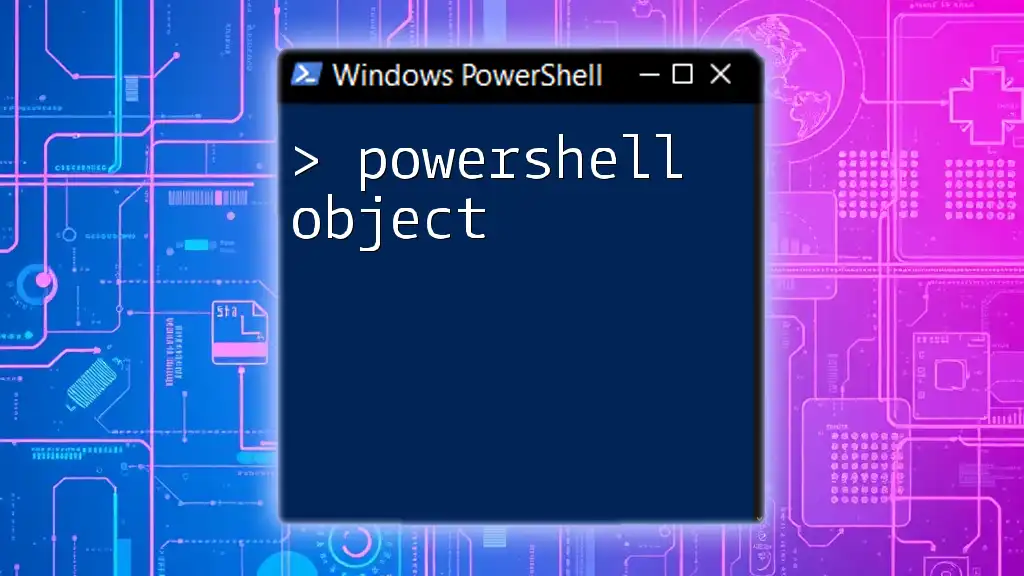
Working with Object Properties and Methods
Accessing Properties
To access the properties of an object in PowerShell, you can use the dot notation. For example, given our `PSCustomObject`:
$customObject.Name
This will output "John Doe." Being able to access and modify properties is essential for dynamically working with your data within scripts.
Using Methods
Objects also come with methods that allow you to perform actions. For instance, using methods on an array, such as `Add`, allows for dynamic list management.
Here’s an example of using the `Add` method:
$array.Add(6)
Such methods bridge the gap between the object's data and the operations applicable to that data, enhancing script functionality.
Working with the Get-Member Cmdlet
To explore the members of an object, `Get-Member` proves to be invaluable. This cmdlet reveals the properties and methods associated with any object, making it easier to understand object capabilities:
$customObject | Get-Member
This command will list all available properties and methods of the `customObject`, providing insight into how you can manipulate or utilize it effectively.
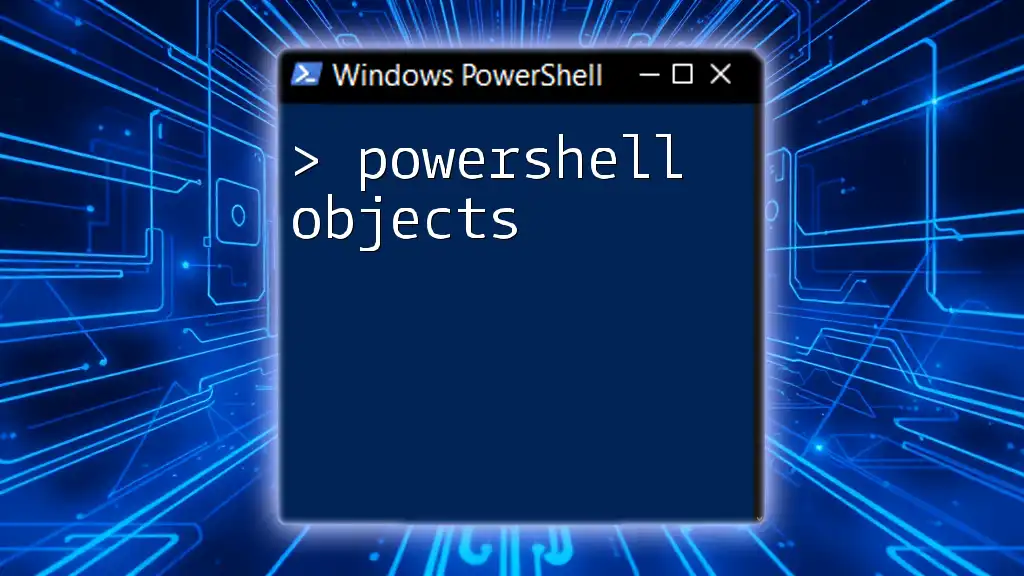
Converting Between Different Object Types
Type Casting
PowerShell handles type casting seamlessly, allowing you to convert an object from one type to another. This capability is essential when you need to ensure that your data conforms to expected formats.
For example, you can cast a simple string into a different type:
[string]$name = "StringObject"
Understanding type casting enables you to work flexibly with different forms of data, making your scripts more robust and adaptable.
Exporting Objects
PowerShell allows for easy exporting of objects into various formats, such as CSV or JSON. This feature is particularly useful for data reporting or persistence.
For instance, exporting a custom object to CSV can be done with:
$customObject | Export-Csv -Path "output.csv" -NoTypeInformation
This command ensures that the structured data is maintained when stored in flat file formats, making it accessible for further analysis.
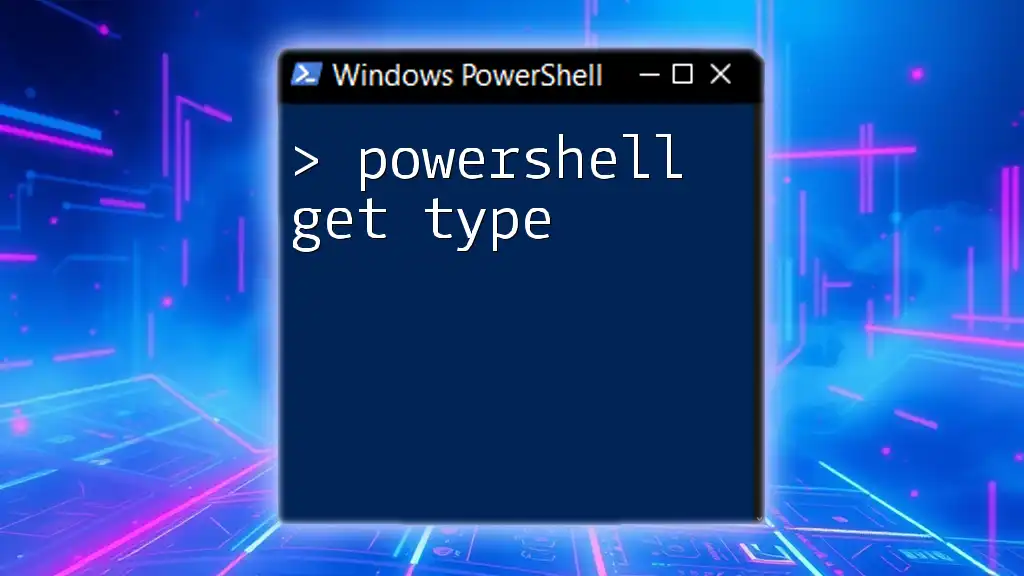
Best Practices for Working with PowerShell Objects
Use Meaningful Names
When creating objects or variables, always use meaningful names. This practice enhances the readability of your scripts and makes it easier to convey your intentions to other users or your future self.
Structuring Data
When using `PSCustomObject`, structure your data logically. Group related information together and use descriptive property names. This makes data handling more intuitive and manageable.
Efficiency in Scripting
Finally, make sure to optimize object manipulation for enhanced script performance. Using efficient coding practices—like using pipelines wisely and avoiding unnecessary loops—can significantly speed up your scripts.
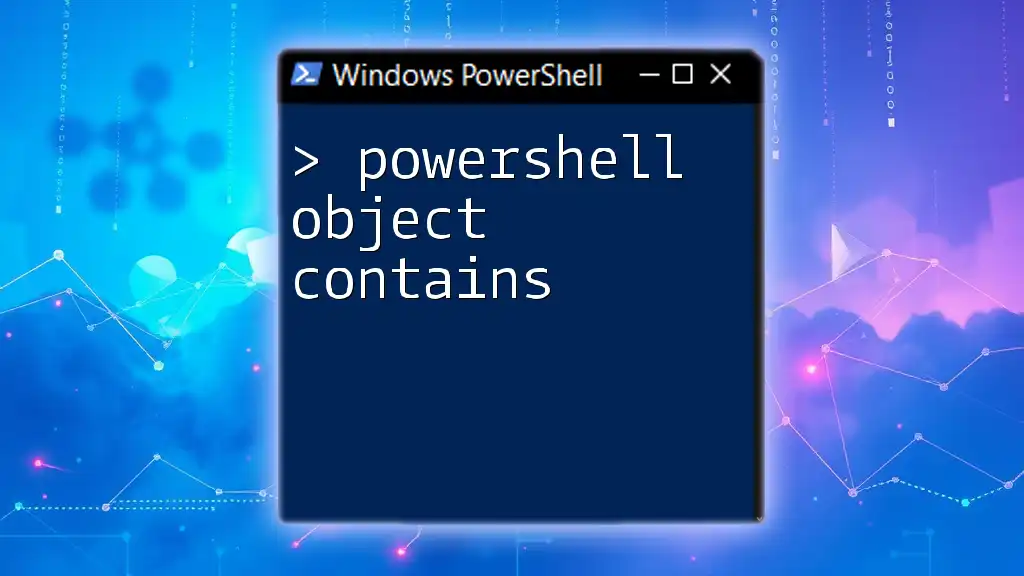
Conclusion
In PowerShell, understanding object types is fundamental to effective scripting. By leveraging the capabilities of various object types, such as `PSCustomObject`, arrays, and `DataTables`, you can craft scripts that are not only powerful but also maintainable. Always practice good coding habits and strive for clarity, as this will greatly assist you and others who may work with your scripts.
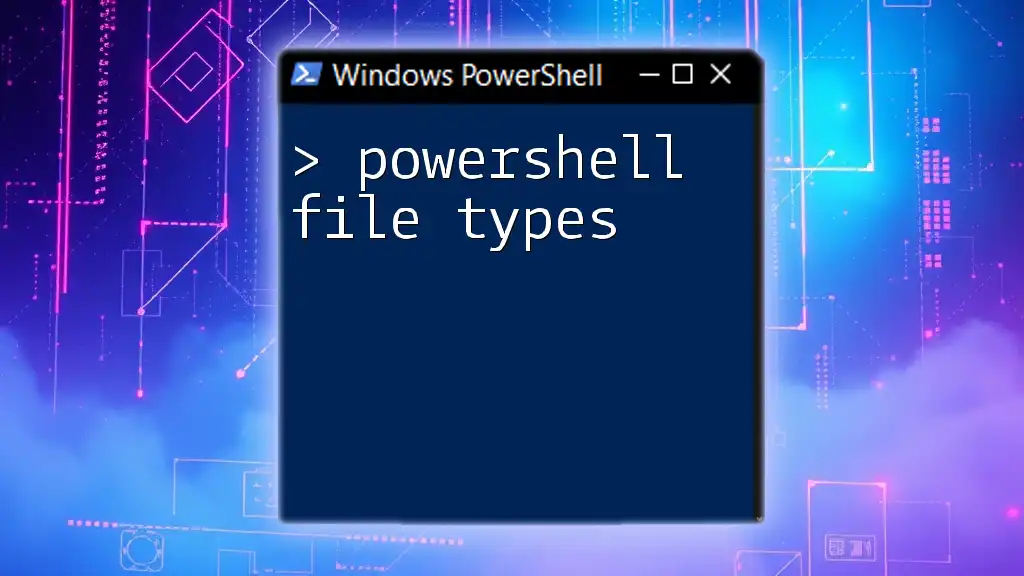
Call to Action
If you found this guide helpful, consider subscribing for more insights on PowerShell. Explore related articles or keep an eye out for upcoming tutorials that dive deeper into advanced scripting techniques.