To determine the type of an object in PowerShell, you can use the `.GetType()` method, which returns metadata about the object's type.
Here's a code snippet demonstrating this:
$object = "Hello, World!"
$object.GetType()
Understanding PowerShell Objects
What is an Object in PowerShell?
In PowerShell, an object is more than just a data type; it represents a collection of properties and methods that can be manipulated and queried. Every item in PowerShell, be it a file, a process, or even a command, is treated as an object.
- Properties are the characteristics of an object. For instance, a service object may have properties such as `Status`, `Name`, and `DisplayName`.
- Methods are actions that can be performed on an object. In the case of a service, methods might include starting, stopping, or restarting the service.
Understanding objects is fundamental in PowerShell because they form the basis of scripting and automation tasks.
The Role of Object Types
Knowing the type of an object is crucial for effective scripting. Each object type has its own properties and methods, and using the wrong assumptions about an object can lead to errors or unintended behavior. Real-world scenarios where this knowledge is pivotal include:
- Scripting: When writing scripts, knowing the object type allows you to access specific properties and methods safely.
- Automation: In automation tasks, determining whether an object is a file or a process impacts how you manipulate it.
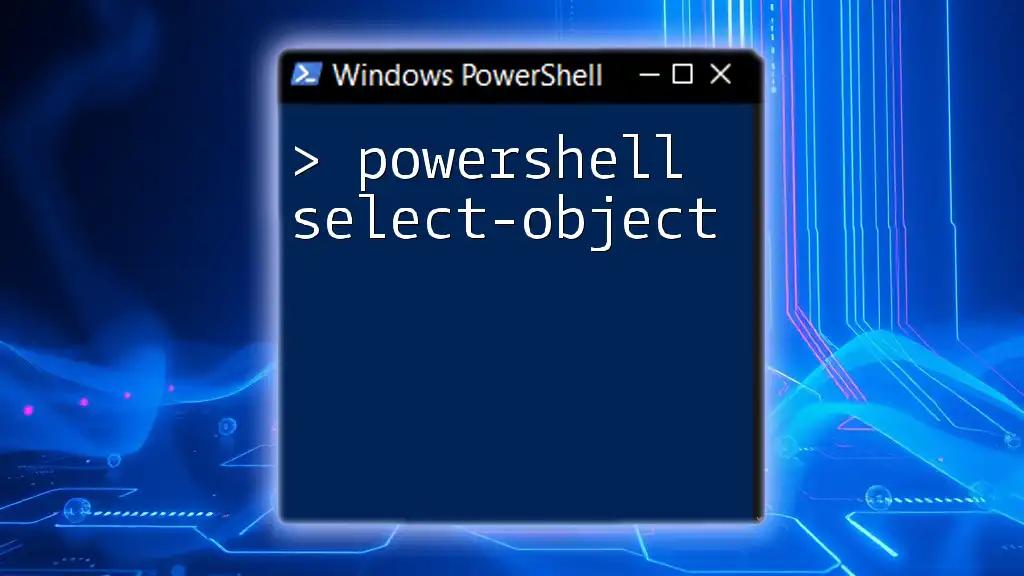
Introduction to the `Get-Type` Command
What is the `Get-Type` Command?
The `Get-Type` command in PowerShell is designed to retrieve the type of a specified object. By leveraging this command, you can gain insights into the kind of object you're dealing with, greatly improving your scripting and automation capabilities.
Why Use `Get-Type`?
The need to identify the type of an object arises in various scenarios:
- Dynamic objects: In cases where objects are created or manipulated dynamically, knowing their type helps ensure that you interact with them correctly.
- Debugging: Troubleshooting code often requires knowing the type of object you're dealing with to understand errors or unexpected outputs.
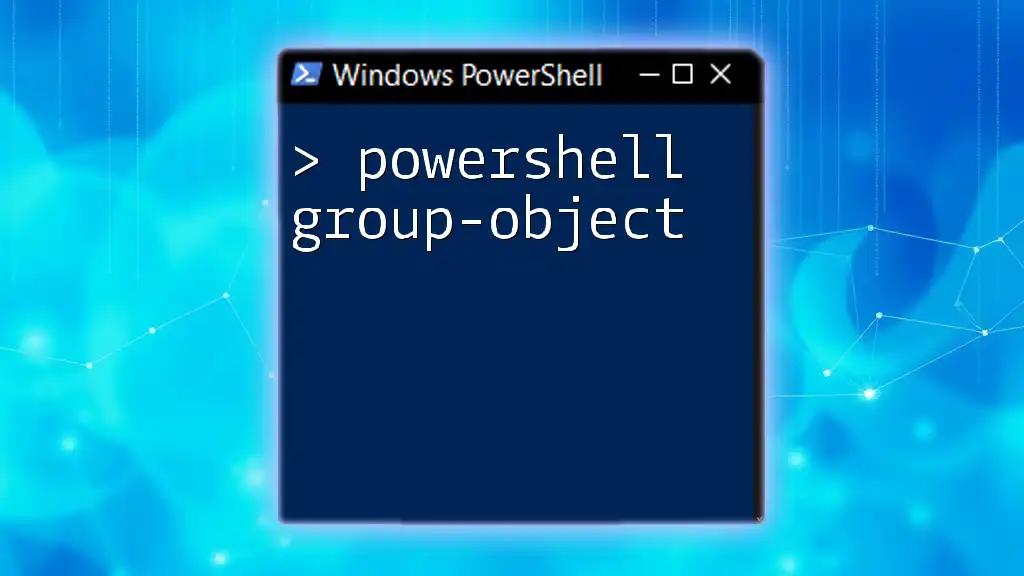
How to Use `Get-Type` in PowerShell
Basic Syntax of `Get-Type`
The basic syntax for using `Get-Type` is as follows:
Get-Type <YourObject>
In this syntax, `<YourObject>` refers to any object that you are referencing in PowerShell.
Example Usage of `Get-Type`
Here’s a simple example to illustrate how to use `Get-Type` effectively:
$object = Get-Service | Select-Object -First 1
Get-Type $object
In this snippet, we first retrieve a single service object and then use `Get-Type` to determine its type. The expected output will describe the object, such as its class name, allowing you to understand what type of object you’re dealing with.
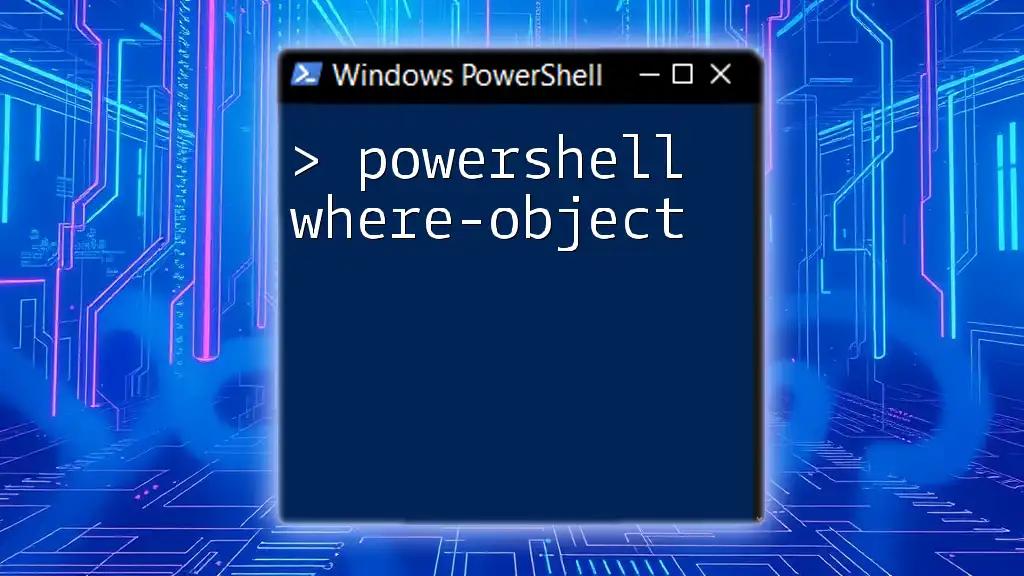
Exploring Object Types with `Get-Member`
Introduction to `Get-Member`
The `Get-Member` cmdlet is a powerful tool used for examining the properties and methods of the object. This cmdlet not only reveals the type of the object but also the capabilities it offers for manipulation.
Using `Get-Member` to Find Object Types
To inspect an object type using `Get-Member`, you can use the following code snippet:
$service = Get-Service | Select-Object -First 1
$service | Get-Member
This command will display a list of properties and methods associated with the selected service object:
- Properties will include the ones mentioned earlier like `Status` and `Name`.
- Methods might involve `Start()` and `Stop()` for managing the service.
Understanding this output is key to leveraging the full potential of the object in your scripts.
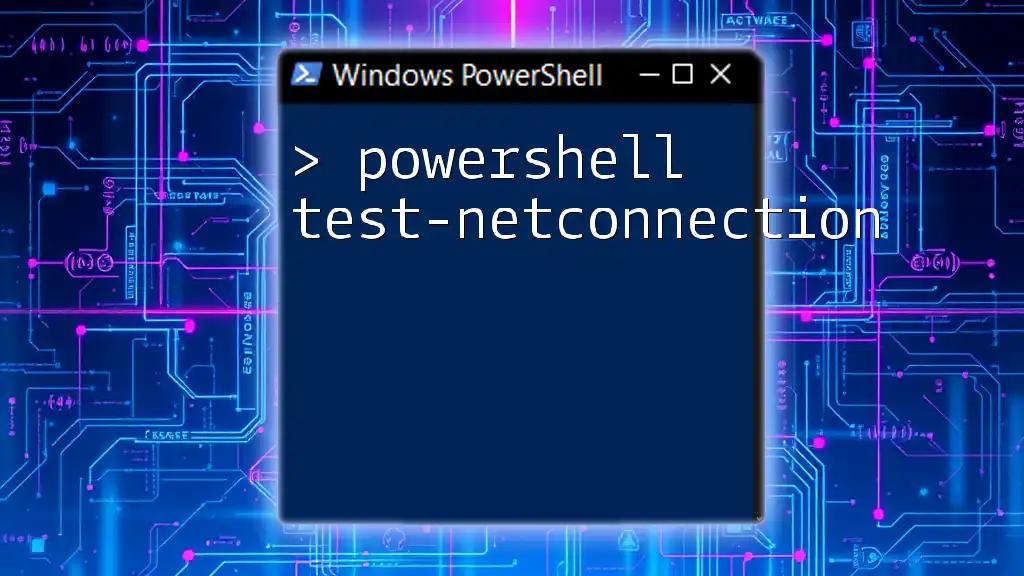
Checking Object Types Using `GetType()`
Using the `.GetType()` Method
Every object in PowerShell has an internal method called `.GetType()`, which can be invoked to retrieve information about the type of the object.
For example:
$object = "Hello, World!"
$object.GetType()
The output of this command will tell you that the object is of type `System.String`. Knowing this allows you to use string-specific methods and properties effectively.
Key Considerations
It is important to note the differences between the `GetType()` method and the `Get-Member` cmdlet:
- `GetType()` provides a quick check on the object’s type.
- `Get-Member` offers a detailed overview of the object’s properties and methods.
In some situations, you may find `GetType()` more suitable for quick checks, while in others, `Get-Member` will be necessary for deeper exploration.
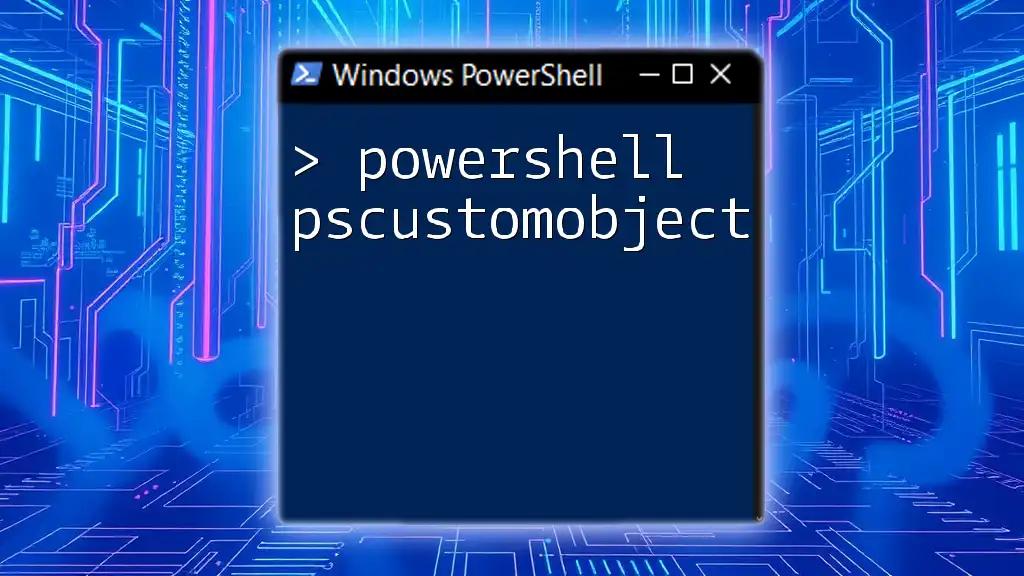
In-Built PowerShell Cmdlets for Object Type Management
Overview of Relevant Cmdlets
Other cmdlets in PowerShell can significantly aid in the management of object types:
- `Get-Command`: This cmdlet allows you to discover cmdlets related to objects, making it easier to know what command you might use for a specific task.
- `Get-Help`: If you need assistance with a specific object type command, `Get-Help` provides valuable insights into the cmdlet's usage.
Examples of Using Other Cmdlets
You can use the following command to find various cmdlets that work with objects:
Get-Command -Noun *Object
This command lists all cmdlets where the noun is related to objects, offering you quick access to a range of useful commands for object management.

Practical Tips for Managing PowerShell Objects
Best Practices for Working with Object Types
When dealing with object types, here are some best practices to follow:
- Always check object types: Before you manipulate an object, it’s wise to ascertain what type it is. This can prevent errors and lead to more robust scripts.
- Use descriptive variable names: Giving your objects meaningful names can help clarify their types and purposes when debugging or revisiting your code.
Common Mistakes to Avoid
A few misunderstandings often arise when working with object types:
- Assuming all objects of similar types share the same properties can lead to errors. Always use `Get-Member` to verify.
- Forgetting to check the type before calling a method, which can cause runtime errors if the object doesn’t support the method being invoked.

Conclusion
In this guide, we've delved into the importance of understanding how to use PowerShell to get the type of an object. By exploring various commands like `Get-Type`, `Get-Member`, and `.GetType()`, you now have the tools necessary to leverage object types strategically in your scripts.
Armed with this knowledge, practice retrieving and manipulating object types, and consider additional resources to further expand your PowerShell expertise. This foundational understanding will enhance your ability to create powerful, efficient scripts that automate tasks with confidence.