The `-ExecutionPolicy Bypass` flag allows you to run PowerShell scripts without being stopped by execution policy settings, enabling you to execute scripts easily in restricted environments.
Here's a code snippet demonstrating its use:
powershell -ExecutionPolicy Bypass -File "C:\path\to\your\script.ps1"
What is PowerShell Execution Policy?
Understanding Execution Policies
PowerShell execution policies are a security feature that determines the conditions under which PowerShell loads and runs scripts. By default, PowerShell restricts the execution of scripts to prevent unauthorized or malicious code from executing on your system. There are several types of execution policies:
- Restricted: This is the default policy; no scripts can be run.
- AllSigned: Only scripts signed by a trusted publisher can be executed.
- RemoteSigned: Scripts created locally can run, but scripts downloaded from the Internet must be signed by a trusted publisher.
- Unrestricted: All scripts can run, but there will be warnings for any scripts downloaded from the Internet.
- Bypass: No restrictions whatsoever; all scripts can run and no warnings are displayed.
- Undefined: No execution policy is defined, which defaults back to the "Restricted" policy.
Understanding these execution policies is crucial, as they enhance the security of your environment by preventing unauthorized script execution.
Importance of Execution Policies
Execution policies serve as a first line of defense against the execution of potentially harmful scripts. They help ensure that only trusted scripts are run, thereby safeguarding your system from malware and other security threats. However, these policies can also create barriers when users attempt to run legitimate scripts that are not signed or are obtained from remote sources.
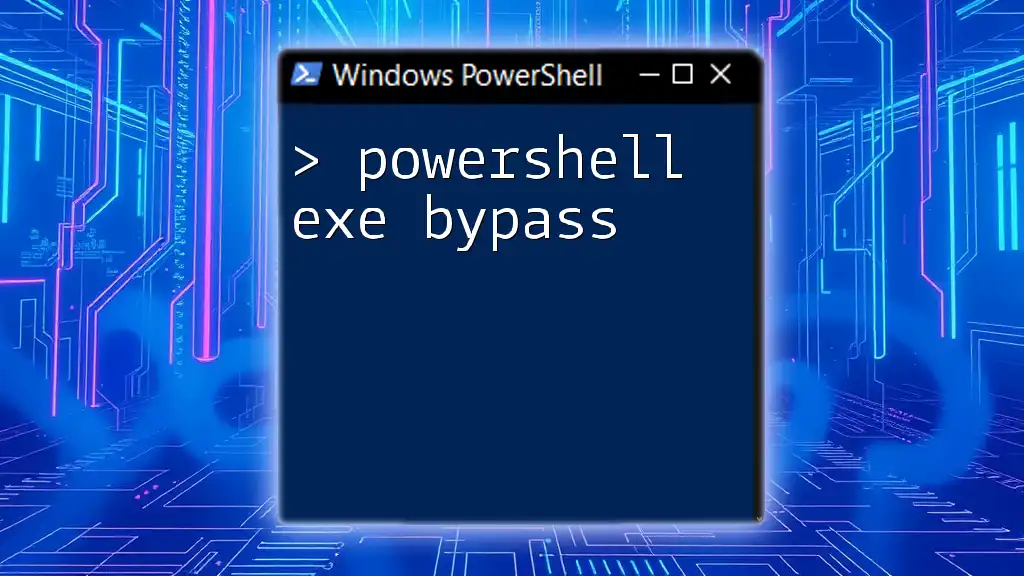
What Does `-ExecutionPolicy Bypass` Mean?
Definition of Bypass
The `Bypass` execution policy is a unique setting that allows all scripts to run without any restrictions. When the `-ExecutionPolicy Bypass` option is used when launching PowerShell, it disables the execution policy checks and alerts, thereby allowing scripts to execute freely. This can be particularly useful in scenarios where script execution is necessary, but restrictions hinder operational tasks.
When to Use `-ExecutionPolicy Bypass`
Using `-ExecutionPolicy Bypass` is appropriate in specific situations, especially when running automation scripts or administration tasks that require immediate execution. For instance, if you're deploying scripts on multiple servers where signing isn't feasible due to time constraints. While it provides flexibility, it is essential to understand that using this option removes security safeguards. Therefore, it's crucial to assess the risks involved each time you opt for it.
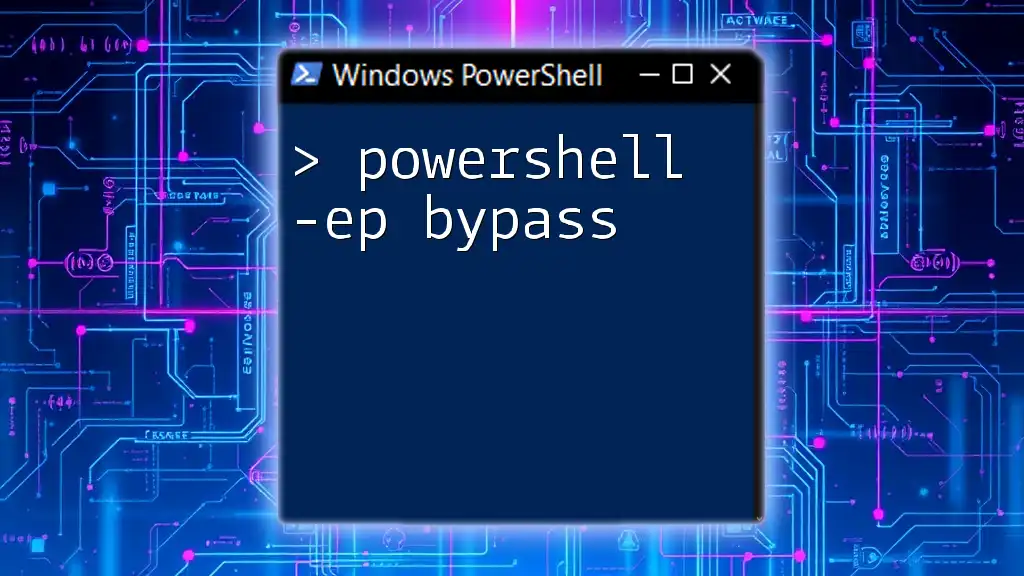
Syntax for Using `-ExecutionPolicy Bypass`
Basic Syntax
To use `-ExecutionPolicy Bypass`, the basic command structure is as follows:
powershell -ExecutionPolicy Bypass -File "path\to\your\script.ps1"
Explanation of Parameters
- `-ExecutionPolicy Bypass`: This parameter tells PowerShell to bypass the execution policy restrictions and allows the specified script to run without interruption.
- `-File`: This parameter is used to specify the path to the script file that you want to execute.
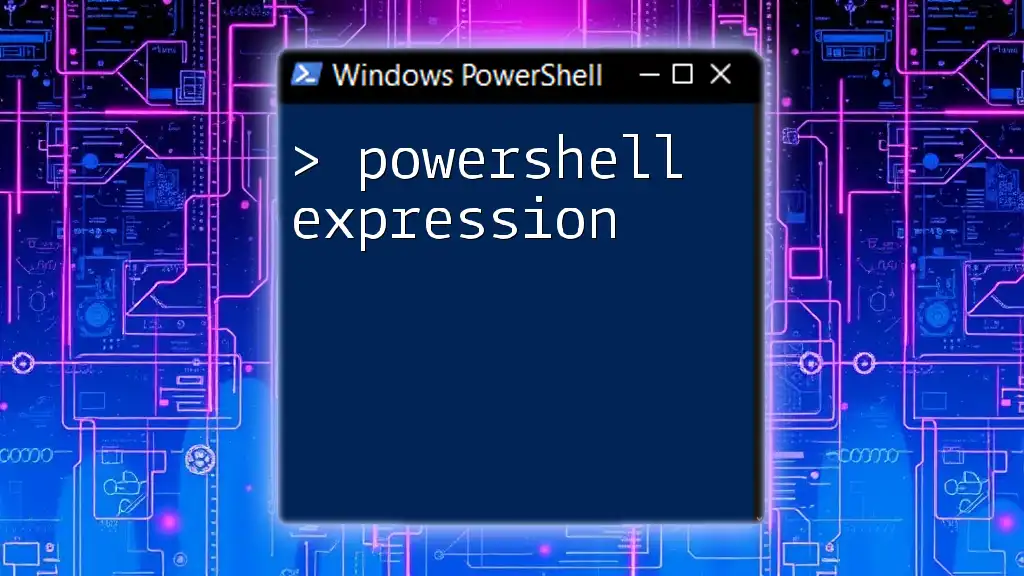
Practical Examples of Using `-ExecutionPolicy Bypass`
Example 1: Running a Local PowerShell Script
If you have a local PowerShell script that you need to run without being hindered by execution policies, you would use:
powershell -ExecutionPolicy Bypass -File ".\myLocalScript.ps1"
In this case, the local script will run immediately, provided you have the necessary permissions. This is particularly useful when testing scripts during development.
Example 2: Downloading and Executing a Script from the Internet
In some cases, you may need to download a script from the internet and execute it in a single command. Here's how you can do it:
Invoke-Expression (New-Object Net.WebClient).DownloadString("http://example.com/script.ps1")
This command downloads a PowerShell script from the specified URL and executes it immediately. While this method can be convenient, it poses significant security risks since it executes downloaded scripts without verification. Always ensure that the source is reputable.
Example 3: Using Bypass in Scheduled Tasks
Another powerful application of `-ExecutionPolicy Bypass` can be found in scheduled tasks. To add a scheduled task that runs a PowerShell script with the `Bypass` execution policy, you can use the following command:
$action = New-ScheduledTaskAction -Execute "powershell.exe" -Argument "-ExecutionPolicy Bypass -File C:\Scripts\myScheduledScript.ps1"
This allows administrative tasks to run without restriction at scheduled intervals, enhancing automation without running into policy barriers.
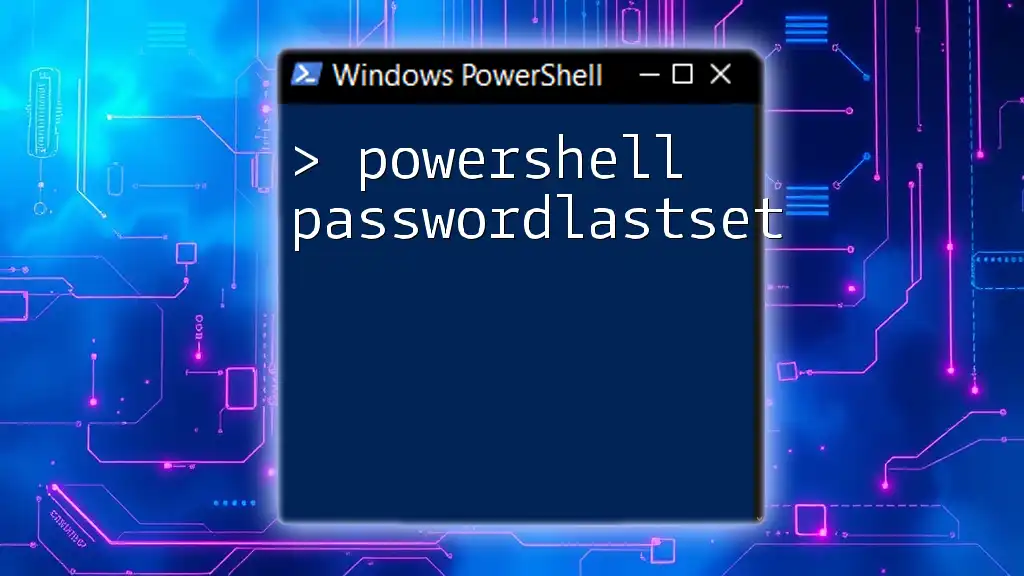
Best Practices for Using `-ExecutionPolicy Bypass`
Security Considerations
While using `-ExecutionPolicy Bypass` certainly adds flexibility, it's vital to remain vigilant. When overriding execution policies, you must recognize the associated risks, including potential exposure to malicious scripts. Always validate the integrity of scripts before execution. You can do this by reviewing the source code, utilizing antivirus software, or downloading from trusted repositories.
Regular Audits and Monitoring
Implementing a routine to monitor and audit script execution is paramount in maintaining security. Keeping track of which scripts are running, when, and by whom can help identify any nefarious activity. Tools like Sysmon or PowerShell’s own logging capabilities can be useful for tracking script executions and ensuring that security policies are followed.
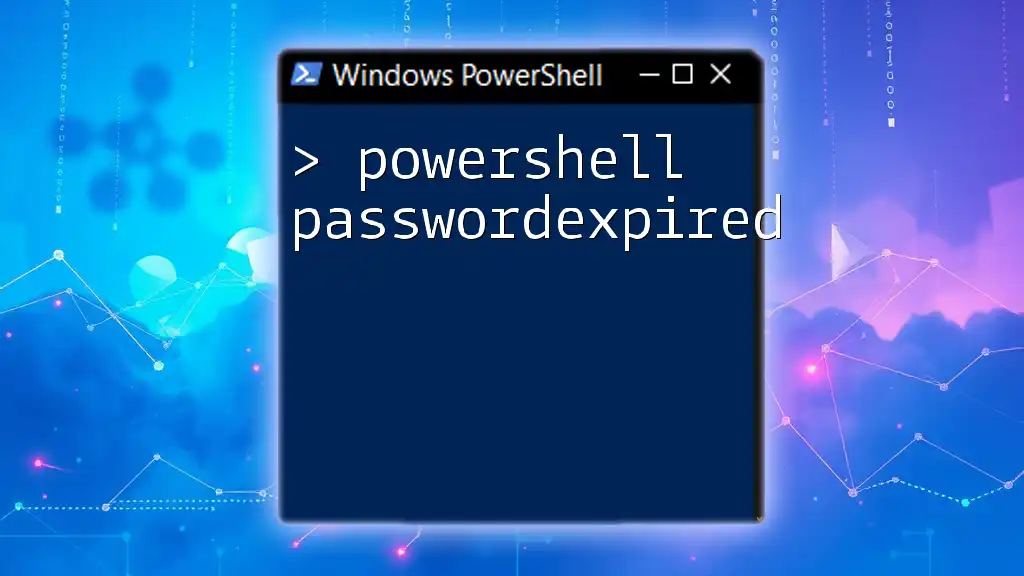
Common Issues and Troubleshooting
Errors Related to Execution Policy
When you attempt to run scripts, you might encounter errors related to execution policies. For example, if a script is blocked due to restrictions, an error message like "File can't be loaded because running scripts is disabled on this system" can appear. Understanding how to use `-ExecutionPolicy Bypass` can help you overcome these obstacles, but ensure you're aware of the implications involved.
Verifying Execution Policy
To check the current execution policy of your environment, you can use:
Get-ExecutionPolicy -List
This command displays the effective execution policy settings for the current session, which can be helpful to understand if any conflicts might arise with your script execution.
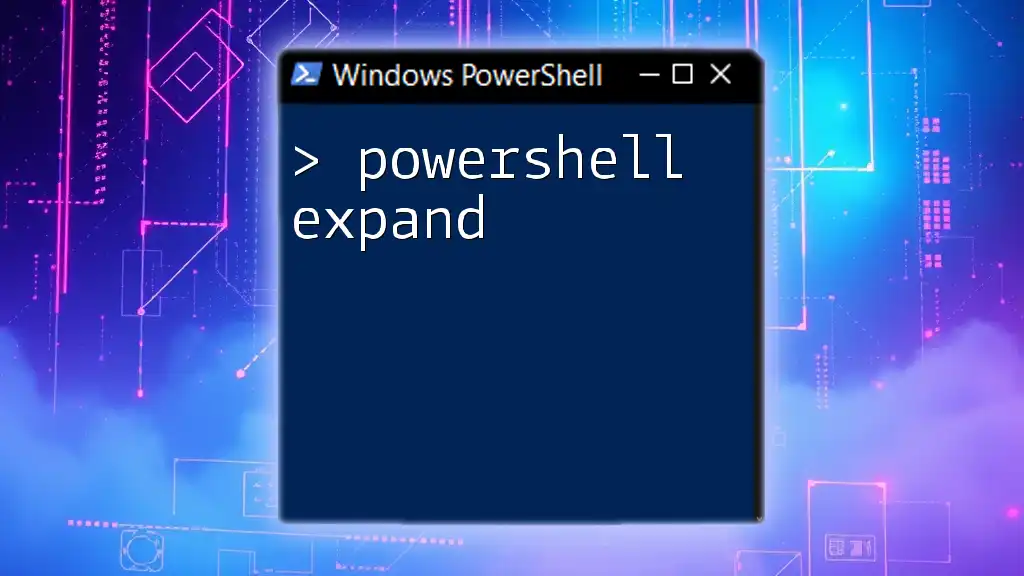
Conclusion
Understanding and effectively utilizing `powershell -exec bypass` can tremendously enhance your scripting capabilities, especially in automation and administrative tasks. However, with great power comes great responsibility. It’s essential to grasp the implications of bypassing execution policies, ensuring that you employ it judiciously and securely in your PowerShell endeavors.
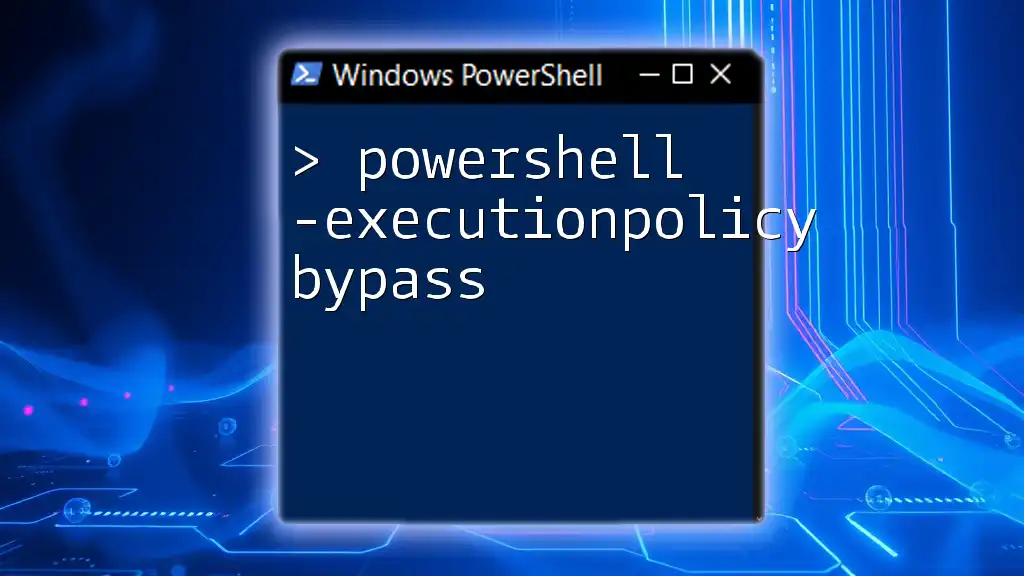
Additional Resources
For further learning, refer to the official Microsoft documentation on PowerShell execution policies. Exploring additional tutorials and reading materials can greatly enhance your PowerShell skills and security practices.