PowerShell file types are essential for scripting and configuration management, commonly including `.ps1` for scripts, `.psd1` for data files, and `.psm1` for module files.
Here's a simple example of a PowerShell script file (`.ps1`):
# Example PowerShell Script
Write-Host 'Hello, World!'
Understanding PowerShell File Types
Definition of File Types in PowerShell
In the context of scripting and automation, file types play a crucial role in defining how PowerShell interacts with the content within these files. Each file type serves a specific purpose, enabling users to perform various tasks efficiently. Understanding these file types is essential for effective scripting and automation in PowerShell.
Commonly Used PowerShell File Types
PowerShell relies on several file types, each tailored to meet specific needs. While there are many file types, the most commonly referenced PowerShell file types include:
- Script Files (.ps1)
- Module Files (.psm1)
- Profile Files
- XML Files (.xml)
- Manifest Files (.psd1)
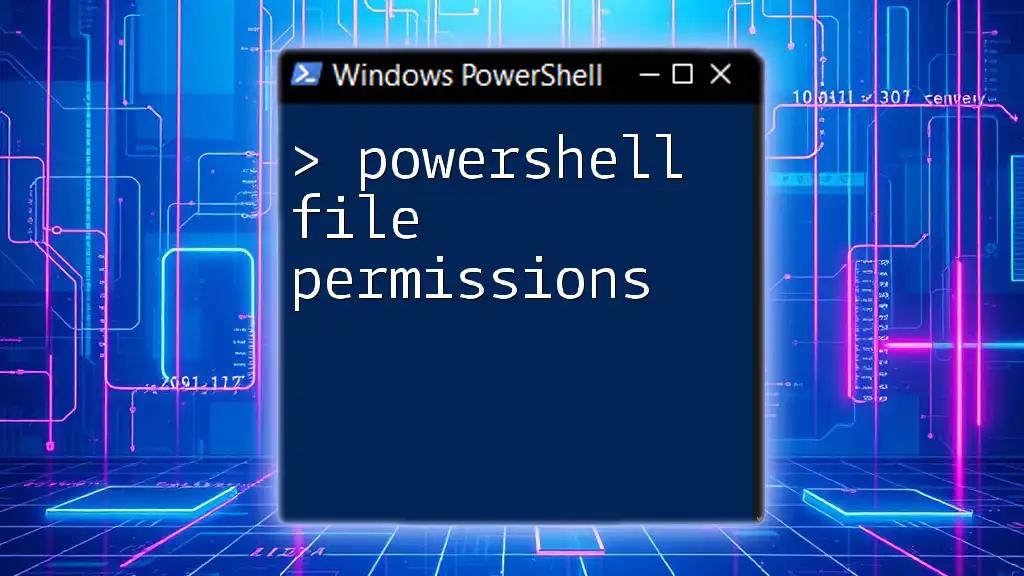
PowerShell Script Files (.ps1)
What is a .ps1 File?
The .ps1 file is the fundamental script file in PowerShell. These files contain a series of PowerShell commands and can be executed sequentially. They allow for the automation of repetitive tasks, making it easy to encapsulate complex logic into a single file.
Creating a Simple .ps1 File
Creating a .ps1 file is straightforward. Open any text editor or PowerShell ISE and write your script. For instance, a simple PowerShell script to display a message can be:
# Example of a simple PowerShell script
Write-Host "Hello, PowerShell!"
In this example, `Write-Host` is a command that outputs the provided string to the console. After saving the file with a .ps1 extension (e.g., `HelloPowerShell.ps1`), you can execute it in the PowerShell console.
Executing .ps1 Files
To run a .ps1 script, you can navigate to its directory in the PowerShell console and execute it like this:
.\HelloPowerShell.ps1
Note: Execution policies in PowerShell may restrict script execution for security purposes. If you encounter an error, you may need to change the execution policy using:
Set-ExecutionPolicy RemoteSigned
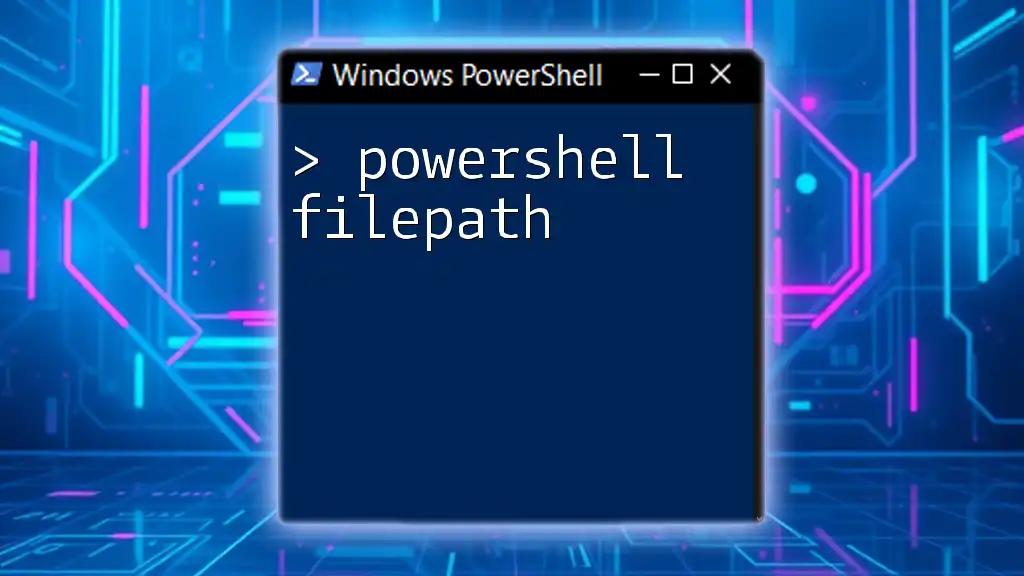
PowerShell Module Files (.psm1)
What is a .psm1 File?
PowerShell module files with the `.psm1` extension are designed to encapsulate related functions, allowing you to organize and reuse your code more efficiently. Utilizing modules promotes better code organization, reuse, and maintenance.
Creating and Importing a Module
You can create a module by defining functions inside a .psm1 file. For example, consider the following module named `MyModule.psm1`:
# MyModule.psm1
function Get-Greeting {
param($Name)
"Hello, $Name!"
}
To use this module, save it as `MyModule.psm1` and execute the following command in your PowerShell console to import it:
Import-Module MyModule.psm1
Now, you can call the function defined in your module:
Get-Greeting -Name "User"
This command will output: `Hello, User!`

PowerShell Profile Files
What are PowerShell Profile Files?
PowerShell profile files allow users to customize their PowerShell experience by executing commands or scripts automatically upon launching a PowerShell session. These profiles can be specific to users or for the entire system, enabling personalized environments.
You can access your user-specific profile by running:
notepad $PROFILE
Customizing Your Profile
Customizing your profile can range from setting environment variables to defining functions and aliases. For example, to add a simple greeting to your profile file, you could include the following line:
Write-Host "Welcome to PowerShell!"
Every time you open PowerShell, you will see this message, providing a friendly reminder of your scripting environment.
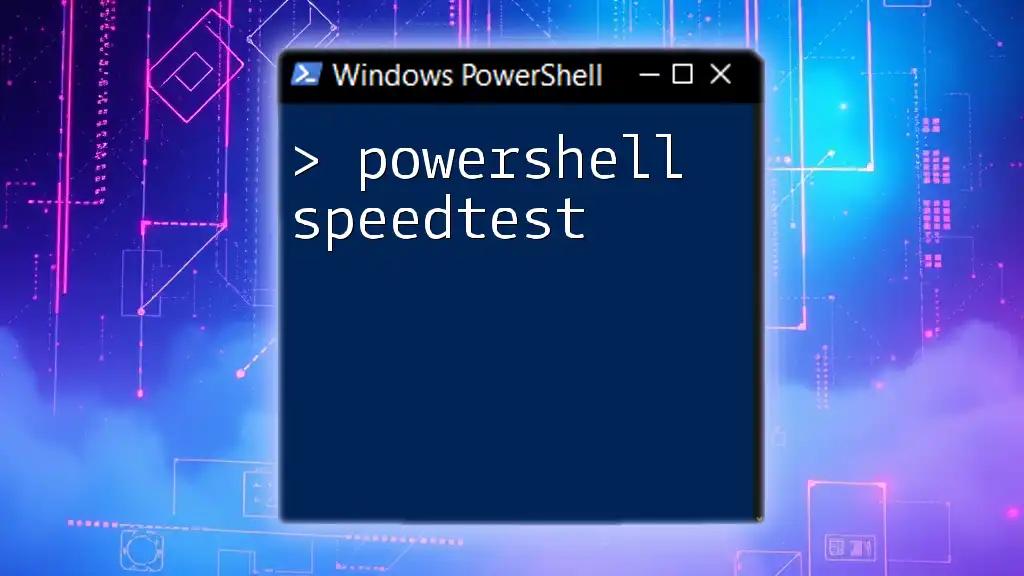
Other PowerShell File Types
PowerShell XML Files (.xml)
XML files are used to store structured data in a format that PowerShell can easily read and manipulate. You can leverage XML in PowerShell for configuration files, data exchange, and more. Knowledge of XML can expand the versatility of your PowerShell scripts.
PowerShell Manifest Files (.psd1)
Manifest files with the `.psd1` extension provide metadata about a module. This metadata includes information such as the module's version, author, and dependencies, which help in managing modules effectively. Creating a manifest ensures that your module is well-organized and easier to distribute.

Working with PowerShell File Types
Listing Files and Extensions
You can use PowerShell to list files with specific extensions in any directory. For example, to find all `.ps1` files in a specific folder, execute:
Get-ChildItem -Path "C:\Scripts\" -Filter "*.ps1"
This command will return all `.ps1` files within the `C:\Scripts\` directory, allowing you to keep track of your scripts.
Best Practices for File Management
When managing PowerShell files, consider the following best practices:
- Organize Scripts: Store scripts in dedicated directories to avoid clutter.
- Use Comments: Document your code with comments to clarify functionality for yourself and others.
- Version Control: Implement version control (e.g., Git) to track changes to your scripts and collaborate with others easily.

Conclusion
Understanding PowerShell file types allows you to harness the full power of PowerShell for automation and scripting. By mastering these file types, you can create organized, reusable scripts that streamline your workflow, improve your productivity, and enhance your overall PowerShell experience.

Additional Resources
For further reading, consider exploring official Microsoft documentation, reputable PowerShell books, or enrolling in online courses tailored to enhancing your PowerShell skills.