PowerShell filters allow users to efficiently narrow down the output of commands by specifying conditions to match specific objects from a collection.
Here’s a code snippet demonstrating how to filter a list of services to show only those that are running:
Get-Service | Where-Object { $_.Status -eq 'Running' }
Understanding the Basics of Filtering in PowerShell
What is a Filter in PowerShell?
A filter in PowerShell is a way to restrict or narrow down the set of data that you are working with. By using filters, the user can obtain only the elements that meet specific criteria, which helps in managing and analyzing data more efficiently. Filtering is crucial when working with large datasets, as it allows you to focus on the relevant portions of your data.
Types of Data Structures in PowerShell
In PowerShell, common data structures include arrays and collections. An array is a list of items that can contain multiple types of data, while collections are a more complex structure that can store objects with more functionality. Understanding these structures is essential for effectively utilizing filters.
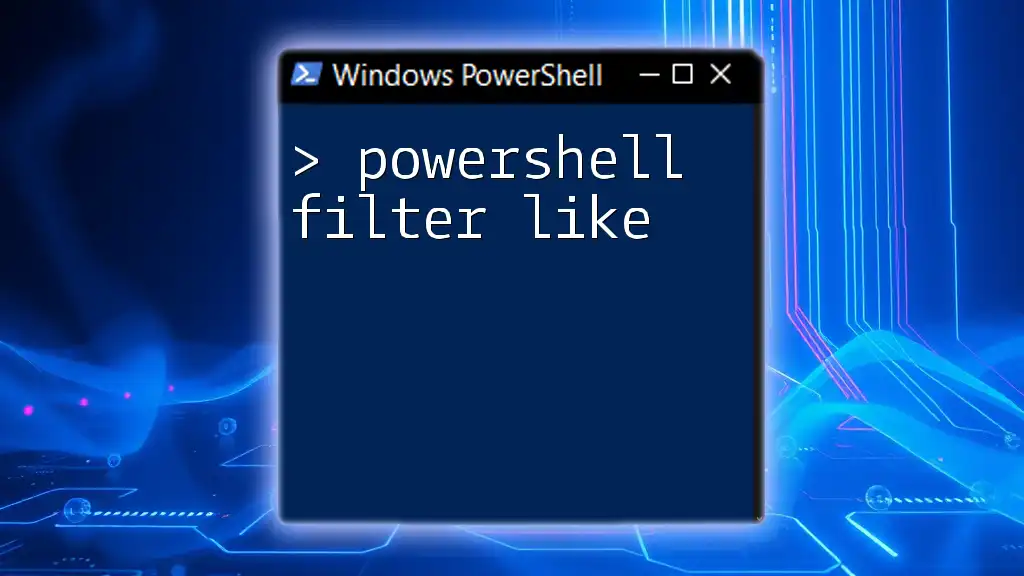
The Power of the `Where-Object` Cmdlet
What is `Where-Object`?
The `Where-Object` Cmdlet is the primary tool used to filter lists in PowerShell. It allows you to select objects from a collection based on conditions you specify. The basic syntax of `Where-Object` is:
Where-Object { condition }
This cmdlet is flexible and can be applied to any collection of objects.
Basic Usage of `Where-Object`
To demonstrate the basic usage of `Where-Object`, consider filtering a simple list of numbers:
$numbers = 1..10
$filteredNumbers = $numbers | Where-Object {$_ -gt 5}
In this example, we create an array of numbers from 1 to 10 and filter it to return only those numbers greater than 5. The placeholder `$_` represents the current object in the pipeline.
Using Operators with `Where-Object`
Comparison Operators
PowerShell provides several comparison operators—such as `-eq` (equal), `-ne` (not equal), `-gt` (greater than), and `-lt` (less than)—that can be used within `Where-Object`.
Consider this example of filtering based on specific conditions:
$users = @("Alice", "Bob", "Charlie", "David")
$filteredUsers = $users | Where-Object {$_ -like "*a*"}
Here, we filter a list of usernames, returning those containing the letter "a". The `-like` operator uses wildcards to match patterns.
Logical Operators
Logical operators (`-and`, `-or`, `-not`) can be combined with `Where-Object` to create more complex filtering conditions:
$mixedNumbers = 1, 5, 8, 12, 20, 30
$filteredMixed = $mixedNumbers | Where-Object {$_ -lt 10 -and $_ -gt 5}
In this example, we filter the list to include only numbers that are less than 10 and greater than 5.
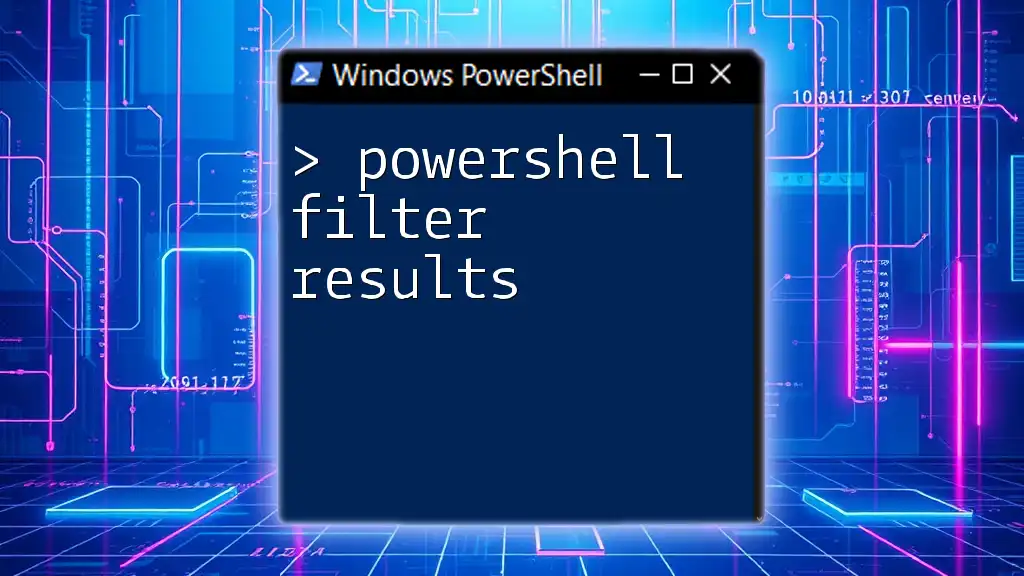
Filtering Lists with `Select-Object`
Overview of `Select-Object`
While `Where-Object` is used for filtering by conditions, `Select-Object` is utilized to select specific properties from the objects in the pipeline. This will help extract the desired information efficiently from complex objects.
Selecting Specific Properties
To illustrate the use of `Select-Object` with filtering, consider this example:
Get-Process | Where-Object {$_.CPU -gt 100} | Select-Object Name, CPU
This command retrieves a list of processes using more than 100 CPU units, and then it extracts only the `Name` and `CPU` properties of those processes. This not only filters the data but also refines the output to show only the required details.
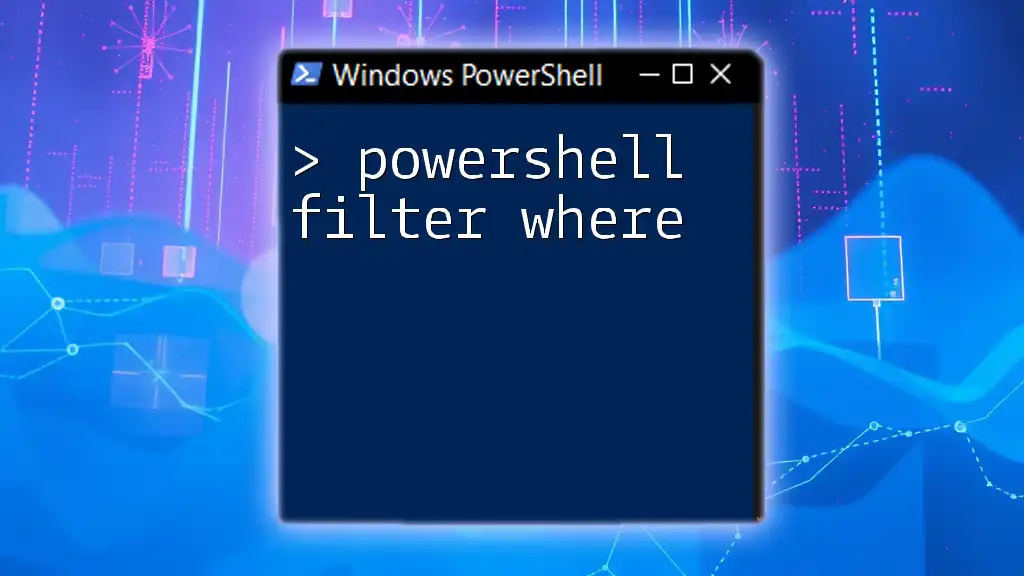
Advanced Filtering Techniques
Using Regular Expressions for Filtering
Regular expressions (regex) provide a powerful way to specify patterns for string matching. They can significantly expand your filtering capabilities in PowerShell.
Example of Regex Filtering
Here's an example of filtering strings using regex:
$strings = "test1", "test2", "sample", "test3"
$filteredStrings = $strings | Where-Object {$_ -match "^test"}
In this case, the regex pattern `^test` checks for strings that start with "test". This technique allows for sophisticated filtering scenarios.
Custom Filtering Functions
Creating custom filtering functions can enhance your data management capabilities. For instance, you can design a function that filters numbers based on a user-defined condition:
function Filter-Numbers {
param ($list, $condition)
return $list | Where-Object {$_ -gt $condition}
}
$myList = 1..20
Filter-Numbers -list $myList -condition 10
In this example, the `Filter-Numbers` function takes a list and a condition as parameters, returning only the numbers greater than the specified condition.
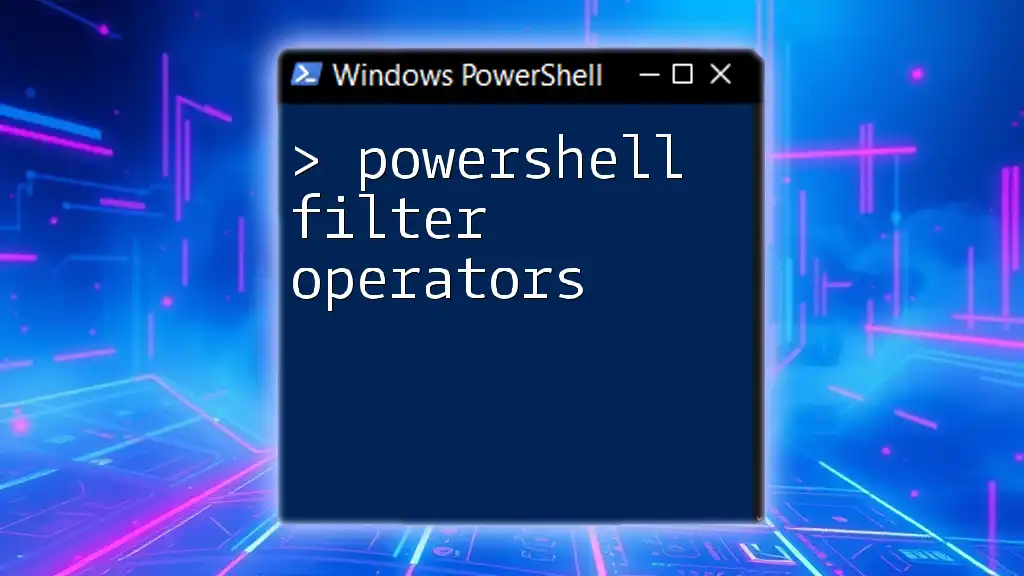
Troubleshooting Common Filtering Issues
Common Mistakes to Avoid
When working with `Where-Object`, a common mistake is not enclosing the condition in curly braces or improperly referencing object properties. Always ensure the syntax is correct, as errors can cause scripts to fail.
Performance Considerations in Filtering
It's critical to consider performance when filtering large datasets. Using specific cmdlets designed for bulk data operations, such as `ForEach-Object`, can enhance performance. Additionally, evaluate your filters for efficiency. For example, using logical comparisons wisely can avoid unnecessary iterations.
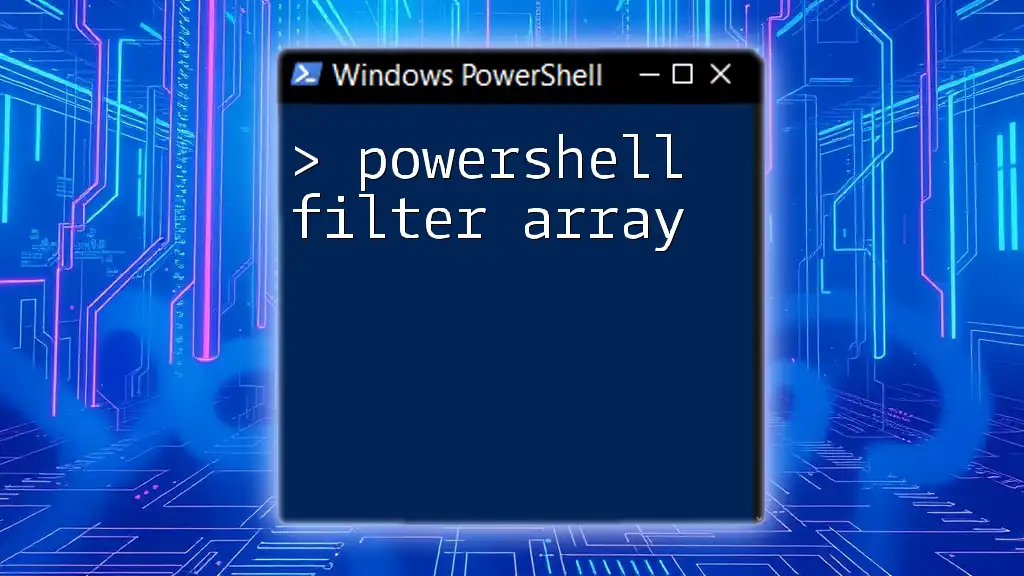
Conclusion
Filtering lists in PowerShell is a fundamental skill that enhances your ability to manage data effectively. By mastering `Where-Object`, utilizing `Select-Object`, and diving into advanced techniques such as regular expressions and custom functions, you empower yourself to handle complex data with ease. Practicing these commands will make your PowerShell scripting more efficient.
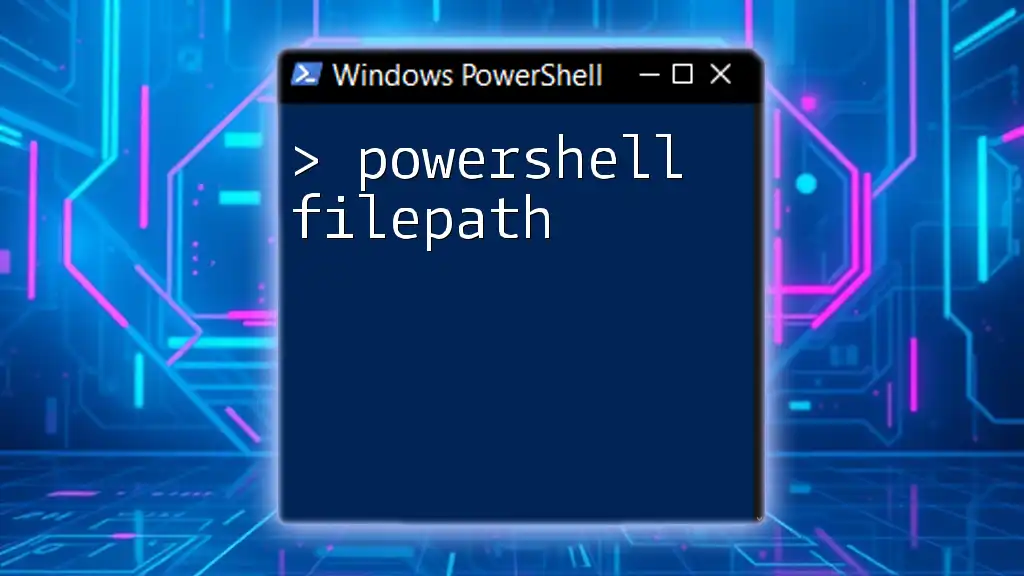
Additional Resources
For further learning, consider exploring the official Microsoft documentation on PowerShell. Additionally, several books and online tutorials focus on advanced PowerShell techniques to help enhance your skills.
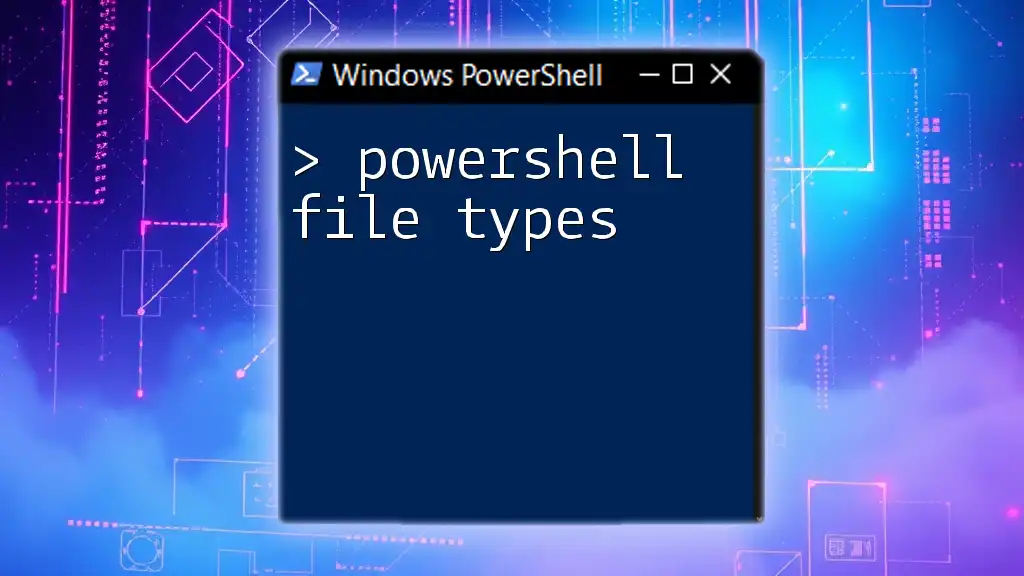
FAQ Section
What is the difference between `Where-Object` and `Select-Object`?
- `Where-Object` filters objects based on a condition.
- `Select-Object` selects specific properties from objects that have already been filtered.
How can I filter complex objects like custom classes?
Filtering complex objects often involves providing property names or using nested filtering conditions, similar to simpler object filtering methods.
Can I filter lists in PowerShell scripts?
Absolutely! You can integrate filtering commands into your PowerShell scripts to enhance their data-processing capabilities effectively.