PowerShell 7's `ForEach-Object -Parallel` feature allows you to run script blocks in parallel, which can significantly improve performance when processing large datasets.
Here's a code snippet demonstrating its usage:
1..10 | ForEach-Object -Parallel {
Start-Sleep -Seconds 1
Write-Host "Processing $_ in parallel"
} -ThrottleLimit 2
Understanding Parallel Processing in PowerShell
Parallel processing allows you to run multiple tasks at the same time, leveraging the power of multi-core processors for improved performance. This is especially useful for long-running operations or when working with large datasets. By using PowerShell 7's `ForEach-Object -Parallel` cmdlet, you can design scripts that significantly cut down processing time.
When to Use ForEach-Object Parallel
Utilizing the `ForEach-Object -Parallel` feature is advantageous in several scenarios:
- Long-running tasks: Heavy computations or network calls can slow down scripts. Running them in parallel can dramatically reduce waiting times.
- Operations on large datasets: Processing thousands of items sequentially can take too long; parallel execution speeds this up.
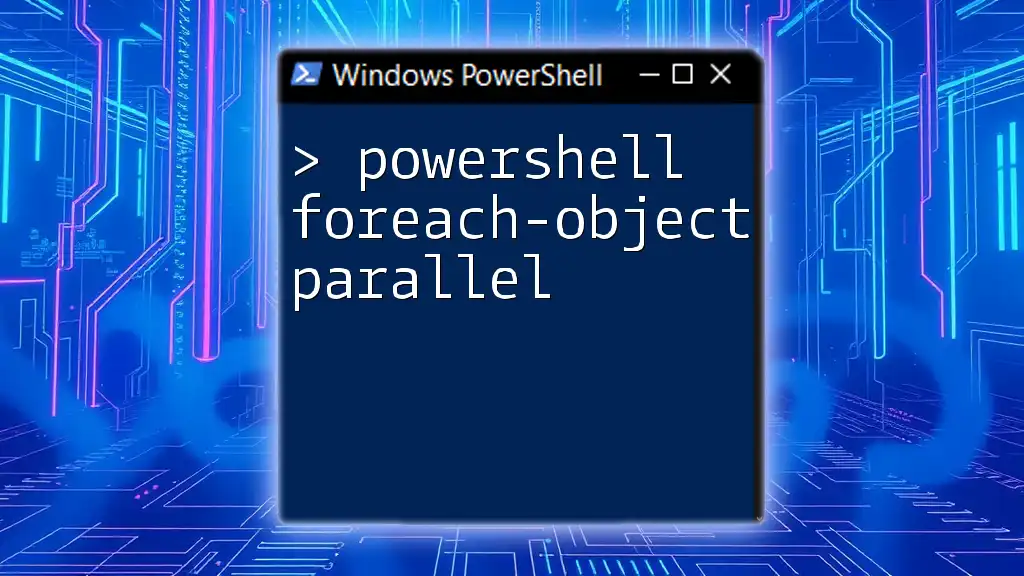
How to Implement ForEach-Object Parallel
To get started, you need to understand the basic syntax of the `ForEach-Object -Parallel` command.
$input | ForEach-Object -Parallel {
# Your code here
}
Key Parameters Explained:
- `-Parallel`: This flag allows you to define a script block that will be executed for each item in parallel.
- `-ThrottleLimit`: This parameter controls how many parallel tasks can run simultaneously, allowing you to manage system resource usage effectively.
- `-AsJob`: When included, this flag runs the command as a background job, letting your script continue executing while the jobs are processed.
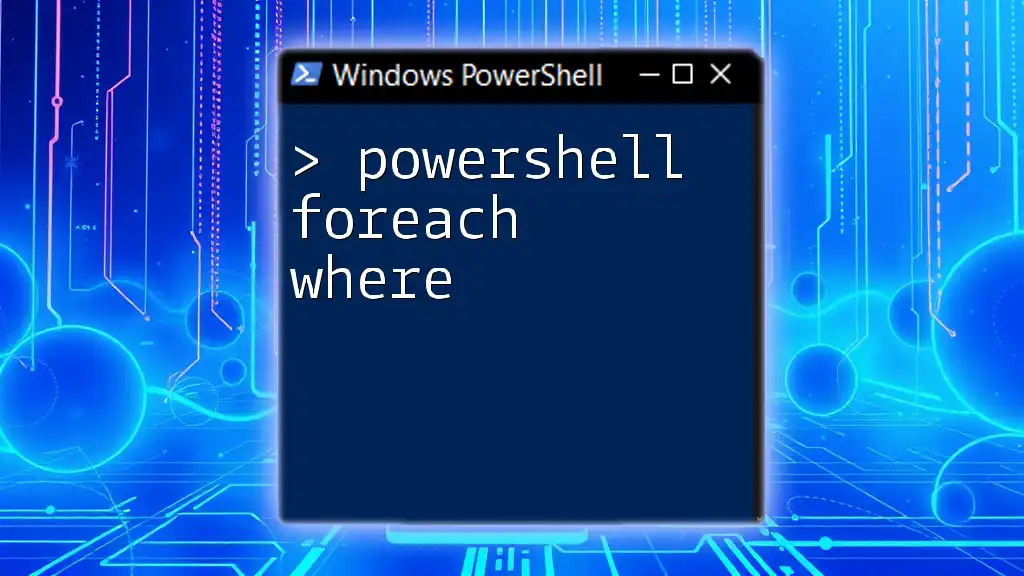
Practical Examples
Example 1: Basic Implementation
Consider a situation where you want to process multiple files. The following example demonstrates how to use `ForEach-Object -Parallel` simply.
Get-ChildItem 'C:\Files' | ForEach-Object -Parallel {
# Process each file
Write-Output "Processing $_"
}
In this script, PowerShell fetches all files in the specified directory and processes them in parallel, significantly speeding up the operation.
Example 2: Using ThrottleLimit
Controlling the number of simultaneous tasks can help you optimize performance. In this example, we set a `-ThrottleLimit` to ensure only three threads run at once.
1..10 | ForEach-Object -Parallel {
Start-Sleep -Seconds 1
"Processed $_"
} -ThrottleLimit 3
Here, each number from 1 to 10 is processed in parallel but limited to three at any given time, preventing your system from becoming overwhelmed.
Example 3: Combining with Other Cmdlets
You can also combine `ForEach-Object -Parallel` with other PowerShell cmdlets to enhance functionality. Below is an example that retrieves web content in parallel.
$urls = @("http://example.com", "http://example.org")
$urls | ForEach-Object -Parallel {
Invoke-WebRequest -Uri $_
}
In this script, PowerShell makes HTTP requests to multiple URLs simultaneously, significantly improving the speed of web data retrieval.
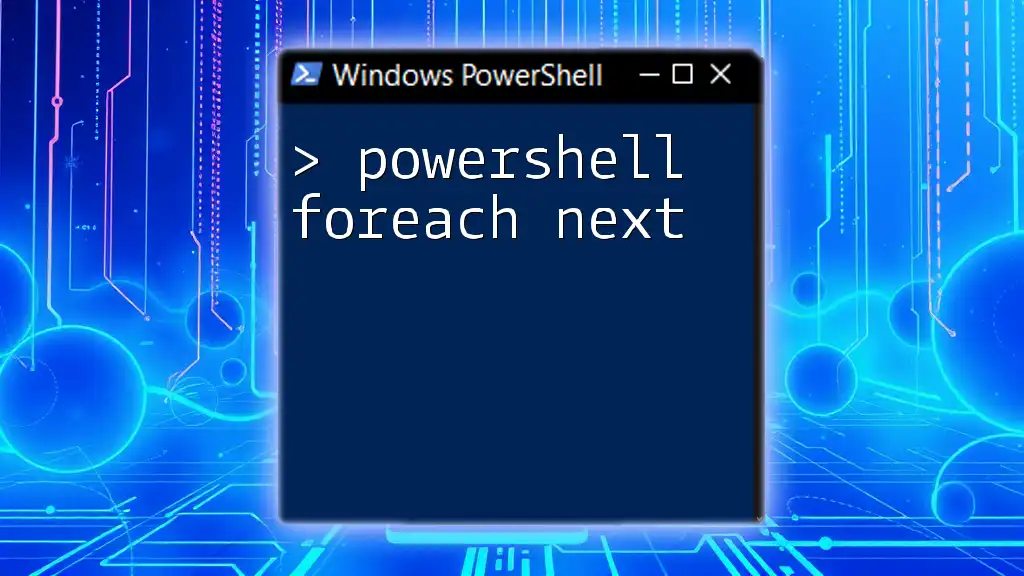
Best Practices for Using ForEach-Object Parallel
Avoiding Shared State: When working with `ForEach-Object -Parallel`, it's crucial to avoid shared state between threads. Each instance runs in its own isolated environment, so if threads need to share data, consider using external resources like databases or files.
Error Handling: Implement robust error handling in your scripts to catch issues as they occur. For example:
$results = 1..5 | ForEach-Object -Parallel {
try {
# Potential error-generating code
"Processing $_"
} catch {
Write-Error "Error processing $_"
}
}
This code captures errors during parallel execution, allowing you to debug effectively.
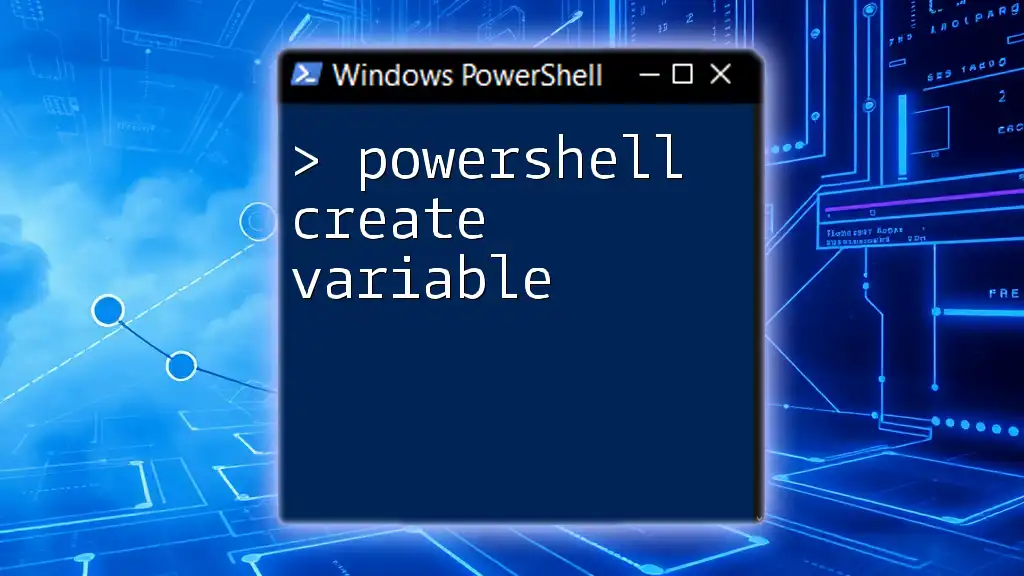
Performance Considerations
Benchmarking Parallel vs. Serial: To determine the performance benefits, you should benchmark your scripts. This can be done by timing operations in both parallel and sequential forms to see how much time is saved.
Resource Management: When utilizing multiple threads, it's essential to manage resources carefully. Monitor CPU and memory usage to avoid system bottlenecks, especially during extensive operations.
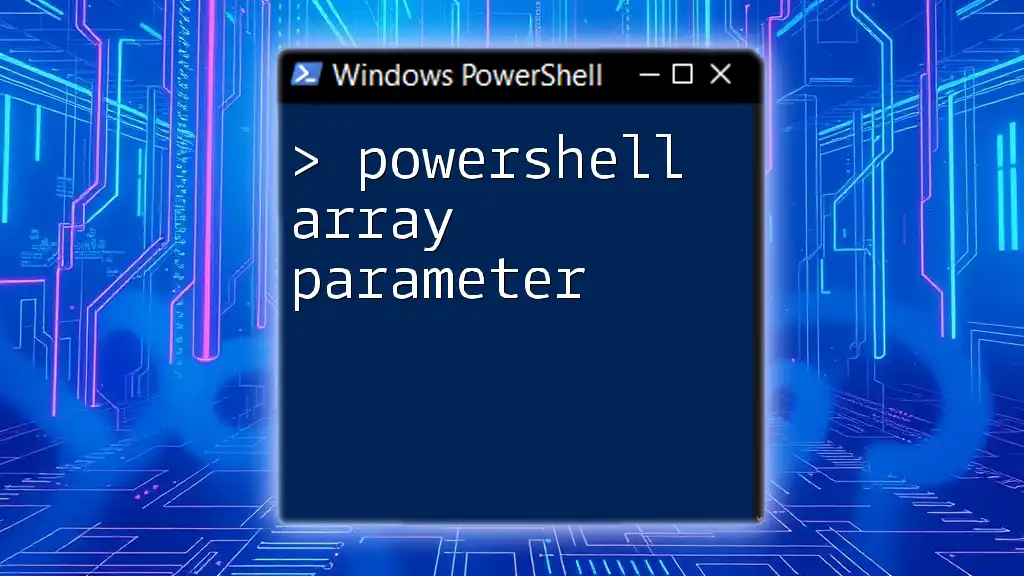
Conclusion
The `ForEach-Object -Parallel` feature in PowerShell 7 is a powerful tool for enhancing script performance. By allowing tasks to run in parallel, you can reduce execution time and improve efficiency, particularly for long-running or resource-intensive operations. The flexibility to manage how many tasks run at once further equips you to optimize the performance while maintaining control over system resources.
By practicing these techniques and learning through trial and error, you will become adept at leveraging parallel processing in PowerShell to streamline your automation tasks.
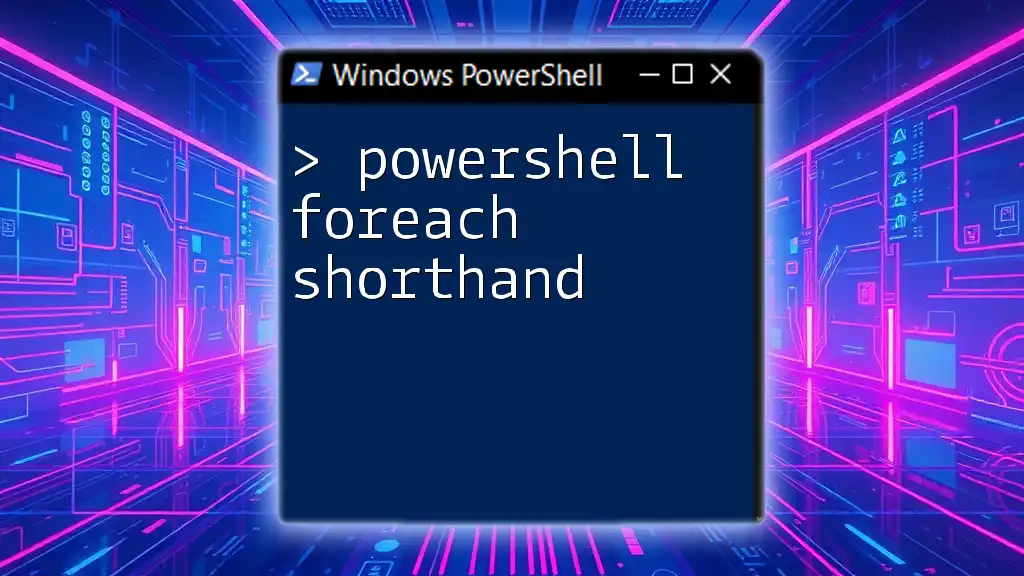
Additional Resources
To deepen your understanding, refer to the official Microsoft PowerShell documentation, which provides comprehensive information about the `ForEach-Object` cmdlet and its parallel capabilities. Additionally, consider joining PowerShell communities and forums where you can share experiences and gain insights from other users.
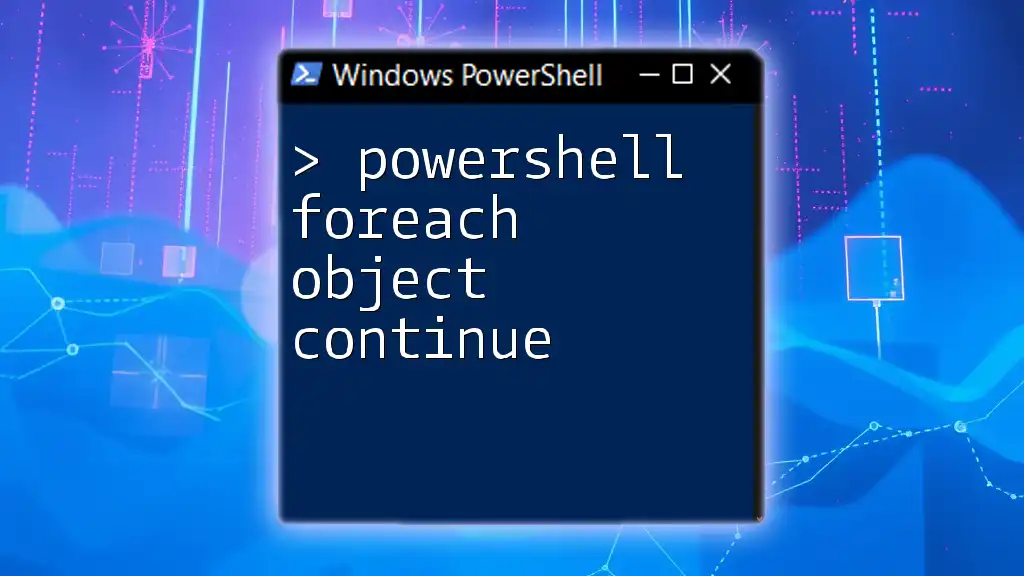
Call to Action
If you’re ready to take your PowerShell skills to the next level, consider enrolling in our online course, where we dive deep into mastering PowerShell commands, including practical sessions on using ForEach-Object -Parallel. Start your journey towards becoming a PowerShell expert today!