In PowerShell, the escape character is the backtick (`), which is used to signify that the following character should be treated literally or to allow multi-line statements.
Here’s a code snippet demonstrating its use:
Write-Host 'This is a backtick: `` and this is a new line:`nSecond Line'
Understanding Escape Characters in PowerShell
What is an Escape Character?
An escape character is a symbol used to indicate that the character following it should be treated differently than it normally would. In programming and scripting languages, escape characters serve the crucial purpose of altering the default behavior or interpretation of specific characters.
In PowerShell, the primary escape character is the backtick (`). Understanding how to use the escape character is vital for handling special situations within your scripts, as it allows you to include symbols, whitespace, and other elements in a controlled manner.
Why Use Escape Characters in PowerShell?
Escape characters are essential in PowerShell for several reasons:
-
Managing Special Characters: Certain characters, like quotes or the dollar sign, have special meanings. Using an escape character allows these symbols to be included literally in your strings.
-
Preventing Unintended Interpretation: If you don’t escape characters that PowerShell recognizes (like `$` for variable expansion), your script might not behave as intended, leading to errors.
-
Enhancing Code Readability and Maintainability: Proper use of escape characters makes your code clearer, enabling other developers (or your future self) to understand it more effortlessly.
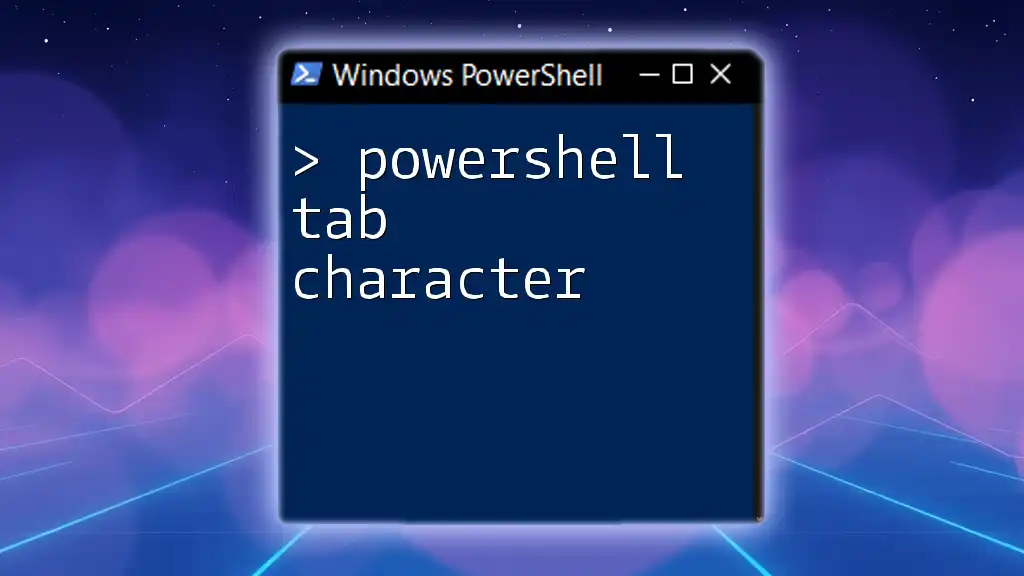
The Power of the Backtick (`)
The Backtick as the Primary Escape Character
In PowerShell, the backtick (`) is the escape character used to modify the default interpretation of characters. To effectively utilize the backtick, you can place it before the character that you want to escape.
For example, when we want to include a newline in a string, we can use:
$greeting = "Hello`nWorld!"
Write-Host $greeting
In this example, `n represents a newline, which causes the output to display “Hello” on one line and “World!” on the next.
Common Uses of the Backtick
Escaping Special Characters
PowerShell has several special characters that may need to be escaped. The backtick can be used to prevent these characters from being interpreted in their usual way.
Example: To represent a tab character, you can use:
$message = "Name:`tJohn Doe"
Write-Host $message
Here, `t inserts a tab space before "John Doe".
Escaping Quotes
When you need to include quotes in your strings, escape characters become particularly useful.
For instance, to include double quotes within a string defined by double quotes, you can use:
$message = "He said, `"`Hello, World!`"`"
Write-Host $message
This method allows you to represent quotes without terminating the string prematurely.
Escaping the Dollar Sign ($) in PowerShell
Importance of the Dollar Sign
In PowerShell, the dollar sign ($) signifies the beginning of a variable. When you want to include a literal dollar sign in your output rather than referencing a variable, you need to escape it.
Methods to Escape the Dollar Sign
To include the dollar sign literally, you can use the backtick:
$amount = "The total is `$100"
Write-Host $amount
In this case, the backtick ensures the dollar sign is included without being interpreted as the start of a variable.
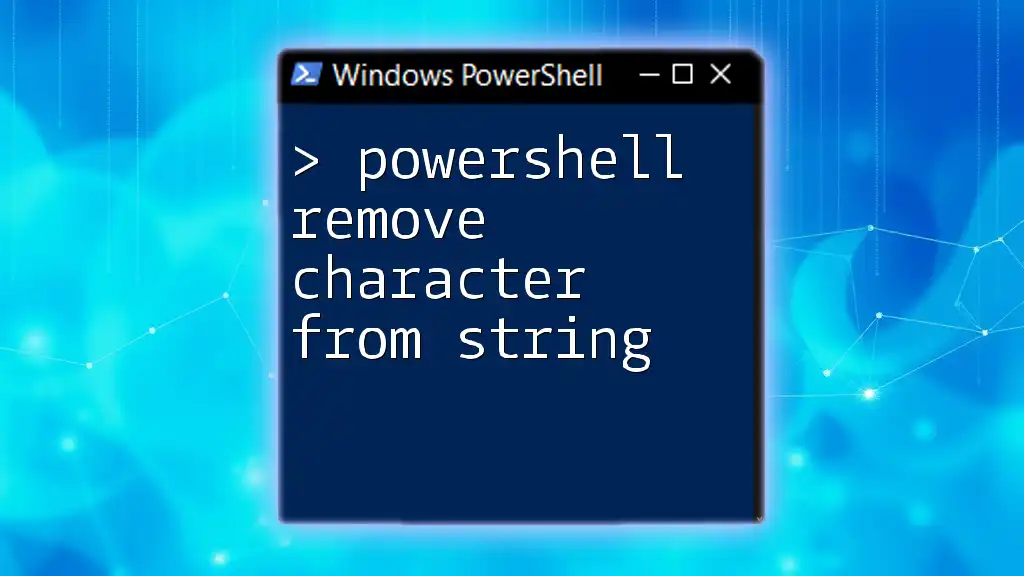
PowerShell Special Characters
List of Special Characters in PowerShell
PowerShell contains several special characters, the most notable being:
- $ - Marks the beginning of a variable name.
- `n - Represents a new line.
- `t - Indicates a tab character.
- `" - Escapes a double quote within a double-quoted string.
- `' - Escapes a single quote within a single-quoted string.
Using Special Characters with Backtick
When working with special characters, it’s essential to apply the backtick appropriately.
For example, if you want to print a line that includes a tab and a new line, you can do:
$message = "First Line`n`nSecond Line`n`tIndented Line"
Write-Host $message
The output will demonstrate how various escape sequences work together, enhancing text formatting.
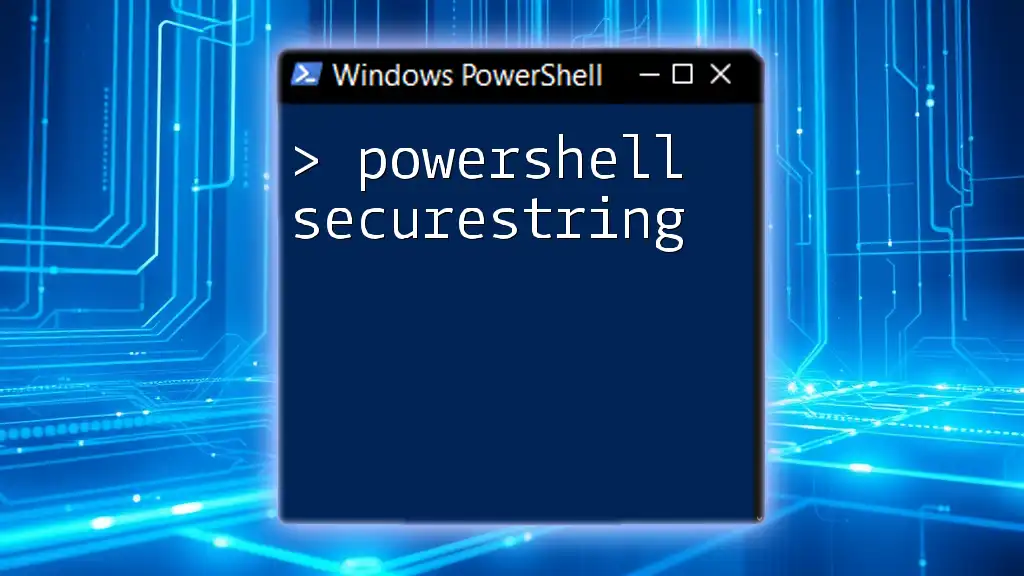
PowerShell Literal Strings
Defining Literal Strings
Literal strings are string values that represent data exactly as they appear, without any interpretation or evaluation. In PowerShell, these are particularly useful when you want to work with strings exactly as they are written.
Using Single and Double Quotes
PowerShell allows the use of both single quotes (`'`) and double quotes (`"`). The main difference lies in how they handle escape characters:
-
Single Quotes: Treat the contents literally; escape characters are ignored except for the single quote itself, which must be escaped using a second single quote.
Example:
$singleQuoteExample = 'This is a single quote: `'' Write-Host $singleQuoteExample
-
Double Quotes: Allow variable expansion and interpret escape characters like `n and `t.
Example:
$name = "John" $doubleQuoteExample = "Hello, $name!`nThis is a new line." Write-Host $doubleQuoteExample
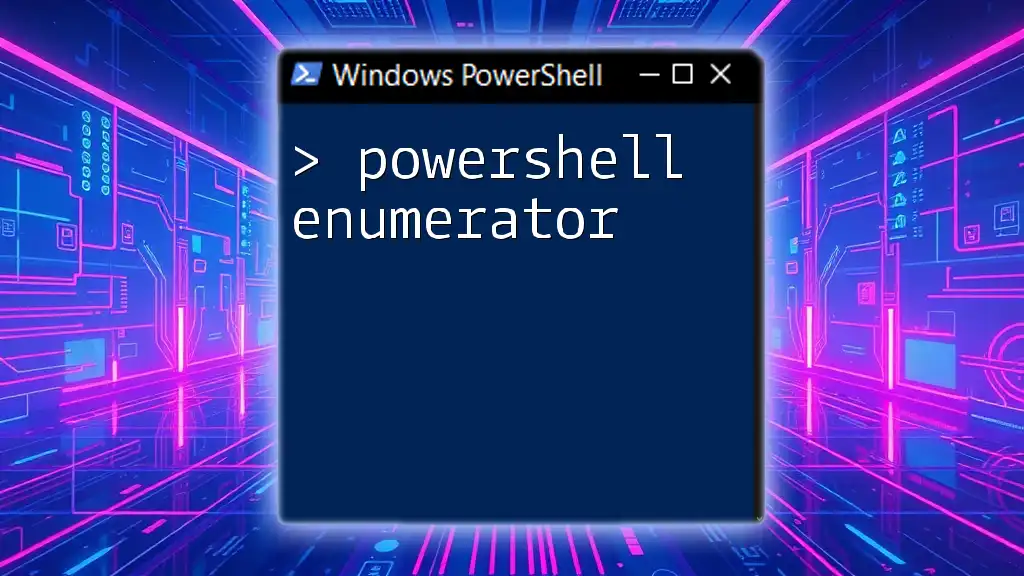
Practical Tips for Escaping in PowerShell
Common Pitfalls to Avoid
When using escape characters, it’s easy to overlook how PowerShell interprets different characters. Some common issues include:
- Forgetting to escape special characters which leads to malformed output.
- Confusing double quotes with single quotes and misapplying escape sequences.
To avoid these pitfalls, always check your strings and test small snippets to see the resulting output.
Best Practices for Writing Escape-Free Code
To write more manageable code:
- Use single quotes when you don't need variable expansion. This reduces the need for escape characters.
- When in doubt, experiment with the backtick in a console or through a script to see what works best for your scenario.
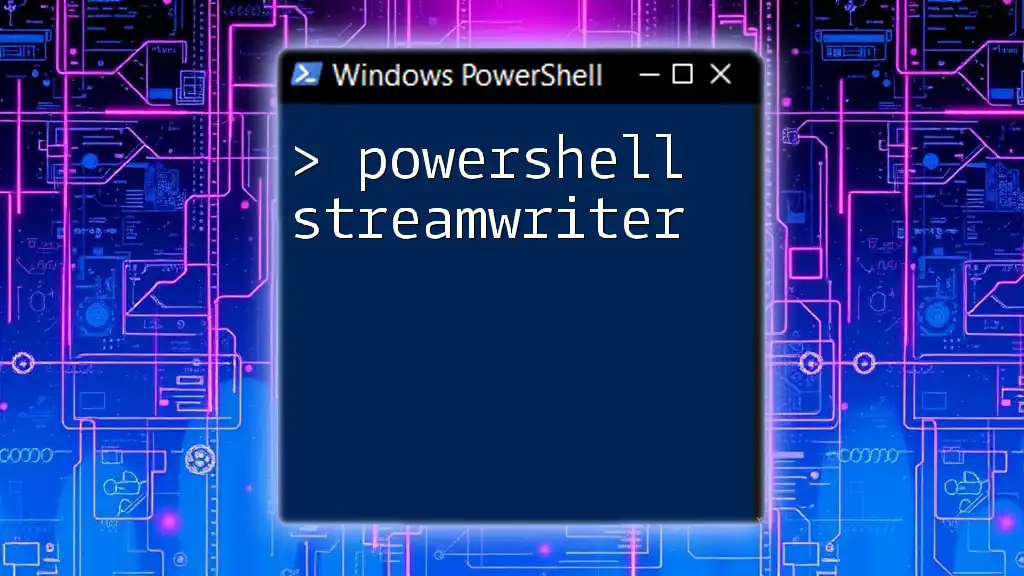
Conclusion
Mastering the PowerShell escape character is fundamental for effective scripting in PowerShell. By understanding the backtick, utilizing it correctly, and being aware of special characters, you can write cleaner, more effective scripts that function as intended. Through practice and time, you’ll find that using escape characters becomes second nature, allowing you to focus on logic and functionality in your scripts.
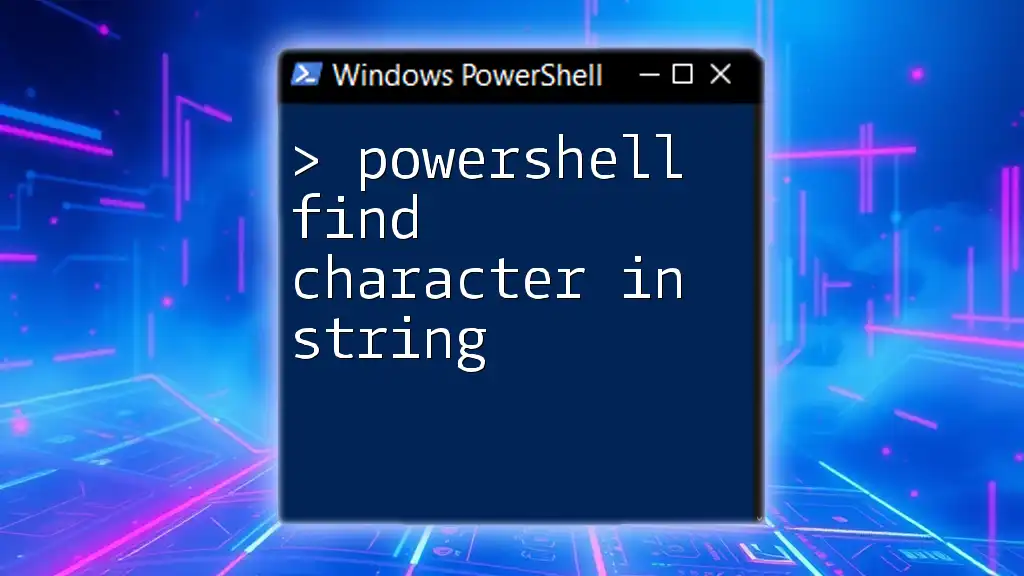
Additional Resources
For further reading on PowerShell commands and scripting, explore PowerShell communities and forums that can provide you with additional support and learning opportunities.