To escape double quotes in PowerShell, you can use the backtick character (`) before the double quote, allowing it to be treated as a literal character within a string.
Write-Host "She said, `"`Hello, World!`"`"
Understanding the Concept of Escaping in PowerShell
What Does It Mean to Escape Characters?
In programming, escaping refers to the act of indicating that a character should be treated differently than its usual interpretation. This is particularly important when dealing with special characters that have reserved meanings in a language.
For example, quoting or delimiters are often used to encapsulate strings. When you need to include these special characters within the string itself, you must escape them to prevent errors. It’s similar to a thumbprint on a login card; if you don’t clear the space for it, the system can't read your card correctly.
The Role of Double Quotes in PowerShell
In PowerShell, double quotes are used to define strings that can include variable interpolations. This means that any variable included in a double-quoted string will be evaluated to its actual value. For instance:
$greeting = "Hello, $name"
By contrast, single quotes define plain strings without variable interpretation, rendering the text as-is. Understanding when to use each type of quote is critical for scripting effectively.
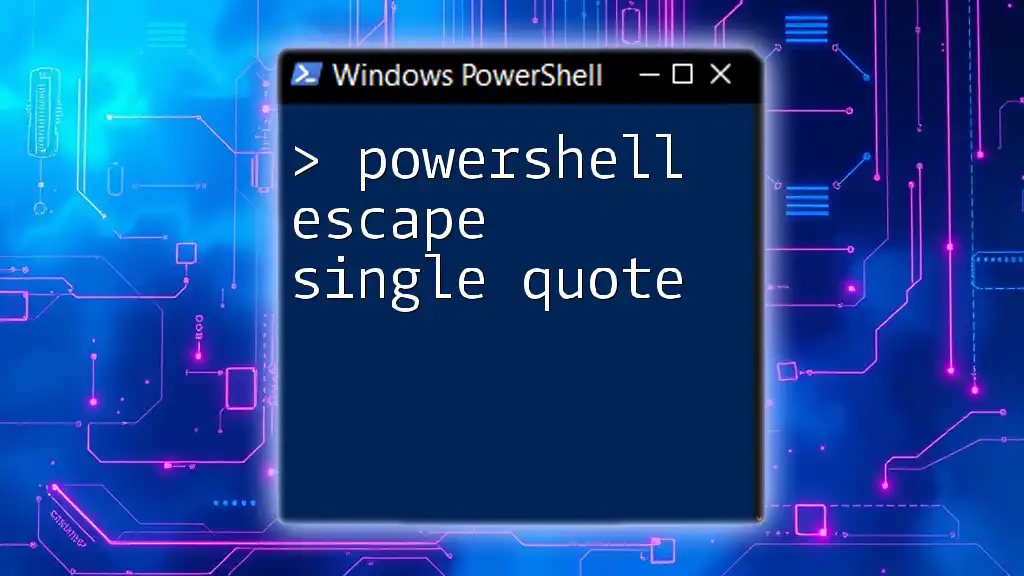
Basic Syntax for Escaping Double Quotes in PowerShell
Using the Backtick (`) Character
The backtick (`) is PowerShell's escape character. To include a double quote inside a double-quoted string, precede it with a backtick, effectively "escaping" it. Here’s how it works:
$example = "He said, `"Hello, World!`""
Write-Output $example
In this example, the output will be:
He said, "Hello, World!"
The backticks allow PowerShell to differentiate between the string delimiters and the internal quotes.
Using Single Quotes for Containment
Single quotes can also be utilized to sidestep the need for escape characters. Any text within single quotes is treated literally. This is particularly useful when crafting strings that contain double quotes. For example:
$example = 'He said, "Hello, World!"'
Write-Output $example
The output will appear as:
He said, "Hello, World!"
When you don’t need to evaluate variables or escape characters frequently, single quotes can simplify string handling.
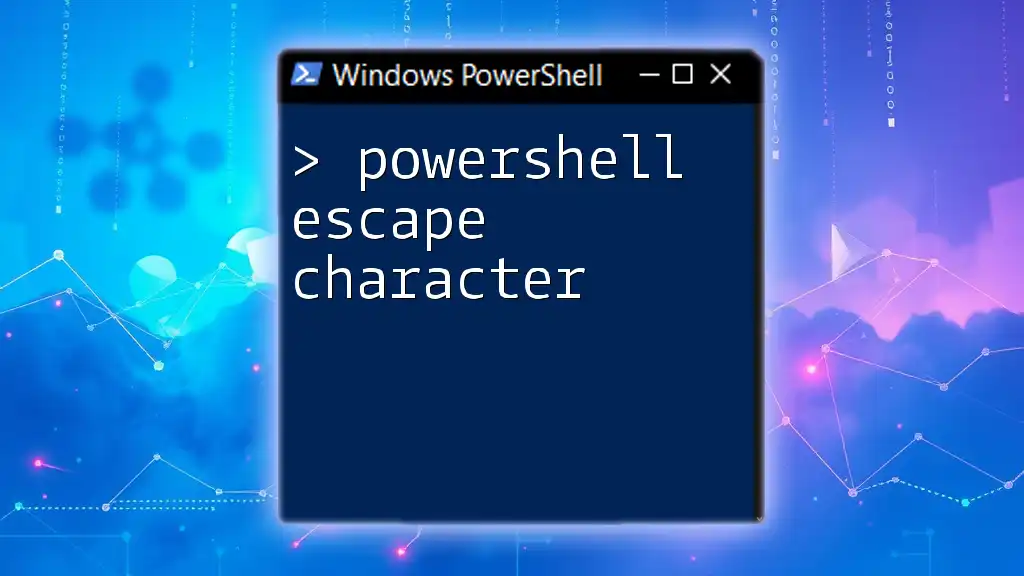
Advanced Techniques for Escaping Double Quotes
Variable Expansion and Escaping
Variable expansion adds another layer to string handling in PowerShell. If you want to include a variable alongside escaped double quotes, you can still use the backtick. Here’s a practical example:
$name = "PowerShell"
$message = "Welcome to `"${name}`"!"
Write-Output $message
The output will be:
Welcome to "PowerShell"!
This demonstrates how to effectively combine escaping with variable interpolations.
Using Here-Strings for Multi-Line Strings
Here-strings are a convenient way to create multi-line strings, especially when double quotes are required. You can define a here-string with `@"` for double quotes and `@'` for single quotes. Here’s how it looks:
$doubleQuotedHereString = @"
This is a "string" with double quotes.
It spans multiple lines.
"@
Write-Output $doubleQuotedHereString
And for single quotes:
$singleQuotedHereString = @'
This is a 'string' with single quotes.
'@
Write-Output $singleQuotedHereString
Here-strings simplify scenarios where multiple quotes and line breaks would complicate string definition.
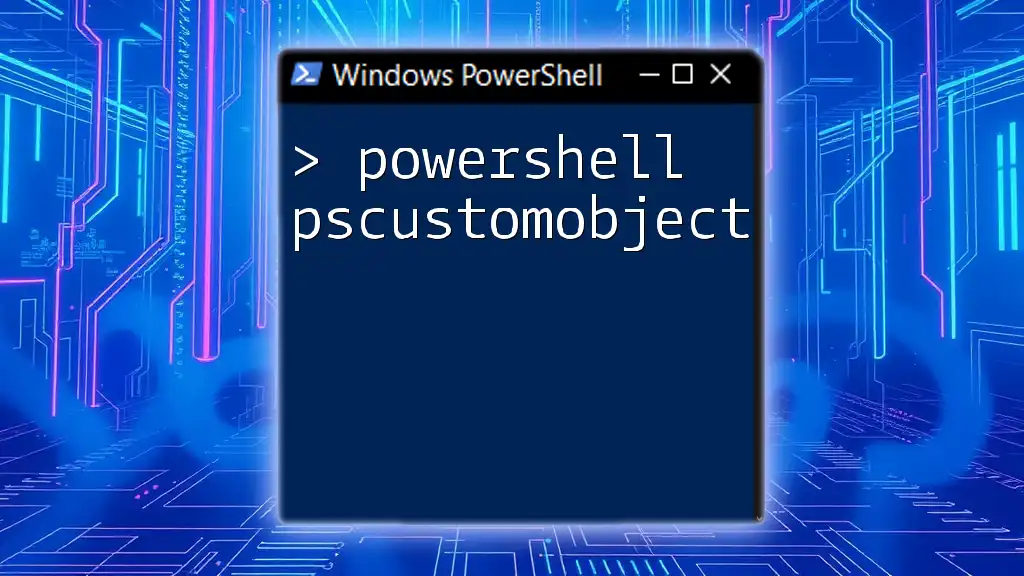
Common Mistakes When Escaping Double Quotes
Forgetting the Escape Character
One common mistake when working with the powershell escape double quote context is neglecting the backtick escape character. Omitting it will lead to syntax errors. For example:
# Incorrect
$greeting = "Hello, "World"!"
This will result in an error because PowerShell cannot determine where the string ends. The corrected code uses escape characters:
# Corrected
$greeting = "Hello, `"World`"!"
Misunderstanding Single vs Double Quotes
Another misunderstanding can occur regarding when to use single versus double quotes. Remember, single quotes treat everything literally, while double quotes can handle variables. This can lead to confusion and errors in scripts if not understood clearly.
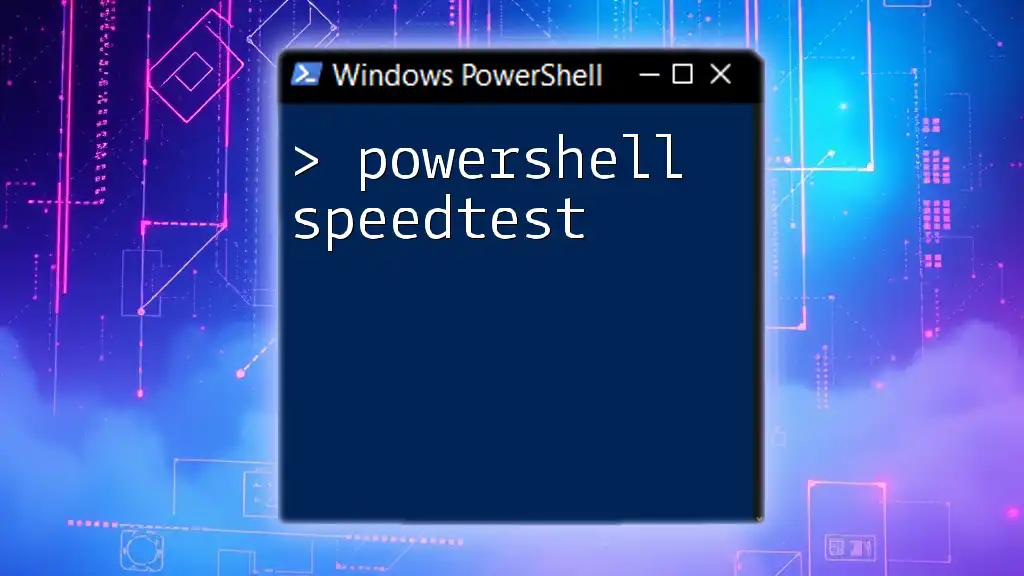
Practical Use Cases for Escaping Double Quotes
Constructing Complex Strings
When building strings with JSON or similar formats, escaping double quotes becomes essential. For example:
$json = '{ "name": "John Doe", "message": "He said, `"Hello!`"" }'
Write-Output $json
This properly formats the JSON string, allowing for both variable content and embedded quotes.
Writing Scripts That Interact with JSON and XML
When parsing JSON or XML data, proper escaping cannot be overlooked. For instance, when constructing JSON in PowerShell, you might use:
$data = @{name = "Jane"; quote = "She said, `"Hello!`""}
$json = $data | ConvertTo-Json
Write-Output $json
This output will yield a correctly formatted JSON object, demonstrating how escaping enhances data structure definition.
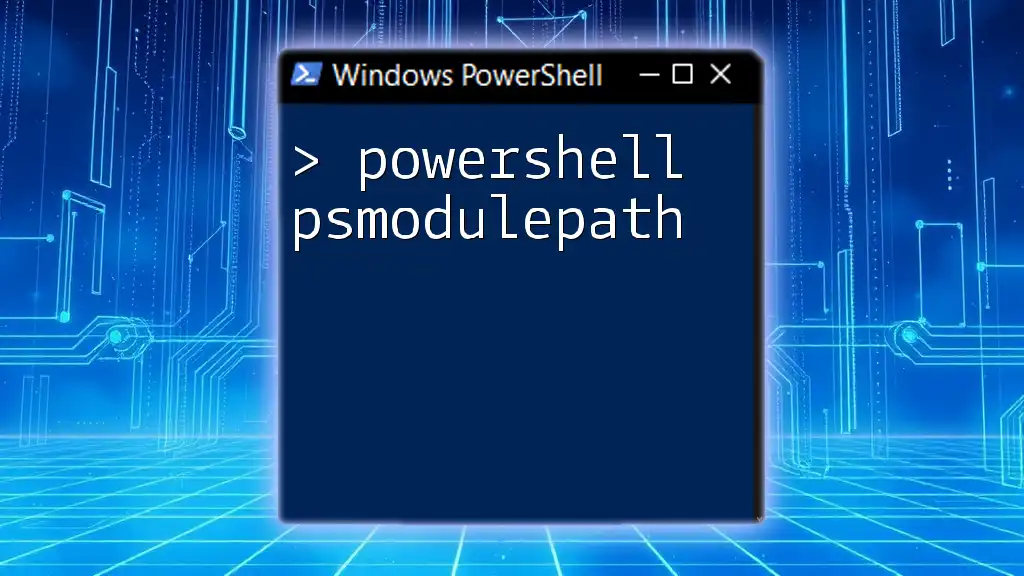
Conclusion
Understanding how to powershell escape double quote is vital for anyone looking to become proficient at scripting in PowerShell. Properly escaping characters can prevent frustrating syntax errors and misinterpretations in variable data. The ability to construct intricate strings while maintaining readability and functionality greatly enriches your scripting capabilities.
If you wish to dive deeper into PowerShell’s intricacies, consider practicing these techniques in real-world scenarios and exploring additional scripting resources.
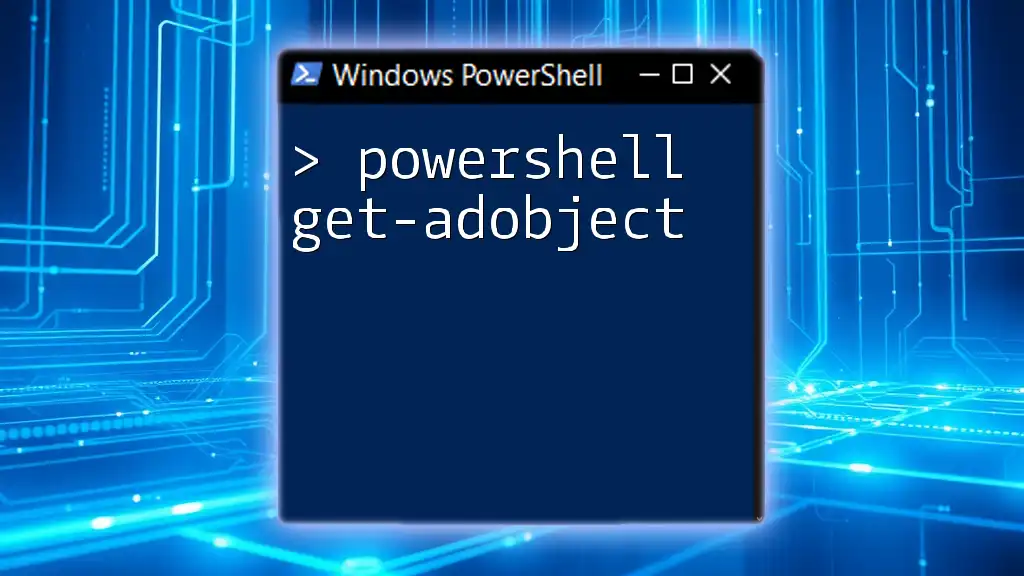
Additional Resources
For further exploration of PowerShell scripting techniques, consider checking out tutorials, books, or online courses that focus on practical applications and advanced concepts. Engaging with communities can also provide valuable insights and practical tips to enhance your learning journey.
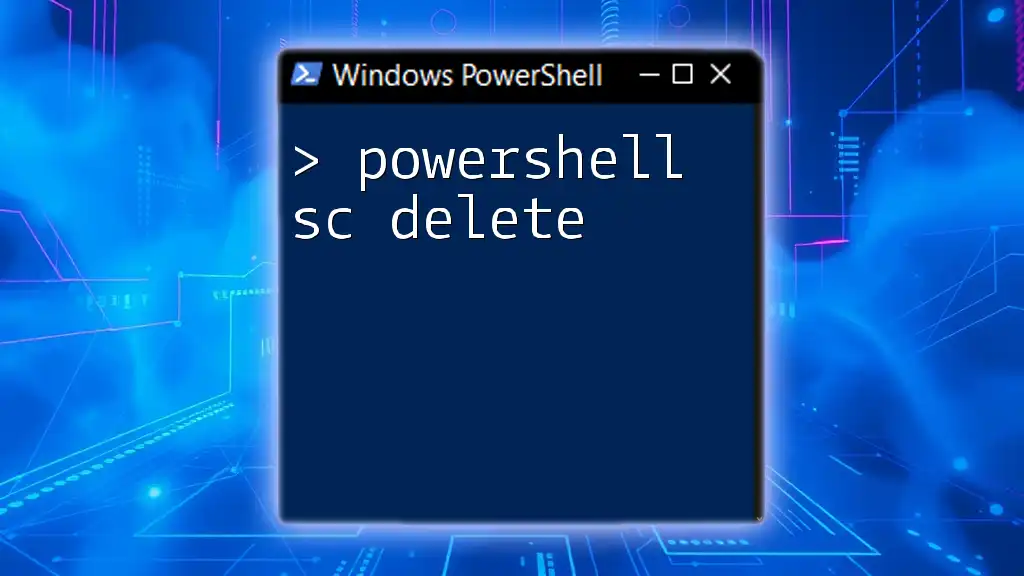
Frequently Asked Questions
What is escaping in PowerShell?
Escaping refers to the method of using specific characters (like the backtick) to indicate that the following character should be treated differently.
Why would I use single quotes over double quotes?
Single quotes are preferred when you want to include text literally without variable expansion or when you want to simplify string creation without worrying about escaping double quotes.
How do I escape double quotes when using them in a string?
You can escape double quotes in a string by using the backtick character (`) before the double quote, or by using single quotes to avoid the need for escaping altogether.