In PowerShell, you can escape a single quote within a string by using two consecutive single quotes.
Write-Host 'That''s how you escape a single quote!'
Understanding Quotes in PowerShell
What Are Single and Double Quotes?
In PowerShell, quotes are used to define string literals. Single quotes (`'`) and double quotes (`"`) serve different purposes.
-
Single Quotes: Strings enclosed in single quotes are treated as literal strings. This means that any characters within the quotes are interpreted exactly as they are shown, including special characters like `$` and `\`. For instance:
$literalString = 'This string includes a dollar sign: $'
-
Double Quotes: Conversely, double quotes interpret strings differently. Variables and expressions enclosed in double quotes are evaluated. For example:
$name = "World" $greeting = "Hello, $name!"
Understanding this distinction is critical for avoiding unexpected results when scripting.
Why Escape Single Quotes?
Escaping single quotes is necessary when a string itself contains a single quote, as unescaped quotes can lead to syntax errors or incorrect outputs.
For example, if you attempt to write:
$message = 'It's a beautiful day!'
You will encounter an error because PowerShell interprets the string as ending after the `t`. This is why it’s essential to know how to escape single quotes correctly.
Common Issues
- Syntax Errors: Omitting escape sequences can cause your script to fail during execution or misinterpret the string.
- Unexpected Behavior: Strings might yield different results if the quotes aren't properly managed, leading to confusion and debugging headaches.

Escaping Single Quotes in PowerShell
Basic Escaping Techniques
The most straightforward method to escape single quotes in PowerShell is by using the backtick character (`).
For instance, to declare a string that includes a single quote:
$escapedString = 'It`'s a sunny day.'
The backtick before the single quote tells PowerShell to treat the single quote as a literal character rather than a string delimiter.
Using Backticks: Details
While the backtick is a quick fix, it's vital to remember that it must immediately precede the character it is escaping. In longer strings, this can become tricky, making it essential to maintain clarity.
Using Double Quotes as an Alternative
In scenarios where you find yourself needing to escape single quotes frequently, using double quotes can be a more straightforward solution. When you use double quotes, the single quote doesn’t need to be escaped:
$noEscapeString = "It's a sunny day."
This method can simplify your code and improve readability, especially when dealing with longer strings containing both single and double quotes.

Advanced Techniques for Escaping Single Quotes
Using Here-Strings
Here-strings are a powerful feature in PowerShell that can be incredibly useful when your string contains multiple lines or requires multiple quotes without the hassle of escaping.
A here-string starts with `@"` and ends with `@"`, allowing you to freely use both single and double quotes within its content without escaping:
$hereString = @"
This example contains single quotes: '
And it also includes double quotes: "
Both quote types are safe here.
"@
Here-strings provide a cleaner, more readable format for longer texts or complex strings, and they maintain all characters as they are.
Using String Replacement
Another approach is to dynamically replace single quotes in your strings using the `-replace` operator. This method is particularly useful if you are handling user input or working with strings that may have variable content.
For example, if you have:
$originalString = "It's a sunny day."
You can replace single quotes by doubling them, which is a convention used in many SQL queries and other scripting languages:
$escapedString = $originalString -replace "'", "''"
This method can be beneficial when building queries or preparing strings for environments where quotes have specific meanings.
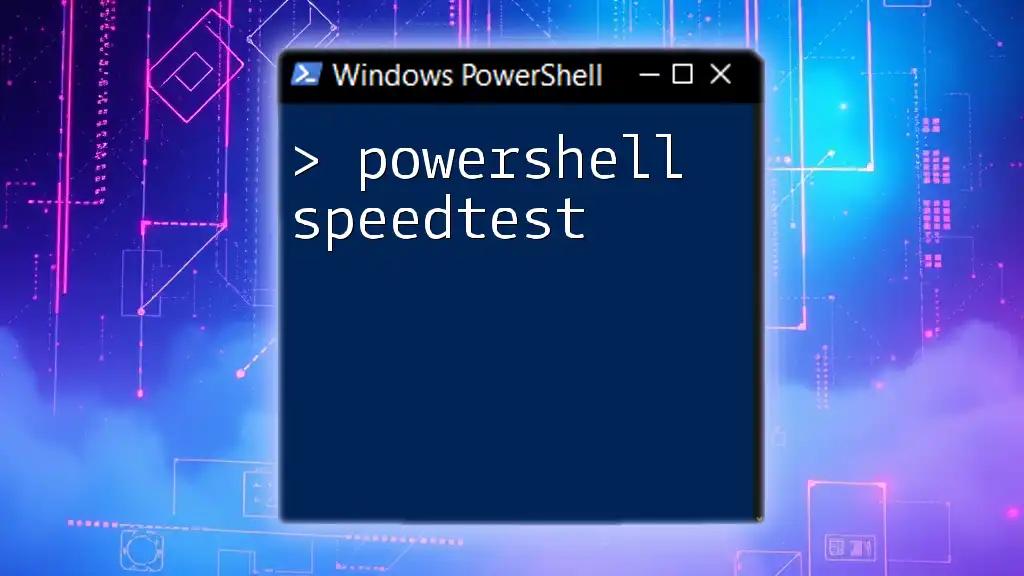
Best Practices for Handling Single Quotes
Consistency is Key
To avoid confusion in your scripts, it's essential to be consistent with your choice of quotes. Establish a standard in your coding practices—decide if you will primarily use single or double quotes—and stick to it throughout your scripts.
Documentation and Comments
Another best practice involves documenting your choices within your scripts. Using comments to explain why you've chosen a particular quoting method or how you handle escaping can help others (and your future self) understand your logic.
Testing Your Scripts
Always test your PowerShell scripts in a safe environment before running them in production. This helps identify any issues related to quote escaping before they can cause significant problems.
Utilizing debugging features or simple print statements can help pinpoint where strings might be incorrectly interpreted due to quoting issues.

Conclusion
In summary, mastering how to handle and escape single quotes in PowerShell is crucial for any user looking to develop efficient scripts. By familiarizing yourself with the various methods for escaping quotes—such as using backticks, double quotes, here-strings, and string replacements—you can avoid common pitfalls and write cleaner, more effective code.
Become adept at managing quotes, and you'll find that your overall PowerShell scripting experience becomes much smoother and more productive.
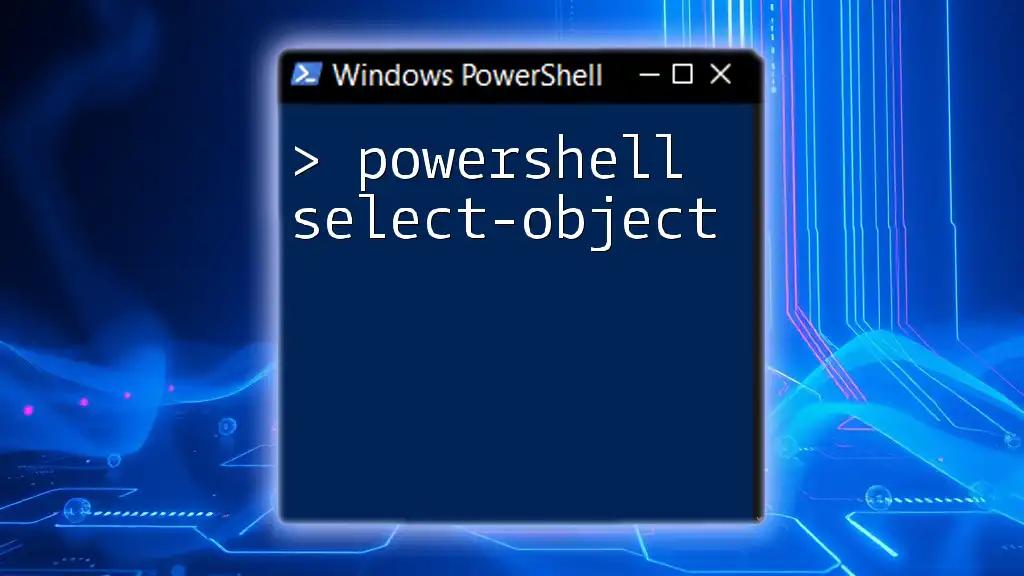
Additional Resources
To further your understanding of PowerShell and string handling, consider exploring official PowerShell documentation or joining community forums where you can learn from other users' experiences. Tools such as PowerShell ISE or Visual Studio Code can also enhance your scripting environment, providing features that assist in managing strings effectively.
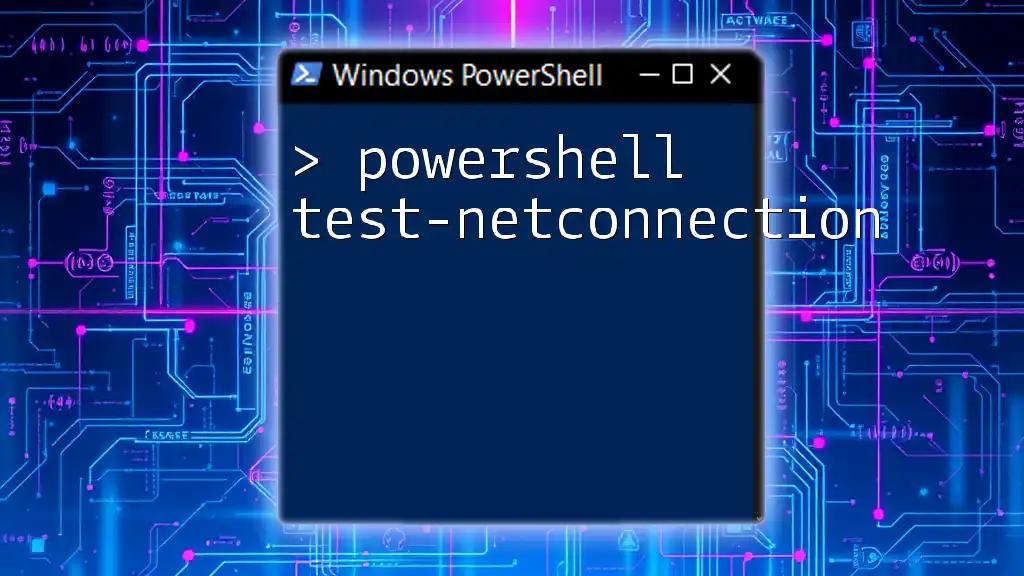
Call to Action
Experiment with these techniques in your own scripts and share your experiences. Engaging with the PowerShell community can provide valuable insights and help deepen your understanding of this powerful scripting language.