In PowerShell, the `-NotNullOrEmpty` operator is used to check if a variable contains a value that is neither null nor an empty string.
Here's a code snippet demonstrating how to use it:
$variable = "Hello, World!"
if (-Not [string]::IsNullOrEmpty($variable)) {
Write-Host "The variable is not null or empty."
} else {
Write-Host "The variable is null or empty."
}
Understanding Null and Empty Values in PowerShell
What is Null in PowerShell?
In PowerShell, null represents a lack of value. It essentially signifies the absence of any object or data type. Null values can arise for various reasons, such as variables that haven't been assigned any value or objects that have been disposed of. Understanding and managing null values is crucial because they can lead to unexpected behaviors or errors in scripts.
To create a null variable, you can simply assign `$null` to it:
$myVariable = $null
This variable, `$myVariable`, is now null and can be checked using various methods.
What is an Empty String?
An empty string is a string of zero length. It is often represented as `""` (two double quotes) in PowerShell. While null means no value, an empty string is a defined value but contains no characters. Recognizing the difference is important for effective scripting.
Here's how you can create an empty string in PowerShell:
$emptyString = ""
In this case, `$emptyString` holds an empty string, which is different from `$null`.
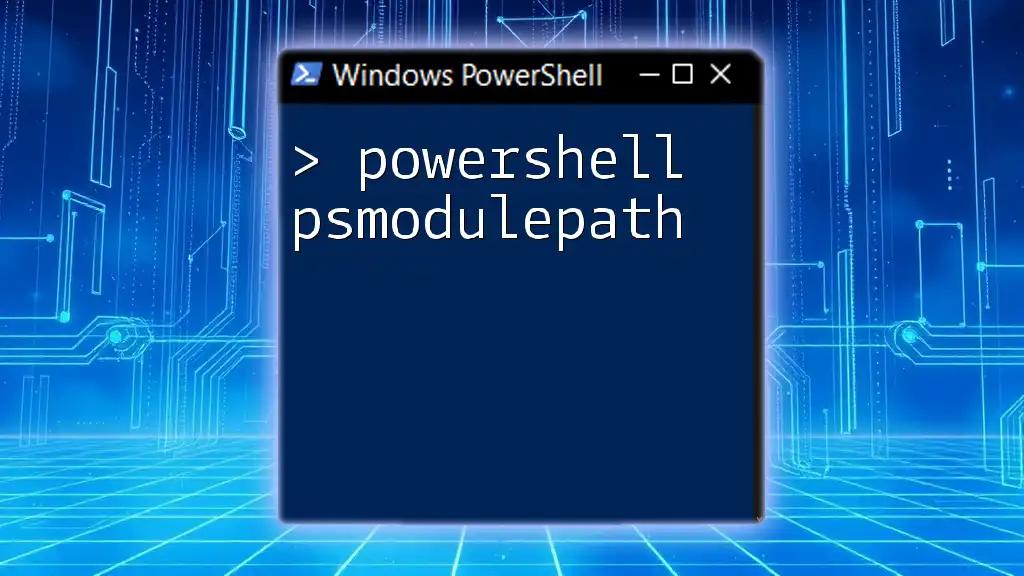
How to Check for Null or Empty Values
Using `IsNullOrEmpty`
The `IsNullOrEmpty` method is a built-in function that checks whether a given string is either null or an empty string. This is essential when validating inputs or conditions in your scripts.
Here’s a quick example:
if ([string]::IsNullOrEmpty($myVariable)) {
Write-Host "The variable is either null or empty."
}
This line effectively checks whether `$myVariable` is null or contains no characters.
Using `IsNotNullOrEmpty`
Conversely, the logic Not Null or Empty checks if a string has a meaningful value. You can construct this check by negating `IsNullOrEmpty`. Checking for non-null and non-empty values allows for more robust validation in scripts.
Here’s how you can implement this:
if (-not [string]::IsNullOrEmpty($myVariable)) {
Write-Host "The variable has a value!"
}
This conditional will execute if `$myVariable` contains a valid, non-empty string.
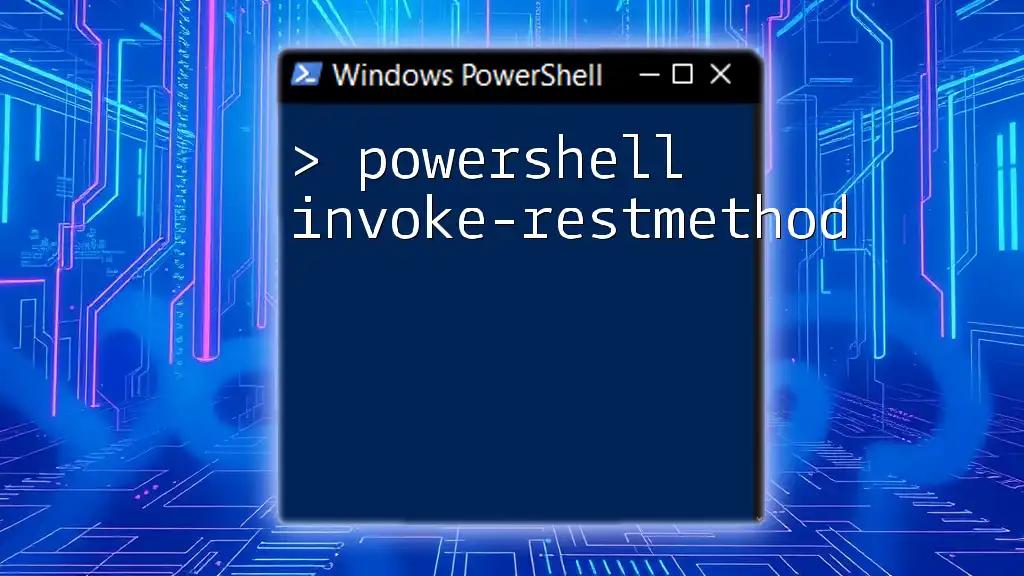
Practical Examples of Using `IsNotNullOrEmpty`
Common Scenarios
Often, you may need to verify user input in scripts, such as during form validation or before processing data. It's crucial to ensure that the input provided is neither null nor an empty value.
Consider this example where you are asking for user input:
$userInput = Read-Host "Please enter your name"
if (-not [string]::IsNullOrEmpty($userInput)) {
Write-Host "Hello, $userInput!"
} else {
Write-Host "You must enter a name."
}
In this snippet, if the user fails to provide a name, the script prompts them further, ensuring that your logic does not proceed with null or empty values.
Combining Conditions
You can also extend your validation by combining multiple conditions. This is particularly helpful when validating configurations or multiple user inputs. Consider a scenario where you check both a username and a password:
$username = "admin"
$password = ""
if (-not [string]::IsNullOrEmpty($username) -and -not [string]::IsNullOrEmpty($password)) {
Write-Host "Both username and password are provided."
} else {
Write-Host "Please ensure both username and password are filled."
}
Using control flow statements like this prepares your script to handle multiple checks efficiently and avoids potential pitfalls.
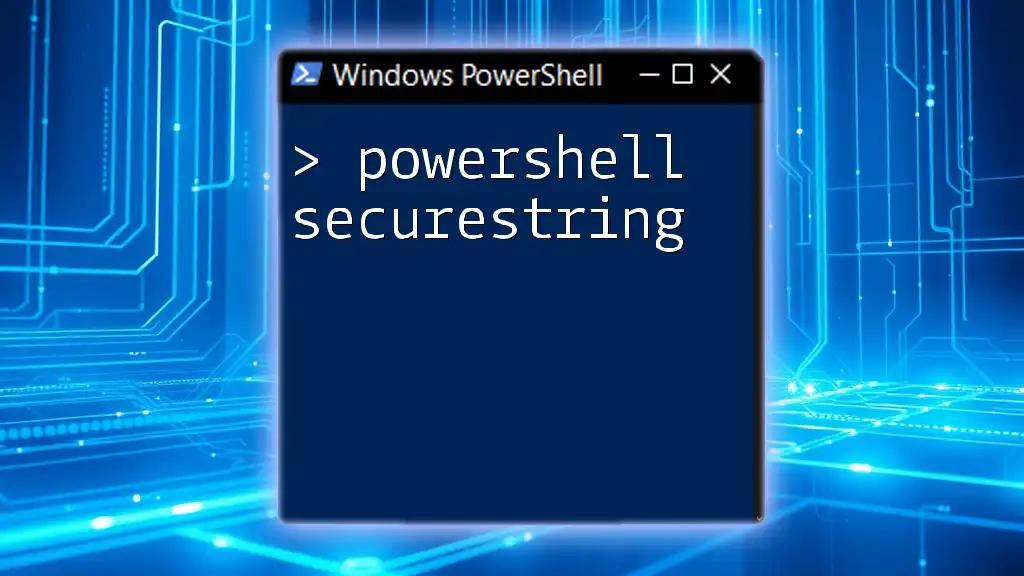
Troubleshooting Common Issues
Unexpected Results
When checking for null or empty values, scripts might yield unexpected results if not written carefully. Common pitfalls include assuming an uninitialized variable is automatically null, or neglecting whitespace, which can lead to mistakenly identifying a string as empty.
Always remember to initialize your variables properly. If you suspect issues, use the following for debugging:
Write-Host "Value of myVariable: '$myVariable'"
This will clearly show you the content of the variable, helping to identify if it's truly null or just an empty string.
Optimizing Code
Efficiency matters, especially in large scripts or loops. When working in performance-sensitive environments, aim for the cleanest and most efficient checks possible.
Instead of repeatedly invoking `[string]::IsNullOrEmpty`, consider creating a function that encapsulates this logic:
function IsNotNullOrEmpty {
param (
[string]$inputString
)
return -not [string]::IsNullOrEmpty($inputString)
}
This abstraction reduces repetitive code, promoting cleaner scripts and improving readability while ensuring every check adheres to the same standard.
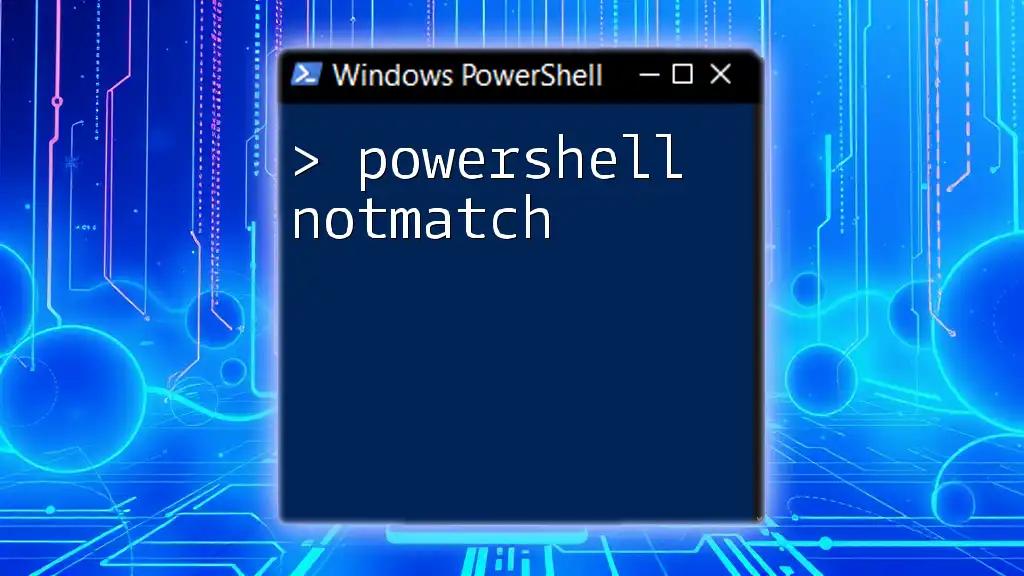
Conclusion
In summary, understanding and using the `IsNotNullOrEmpty` method in PowerShell is crucial for creating robust scripts that handle user input and data validation effectively. By checking for null and empty values, you enhance the reliability of your PowerShell scripts.
Being diligent in these checks not only saves time debugging but also improves the overall workflow of your automation processes. As you explore your PowerShell journey further, remembering these foundational elements will serve you well in your coding practices.
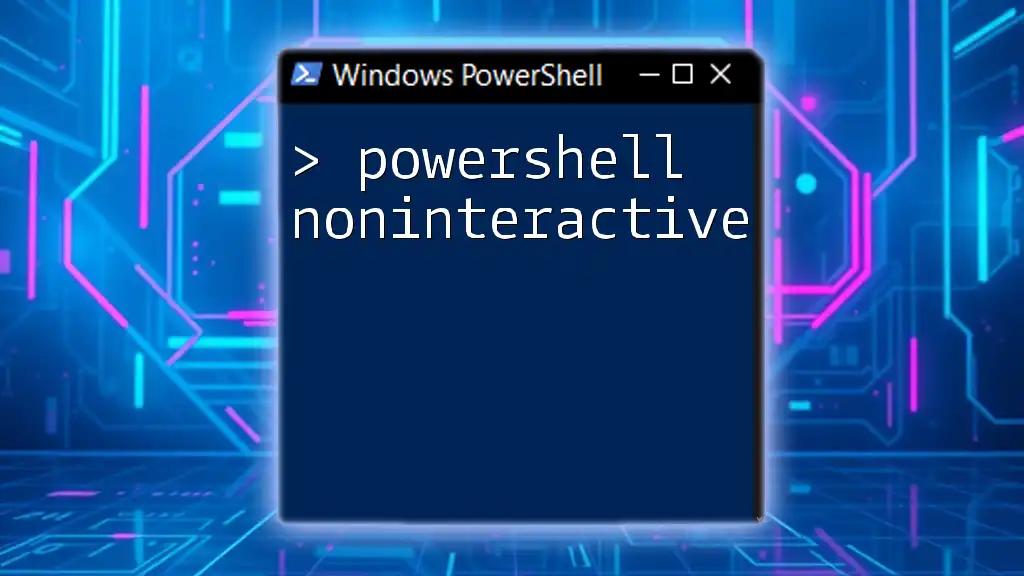
Additional Resources
For deeper insights and advanced techniques, refer to the official PowerShell documentation. Engage in community forums or enroll in specialized courses to enrich your skills and connect with fellow PowerShell enthusiasts.
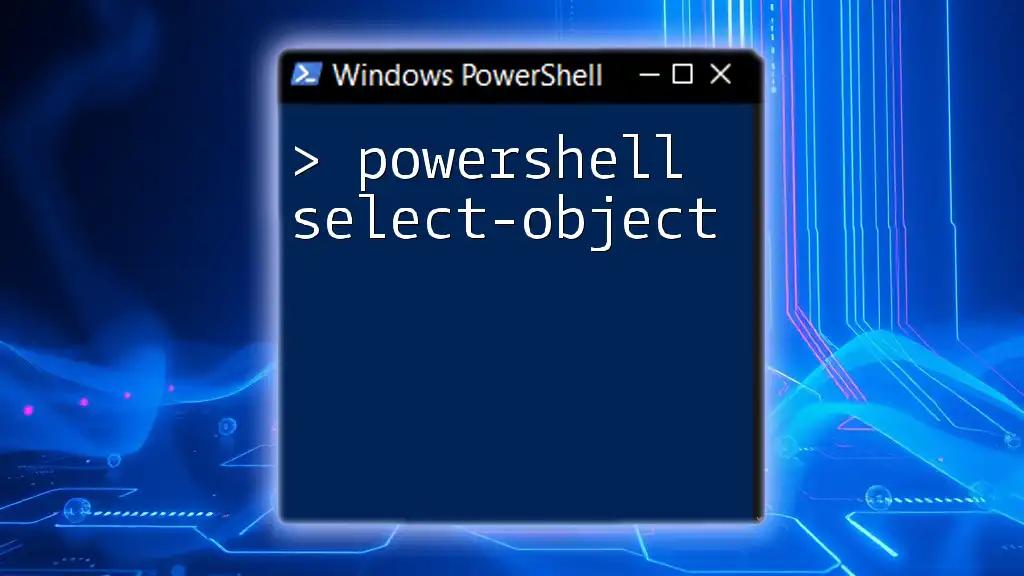
Call to Action
We encourage you to share your experiences with null and empty checks in PowerShell. Your insights could help others facing similar challenges. Don't forget to subscribe for more tips and tricks to mastering PowerShell!