PowerShell format allows you to control the output of your commands by specifying how data is displayed, making it easier to read and understand.
Here's a simple code snippet to demonstrate string formatting using PowerShell:
$Name = "John"
$Age = 30
Write-Host ("My name is {0} and I am {1} years old." -f $Name, $Age)
Understanding PowerShell Format
What is PowerShell Format?
PowerShell format is a crucial aspect of displaying output in a clear and readable manner. Formatting refers to the arrangement and presentation of data, which enhances its visibility and usability for the user. It plays a key role in interpreting and understanding command results, especially when dealing with large datasets or complex objects. Well-formatted output can quickly provide insights and aid in decision-making, making it an essential skill for anyone using PowerShell.
The Role of Format Commands
PowerShell provides several format commands that allow users to present data in various styles according to their needs. The primary formatting commands include:
- Format-Table: Used to display data in a tabular format.
- Format-List: Provides detailed information in a list format, showing all properties of an object.
- Format-Wide: Displays a list of values without additional detail, useful for concise output.
Each of these commands serves a unique purpose and can dramatically alter how information is presented, thus enhancing comprehension.

Format-Table Command
Syntax and Basic Usage
The `Format-Table` command is utilized when you want to display data in a structured table format, which is often easy to read at a glance. The syntax is as follows:
Get-Process | Format-Table
This command retrieves processes and formats the results into a table, showcasing the default properties.
Customizing Table Output
To tailor the table output to suit your specific needs, you can use the `-Property` parameter to select the properties you want displayed. For instance:
Get-Process | Format-Table -Property Name, CPU, Id
In this example, only the Name, CPU usage, and Id are shown, making it simpler to focus on relevant data.
Formatting Options
The `Format-Table` command also supports several formatting options. For example, using `-AutoSize` adjusts column widths to fit content, and `-Wrap` will ensure that long text entries don’t truncate. Consider this code snippet:
Get-Process | Format-Table -Property Name, CPU -AutoSize
You can also group results using `-GroupBy`, which is helpful for organizing similar items, as seen here:
Get-Process | Format-Table -Property Name, CPU -GroupBy ProcessName
This command groups the output by the process name, allowing for quick comparisons.

Format-List Command
When to Use Format-List
The `Format-List` command shines when you need detailed insights into the properties of an object. It's particularly beneficial when working with complex objects where multiple attributes are present.
Syntax and Customization
The command structure is straightforward:
Get-EventLog -LogName Application | Format-List
This command provides a detailed output of all properties in the event log, creating a comprehensive view of each log entry.
Selecting Specific Properties
To focus on key information, you can specify properties using the `-Property` parameter. For example:
Get-Service | Format-List -Property Name, Status, DisplayName
This outputs only the Name, Status, and DisplayName of services, making the information more digestible.

Format-Wide Command
Overview and Usage
The `Format-Wide` command is particularly useful for scenarios where you want to list values concisely without additional detail. It’s great for gaining a quick overview of a long list, making it ideal for instances where brevity is key.
Syntax and Examples
To display a simple list using `Format-Wide`, you can use:
Get-Service | Format-Wide -Property Name
This output will show just the names of the services in a single-column format, providing straightforward access to information.
Limitations of Format-Wide
However, it’s important to recognize that `Format-Wide` may not be suitable for all tasks, especially when you need context or relationships between properties. It provides limited data and might obscure critical information necessary for informed decisions.

Additional Formatting Techniques
Format-Custom Command
The `Format-Custom` command allows for creating tailored format configurations that suit specific needs. By defining a custom format, users can dictate precisely how properties are displayed, offering a unique presentation not covered by other commands.
Using Select-Object for Initial Formatting
Before piping to formatting commands, consider using `Select-Object` to refine your output. This cmdlet allows for the selection of particular properties and can simplify subsequent formatting. For example:
Get-Process | Select-Object Name, ID | Format-Table -AutoSize
This combination displays only the Name and ID of processes in a well-structured table, preventing unnecessary details from cluttering the output.
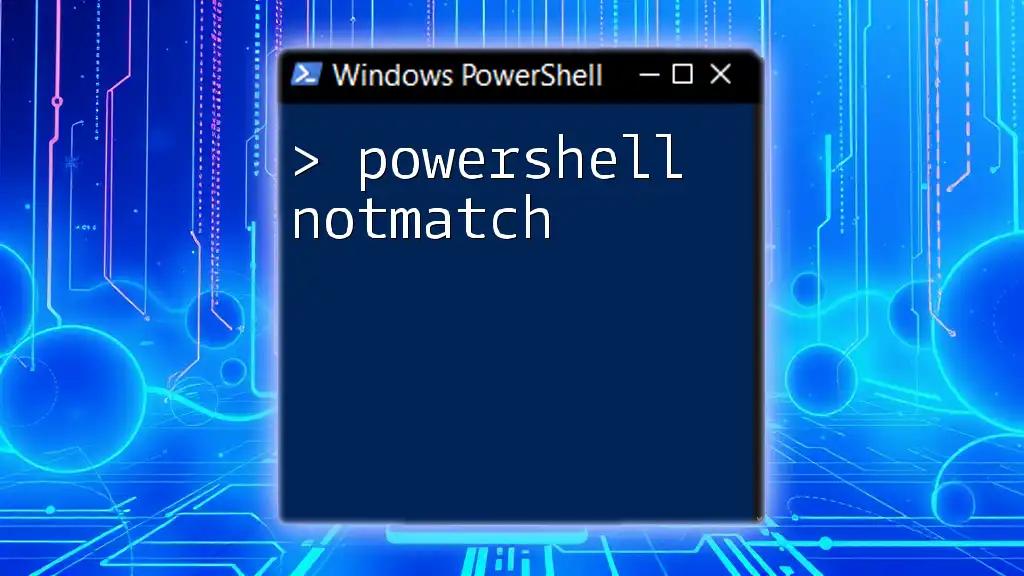
Best Practices for Formatting in PowerShell
Readability Over Complexity
When using PowerShell format features, always prioritize readability. Information should be straightforward, allowing users to quickly grasp the necessary data without wading through convoluted outputs.
Consistent Formatting
Maintaining a consistent format across scripts can enhance usability and provide a familiar environment. This approach fosters efficiency and allows for streamlined collaboration among users.
Leveraging Formatting in Scripts
Integrating formatting commands into scripts significantly improves user experience. For example:
$processes = Get-Process
$processes | Format-Table -Property Name, CPU -AutoSize
In this way, scripts not only execute tasks but also present results in a clear, engaging format.

Troubleshooting Common Formatting Issues
Common Errors in Formatting Commands
While using formatting commands, you may encounter common errors such as missing properties or incorrect syntax. To troubleshoot, always double-check command syntax and ensure that the properties you wish to display exist on the object being formatted.
Confirming Format Output
You can verify that your format has been applied as intended by checking the output carefully, and you might want to use piping to redirect to a file for further analysis or debugging.

Conclusion
Recap of Key Formatting Commands
In this guide, we have explored the essential PowerShell format commands, including Format-Table, Format-List, and Format-Wide. Understanding when and how to use these commands allows for effective data presentation.
Encouragement to Experiment
Experimenting with different formatting options can lead to more intuitive data handling in your PowerShell activities. Challenge yourself to explore these commands, as mastering them will undoubtedly enhance your productivity and effectiveness in PowerShell.
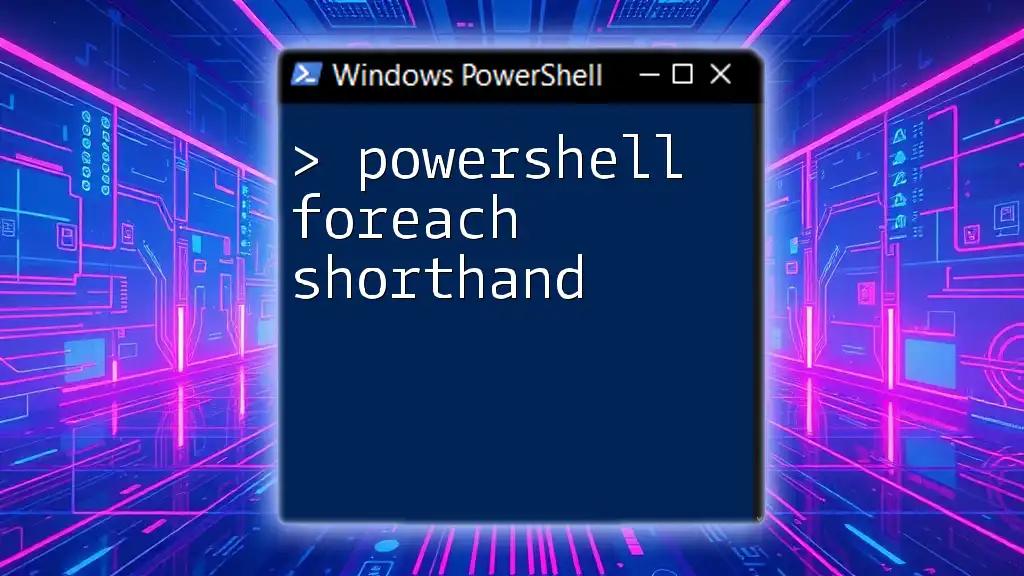
Additional Resources
Further Reading
Consider diving deeper into related documentation, tutorials, and community forums to expand your knowledge of PowerShell formatting techniques.
FAQs
Lastly, reviewing frequently asked questions regarding PowerShell format commands can provide insights into issues you may encounter, enriching your learning and application of best practices.