In PowerShell, you can format the current date in a desired style using the `Get-Date` cmdlet along with the `-Format` parameter.
Get-Date -Format "yyyy-MM-dd"
Understanding DateTime in PowerShell
What is DateTime?
The DateTime structure in .NET is an integral part of how PowerShell handles dates and times. This powerful structure allows you to represent dates and times with precision, enabling scripting and automation for a broad range of applications. In PowerShell, a DateTime object can be easily created and manipulated, making it essential for developers and system administrators alike.
Creating a DateTime Object
Creating a DateTime object in PowerShell can be done using the `Get-Date` cmdlet. This cmdlet retrieves the current date and time or allows for the creation of a specific date and time.
For example, to get the current date and time:
$currentDateTime = Get-Date
Similarly, you can create a DateTime object for a specific date:
$specificDateTime = Get-Date "2023-10-25 14:30"
These simple commands serve as the foundation upon which all date-related operations can be built.

Formatting Dates in PowerShell
The Importance of Date Formatting
Proper date formatting is crucial in scripting. It ensures that the output is readable, understandable, and useful. Correctly formatted dates are essential for reports, logs, and notifications, as they remove ambiguity and promote effective communication.
PowerShell DateTime Format Command
PowerShell provides the capability to format dates using the `ToString()` method in combination with the `-Format` parameter. This allows for easy customization of how date and time information is presented.
For instance:
$currentDateTime.ToString("MM/dd/yyyy")
This command formats the current date into the popular month/day/year structure. Understanding this formatting technique is fundamental to displaying date information accurately.
Common Date and Time Formats
Using Standard DateTime Format Strings
PowerShell offers several built-in standard format strings that simplify the process:
- Short Date Pattern ("d")
- Long Date Pattern ("D")
- General Date/Time Pattern ("g")
Here's how you can use these standard formats:
$currentDateTime.ToString("d") # Short date pattern
$currentDateTime.ToString("D") # Long date pattern
$currentDateTime.ToString("g") # General date/time pattern
Each of these formats serves distinct purposes; the short date keeps it simple, the long date provides context, and the general pattern gives a quick snapshot of both date and time.
Custom DateTime Format Strings
If the standard formats do not meet your needs, you can utilize custom format strings, which offer immense flexibility. For instance:
$currentDateTime.ToString("yyyy-MM-dd HH:mm:ss")
In this example, the format `yyyy`, `MM`, `dd`, `HH`, and `mm` specify the year, month, day, hour, and minute, respectively. Custom formats allow you to tailor the output exactly to your requirements.

Converting Dates to String
PowerShell Date to String Conversion
In many cases, it's necessary to convert a DateTime object to a string for various applications or integrations. The conversion process can be seamlessly performed with the `ToString()` method.
For example:
$dateString = $currentDateTime.ToString("yyyy-MM-dd")
This command converts the current date into a string formatted as year-month-day, making it easy to use in file names or API calls.
Handling Time Zones
Understanding Time Zone Adjustments
Time zone considerations are essential when dealing with date and time data, especially in global applications. You can adjust for time zones using the .NET System.TimeZoneInfo class.
For example:
$timeZone = [System.TimeZoneInfo]::FindSystemTimeZoneById("Eastern Standard Time")
$adjustedTime = [System.TimeZoneInfo]::ConvertTime($currentDateTime, $timeZone)
This snippet converts the current date and time to Eastern Standard Time. Understanding how to manage time zones ensures that you avoid common errors when scheduling events or logging activities across different locations.
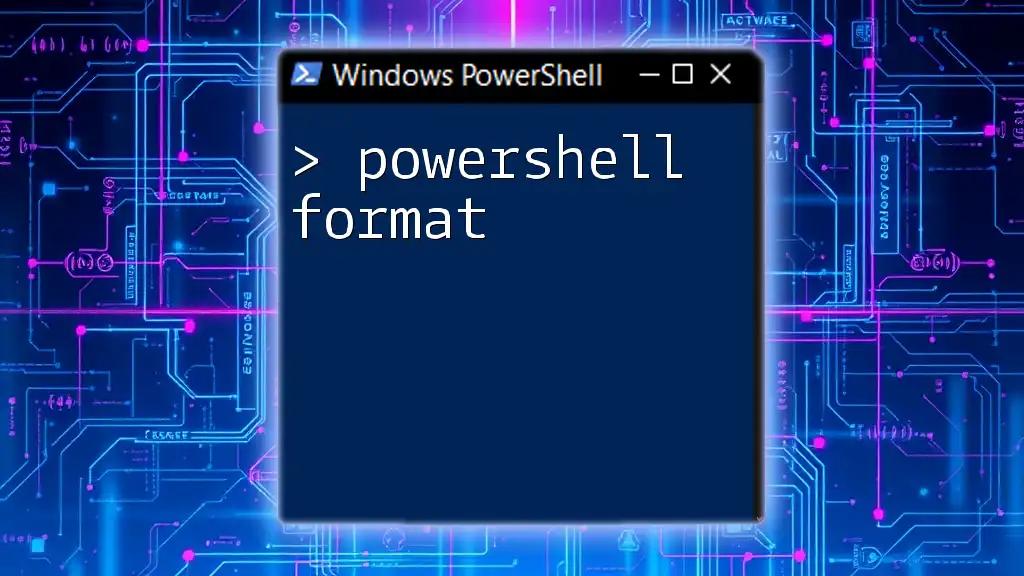
Practical Use Cases of Date Formatting in PowerShell
Logging and Monitoring Scripts
Formatted dates are invaluable in logging and monitoring scripts. Readable timestamps enhance the utility and clarity of logs, enabling better troubleshooting and analysis.
Scheduling Tasks
When automating tasks, precise date formatting is crucial. For instance, if you're scheduling tasks using Windows Task Scheduler, formatted dates ensure the tasks run at the correct times, avoiding confusion and errors.
Generating Reports
In any reporting script, formatted dates are vital for clarity and professionalism. They ensure that stakeholders understand the timing of data. A well-formatted date adds legitimacy and enhances the interpretation of reports.

Troubleshooting Common Issues
Common Date Formatting Errors
Despite the flexibility and power of PowerShell's date formatting capabilities, there are common pitfalls. Users often experience culture-specific format issues or run into trouble with invalid dates.
Best practices involve:
- Always validating user input when dealing with date strings.
- Utilizing tried-and-tested format strings to avoid ambiguity.
Debugging Date Variables
When working with date variables, it’s crucial to verify their types to ensure smooth processing. You can easily check the type of a date variable by:
$dateType = $currentDateTime.GetType()
Understanding the importance of variable types prevents unexpected behavior in scripts, fostering a more reliable coding environment.
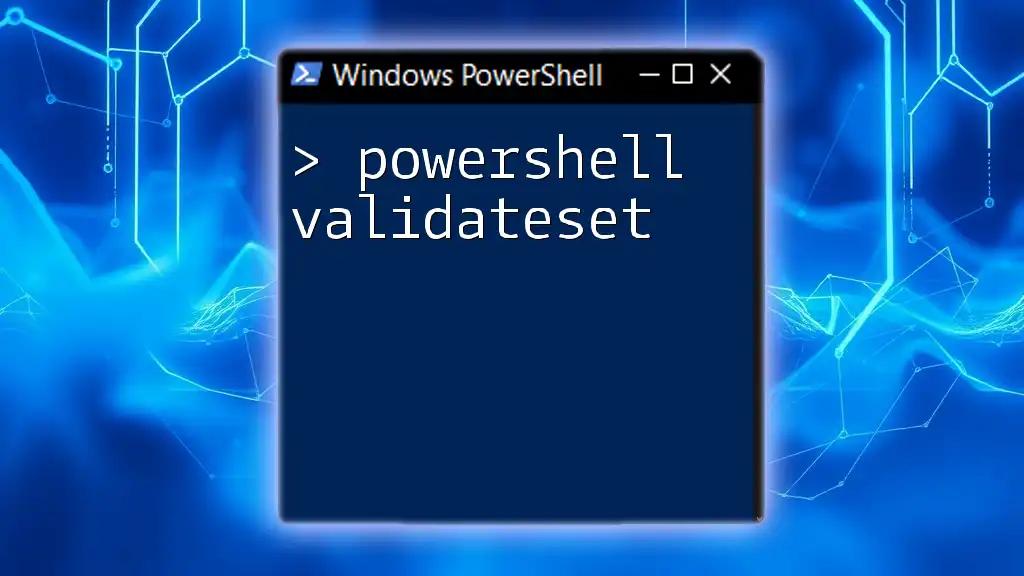
Conclusion
In conclusion, mastering the command to format dates in PowerShell is key for anyone working within the PowerShell environment. From logging to scheduling, effective date management saves time and eliminates confusion. Whether using built-in standard formats or creating custom strings, these techniques enhance your scripting abilities. Practice and experimentation will lead to better automation scripts and effective solutions as you continue to explore PowerShell’s powerful features.
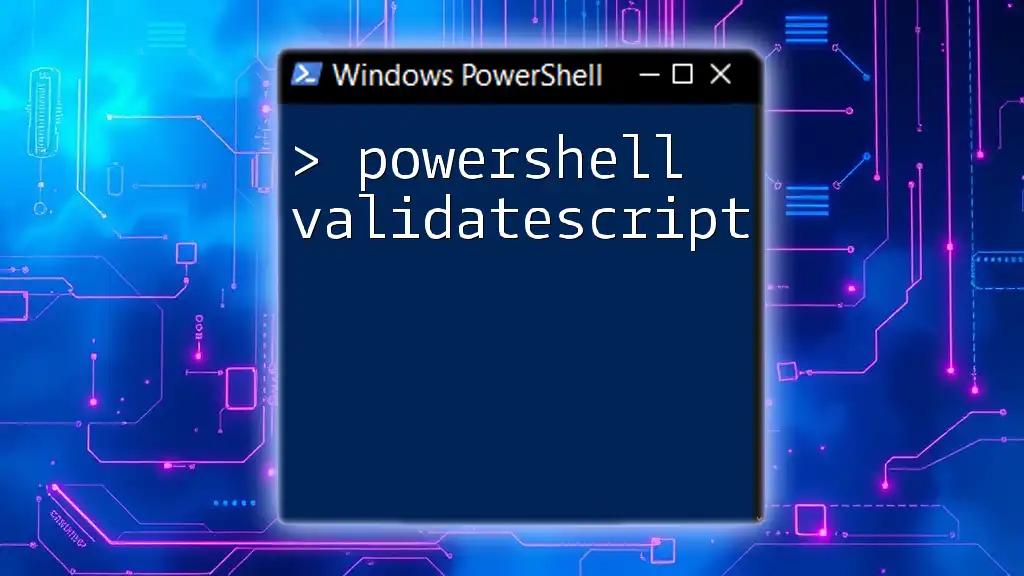
Additional Resources
For those interested in delving deeper into PowerShell's date and time functionalities, I recommend exploring the official PowerShell documentation. Here, you can find more examples and best practices, aiding your journey toward mastering PowerShell scripting. Additionally, consider joining our training sessions to unlock the full potential of PowerShell in your projects.