In PowerShell, you can format numbers using the `-f` format operator, which allows you to specify the format, such as grouping for thousands or setting decimal places.
$number = 1234567.89
$formattedNumber = "{0:N2}" -f $number
Write-Host $formattedNumber # Output: 1,234,567.89
Understanding Number Formatting in PowerShell
What is Number Formatting?
Number formatting is the process of representing numbers in a more understandable and visually appealing way. It involves controlling how numerical values are shown, which can significantly enhance readability and interpretation. In scripting, particularly with PowerShell, proper number formatting ensures that outputs are correct and suited for the intended audience.
Default Number Format in PowerShell
By default, PowerShell handles numbers in a straightforward manner, displaying them as they are input. For instance, if you enter a number:
$number = 1234567.89
$number
This will output:
1234567.89
However, for larger numbers or those involving decimal places, the default format can be less readable. Understanding how to manipulate formats can improve the clarity of your scripts.

Exploring Format-Number Cmdlet
Introduction to Format-Number
The `Format-Number` cmdlet enhances the way numbers are presented in PowerShell. It is particularly useful when preparing data for reports or when making output more digestible for users who may not be familiar with numerical data.
Basic Syntax of Format-Number
The basic syntax for using the `Format-Number` cmdlet is straightforward. Here’s how you can utilize it:
Format-Number -Number 1234.5678
This will give you a formatted output of the number specified. Here’s an example:
"Formatted Number: $(Format-Number 1234.5678)"
Output:
Formatted Number: 1235

Custom Number Formats
Overview of Custom Formats
Custom formats allow users to dictate exactly how they want numbers to appear. This can include specifying decimal places, achieving percentage representations, or adding commas for thousands. This level of control is essential in professional settings where precision matters.
Specifying Decimal Places
To specify the number of decimal places, you can use the following format:
"{0:N2}" -f 1234.5678
This will round the number to two decimal places, resulting in an output of:
1,234.57
Formatting Percentages
Formatting numbers as percentages can clarify values that represent proportions. In PowerShell, you can achieve this with:
"{0:P1}" -f 0.1234
This will output:
12.3%
Adding Commas to Numbers
For better readability, especially with larger numbers, you can incorporate commas. The following format does just that:
"{0:N0}" -f 1234567
Output:
1,234,567

Advanced Number Formatting Techniques
Using Culture-Specific Formats
PowerShell supports culture-specific formatting, allowing numbers to be displayed according to regional settings. By using the appropriate culture info, numeric outputs will reflect conventional practices in different cultures:
[System.Globalization.CultureInfo]::CurrentCulture.NumberFormat.CurrencySymbol
Formatting Numbers in Tables
Displaying formatted numbers in tables is essential for presenting data clearly. You can use `Format-Table` in conjunction with custom formatting:
Get-Process | Format-Table ID, @{Label="Memory (MB)"; Expression={[math]::round($_.WorkingSet/1MB, 2)}}
This effectively shows memory usage in megabytes, neatly formatted, making it easier for users to interpret the data at a glance.
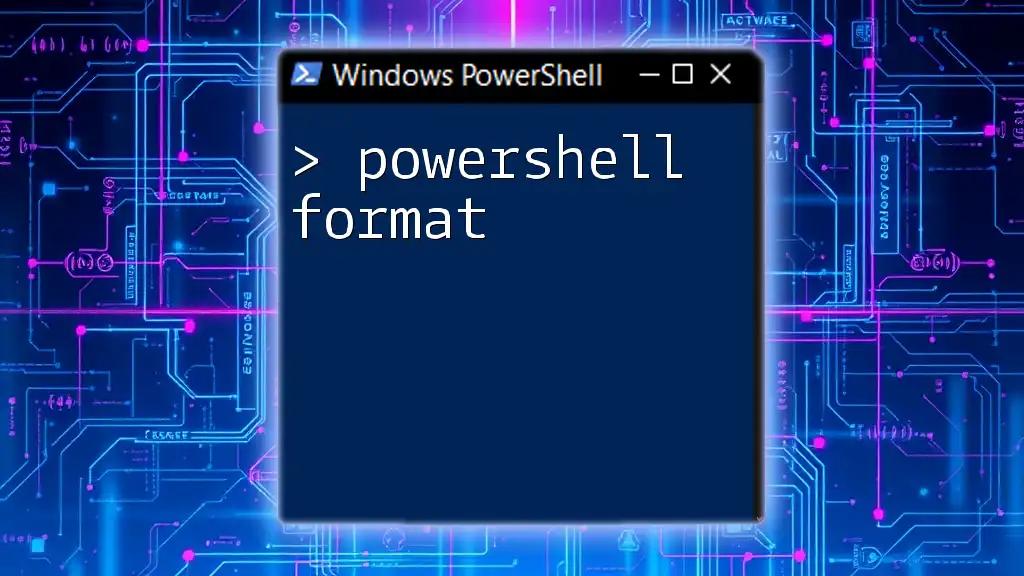
Common Pitfalls and Troubleshooting
Common Formatting Errors
When formatting numbers, users may encounter typical mistakes such as incorrect specifier usage or forgetting to specify the format entirely.
- Example of error: Using `"{0:.2f}"` instead of `"{0:N2}"` for specifying two decimal places.
Troubleshooting Formatting Issues
To tackle common formatting issues, double-check your format strings and ensure they align with PowerShell’s formatting conventions. If you find that numbers are not displaying as intended, revisit your format specifiers and verify that they are correctly defined.

Conclusion
Understanding how to effectively use the `powershell format number` functionality is crucial for anyone looking to enhance their scripting skills. Proper number formatting not only improves the aesthetics of your outputs but also clarifies the data for your audience. As you experiment with different formatting techniques, you’ll become more adept at presenting numerical information accurately and attractively.
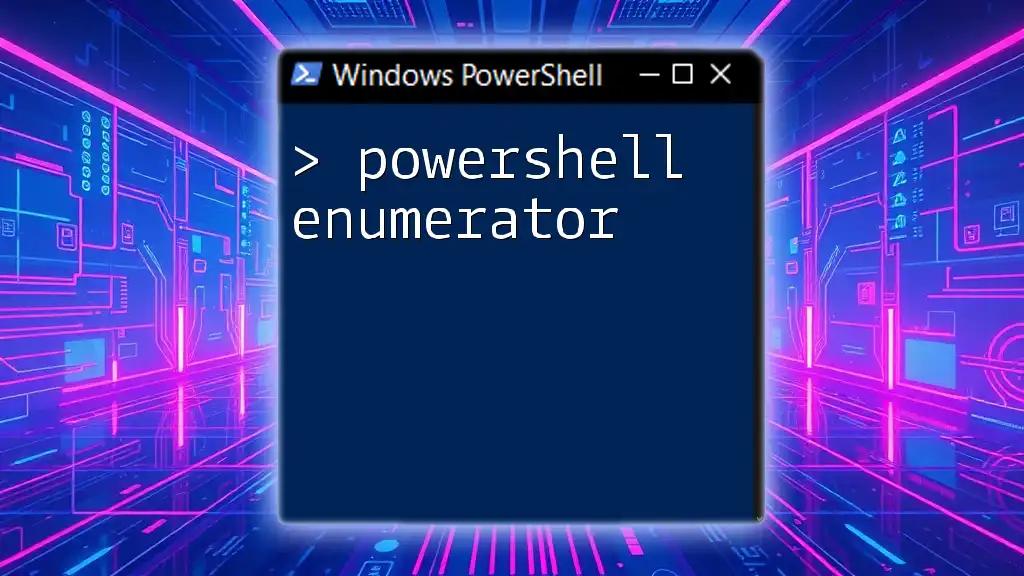
Additional Resources
To deepen your understanding of number formatting, refer to the official PowerShell documentation and look out for articles and tutorials specifically focused on practical applications in various scenarios.
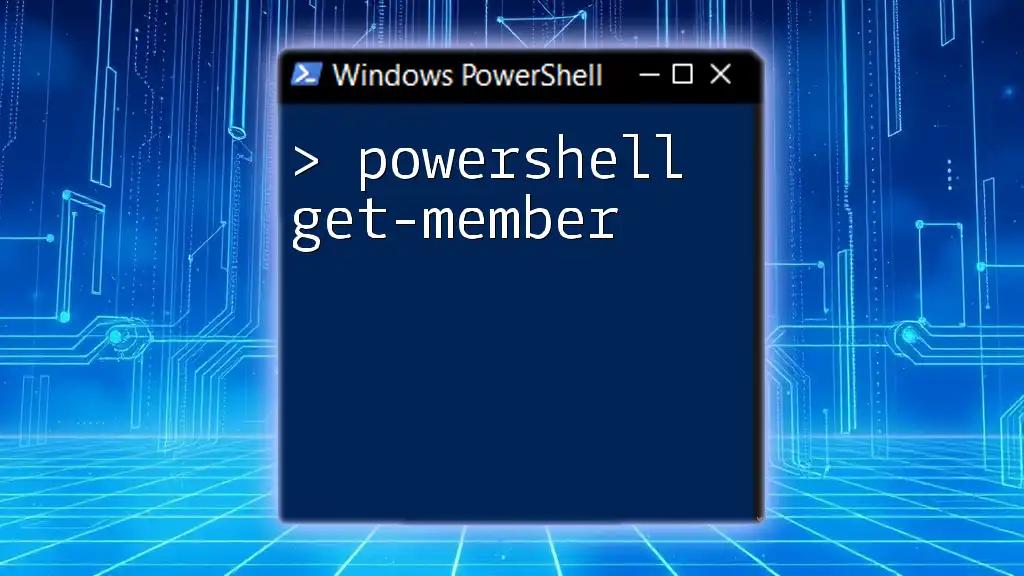
Call to Action
You’re encouraged to share your experiences with number formatting in PowerShell. Have questions or want to discuss specific scenarios? Leave a comment below and join the conversation!