PowerShell allows users to perform mathematical operations easily using its built-in capabilities, such as addition, subtraction, multiplication, and division.
Here’s a simple code snippet to demonstrate basic arithmetic:
# Simple arithmetic operations in PowerShell
$a = 10
$b = 5
$sum = $a + $b
$diff = $a - $b
$product = $a * $b
$quotient = $a / $b
Write-Host "Sum: $sum, Difference: $diff, Product: $product, Quotient: $quotient"
Basic Arithmetic Operations in PowerShell
In PowerShell, performing basic arithmetic operations is straightforward and intuitive. You can easily manipulate numbers to get your desired results. The four core arithmetic operations include addition, subtraction, multiplication, and division.
To illustrate these operations, let's look at an example:
$a = 10
$b = 5
$sum = $a + $b
$difference = $a - $b
$product = $a * $b
$quotient = $a / $b
Here’s what happens in this code:
- Addition: The variable `$sum` now contains the value of `15` (10 + 5).
- Subtraction: The variable `$difference` contains `5` (10 - 5).
- Multiplication: The variable `$product` is `50` (10 * 5).
- Division: The variable `$quotient` equals `2` (10 / 5).
These operations can be combined for more complex calculations as well.
Modulus Operator
The modulus operator (`%`) is used to determine the remainder of a division operation. This can be particularly useful in scenarios where you want to check for even or odd numbers or cycle through a range.
Consider this example using the modulus operator:
$remainder = $a % $b
In this case, `$remainder` will equate to `0` because `10` is evenly divisible by `5`. Understanding how to use the modulus operator enriches your functional capability in PowerShell math.
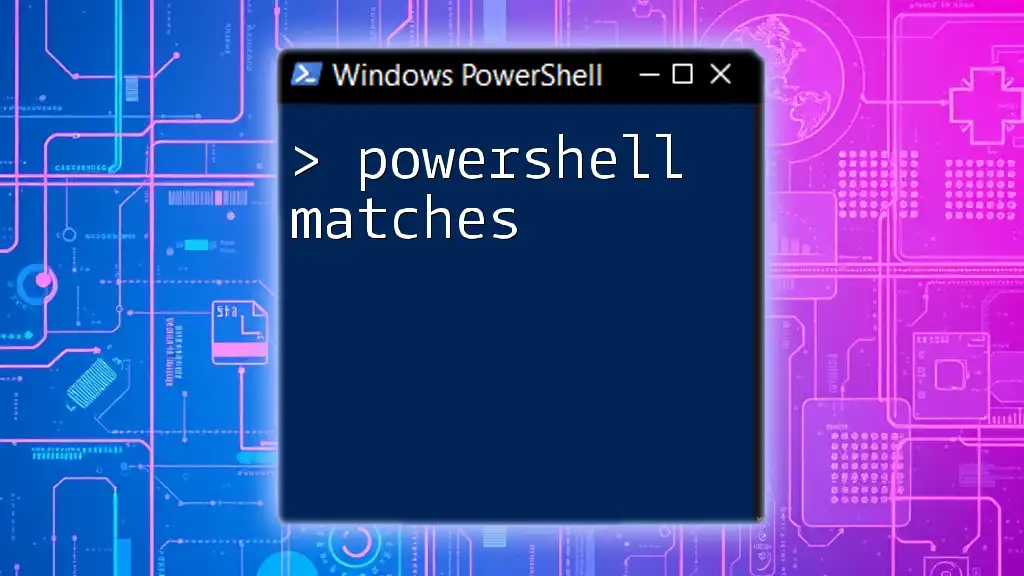
Utilizing PowerShell’s Built-in Math Functions
PowerShell offers various built-in functions, most of which can facilitate more complex calculations without the need to develop custom logic. The powerful `System.Math` class provides a variety of mathematical functions useful in scripting.
Overview of Built-in Functions
Built-in functions simplify calculations significantly by saving time and reducing code complexity. Common methods include mathematical operations such as maximum, minimum, power, and square root.
PowerShell’s `Math` Class
Within PowerShell, the `Math` class can be called directly to leverage several functions:
- Max: Returns the larger of two numbers.
- Min: Returns the smaller of two numbers.
- Pow: Calculates the power of a number.
- Sqrt: Determines the square root.
Here’s how these functions work in practice:
$maxValue = [Math]::Max(10, 20)
$squareRoot = [Math]::Sqrt(49)
In this snippet:
- `$maxValue` will be set to `20`.
- `$squareRoot` will be `7`, highlighting the succinct utility of the Math class.
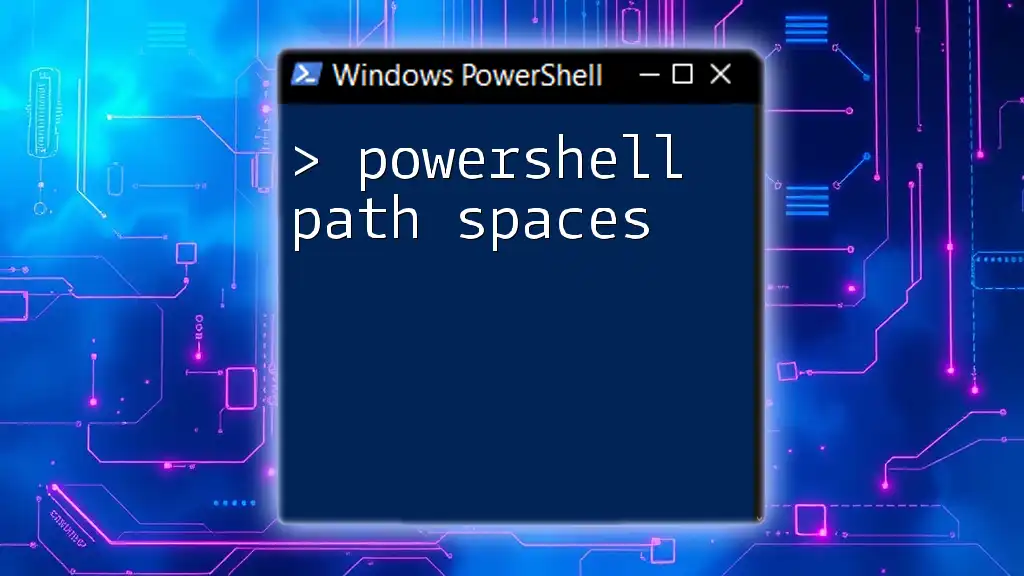
Working with Variables and Data Types
Declaring and manipulating variables with different data types is essential for effective calculations in PowerShell.
Declaring and Using Variables for Math
To perform calculations effectively, we need to declare variables appropriately.
$x = 4
$y = 3
$result = $x * $y + 10
In this example:
- Variables `$x` and `$y` hold the integer values `4` and `3`.
- The expression calculates the product of `$x` and `$y`, adding `10`, which results in `22`.
Type Conversion and Rounding
When handling different data types, type conversion can become necessary. For instance, working with decimal values can lead to different results compared to integers. Additionally, rounding can be crucial for precision in calculations.
Consider the following:
$decimalValue = 4.4567
$roundedValue = [Math]::Round($decimalValue, 2)
Here, `$roundedValue` will equal `4.46`, showcasing the use of the rounding function, which is especially useful in financial calculations to avoid discrepancies.
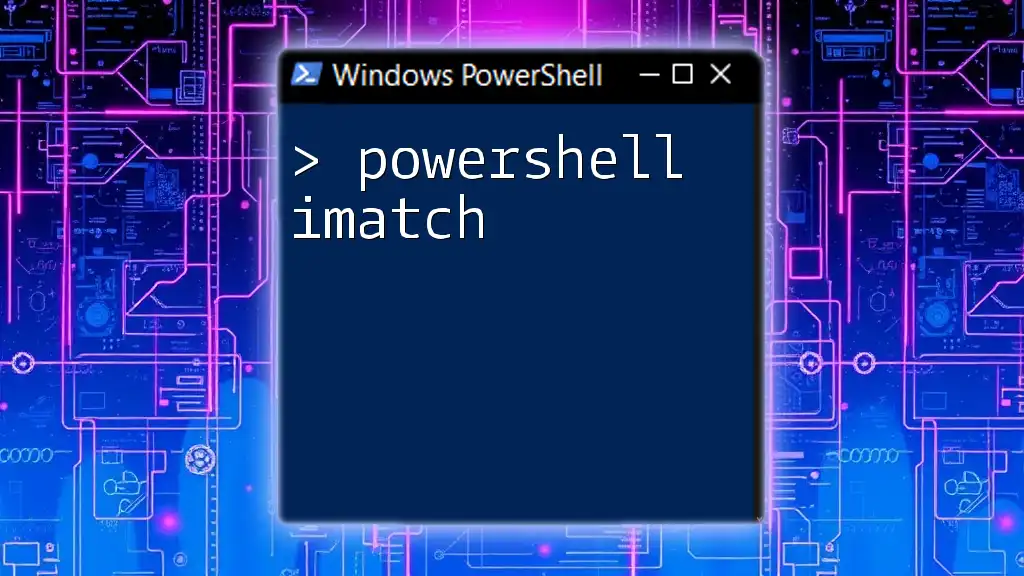
Advanced Mathematical Operations in PowerShell
To leverage the full potential of PowerShell for mathematical operations, it’s useful to explore more advanced functions such as trigonometric, exponential, and logarithmic operations.
Trigonometric Functions
PowerShell incorporates various trigonometric functions as part of its `Math` class. These functions are widely used in various scenarios, such as graphics programming and simulations.
For example:
$angle = 30
$radians = [math]::PI * $angle / 180
$sinValue = [Math]::Sin($radians)
This code converts degrees to radians and calculates the sine value of the given angle. Here, `$sinValue` will contain `0.5`, providing practical applications for geometrical calculations.
Exponential and Logarithmic Functions
Exponential and logarithmic operations are equally important in many fields, such as data analysis and scientific computing.
Consider this code snippet:
$exponential = [Math]::Exp(2)
$logarithm = [Math]::Log(10)
In this case:
- `$exponential` computes e raised to the power of `2`, yielding approximately `7.389`.
- `$logarithm` computes the natural logarithm of `10`.
These functions can help model complex systems, such as growth decay and scaling phenomena.
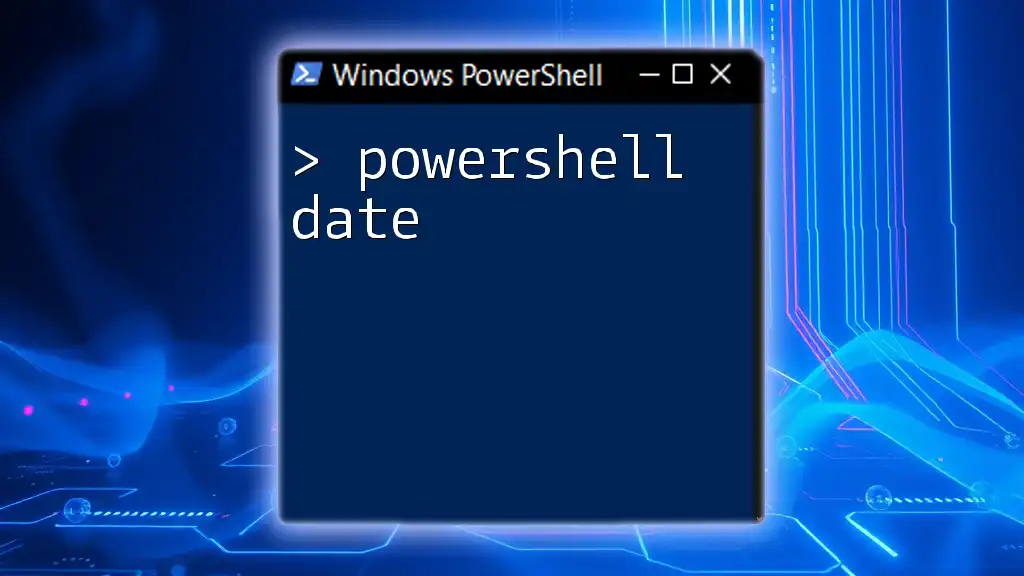
Practical Applications of Math in PowerShell
Automating Calculations in Scripts
One of the most significant advantages of using PowerShell is the ability to automate repetitive tasks effectively. By defining reusable functions, you can streamline your code.
Here's an example function for calculating an invoice:
function Calculate-Invoice {
param (
[decimal]$price,
[int]$quantity
)
return $price * $quantity
}
This function takes the price and the quantity of items, then produces the total invoice amount, making it highly reusable for any set of invoice calculations you may encounter.
Integrating PowerShell Math with Other cmdlets
PowerShell allows you to integrate mathematics with various cmdlets for data processing, enhancing your workflow. For instance, combining math operations with the `Measure-Object` cmdlet can provide valuable insights.
Consider the following code:
$data = Get-Content 'numbers.txt' | Measure-Object -Sum
$average = $data.Sum / $data.Count
In this case, the script retrieves numbers from a file and calculates both the sum and average, demonstrating how mathematics can be effectively merged with data management tasks.
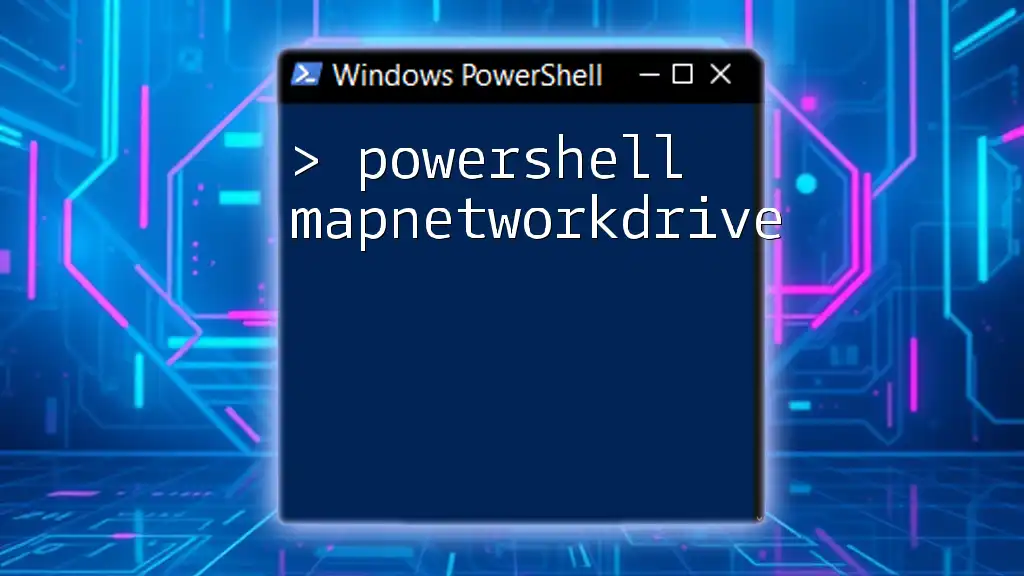
Troubleshooting Common Issues with PowerShell Math
Type Errors in Calculations
Type errors are common pitfalls in programming. When you attempt calculations on incompatible data types, errors will arise. To avoid these, ensure that the variables you are working with are of the correct type before any mathematical operation.
Debugging Complex Calculations
When working on complex mathematical scripts, debugging becomes crucial. Utilize `Write-Host` to provide output for your calculations at various steps, aiding in tracing potential issues in your logic.
In conclusion, mastering PowerShell math equips you with powerful tools and techniques for automating calculations and enhancing your scripting capabilities. The integration of mathematical operations within PowerShell opens a wide array of possibilities for creative problem-solving and data analysis. By harnessing both foundational and advanced mathematical concepts, you can streamline your workflows and achieve efficient scripting results.