In PowerShell, when dealing with file paths that contain spaces, you must encapsulate the path in quotation marks to prevent errors.
Here’s a code snippet demonstrating this:
Get-ChildItem "C:\Program Files\My Application\"
Understanding Paths in PowerShell
What Are Paths?
In PowerShell, paths refer to the locations of files and directories in the file system. There are two types of paths: absolute and relative. An absolute path specifies the location from the root of the file system, while a relative path specifies the location in relation to the current directory. Understanding these path types is essential for efficient navigation and command execution within PowerShell.
Importance of Accurate Paths in Scripts
Accurate paths are crucial for the successful execution of scripts. Using incorrect or improperly formatted paths can lead to script failures. Common path-related errors include file not found messages or permission denied alerts. Therefore, mastering the handling of paths, particularly those containing spaces, is vital for ensuring your scripts run smoothly.
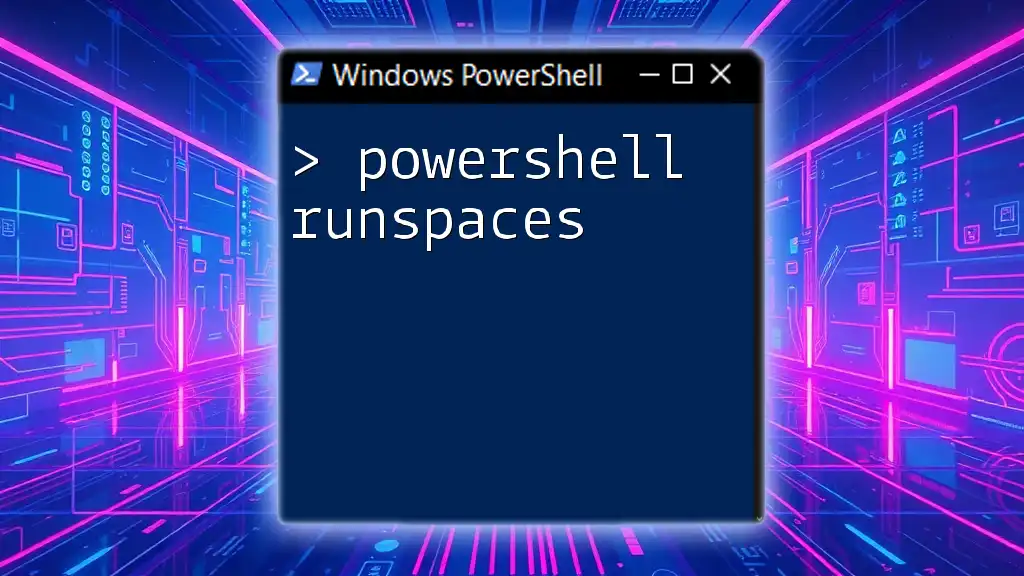
Handling Spaces in PowerShell Paths
The Issue with Spaces
Spaces in paths can complicate command execution. When a path contains spaces, PowerShell may misinterpret the command or parameters, resulting in failures. For instance, a command like `Get-ChildItem C:\Program Files\` without proper handling will not function as intended.
Different Methods to Handle Spaces
Using Quotation Marks
One of the simplest ways to manage paths with spaces is by enclosing them in quotation marks. PowerShell accepts both single (`'`) and double (`"`) quotes, but they serve different purposes. Single quotes are used for literal strings, while double quotes allow for variable expansion.
Example: Using quotes with a command:
Get-ChildItem "C:\Program Files\My App"
In this example, using double quotes ensures that the path is correctly interpreted, allowing the command to execute as intended.
Escaping Spaces with Backticks
Another method of handling spaces is to use the backtick (` `) as an escape character. This approach is particularly useful in scenarios where quotes may complicate the command.
Example: How to use backticks in file paths:
Get-ChildItem C:\Program` Files\My` App
Here, the backticks effectively signal to PowerShell that the spaces are part of the path, allowing the command to proceed without issue.
Using the `&` Call Operator
The call operator (`&`) can also simplify executing commands with paths that include spaces. Using it allows you to treat the command as a single expression.
Example: Running an executable with a path that includes spaces:
& "C:\Program Files\My App\myapp.exe"
By enclosing the path in quotes and using the `&` operator, this command executes successfully by treating the entire path as a single entity.
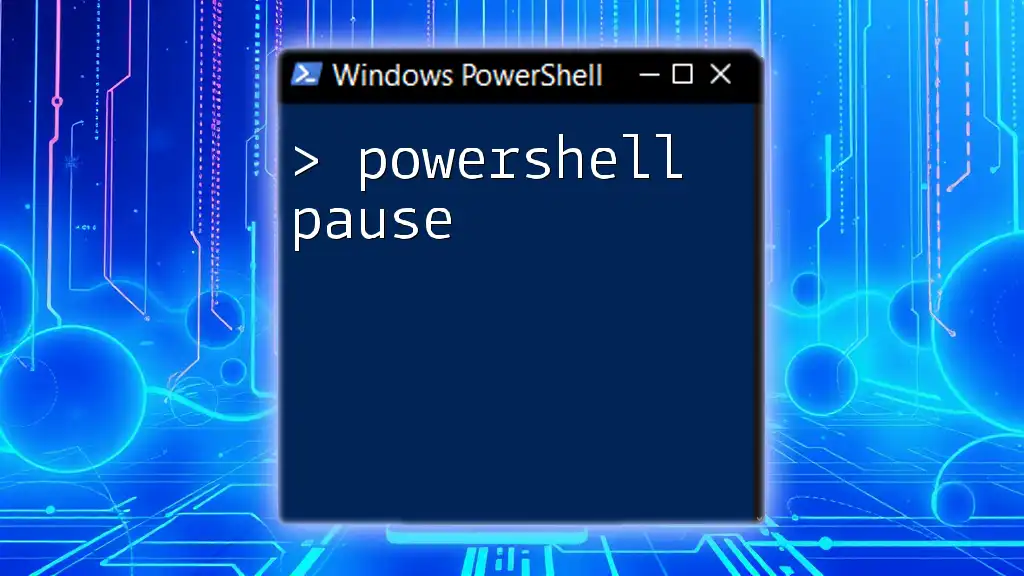
Working with Variables and Paths
Storing Paths in Variables
Using variables to store paths improves readability and maintainability in scripts. By defining a variable, you can reference the path multiple times without repeating the entire string.
Example: Defining a variable with a path including spaces:
$myPath = "C:\Program Files\My App"
Get-ChildItem "$myPath"
In this case, the variable `$myPath` holds the path, making the command concise while ensuring clarity.
Combining Paths Dynamically
PowerShell makes it easy to concatenate strings, enabling the dynamic construction of paths. This is especially helpful in scripts requiring conditional logic or user input.
Example: Building complex paths with split strings:
$folderName = "My App"
$fullPath = "C:\Program Files\$folderName"
Get-ChildItem "$fullPath"
This example demonstrates how to dynamically build paths, ensuring that you handle spaces correctly while maintaining a clean and flexible script structure.
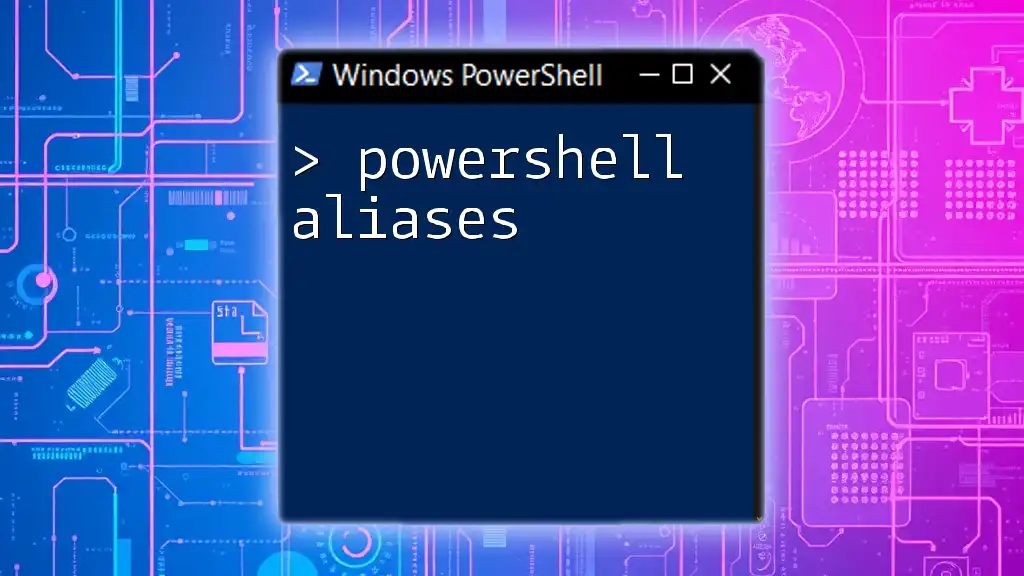
Common Pitfalls with Spaces in PowerShell Paths
Error Messages and Their Meanings
When paths with spaces are not handled properly, you may encounter various error messages. Common issues include "Path not found" errors or warnings about unexpected tokens. Understanding these messages is crucial for diagnosing script problems.
Strategies to Avoid Issues
To prevent issues with spaces in paths, consider implementing the following strategies:
- Always use quotation marks or escaping where appropriate.
- Validate paths before executing commands.
- Utilize variables for better path management.
These practices will help streamline your PowerShell scripting experience, reducing the likelihood of errors.
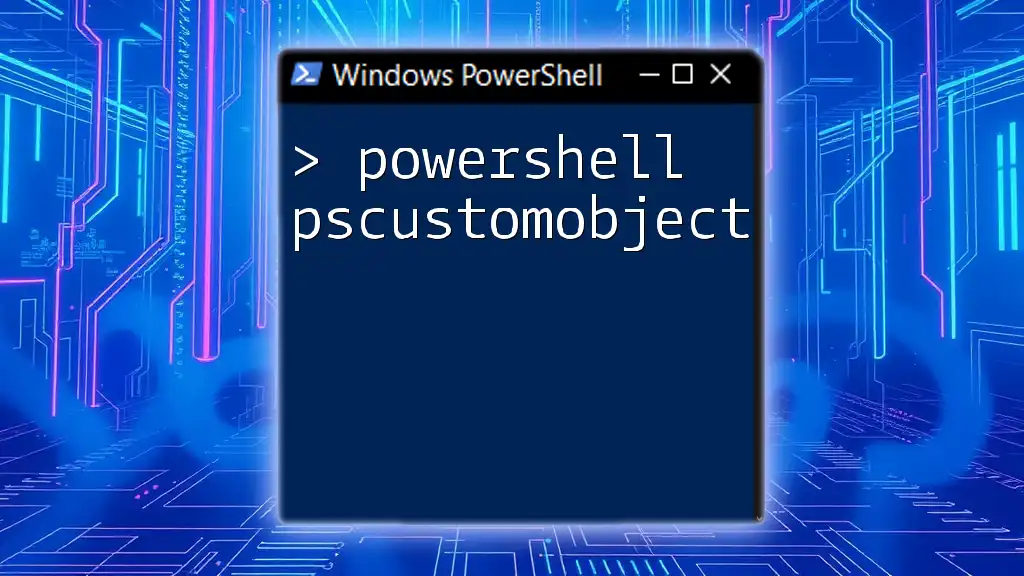
Advanced Techniques
Utilizing PowerShell Cmdlets to Manage Paths
PowerShell provides cmdlets designed to help manage and manipulate paths effectively. One helpful cmdlet is `Join-Path`, which combines a base path with a child path while managing spaces for you automatically.
Example: Using `Join-Path` to handle multi-part paths:
$basePath = "C:\Program Files"
$appPath = "My App"
$fullPath = Join-Path -Path $basePath -ChildPath $appPath
Get-ChildItem "$fullPath"
This approach not only ensures that spaces are managed correctly but also improves the readability of your code.
Creating Functions to Simplify Path Handling
Developing reusable functions can significantly improve your workflow when dealing with paths that contain spaces. A well-defined function can encapsulate the logic for handling paths, allowing you to reuse it across various scripts seamlessly.
Example: A function that normalizes paths with spaces:
function Normalize-Path {
param ([string]$Path)
return "& { "$Path" }"
}
Normalize-Path "C:\Program Files\My App"
Creating such functions reduces redundancy and helps standardize how paths are managed across your scripts.
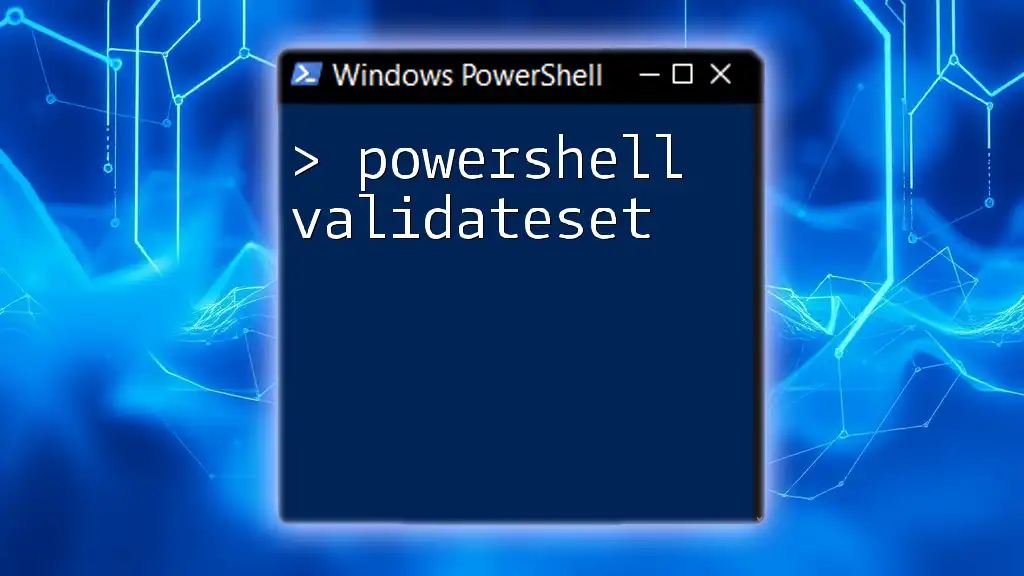
Conclusion
Understanding how to handle PowerShell path spaces is crucial for effective scripting and automation. Using methods like quotation marks, backticks, and call operators allows for the smooth handling of paths, making your commands more robust. By implementing best practices and utilizing features like variables and cmdlets, you can further enhance your path management skills in PowerShell. As you practice, you’ll find that proper handling of paths significantly improves the reliability of your scripts.