To rename a file extension in PowerShell, you can use the `Rename-Item` cmdlet as shown in the following example:
Rename-Item "C:\Path\To\File.txt" "C:\Path\To\File.md"
Understanding File Extensions
What is a File Extension?
A file extension is a suffix at the end of a filename, typically composed of a period (`.`) followed by a few letters. It serves as an indicator of the file type, guiding both the operating system and users regarding how to handle or open that file. For example, a file named `document.txt` has a `.txt` extension, signaling that it's a plain text document.
Importance of File Extensions in PowerShell
In the realm of PowerShell and scripting, file extensions are crucial. They determine how files are processed and managed. Different file types require specific cmdlets and methods for management. For instance, `.jpg` files can be manipulated differently from `.zip` files due to their intrinsic properties.
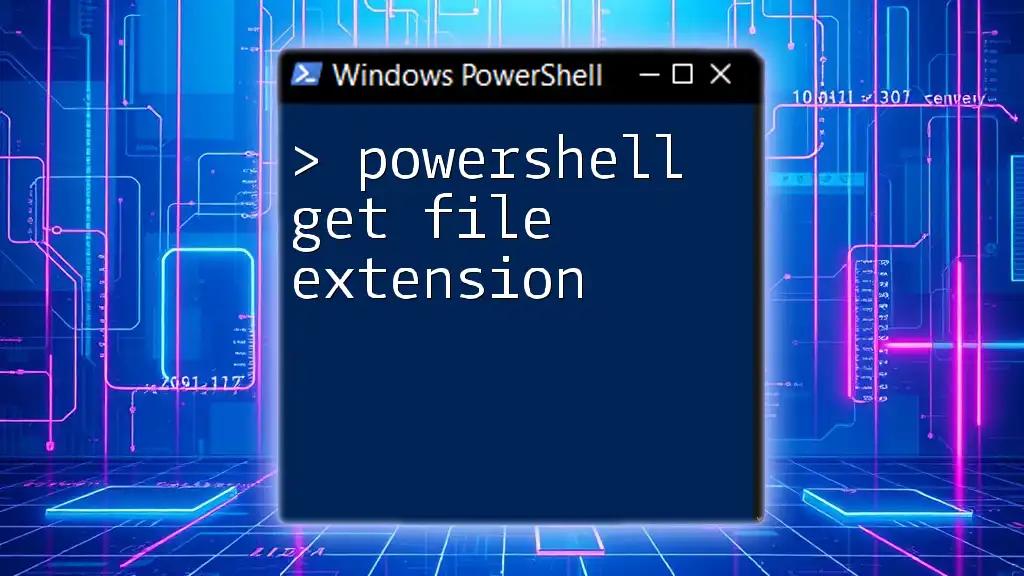
The Basics of Renaming Files in PowerShell
How to Rename Files
To rename files in PowerShell, the primary cmdlet is `Rename-Item`. This command enables users to rename files or folders, making it an essential tool for file management. The general syntax follows this structure:
Rename-Item -Path "CurrentFilePath" -NewName "NewFileName"
Common Scenarios for Renaming File Extensions
Renaming file extensions can be beneficial in various scenarios, like changing formats to suit different applications. For example, you may want to convert `data.txt` to `data.csv` to ensure compatibility with a spreadsheet program. Additionally, you might need to work on multiple files at once, making a solid understanding of bulk processing integral.
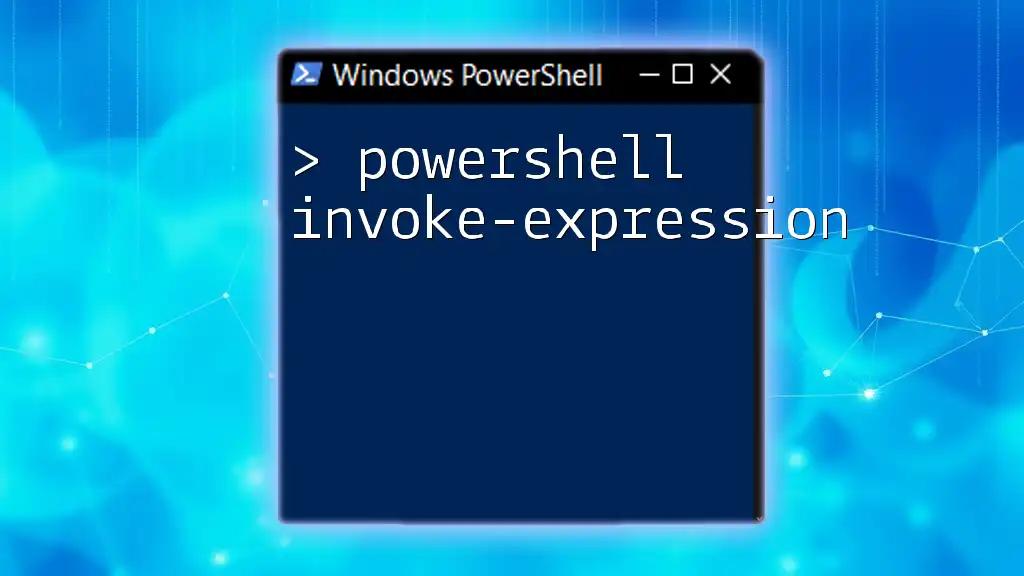
Renaming File Extensions: Step-by-Step Guide
Single File Renaming
Renaming a single file extension in PowerShell is straightforward. Here’s a practical example of changing the extension from `.txt` to `.md`:
Rename-Item -Path "C:\path\to\file.txt" -NewName "file.md"
In this command:
- The `-Path` parameter specifies the location of the file to be renamed.
- The `-NewName` parameter indicates the new file name, including the desired extension.
Bulk Renaming of File Extensions
Using PowerShell's `Get-ChildItem` and `ForEach-Object`
For bulk renaming, combining `Get-ChildItem` with `ForEach-Object` is powerful. This method allows you to apply changes to multiple files simultaneously. For example, to change all `.txt` files in a directory to `.md`, you would execute:
Get-ChildItem -Path "C:\path\to\directory\*.txt" | ForEach-Object {
Rename-Item -Path $_.FullName -NewName "$($_.BaseName).md"
}
In this snippet:
- `Get-ChildItem` retrieves every `.txt` file in the specified directory.
- The `ForEach-Object` cmdlet processes each file, renaming it while preserving its base name.
Using Regular Expressions for Advanced Renaming
For advanced renaming needs, regular expressions (regex) can be employed. This is particularly useful for conditional renaming based on specific patterns. Here’s an example of renaming files that match a particular pattern:
Get-ChildItem -Path "C:\path\to\directory\*.txt" | ForEach-Object {
if ($_.Name -match "^(.*)\.txt$") {
Rename-Item -Path $_.FullName -NewName "$($matches[1]).md"
}
}
In this example:
- The regex checks if the filename ends in `.txt`.
- If it matches, the file is renamed to the same base name but with a new extension.
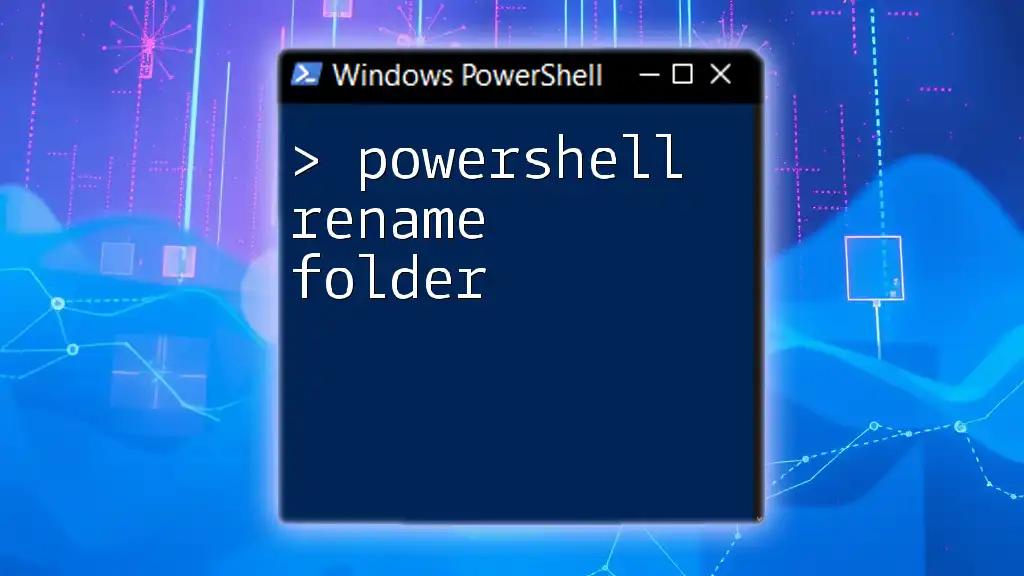
Error Handling in File Renaming
Common Errors and Solutions
When renaming files, various errors may arise, including permission issues and missing files. Using `Try` and `Catch` blocks can effectively manage these errors:
Try {
Rename-Item -Path "C:\path\to\file.txt" -NewName "file.md" -ErrorAction Stop
} Catch {
Write-Host "Error occurred: $_"
}
This construct ensures that if an error occurs, a user-friendly message is displayed instead of halting the entire script.
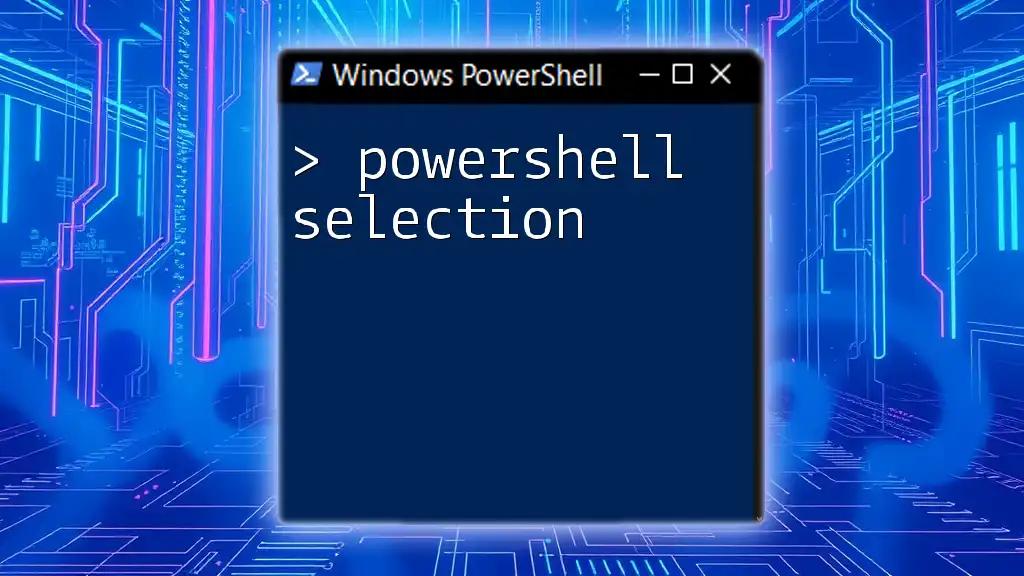
Tips and Best Practices
Testing Before Committing Changes
Before executing a rename command, testing your command is essential to avoid unwanted changes. Incorporating the `-WhatIf` parameter provides a simulation without making actual changes:
Rename-Item -Path "C:\path\to\file.txt" -NewName "file.md" -WhatIf
This safeguard allows you to see what would happen without affecting your files.
Backup Important Files
Always backup essential files before performing batch renaming or significant changes. Creating a copy of important data ensures that you can recover it in case something goes wrong.
Maintain Organization and Documentation
Keeping track of renamed files is vital, especially for large projects. Maintain logs to document changes, providing a reference for future actions or audits. Simple text files or spreadsheets can serve this purpose effectively.
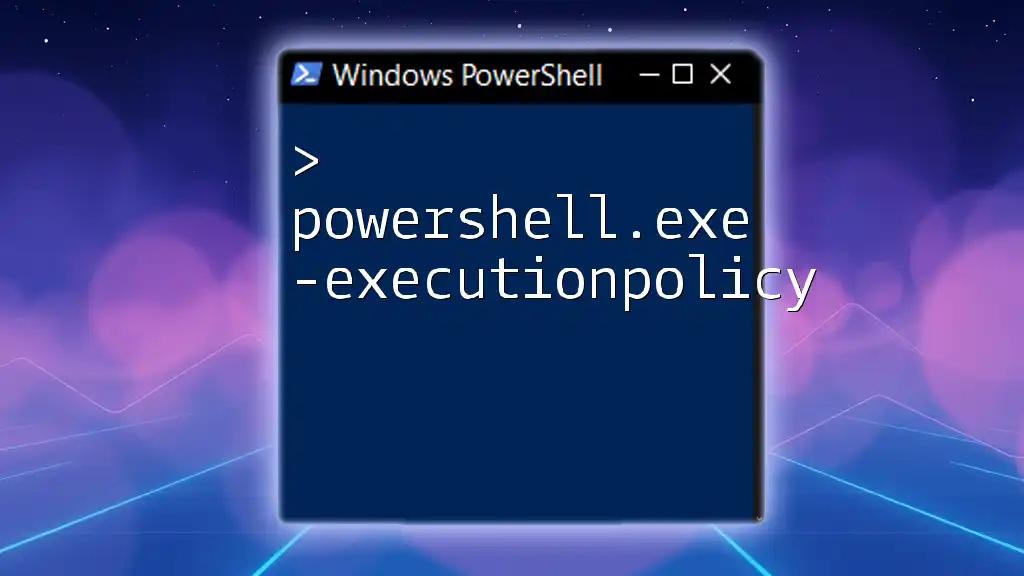
Conclusion
Understanding how to powerfully rename file extensions using PowerShell is invaluable for managing files efficiently. Whether working on a single file or processing multiple files in bulk, the flexibility and strength of PowerShell commands can enhance your workflow.
As you grow in your PowerShell journey, practice the examples provided here and explore even more commands and capabilities. With the right knowledge and tools, you can master PowerShell file management and elevate your scripting skills.
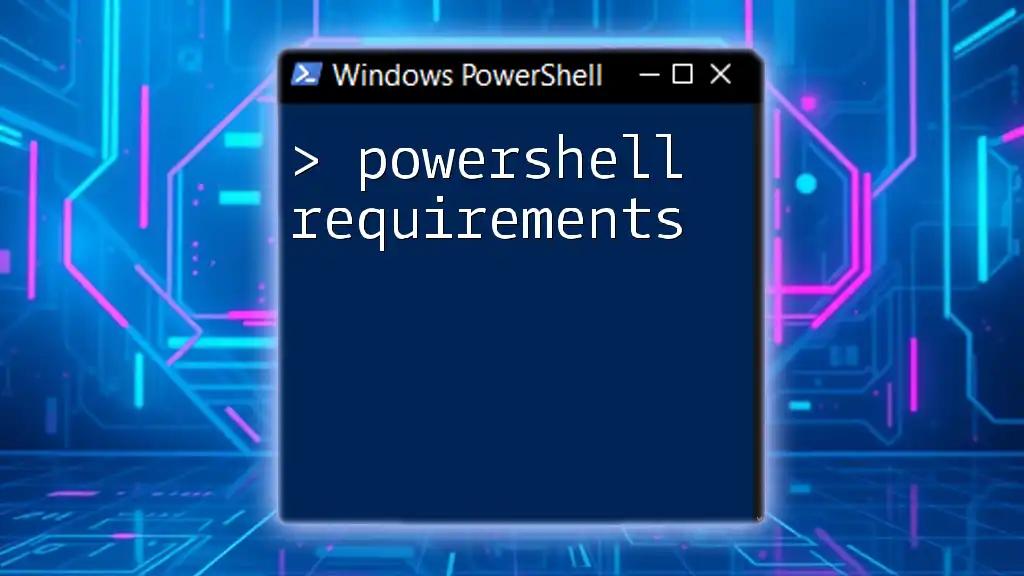
Additional Resources
Links to PowerShell Documentation
To deepen your understanding and enhance your skills, check the official [Microsoft PowerShell documentation](https://docs.microsoft.com/powershell/scripting/overview?view=powershell-7.2) and engage with community forums for diverse insights and solutions.
Invitation for Feedback and Questions
We encourage you to share your experiences and insights! Feel free to comment with any questions or tips you've discovered in your own PowerShell renaming adventures. Your feedback enriches this community and fosters growth for us all.