To create a file with specified content in PowerShell, you can use the `Set-Content` cmdlet followed by the file path and the desired text. Here's how you can do it:
Set-Content -Path "C:\Path\To\Your\File.txt" -Value "Hello, World!"
PowerShell Basics
Getting Started with PowerShell
Before diving into how to create files with content using PowerShell, it's essential to familiarize yourself with the PowerShell environment. You can open PowerShell by searching for it in your Start menu. Once it's launched, you can begin entering commands directly into the interface.
Syntax Overview
Understanding the syntax used in PowerShell is crucial for effective command execution. PowerShell commands are known as cmdlets, which follow a specific format: `Verb-Noun`, such as `Get-Item` or `New-Item`. This structure helps you easily identify the action performed (verb) and the item being acted upon (noun). Additionally, cmdlets can take parameters and arguments to refine their functionality, providing a flexible way to tailor commands to your needs.

Creating a File using PowerShell
Using `New-Item` Cmdlet
One of the foundational cmdlets in PowerShell is `New-Item`. This cmdlet allows users to create a new item in a specified path. When you want to create a file specifically, you use the `-ItemType` parameter set to `File`.
Basic Syntax
To create a new file without any content, you would use the following command:
New-Item -Path "C:\example\myfile.txt" -ItemType "File"
This command creates an empty text file named myfile.txt in the specified directory. However, it's important to note that while `New-Item` is effective for file creation, it does not allow for content insertion during the creation process.
Using `Out-File` Cmdlet
To create a file while simultaneously adding content, the `Out-File` cmdlet is your go-to. This cmdlet takes output from a pipeline and writes it to a file, making it incredibly versatile for inserting content directly.
Basic Example
Here’s a simple example to create a file with content:
"This is some text content." | Out-File -FilePath "C:\example\myfile.txt"
In this command, the string "This is some text content." is piped directly to `Out-File`, resulting in the creation of myfile.txt with the specified content.
Appending vs. Overwriting
PowerShell's `Out-File` cmdlet behaves in a specific manner regarding file content:
-
Append Content
By default, using `Out-File` will overwrite any existing file with the same name. If you want to append content instead, you can use the `-Append` parameter. Here’s how:"This is additional content." | Out-File -FilePath "C:\example\myfile.txt" -Append
This command adds "This is additional content." to the end of myfile.txt without erasing the previous content.
-
Overwriting Content
Since overwriting is the default behavior, simply omitting the `-Append` parameter will result in refreshing the file with the new input.
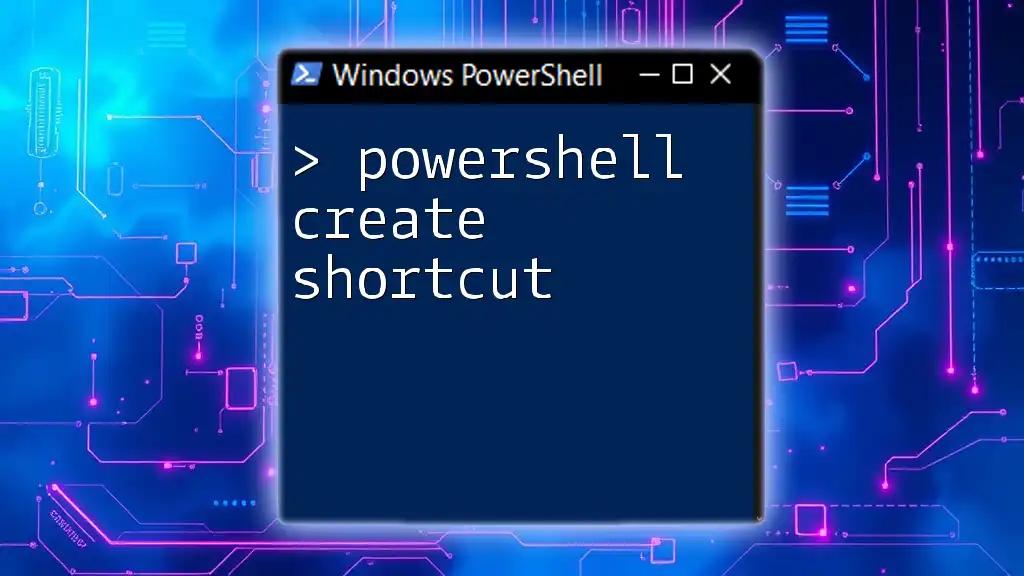
Creating Files with Multi-Line Content
Using Here-Strings
When you need to create files containing multiple lines of text, Here-Strings come in handy. A Here-String is a way to define a string that spans multiple lines conveniently.
Basic Example
Here’s how to create a file with multi-line content using a Here-String:
@"
This is line one.
This is line two.
This is line three.
"@ | Out-File -FilePath "C:\example\mymultilinefile.txt"
The syntax allows you to include line breaks easily, creating a file that consists of the specified lines of text when executed.
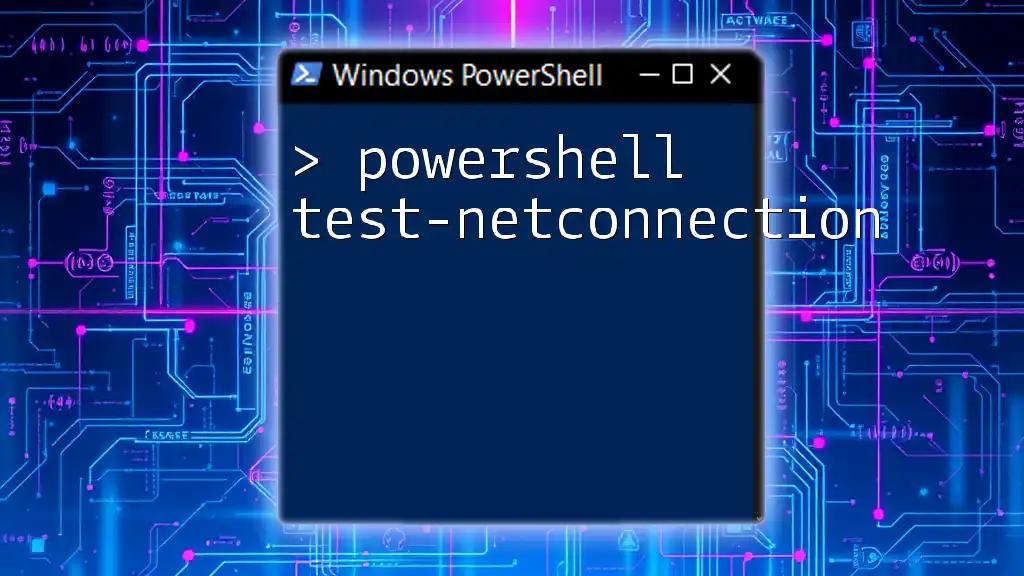
Creating Files from Existing Content
Copying Content from One File to Another
Sometimes, you may want to create a new file that contains the same content as an existing file. PowerShell makes it easy with the `Get-Content` and `Set-Content` cmdlets.
Basic Example
Here’s how to accomplish this task effectively:
Get-Content -Path "C:\example\sourcefile.txt" | Set-Content -Path "C:\example\destinationfile.txt"
In this command, `Get-Content` retrieves the content from sourcefile.txt and pipes it directly to `Set-Content`, which creates destinationfile.txt with the same content.
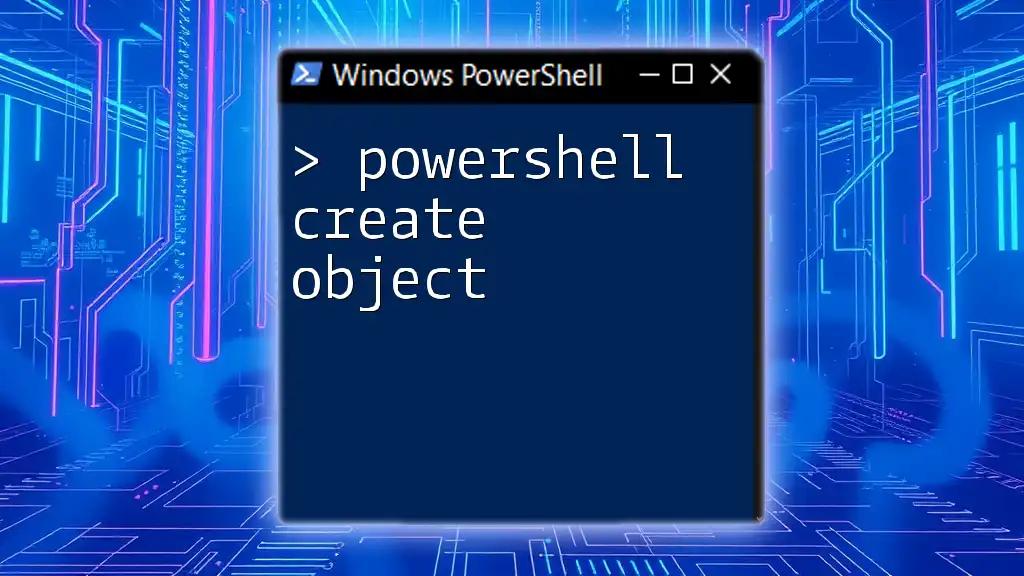
Common Use Cases
Batch File Creation
You may find yourself frequently needing to create multiple files at once. PowerShell allows you to automate this process with loops.
Example
Using a straightforward loop command can help create multiple files seamlessly:
1..5 | ForEach-Object { "This is file $_" | Out-File -FilePath "C:\example\file$_.txt" }
In this case, the loop generates five separate files named file1.txt through file5.txt, each containing text that corresponds to its file number.
Logging and Error Handling
When creating files, especially in scripts, it’s prudent to implement logging strategies and error handling. This can include directing errors to a log file or displaying messages indicating the success or failure of file operations.

PowerShell Best Practices
File Naming Conventions
Adhering to consistent file naming conventions is vital for organization and easy retrieval later on. It’s advisable to use descriptive names that clearly indicate file content or purpose.
Secure File Management
When creating files, consider their security permissions. Ensure that only authorized users have access to sensitive files, utilizing PowerShell to set permissions as part of your workflow.
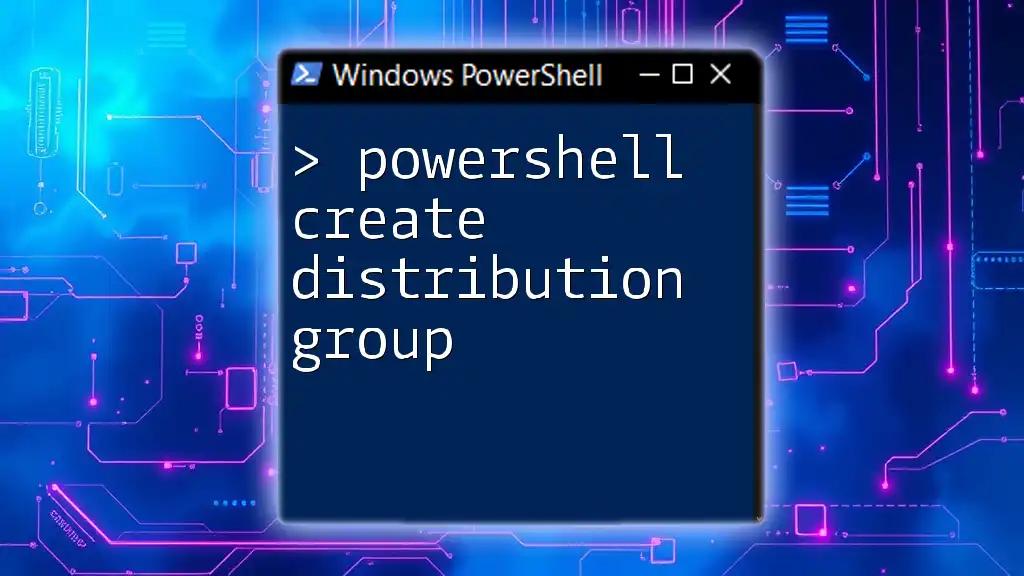
Troubleshooting
Common Errors
Errors can occur when creating files, such as permissions issues or incorrect paths. Always double-check the specified path and ensure you have the necessary write permissions for that location.
File Location Problems
If a file is not found after creation, it's a sign to verify the path being used. Always use absolute paths to avoid confusion related to the current working directory.

Conclusion
In this article, we covered how to create files with content in PowerShell utilizing various cmdlets like `New-Item` and `Out-File`. From basic file creation to incorporating multi-line content and handling existing files, these techniques equip you with essential skills for file management within PowerShell. Regular practice of these commands will not only enhance your proficiency but also streamline your workflow, making file operations more efficient.

Call to Action
We invite you to explore further with PowerShell and enhance your skills by trying out the commands mentioned above. For more resources and detailed guides, consider subscribing to our mailing list or following our organization. Join our community, and feel free to share your experiences and questions with us!