To read a file into a variable in PowerShell, you can use the `Get-Content` cmdlet, which retrieves the contents of a file and stores it as an array of strings.
$fileContents = Get-Content -Path "C:\Path\To\Your\File.txt"
Understanding PowerShell Variables
What is a Variable in PowerShell?
A variable in PowerShell is a named storage location that can hold data. This data can be anything from a simple string to complex objects. Variables are essential as they allow you to store and manipulate data throughout your PowerShell scripts or sessions. By using variables, you can make your scripts dynamic and reusable.
Data Types in PowerShell
PowerShell supports various data types for its variables. The most common types include:
-
String: A sequence of characters. For example:
$greeting = "Hello, World!"
-
Array: A collection of items. You can create an array like so:
$numbers = 1, 2, 3, 4, 5
-
Hash Table: A collection of key-value pairs, useful for structured data. For example:
$person = @{ Name = "John"; Age = 30 }
Each data type allows you to manipulate and interact with your data in different ways.
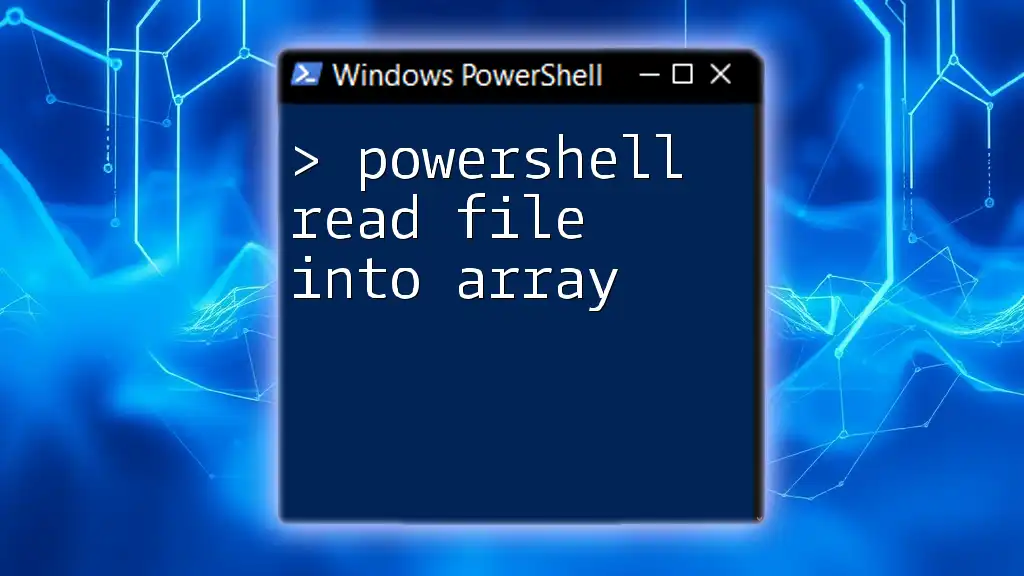
Reading Files in PowerShell
File Types and Formats
PowerShell is capable of reading various file types, including plain text files (TXT), comma-separated values (CSV), JSON, and XML files. Understanding the type of data you are working with is crucial for choosing the appropriate cmdlet for reading it.
The Get-Content Cmdlet
The Get-Content cmdlet is a powerful tool for reading the contents of a file.
Basic Syntax
The basic syntax for using Get-Content is as follows:
Get-Content <path-to-file>
Example
To read a text file into a variable, you would use:
$filePath = "C:\path\to\your\file.txt"
$fileContent = Get-Content $filePath
Here, the contents of the specified text file are stored in the variable $fileContent. This variable will now contain an array where each line of the text file is an individual element.
Reading a File into a Variable
Example with a Text File
Let's break down how to read a text file into a variable properly:
$filePath = "C:\path\to\your\file.txt"
$fileContent = Get-Content $filePath
Write-Output $fileContent
In the above example:
- $filePath is defined to hold the location of your file.
- The call to `Get-Content` reads the file and assigns its contents to $fileContent.
- The `Write-Output` command displays the contents of the variable.
Example with a CSV File
For CSV files, you would typically use the Import-Csv cmdlet, which reads the structured data and converts it into PowerShell objects. For example:
$csvPath = "C:\path\to\your\data.csv"
$csvData = Import-Csv $csvPath
This command results in $csvData being an array of objects, where each object corresponds to a row from the CSV file, and properties match the column names.
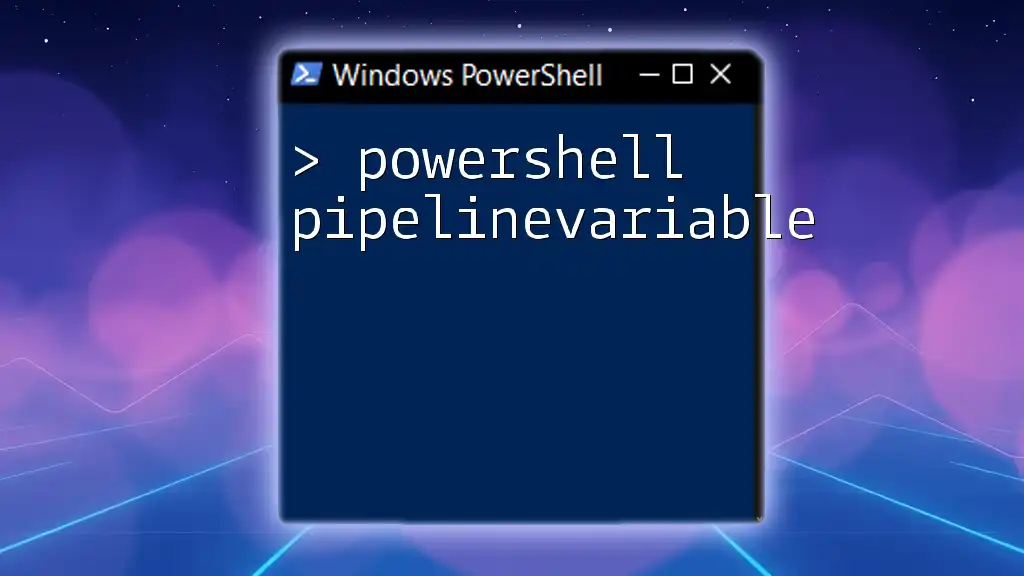
Accessing and Manipulating Data
Accessing Data in Variables
Once you've successfully read your data into a variable, you can access individual elements. For example:
$firstLine = $fileContent[0]
Write-Output $firstLine
In this code snippet, $firstLine will be assigned the first line of the text file, which can then be processed or displayed.
Common Data Manipulations
You can perform various manipulations on your data by leveraging additional cmdlets. For instance, to filter data from a CSV file, you can use Where-Object:
$filteredData = $csvData | Where-Object { $_.ColumnName -eq 'Value' }
Here, the $_ represents the current object in the pipeline, and this example filters rows where a specific column matches a given value.
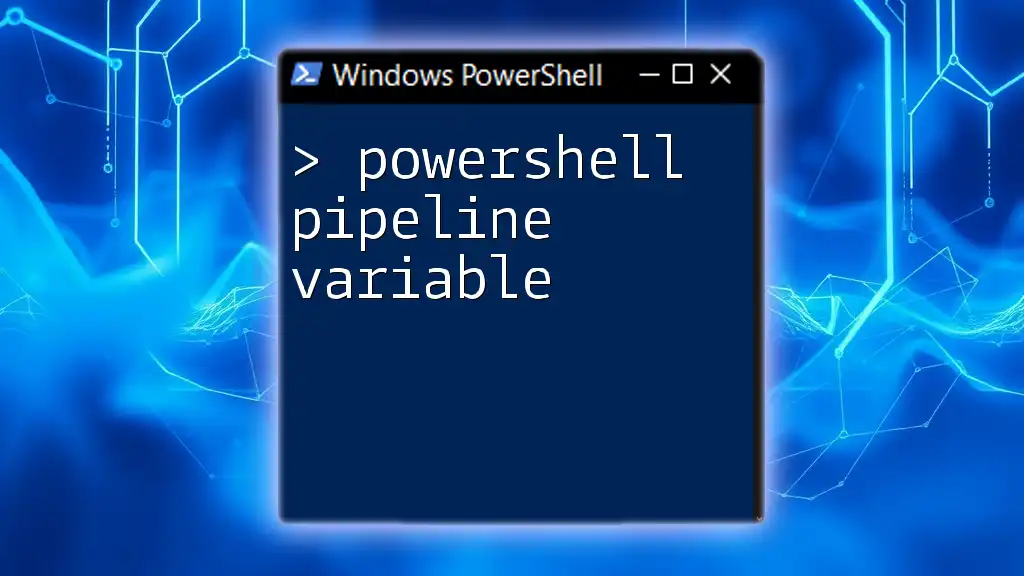
Handling Errors and Exceptions
Common Errors When Reading Files
When dealing with file input, common errors may arise, such as:
- File not found (typo in path)
- Unsupported file format
- Access denied errors
Using Try-Catch for Error Handling
To handle errors gracefully, you can use a try-catch block. This allows your script to continue running without crashing if an error occurs:
try {
$fileContent = Get-Content "C:\path\to\your\file.txt"
} catch {
Write-Error "File could not be read: $_"
}
In this example, if the file cannot be accessed, the catch block will output a clear error message instead of terminating the script unexpectedly.
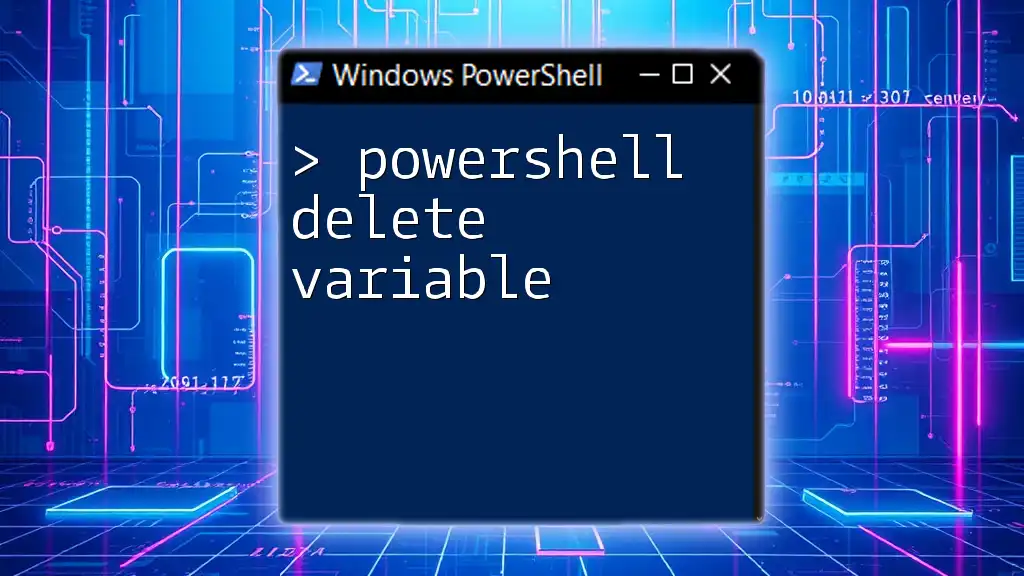
Advanced Techniques
Reading Large Files Efficiently
When working with large files, reading all the data at once may not be practical. You can use the -ReadCount parameter with Get-Content to manage memory better:
$largeFileContent = Get-Content "C:\path\to\largefile.txt" -ReadCount 1000
This method batches the file read operation, thus minimizing memory usage and improving performance.
Working With JSON Files
PowerShell also allows you to read JSON data easily. You can combine Get-Content with ConvertFrom-Json to achieve this:
$jsonPath = "C:\path\to\your\data.json"
$jsonContent = Get-Content $jsonPath | ConvertFrom-Json
Now, $jsonContent will be an object that allows for easy access to the properties defined in your JSON structure.
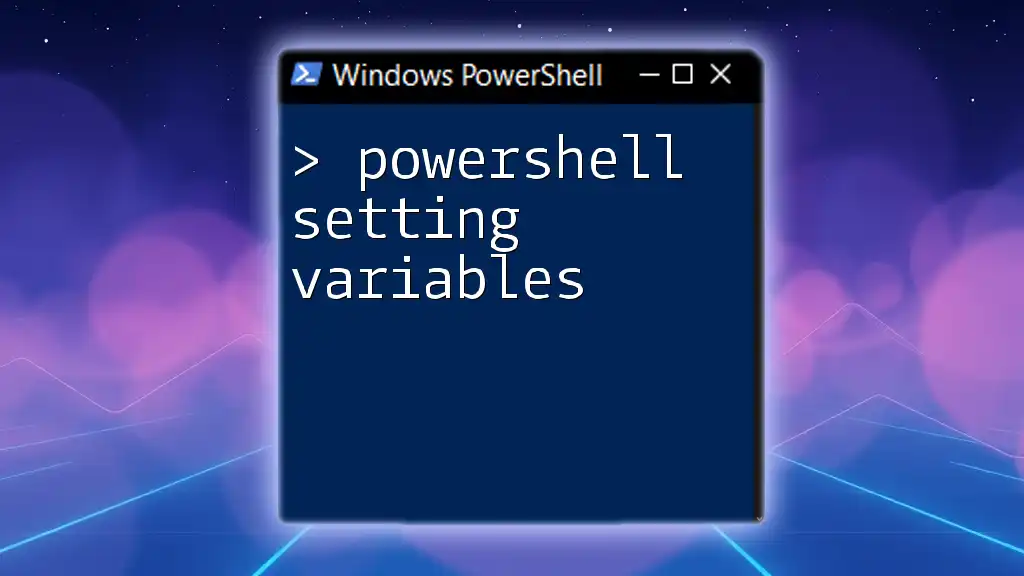
Conclusion
Mastering how to read files into variables using PowerShell is essential for data automation and management. Understanding the different file types and cmdlets available allows you to handle data effectively. By following these practices, you'll become more adept at writing scripts that are not only functional but also efficient.
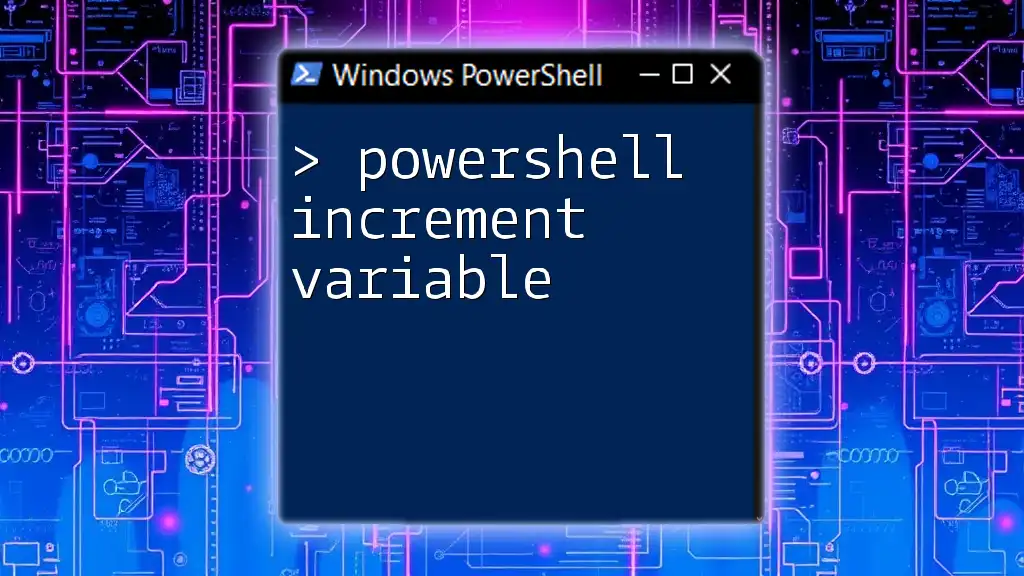
Additional Resources
For more information, you can delve into the official PowerShell documentation or explore books and online courses focused on advanced PowerShell scripting techniques. By continually learning, you can improve your PowerShell skills and knowledge, making your scripts more powerful and versatile.