To clear the error variable in PowerShell, you can use the following command to reset the `$error` automatic variable, which stores the error messages from the current session.
Clear-Variable -Name error -Scope Global
Understanding the `$error` Variable
Definition of the `$error` Variable
In PowerShell, the `$error` variable is an automatic array that stores error information generated during script execution. It allows users to capture and inspect the details of errors that occur, enabling effective troubleshooting. The structure of the `$error` variable consists of a collection of error objects, each containing various properties that provide insight into the nature of the error, such as the error message and the invocation of the command that caused the error.
To see its contents, you can simply execute:
$error
Common Scenarios for Errors in PowerShell
Errors can arise from numerous situations while working with PowerShell. Common sources include syntax errors, which occur when the script is not written correctly, and runtime errors, which happen during script execution due to unexpected conditions.
For example, if you try to access a file that does not exist, PowerShell will generate an error and populate the `$error` variable with relevant information. To generate a sample error intentionally, you could run the following command:
Get-Item "C:\NonExistentFile.txt"
After executing this command, check the `$error` variable to see the details of the error generated.
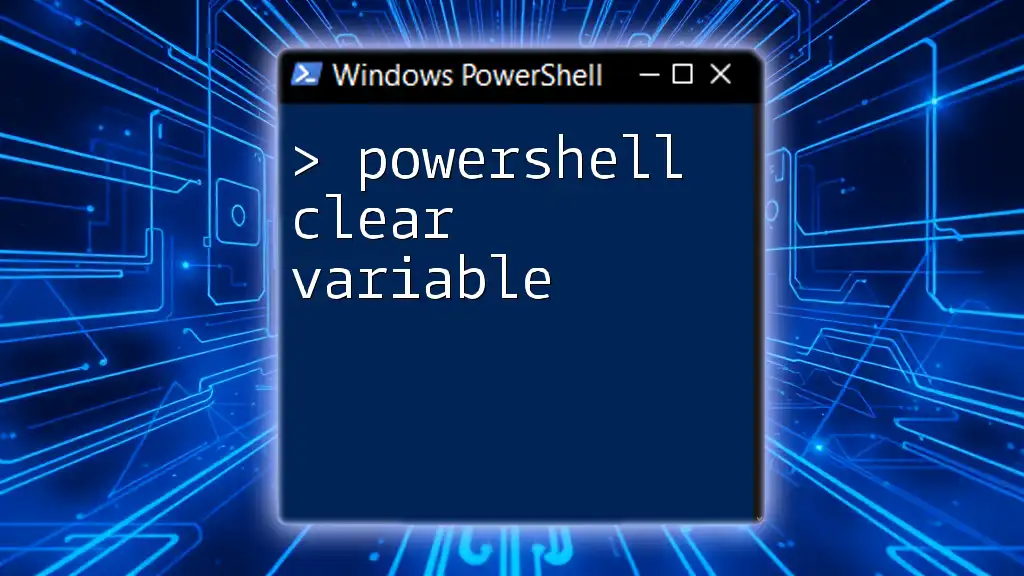
Why Clear the `$error` Variable?
Importance of Clearing Errors
Maintaining a cluttered `$error` variable can significantly hinder your troubleshooting efforts. It makes it challenging to isolate new problems that may arise in your script. By clearing the `$error` variable, you allow yourself space to work with fresh error information, ensuring that you are focused on the current state of affairs.
For instance, after handling an error, clearing the `$error` variable provides a clean slate, preventing confusion over legacy errors that are no longer relevant. Consider this scenario: before clearing:
$error
After clearing, you should see an empty array:
$error
When to Consider Clearing the `$error` Variable
You should consider clearing the `$error` variable at strategic points in your scripting journey. Key moments include:
- When starting a new task: Prioritizing a fresh start to avoid previous errors affecting your current execution.
- After handling or logging exceptions: Once you’ve logged necessary information for debugging, clearing `$error` prevents unnecessarily cluttering your workspace with outdated error details.
As an illustration, if your script experiences a failure and you log the error, immediately after handling this, execute `Clear-Error` to improve your workflow for subsequent operations.
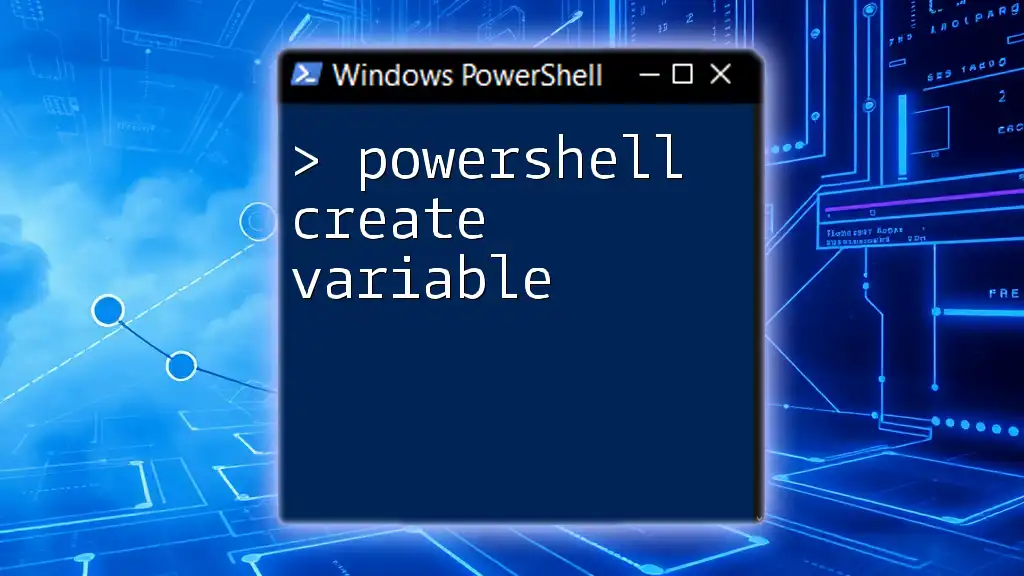
How to Clear the `$error` Variable
The Basic Command to Clear `$error`
Clearing the `$error` variable is straightforward. PowerShell provides a built-in command specifically designed for this task. Simply execute:
Clear-Error
Practical Example of Clearing `$error`
To demonstrate the process of error creation and clearing, consider this example. First, intentionally generate an error by attempting to fetch a non-existing file:
Get-Item "C:\NonExistentFile.txt"
Next, inspect the `$error` variable to view the generated error details:
$error
Now, clear the error using the command:
Clear-Error
Finally, verify that the `$error` variable has been cleared by checking its contents once more:
$error
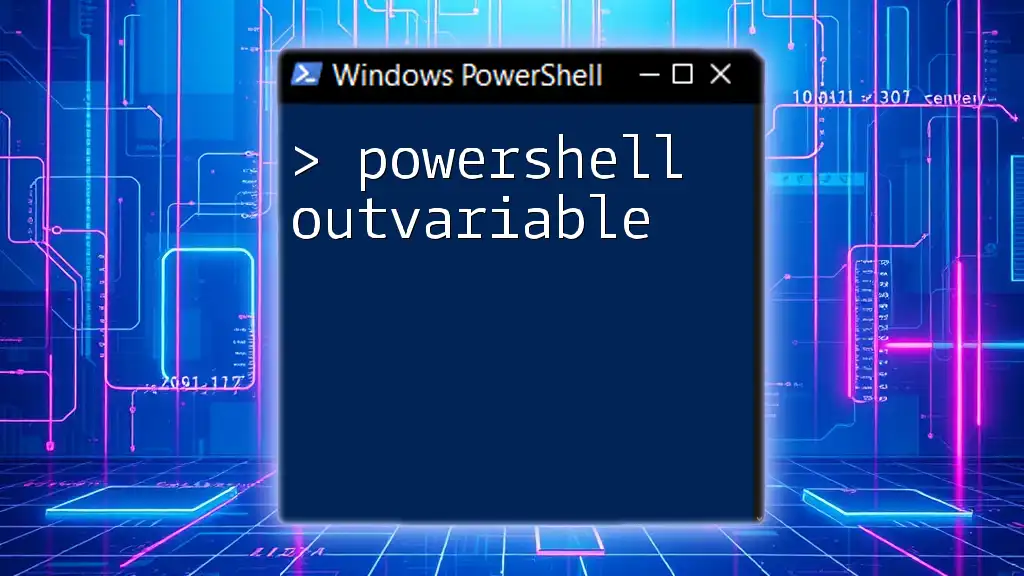
Troubleshooting Common Issues
What if Clearing `$error` Doesn’t Seem to Work?
While `Clear-Error` should clear the error information, occasionally, you may encounter situations where it appears to not function as expected. Common challenges include executing `Clear-Error` before any errors are generated or using nested scopes where `$error` can behave unexpectedly.
To troubleshoot this, ensure you call `Clear-Error` after you have addressed any errors that have occurred during your script execution. Always check the command's placement within your script to avoid mishaps.
Monitoring the State of the `$error` Variable
An effective way to manage your `$error` variable status is by incorporating checks within your script. You can examine the count and content of the variable to determine if errors still exist:
if ($error.Count -gt 0) {
Write-Host "Errors still exist"
} else {
Write-Host "No errors found"
}
This simple check enables you to tailor your error handling according to the current state of your script.
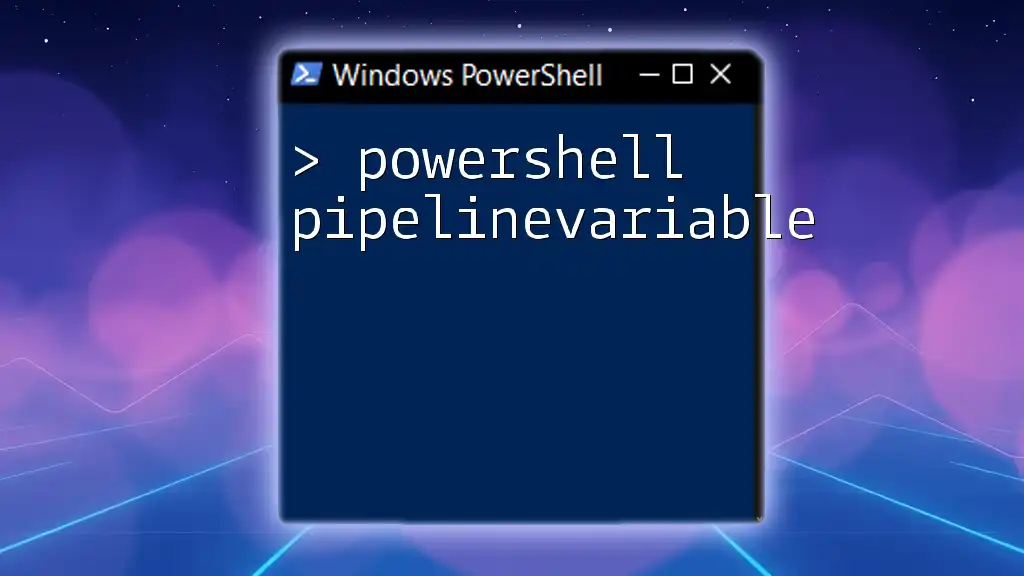
Best Practices for Managing the `$error` Variable
Implementing Error Handling Strategies
A robust error handling mechanism is essential for productive scripting. Embrace the use of `try/catch` blocks to capture and manage exceptions effectively. This structured approach allows you to define specific actions when errors are caught and ensures that your error variable is maintained cleanly. Here’s an illustrative example:
try {
Get-Item "C:\NonExistentFile.txt"
} catch {
Write-Host "An error occurred: $_"
} finally {
Clear-Error
}
In this snippet, an error caused by trying to fetch a non-existent file is verbally acknowledged, and the `$error` variable is cleared afterward, leaving no trace of the past error.
Regular Maintenance of Script Errors
As a best practice, consider adopting a routine for checking and clearing the `$error` variable in long-running scripts. This approach not only enhances the clarity of error handling but also ensures that new errors are easily identifiable and manageable.
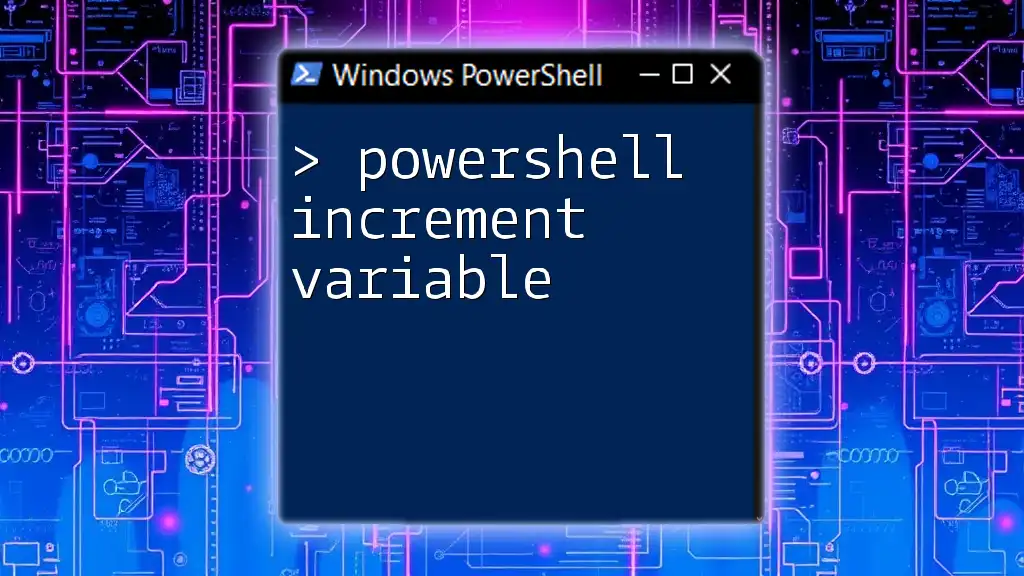
Conclusion
Effectively managing the `$error` variable in PowerShell is a critical aspect of script development and execution. By understanding how to clear the `$error` variable, monitoring its state, and employing structured error handling strategies, you can enhance your scripting efficiency and troubleshoot issues more effectively. Practice incorporating these techniques in your scripts, and you will find that maintaining a clear and manageable error handling process significantly improves your PowerShell experience.
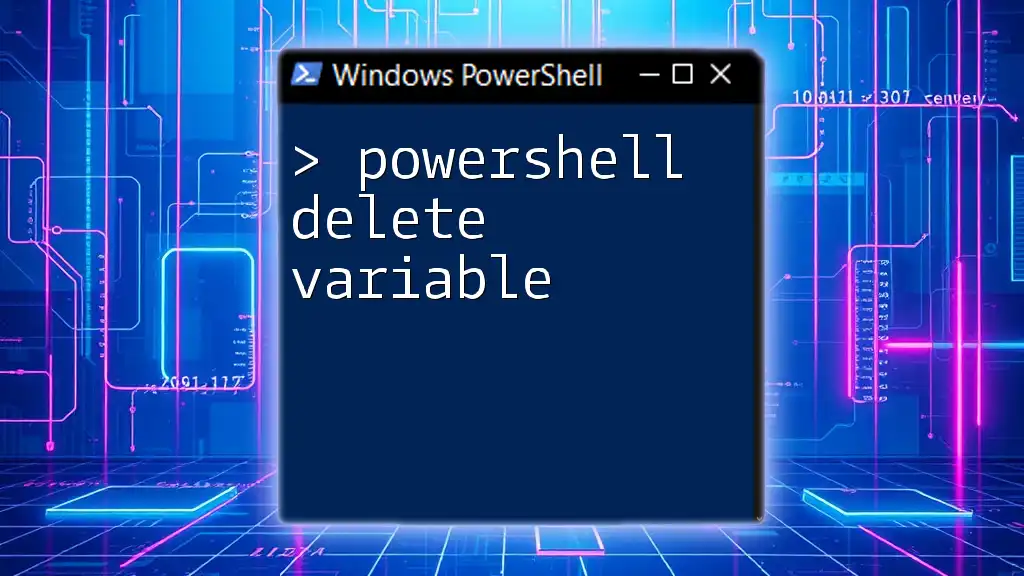
Additional Resources
For further reading, consider exploring the official PowerShell documentation on error handling, as well as other materials aimed at mastering PowerShell scripting techniques.