In PowerShell, the `Write-Host` cmdlet is used to display output directly to the console, allowing you to show the value of a variable by concatenating it within a string. Here's an example:
$greeting = 'Hello, World!'
Write-Host $greeting
Understanding Write-Host
What is Write-Host?
The `Write-Host` cmdlet is an essential part of PowerShell, primarily used to display output directly to the console. Unlike other output cmdlets such as `Write-Output`, which send data to the output stream that can be piped or redirected, `Write-Host` is primarily intended for user-friendly console messages. It is ideal for providing immediate feedback during script execution or when debugging.
Why Use Write-Host?
Using `Write-Host` allows script developers to deliver output that is directly visible to users. It can be great for debugging or conveying important information during script execution. For instance, if you're running a long script, using `Write-Host` strategically can help show the progress or highlight specific results.
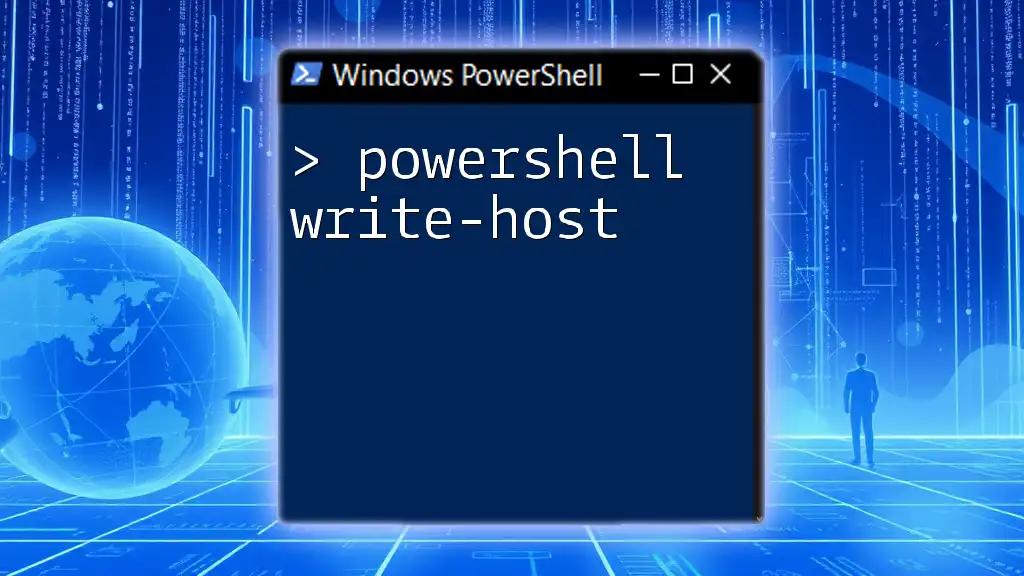
Variables in PowerShell
Defining Variables
In PowerShell, defining a variable is straightforward. You declare a variable by starting it with a `$` followed by its name. Here's an example of a simple variable assignment:
$greeting = "Hello, World!"
This line creates a variable `$greeting` and assigns it the value `"Hello, World!"`.
Variable Types
PowerShell supports various data types, including strings, integers, arrays, and custom objects. It's crucial to understand these variable types—particularly strings—when using `Write-Host`, as you might want different formatting or output styles depending on the type of data you're working with.
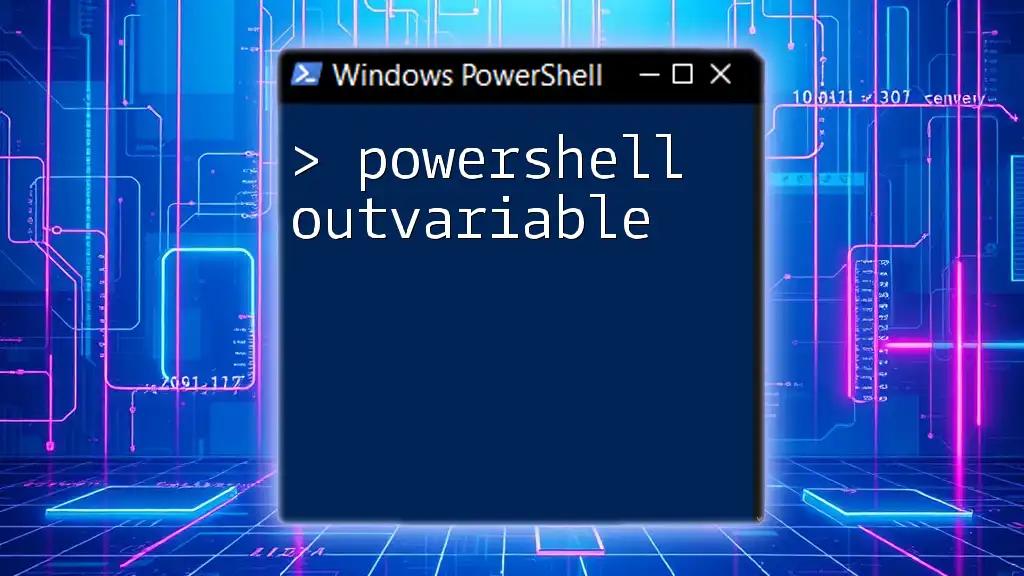
Using Write-Host with Variables
Basic Usage with Variables
To display variable values with `Write-Host`, you can insert variables directly into strings. For example:
$name = "Alice"
Write-Host "My name is $name"
In this snippet, `Write-Host` will output: My name is Alice. The variable `$name` is referenced within the string, showcasing how simple embedding works.
String Interpolation
PowerShell supports string interpolation, which means you can easily combine static text with variable values. This feature is beneficial for creating dynamic messages. For instance:
$age = 30
Write-Host "I am $age years old"
Here, the output will be: I am 30 years old. This capability allows developers to create more informative outputs without cumbersome concatenation.
Formatting Output
Changing Text Color and Background
One of the attractive features of `Write-Host` is its ability to change text colors and backgrounds, enhancing visibility for critical messages. By using parameters like `-ForegroundColor` and `-BackgroundColor`, you can make your messages stand out.
For example:
Write-Host "Error: Something went wrong" -ForegroundColor Red -BackgroundColor Black
This will display the message in red text on a black background, making it immediately obvious to the user.
Customizing Output
You can also customize the output using the `-Separator` parameter. This is particularly useful when displaying items from an array. Here’s how it works:
$fruits = "Apple", "Banana", "Cherry"
Write-Host $fruits -Separator ", "
In this case, the output will be: Apple, Banana, Cherry. The `-Separator` parameter allows you to dictate how the items are presented, adding flexibility to your outputs.
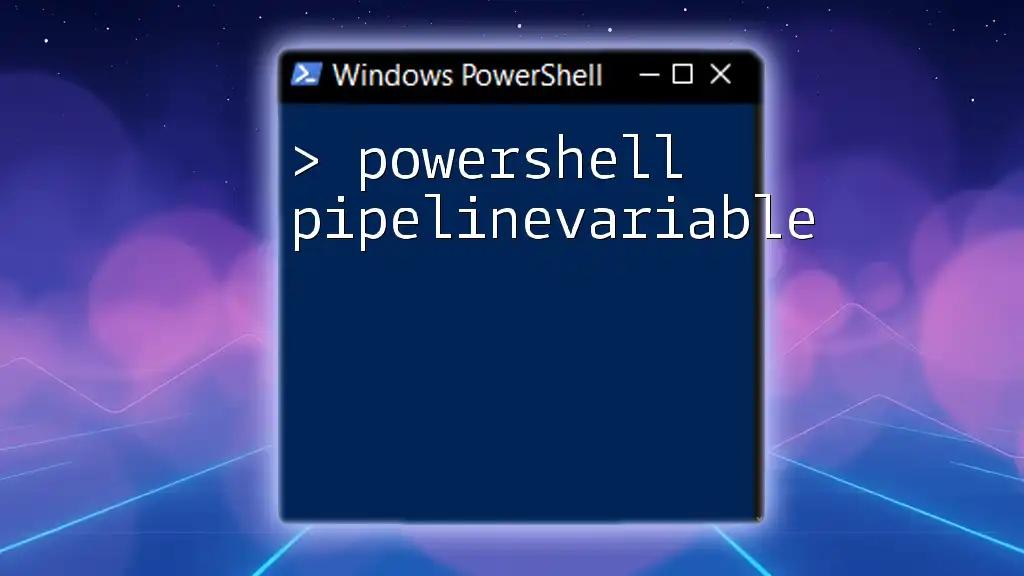
When to Avoid Using Write-Host
Situations Where Write-Host is Not Recommended
Although `Write-Host` is useful, it does have limitations. For instance, outputs generated by `Write-Host` cannot be captured, piped, or redirected. This makes it less suitable for logging and automation tasks where output needs to be processed further.
When creating scripts meant for automation, consider using `Write-Output` instead. This cmdlet allows the output to be sent through the pipeline, giving greater flexibility for further manipulation.
Performance Considerations
Using `Write-Host` excessively in large scripts can have performance implications. Since `Write-Host` outputs content directly to the screen during execution, it may slow down your script if it generates a lot of output. Hence, employing it judiciously is crucial, especially in production environments.
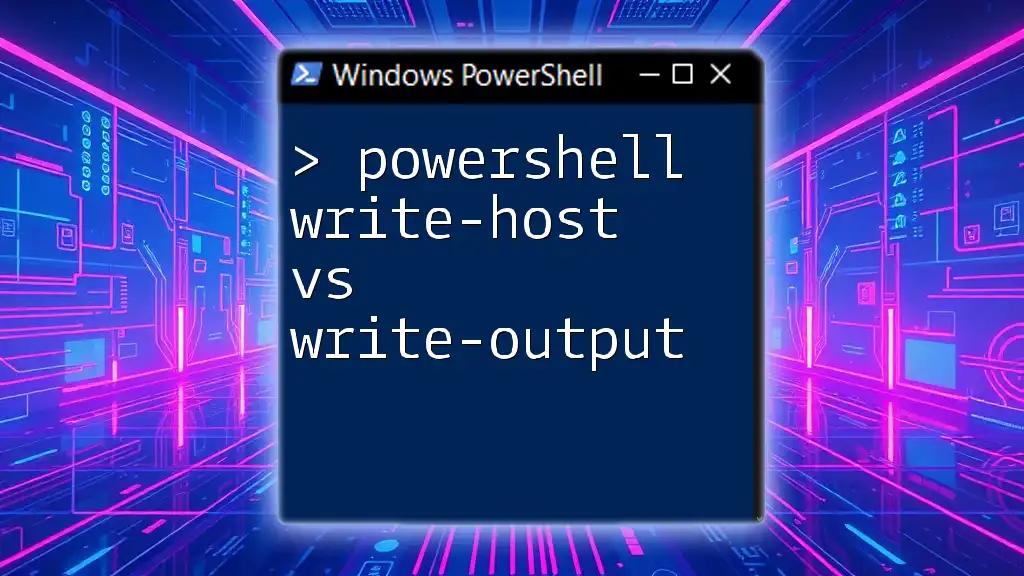
Real-World Examples
Example 1: Logging User Info
Consider a scenario where you want to log user information while ensuring the output is clear visually. Here’s how you might do it:
$username = "JohnDoe"
$status = "Active"
Write-Host "User: $username, Status: $status" -ForegroundColor Green
This example outputs: User: JohnDoe, Status: Active, in green text, indicating the information presented is currently relevant and positive.
Example 2: Creating a Menu Interface
You can also use `Write-Host` to create a console menu, making scripts interactive. A simple console menu can look like this:
Write-Host "1. Start" -ForegroundColor Cyan
Write-Host "2. Exit" -ForegroundColor Cyan
This code displays the options in a visually pleasing cyan color, inviting user input while maintaining simplicity.
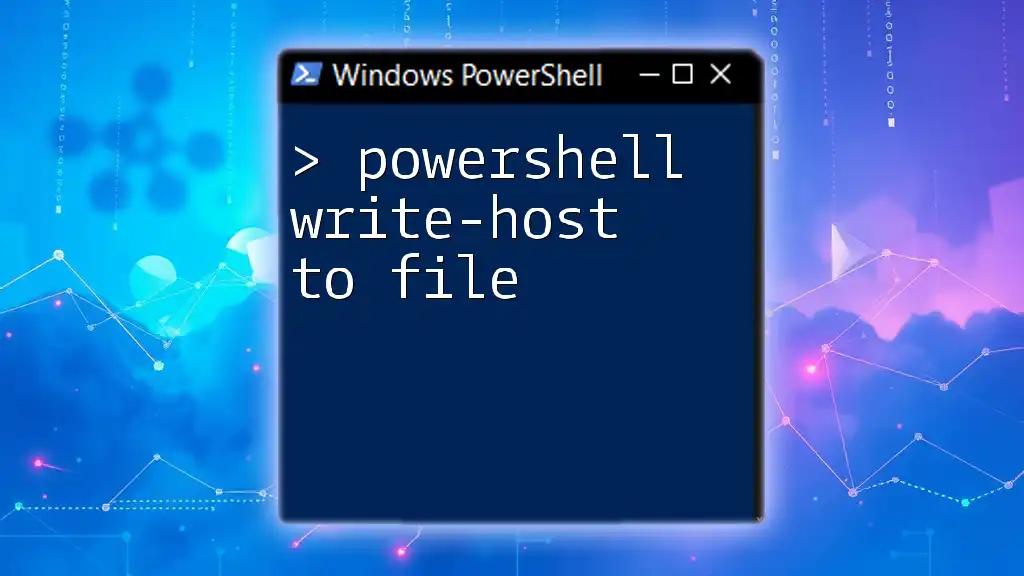
Conclusion
Throughout this guide, we’ve explored the significance of the PowerShell Write-Host variable and its versatile application in scripting. We discussed the differences between `Write-Host` and other output methods, the importance of understanding variables and their types, and the way to enhance output visibility through formatting options.
Using `Write-Host` correctly can elevate your PowerShell scripting, especially when displaying important console messages or debugging output. Explore these methods and incorporate them into your scripting practices to improve your PowerShell proficiency!

Additional Resources
For more information on PowerShell and its capabilities, check the official PowerShell documentation and community forums where you can engage with other PowerShell developers and enthusiasts.
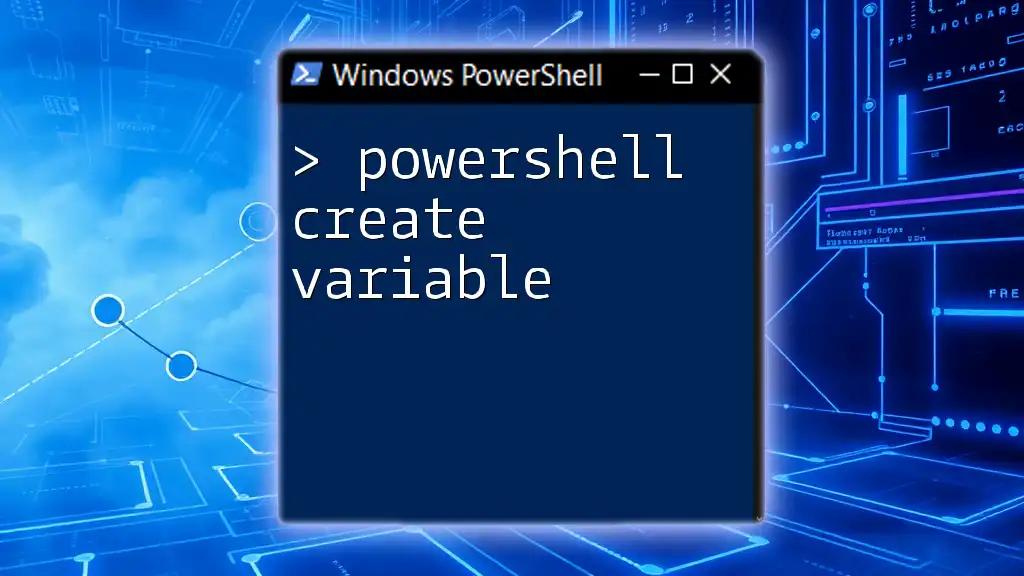
Call to Action
Have you had experience using `Write-Host`? Share your tips and lessons learned with us, or consider enrolling in our PowerShell training services for personalized learning designed to enhance your skills!