In PowerShell, you can display the value of a variable using the `Write-Host` or simply by typing the variable's name, as shown in the example below.
$myVariable = 'Hello, World!'
Write-Host $myVariable
Understanding Variables in PowerShell
What is a Variable?
A variable is a fundamental concept in programming that allows you to store data that can be referenced and manipulated within your scripts. In PowerShell, variables are used to store strings, numbers, arrays, objects, and more. This flexibility makes them essential for creating dynamic and efficient scripts.
How to Create a Variable in PowerShell
To create a variable in PowerShell, you need to use the `$` symbol followed by the variable name. Here’s a simple syntax example:
$myVariable = "Hello, World!"
In this example, `$myVariable` holds the string "Hello, World!". PowerShell variables are case-insensitive, and naming conventions generally suggest using meaningful names to make your scripts easier to read.
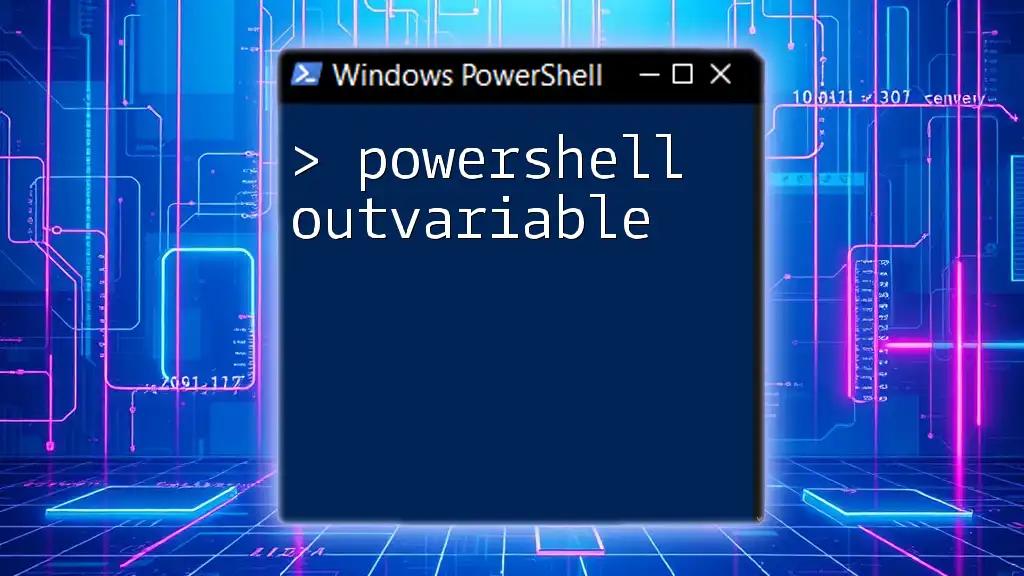
How to Show Variable Values in PowerShell
Using the Write-Host Cmdlet
One of the most straightforward ways to display the value of a variable is by using the `Write-Host` cmdlet. This cmdlet writes the output directly to the console. For instance, to show the value of `$myVariable`, you can write:
Write-Host "The value of myVariable is: $myVariable"
This line outputs: `The value of myVariable is: Hello, World!`. Write-Host is particularly useful for providing information to users in interactive scripts.
Using the Write-Output Cmdlet
Another cmdlet that can be used for displaying information is `Write-Output`. This cmdlet sends output to the pipeline, allowing further processing if desired. For example:
Write-Output $myVariable
This will produce the same output as before, displaying `Hello, World!`. The difference is that `Write-Output` is more flexible, as it can be piped into other cmdlets for additional processing.
Directly Displaying Variable Values
You can also display the value of a variable simply by typing the variable name on its own. This method is quick and effective, as shown below:
$myVariable # Displays the value directly
When executed, PowerShell outputs `Hello, World!`. This method is convenient for quick checks, especially when working in an interactive session.

Advanced Techniques for Showing Variable Values
Formatting Output with Format-Table
For more organized output, especially when dealing with collections or arrays, you can use the `Format-Table` cmdlet. This cmdlet formats your data into a neatly organized table. Here’s an example:
$myArray = @("Item1", "Item2", "Item3")
$myArray | Format-Table
The output will display `Item1`, `Item2`, and `Item3` in a tidy table format, making it easier to read at a glance.
Using ConvertTo-Json for JSON Output
If you want to convert your variables to JSON format, PowerShell provides the `ConvertTo-Json` cmdlet. This is especially handy when you need to export data or work with APIs. Here’s how you can use it:
$myObject = New-Object PSObject -Property @{ Name = "Item1"; Value = 100 }
$myObject | ConvertTo-Json
This command converts the `$myObject` variable into a JSON representation, allowing you to easily share or integrate data with web services.
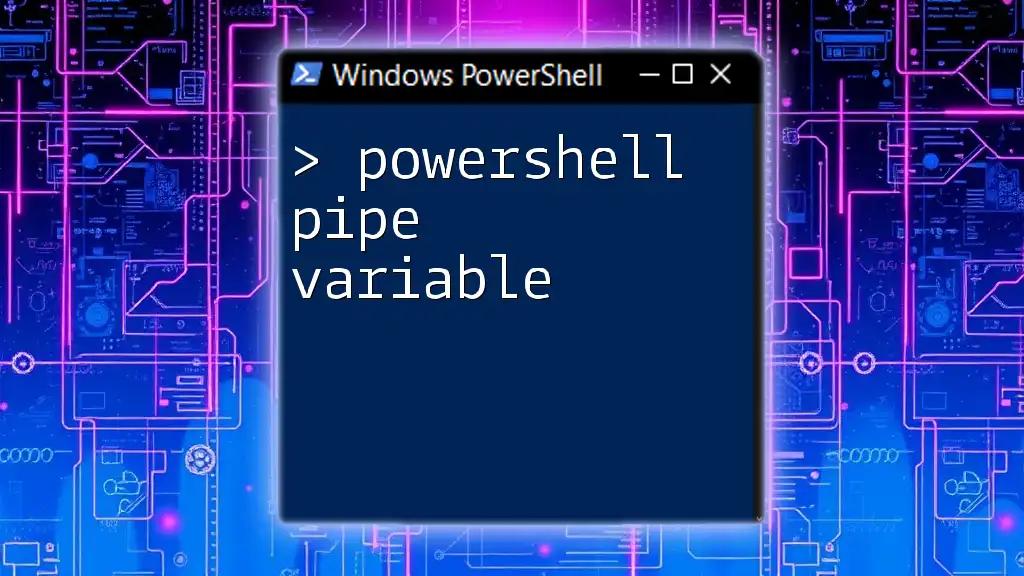
Debugging with Variable Values
Using Write-Debug to Show Variable Values
The `Write-Debug` cmdlet is a powerful tool in your scripting arsenal, particularly for debugging. It helps to inspect variable values without impacting the regular output of your script. To use it, include this line in your code:
Write-Debug "The current value of myVariable is: $myVariable"
When running your script, enabling debugging will show the output if debugging is activated. You can enable debugging by using the `-Debug` switch when executing your script.
Using Breakpoints and the PowerShell ISE
If you use the PowerShell Integrated Scripting Environment (ISE), you can set breakpoints that allow you to pause execution and inspect variables interactively. To set a breakpoint, click on the left margin next to the line number in your script. When execution pauses, you can hover over any variable to see its current value or use the console to query its value directly.
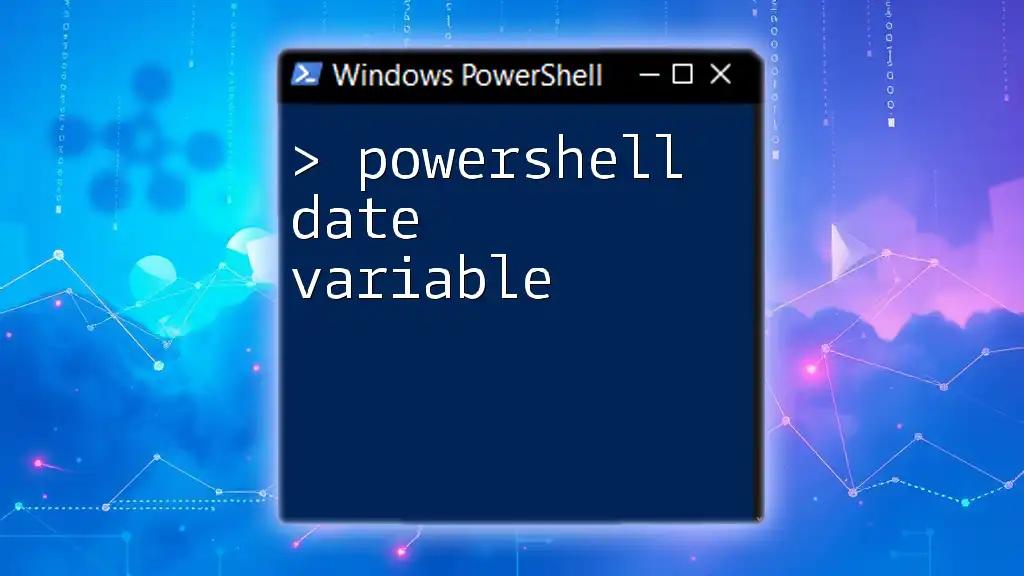
Best Practices for Showing Variable Values
Consistency in Naming Conventions
Maintaining a consistent naming convention for your variables is critical. This not only enhances code readability but also helps in understanding the purpose of each variable at a glance. Use meaningful names that reflect the data they hold. For example, instead of using `$x`, use `$userResponse`.
Documenting Your Scripts
Documentation is key to maintainable scripts. Commenting is a simple yet effective way of explaining the purpose of each variable. Consider the following:
# Variable to hold user's name
$userName = "John Doe"
Including such comments helps you and others understand your code in the future.
Performance Considerations
When displaying large amounts of data, be mindful of performance. Write-Host is less performance-friendly with extensive outputs since it writes directly to the console each time. For larger datasets, consider grouping data for summarized output or use `Out-GridView`, which allows for easier data navigation.
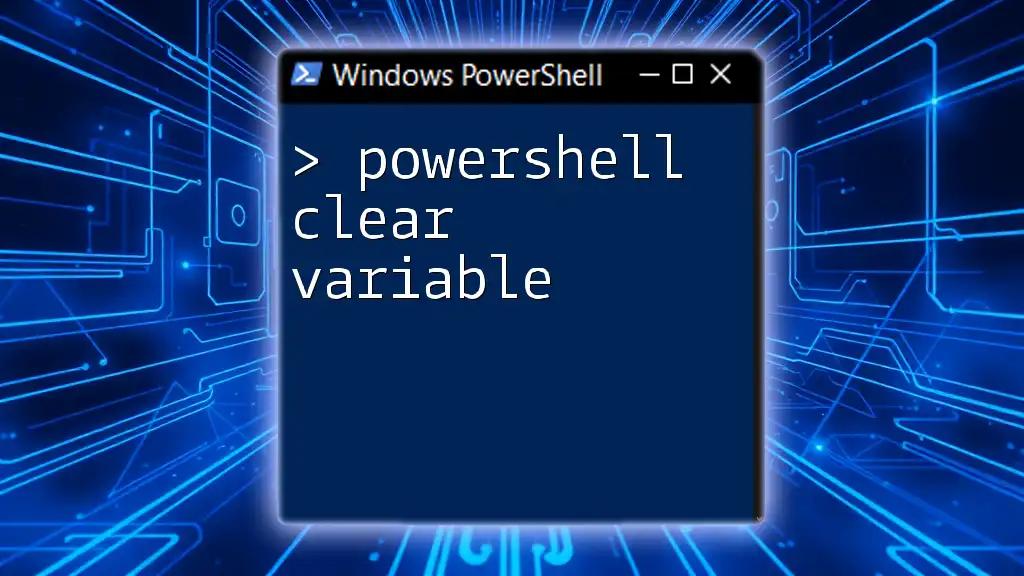
Conclusion
Understanding how to effectively display variable values in PowerShell is vital for debugging and user interaction. By using various cmdlets and techniques, you enhance the readability and utility of your scripts. Practice showcasing variable values with the methods discussed to become proficient in PowerShell scripting.
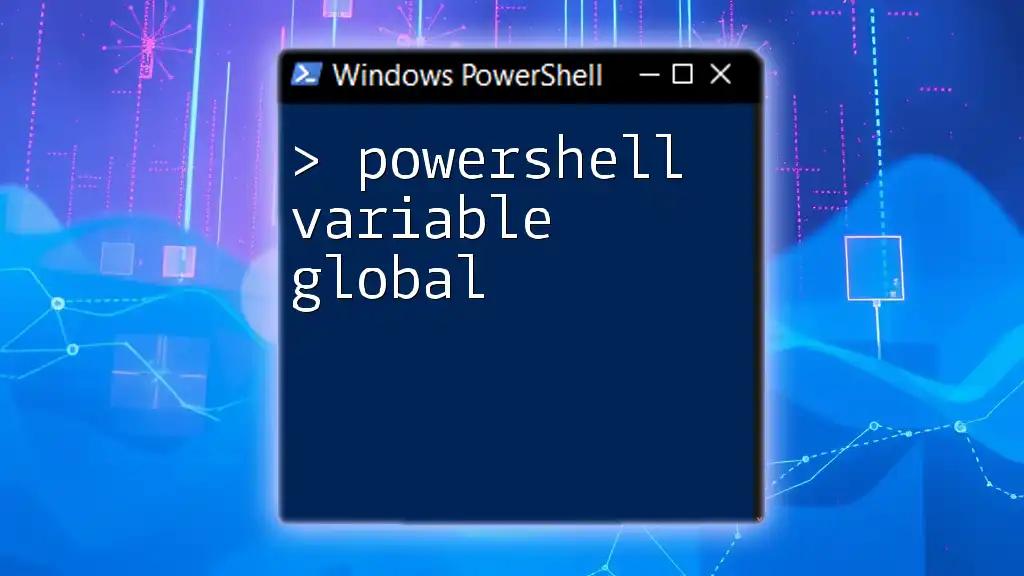
Call to Action
We encourage readers to leave comments or ask questions about their experiences with PowerShell. Don’t forget to explore additional resources on our website to further enhance your PowerShell skills!