In PowerShell, a global variable is accessible from anywhere within the script or function, allowing for seamless data sharing across different scopes.
$Global:MyVariable = "This is a global variable."
Write-Host $Global:MyVariable
Understanding Variables in PowerShell
What are Variables?
In PowerShell, variables are containers used to store data. They serve as a means to hold information, such as numbers, strings, objects, or arrays, which can be easily referenced throughout your scripts. The ability to manipulate these variables is crucial for efficient scripting, allowing users to perform a range of tasks and automate processes.
Different Types of Variables in PowerShell
PowerShell supports various types of variables, each with its own scope and specific use cases. These include:
- Local Variables: Accessible only within the function or script block where they are defined.
- Script Variables: Available throughout the script, but not outside of it.
- Global Variables: Accessible from anywhere in the PowerShell session, making them ideal for data that needs to be shared across multiple functions or scripts.
Understanding the scope of these variables is important as it influences how you manage and utilize data in your scripts.

What Are PowerShell Global Variables?
Definition of Global Variables in PowerShell
Global variables in PowerShell are defined using the `$global` scope modifier. They are available throughout the entire PowerShell session, which means you can access them from any function, loop, or script that runs during that session. This is particularly useful when you need to share data across multiple parts of your code without passing it explicitly as an argument.
Creating Global Variables in PowerShell
To create a global variable, you simply prefix your variable name with `$global:`. Here's the syntax to declare a global variable:
$global:MyGlobalVar = "Hello, World!"
In this example, `MyGlobalVar` is a global variable containing the string "Hello, World!". By using `$global:`, you ensure that this variable is accessible from any context in your PowerShell session.

Accessing PowerShell Global Variables
How to Retrieve a Global Variable
Accessing a global variable is straightforward. You use the same `$global:` prefix, followed by the variable name. For example, to retrieve the previously defined `MyGlobalVar`, you can use:
Write-Output $global:MyGlobalVar
This command will output "Hello, World!" to the console, demonstrating how global variables maintain their state across different parts of your script.
Modifying Global Variables
You can also modify global variables in a similar manner. To change the value of an existing global variable, simply assign a new value:
$global:MyGlobalVar = "New Value!"
After executing this command, if you retrieve `MyGlobalVar` again using `Write-Output` as shown previously, it will now display "New Value!".
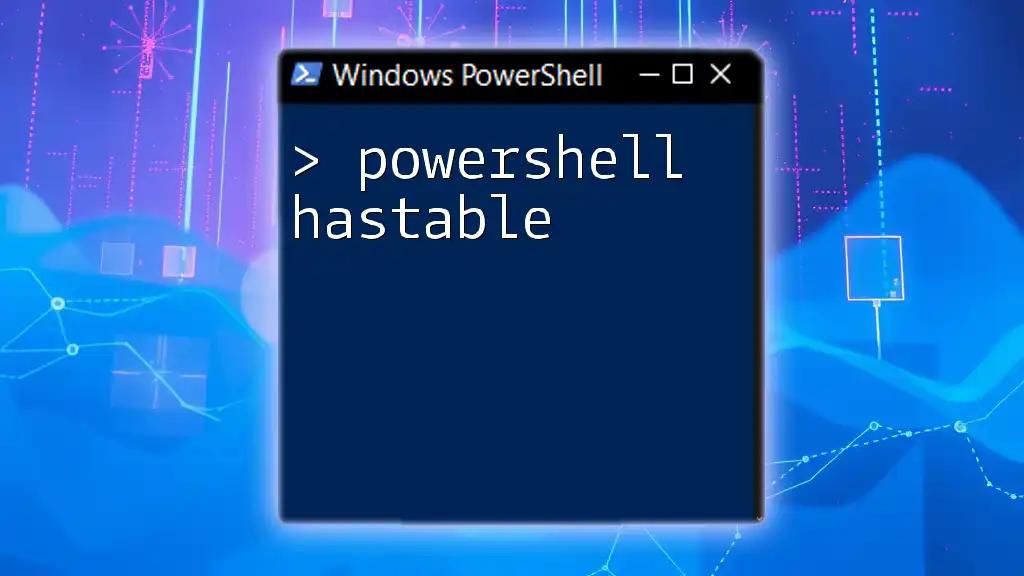
Use Cases for PowerShell Global Variables
When to Use Global Variables
Global variables can be particularly useful in certain scenarios:
-
Configuration Settings: If you have settings that need to be accessed by multiple scripts or functions, storing them in global variables can reduce redundancy and ease updates.
$global:ConfigFilePath = "C:\Config\settings.json"
-
Shared Resources Across Functions: Using global variables allows you to define resources that can be shared easily across different functions.
function Initialize-GlobalValues { $global:SharedValue = 100 } function Access-SharedValue { Write-Output $global:SharedValue }
Example Use Case: Configuration Settings
Imagine you have a script that relies on configuration settings from a JSON file. By defining the file path as a global variable, you can easily access it across different functions:
$global:ConfigFilePath = "C:\Path\To\Config.json"
function Load-Configuration {
# Load the configuration using the global variable
$config = Get-Content $global:ConfigFilePath | ConvertFrom-Json
return $config
}
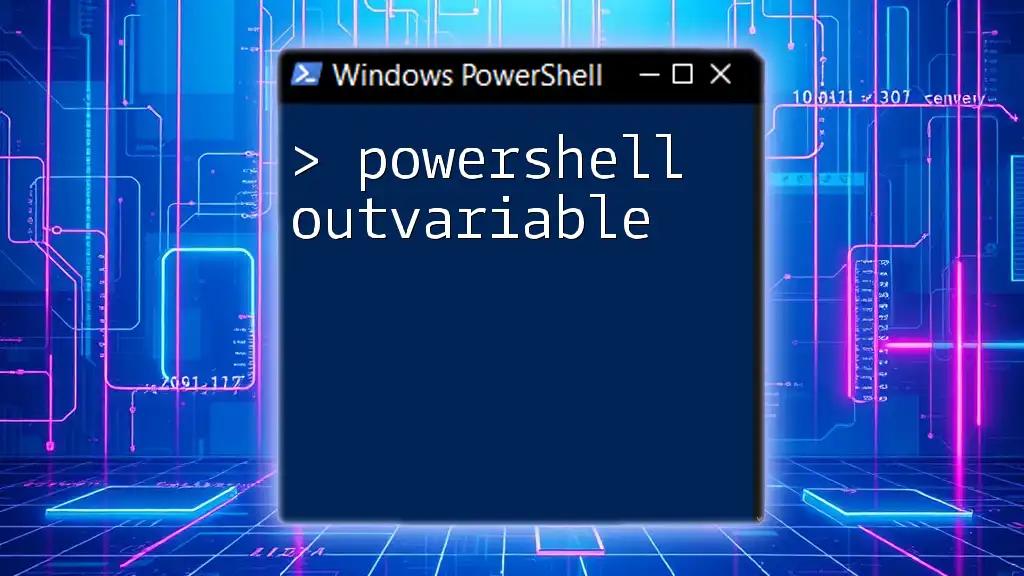
Common Pitfalls with Global Variables in PowerShell
Overwriting Global Variables
One significant risk associated with global variables is unintentional variable overwriting. If you have a global variable and then define a local variable with the same name, the local variable will take precedence within its scope, which could lead to unexpected behavior.
Performance Considerations
Overusing global variables can negatively impact performance and maintainability. They introduce hidden dependencies in your script, making it harder for others (or even yourself in the future) to understand what data is being manipulated. As a best practice, use global variables sparingly and prefer local or script scoped variables when possible.

Best Practices for Using Global Variables in PowerShell
When to Avoid Global Variables
While global variables can be useful, you should avoid them in scenarios that require encapsulation or when functions can be self-contained. Using local variables can lead to clearer and more predictable code, which can help in debugging and maintaining scripts.
Naming Conventions for Global Variables
To prevent conflicts and possible confusion, adhere to a clear naming convention for global variables. Consider the following tips:
- Use a prefix, such as `g_` or `Global_`, to clearly indicate a variable's scope.
- Provide descriptive names that indicate the purpose of the variable.
By following these conventions, you help maintain code clarity and prevent potential naming clashes.
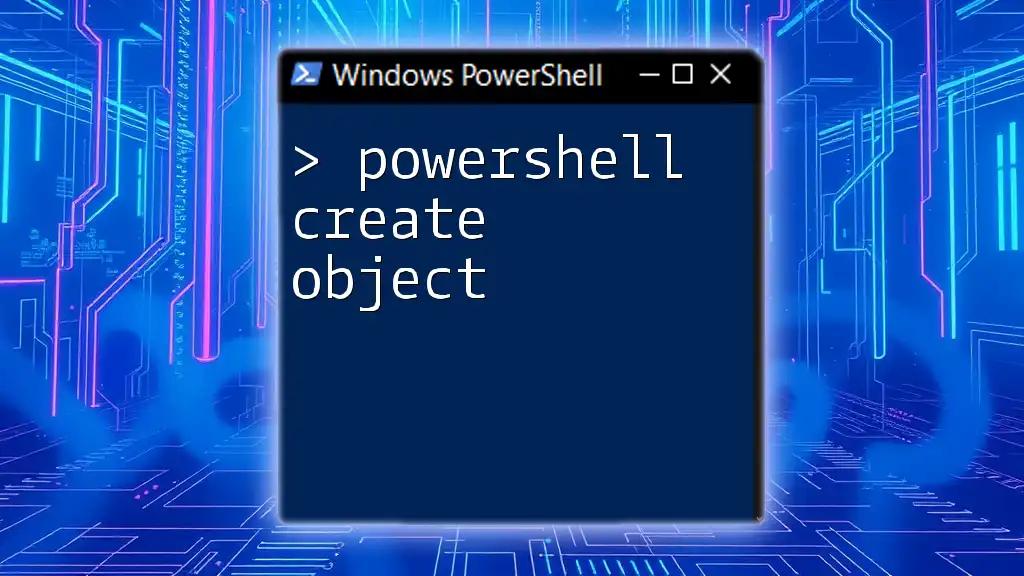
Conclusion
In summary, PowerShell global variables are a powerful tool when used appropriately. They provide an effective way to share information across various functions and scripts within a session. However, it's essential to be mindful of their potential pitfalls, such as unintentional overwriting and performance impacts. Following best practices and understanding when to use local vs. global variables will help you write efficient and clear scripts.
For further learning, consider exploring PowerShell documentation, community forums, and other educational resources that delve deeper into variable management in PowerShell.