Powershell garbage collection refers to the automatic process of reclaiming memory by removing unused or unreferenced objects from memory, thereby improving application performance.
Here's a simple code snippet to manually trigger garbage collection in PowerShell:
[System.GC]::Collect()
What is Garbage Collection?
Garbage Collection is an automatic memory management process that reclaims memory occupied by objects that are no longer in use. It plays a crucial role in application performance and stability by preventing memory leaks, which can lead to increased memory consumption and diminished performance over time.
Purpose
Understanding garbage collection is essential for effective memory management. It alleviates the burden on developers to manually manage memory allocation and deallocation, making it easier to build reliable applications without leaks. PowerShell garbage collection works under the principle that the system must reclaim memory from objects that are no longer referenced, allowing for the efficient use of system resources.
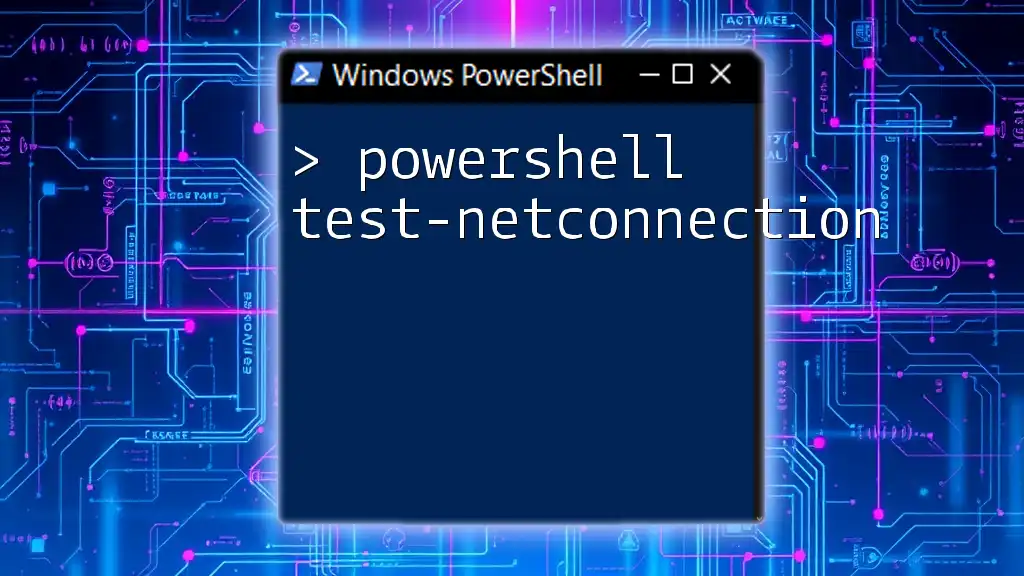
How PowerShell Handles Garbage Collection
PowerShell and the .NET Framework
PowerShell is closely integrated with the .NET Framework, inheriting its memory management features. The .NET garbage collector is responsible for managing memory in PowerShell scripts, working in the background to assess which objects are still being used and which can release their allocated memory.
The Role of the .NET Garbage Collector
The .NET garbage collector operates on a generational approach to optimize performance. Here’s how it categorizes objects in memory:
- Generation 0: This is where newly created objects reside. They are typically very short-lived.
- Generation 1: Objects that have survived the initial garbage collection are promoted to this generation, implying they are somewhat longer-lived.
- Generation 2: This generation holds the oldest objects and is subject to collection less frequently.
This hierarchy allows the garbage collector to be efficient, primarily focusing on collecting short-lived objects in Generation 0 while collecting older objects less often.
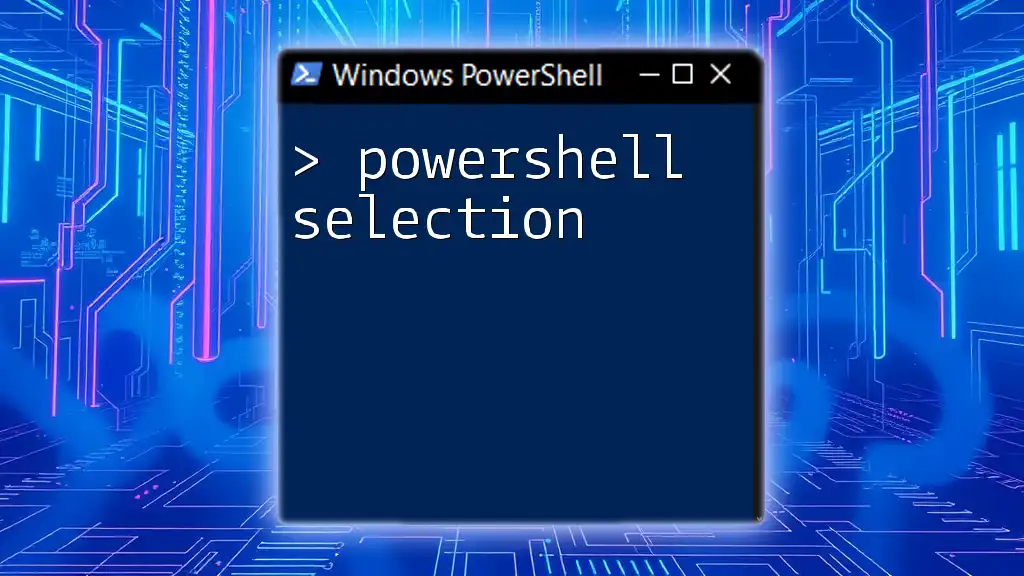
Understanding Garbage Collection in PowerShell
Automatic vs. Manual Garbage Collection
In PowerShell, garbage collection is mostly an automatic process. The garbage collector automatically triggers collections based on specific conditions such as memory pressure—when the system runs low on available memory or the application's memory limit is reached.
However, developers can also trigger garbage collection manually using the following command:
[System.GC]::Collect()
This command explicitly instructs the garbage collector to reclaim memory, although it should be used sparingly to avoid potential performance penalties.
When is Garbage Collection Triggered?
Several factors can influence when garbage collection is performed:
- Memory Pressure: If the system detects low memory availability, garbage collection is triggered to free up space.
- Object Lifetime: Objects that stick around longer than typical, especially in long-running applications, can prompt garbage collection for older generations.
Understanding these triggers can greatly aid in resource management in PowerShell scripts.
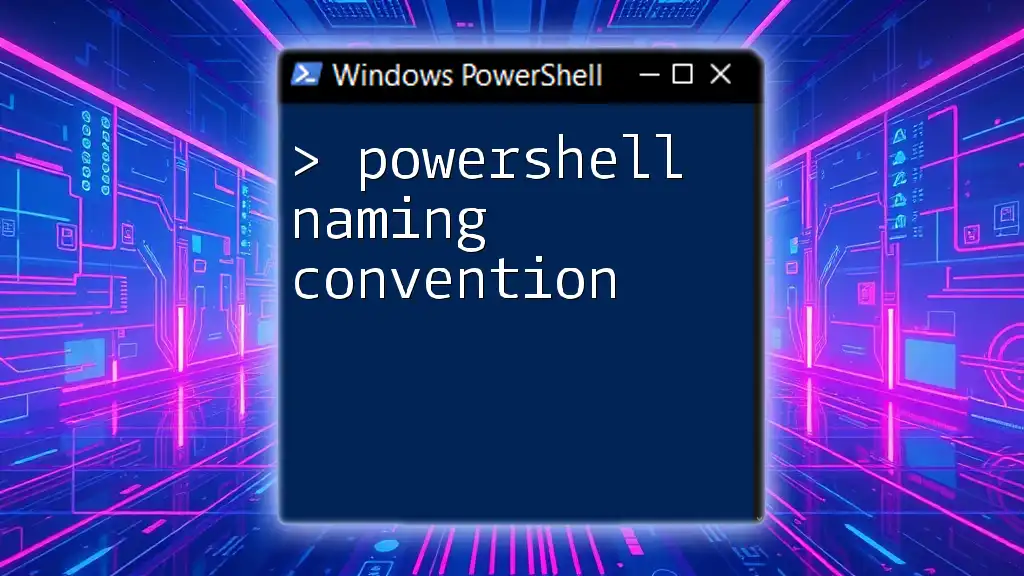
Monitoring and Managing Memory Usage in PowerShell
Memory Management Tools in PowerShell
PowerShell provides built-in cmdlets that can be leveraged to monitor memory usage effectively. One useful cmdlet is `Get-Process`, which can provide insights into running processes and their memory consumption.
For example, you can quickly identify the top 10 memory-consuming processes using the following command:
Get-Process | Sort-Object WorkingSet -Descending | Select-Object -First 10
This command lists processes sorted by their working set size, allowing developers to pinpoint which applications might be consuming excessive resources.
Using Performance Counter in PowerShell
You can also utilize performance counters to monitor various .NET memory metrics. For example, the following command retrieves the size of all heaps managed by the CLR:
Get-Counter '\.NET CLR Memory(*)\# Bytes in all Heaps'
This allows for a deeper analysis of memory utilization and can help identify trends or potential issues over time.
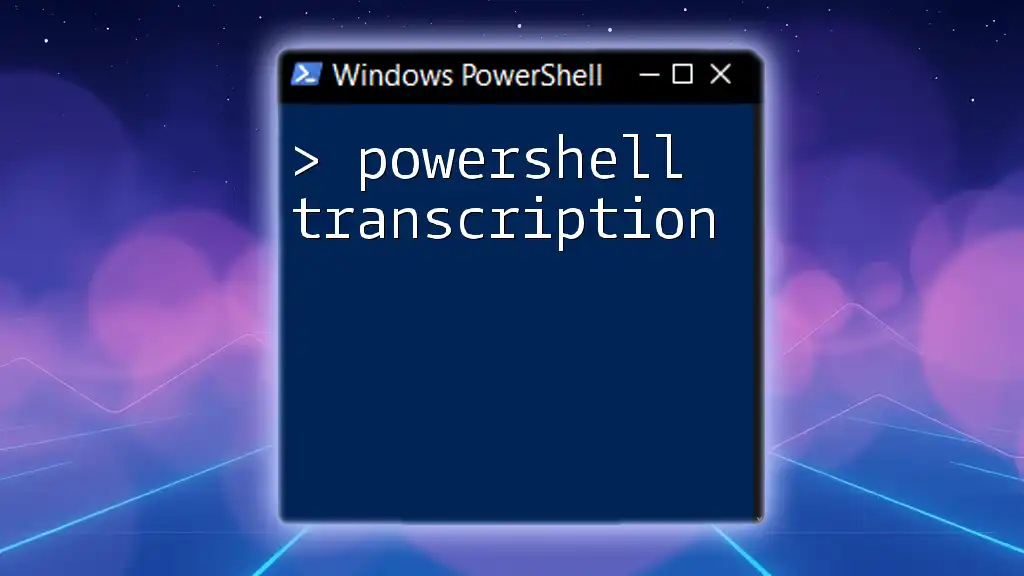
Best Practices for Effective Memory Management
Dispose Unmanaged Resources
A significant aspect of memory management in PowerShell is properly disposing of unmanaged resources, which do not get cleaned up automatically. The `Dispose` method is essential for releasing these resources.
For instance:
$resource = New-Object SomeResource
try {
# Use the resource
} finally {
$resource.Dispose()
}
Employing the `try`...`finally` pattern ensures that resources are always disposed of, even if an error occurs during their use.
Avoiding Memory Leaks
Memory leaks can negatively impact application performance and stability. Common pitfalls include unremoved event handlers and circular references, which can inadvertently prevent garbage collection.
To manage event handlers more effectively, you should explicitly remove them when no longer needed:
$someObject.RemoveHandler('EventName', $someEventHandler)
By following these practices, you can significantly reduce the risk of memory leaks and ensure that your PowerShell scripts run efficiently.
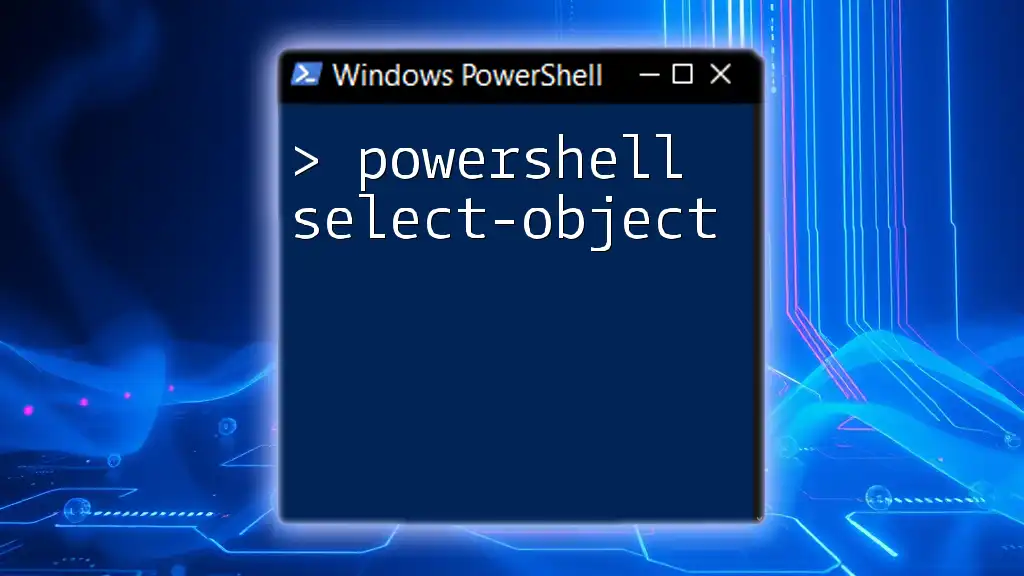
Conclusion
Understanding PowerShell garbage collection is essential for effective memory management and optimal application performance. By utilizing automatic and manual garbage collection techniques, leveraging monitoring tools, and adhering to best practices, developers can maintain the efficiency of their scripts. Emphasizing proper disposal of unmanaged resources and recognizing the potential for memory leaks will prepare you for building robust, efficient applications in PowerShell.
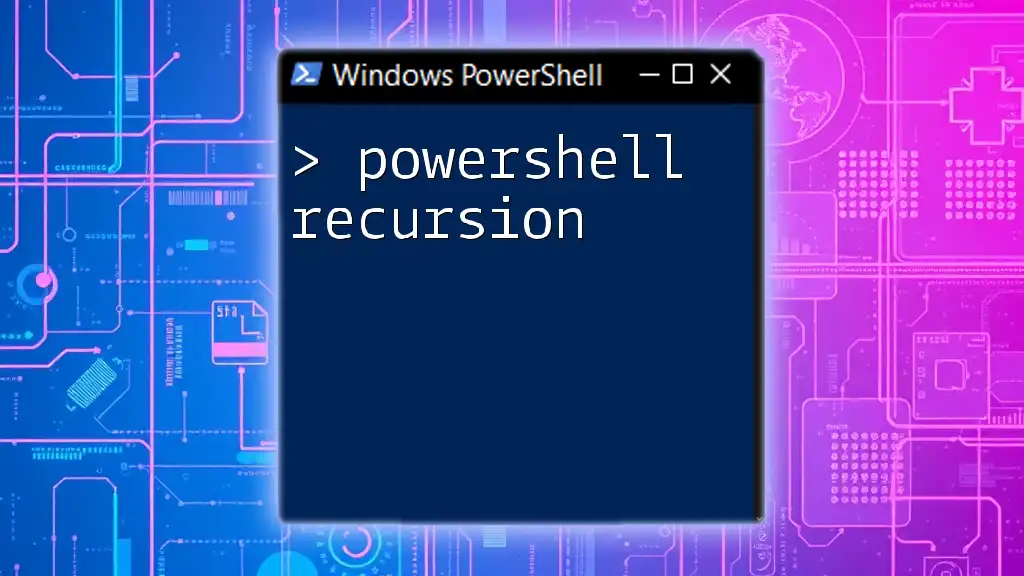
Additional Resources
For additional insights, consider exploring the official Microsoft documentation on PowerShell and garbage collection, as well as advanced memory management techniques within the .NET ecosystem.
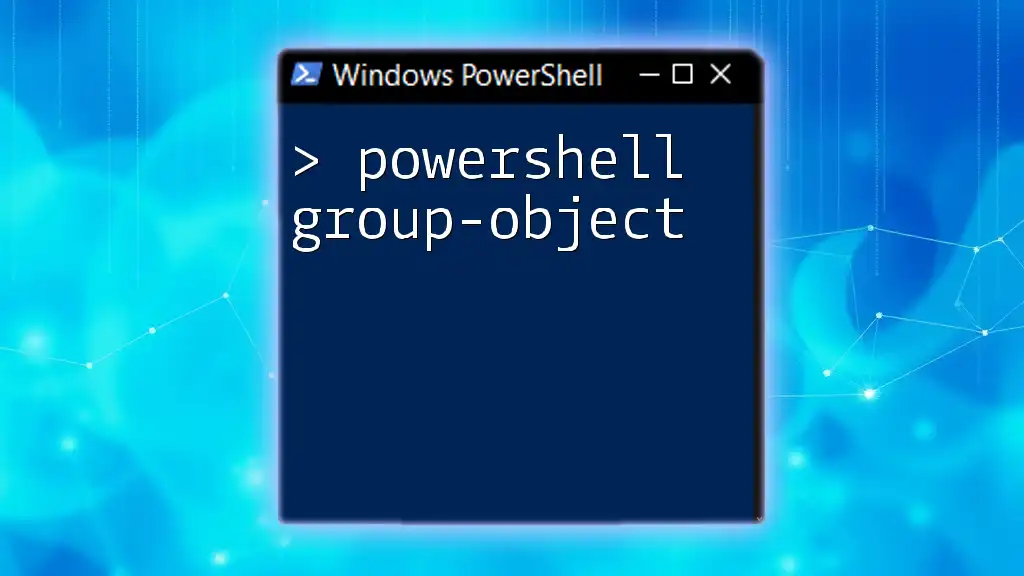
Call to Action
Stay ahead in your PowerShell journey by subscribing for more concise tips and tricks. Don’t forget to download our free checklist on best practices for effective memory management!