Creating a function in PowerShell allows you to encapsulate a block of code that can be executed whenever the function is called, streamlining repetitive tasks.
Here's a simple example:
function Say-Hello {
Write-Host 'Hello, World!'
}
What is a PowerShell Function?
A PowerShell function is a block of code designed to perform a specific task. Functions in programming allow for encapsulation of functionality, supporting the principles of code reuse and organization. By creating functions in PowerShell, you can structure your scripts more logically, making them easier to read and maintain.
Key Benefits of Using Functions
Using PowerShell functions provides numerous advantages:
- Simplifies Complex Scripts: Breaking down large tasks into smaller, manageable functions allows for clearer logic.
- Improves Code Readability: Named functions convey their purpose, enhancing understanding of script functionality.
- Promotes Code Reusability: Once a function is defined, it can be called multiple times without rewriting the same code.
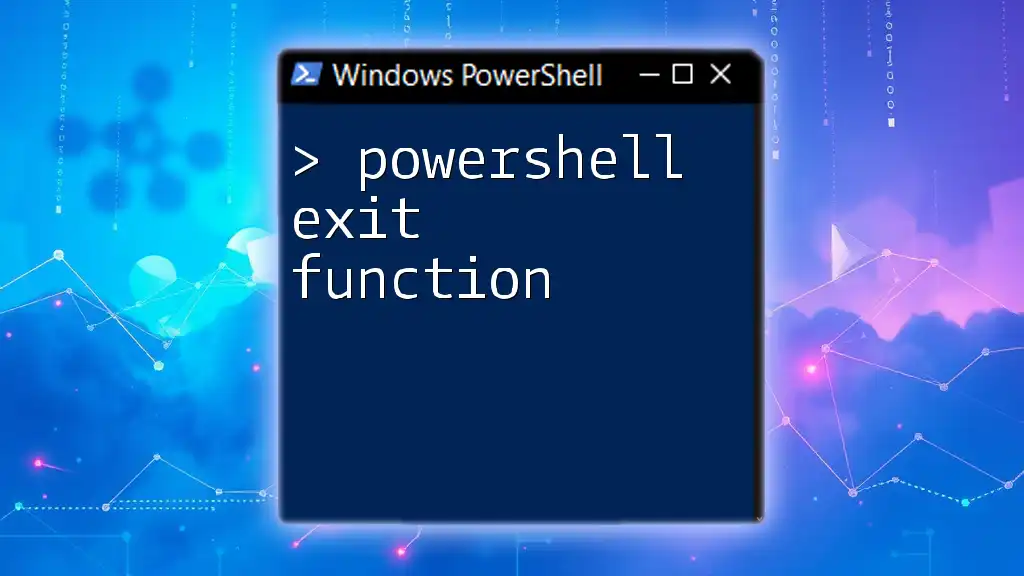
Syntax of PowerShell Functions
Basic Structure
The syntax for declaring a PowerShell function is straightforward. Here’s the basic structure:
function FunctionName {
# Code block
}
In this structure, `FunctionName` is the name you'll use to call your function.
Parameters in Functions
Functions often require input in the form of parameters. You can define parameters in your function declaration like this:
function FunctionName {
param (
[string]$Parameter1,
[int]$Parameter2
)
# Code block utilizing parameters
}
In this example, `FunctionName` takes a string and an integer as parameters. This design allows for dynamic input, adapting the function’s behavior based on different values.
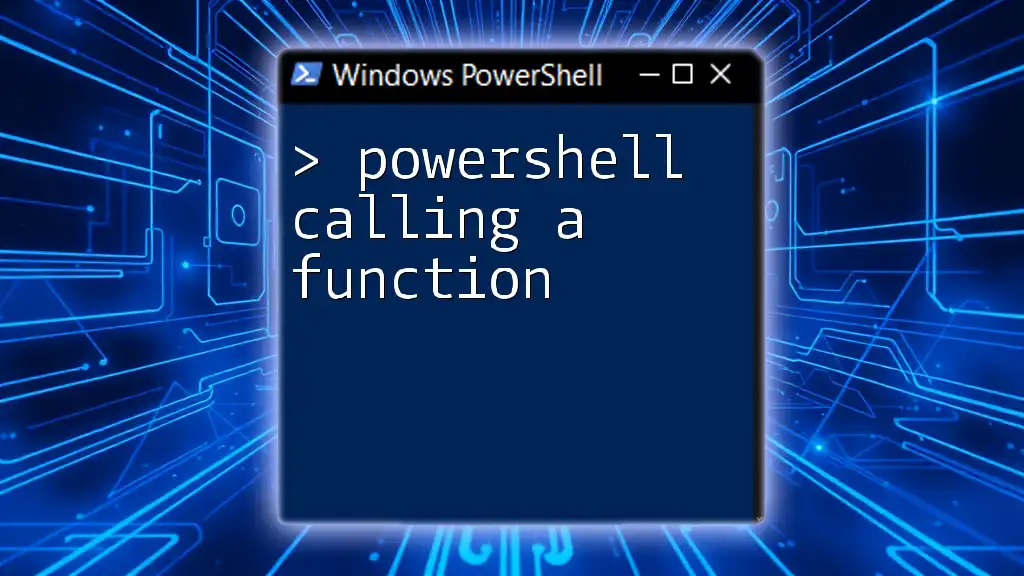
Creating a Simple PowerShell Function
Step-by-Step Example
Let’s create a simple function that greets users. This example will help illustrate how functions work in practical terms.
function Greet-User {
param (
[string]$UserName
)
"Hello, $UserName!"
}
This `Greet-User` function takes a single parameter, `$UserName`, and outputs a greeting.
How to Call Your Function
Invoking your function is straightforward. To greet a user named Alice, simply call the function like this:
Greet-User -UserName "Alice"
Executing this command will result in the output: Hello, Alice!
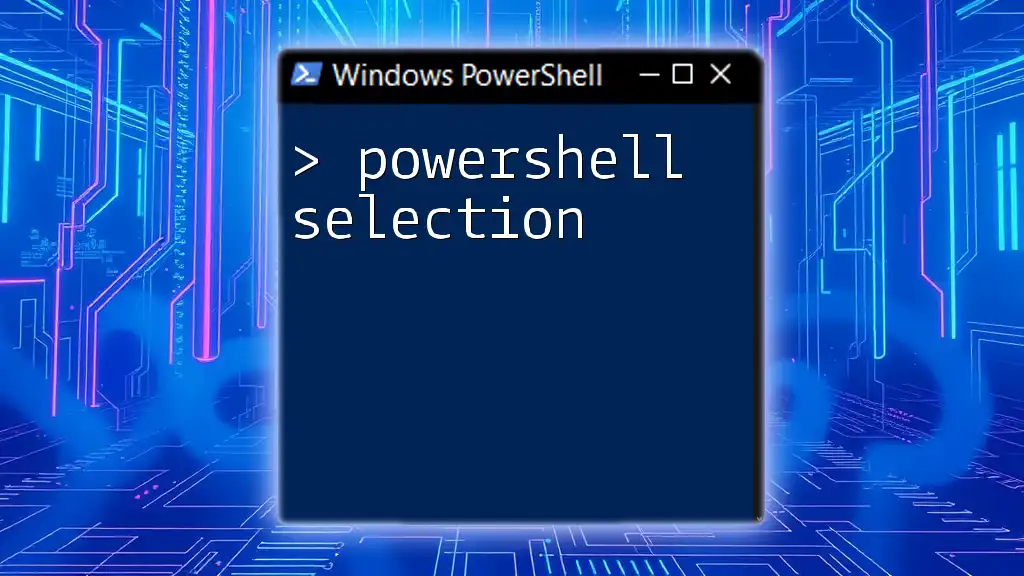
Advanced PowerShell Function Features
Return Values from Functions
Functions can also return values, enhancing their utility. Here’s how to create a function that adds two numbers:
function Add-Numbers {
param (
[int]$Num1,
[int]$Num2
)
return $Num1 + $Num2
}
In this example, `Add-Numbers` takes two integers and returns their sum. You can call this function as follows:
$sum = Add-Numbers -Num1 5 -Num2 10
Write-Host "The sum is: $sum"
This will display: The sum is: 15.
Using Cmdlets and Other Functions in PowerShell Functions
You can heighten the complexity of your functions by incorporating cmdlets or other functions. Here’s a sample function that retrieves file information:
function Get-FileInfo {
param (
[string]$Path
)
Get-ChildItem -Path $Path | Select-Object Name, Length, LastWriteTime
}
This function utilizes the `Get-ChildItem` cmdlet to fetch details about files in the specified path. When you call this function with a valid path, it returns the file names along with their sizes and last modified dates.
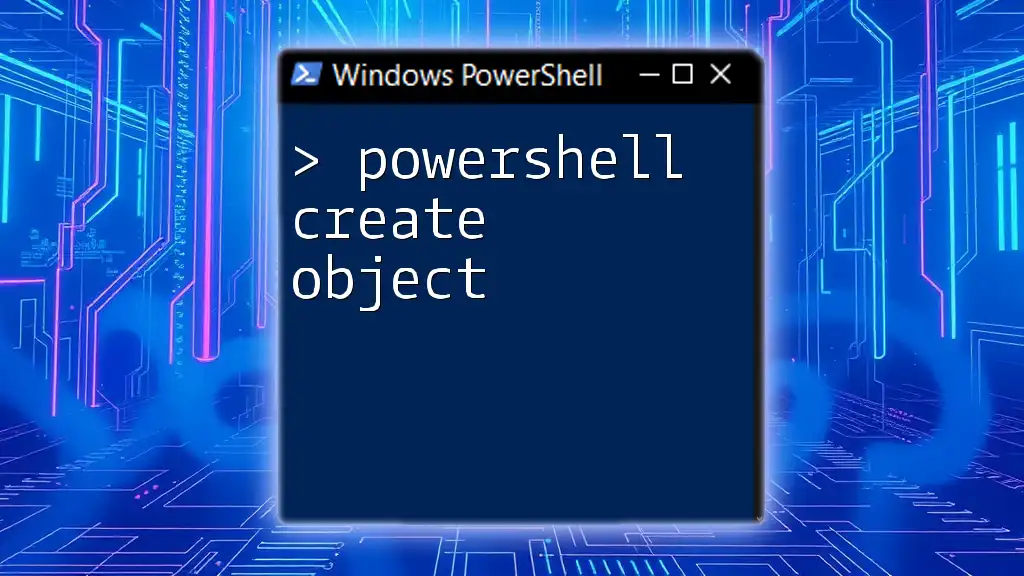
Function Scopes and Lifetime
Understanding Scope
Scope in PowerShell refers to the visibility of variables and functions.
- Global Scope: Variables and functions declared in the global scope are accessible anywhere in the PowerShell session.
- Local Scope: Variables initialized inside a function are local to that function and cannot be accessed outside.
Understanding scope is crucial for effective scripting. For example:
function Test-Scope {
$localVariable = "I am local"
$global:globalVariable = "I am global"
}
Test-Scope
Write-Host $localVariable # This will generate an error.
Write-Host $global:globalVariable # This will output "I am global".
Lifetime of Variables in Functions
Variables defined within a function only exist while that function is executing. Once the function completes, those variables are gone unless explicitly declared as global.
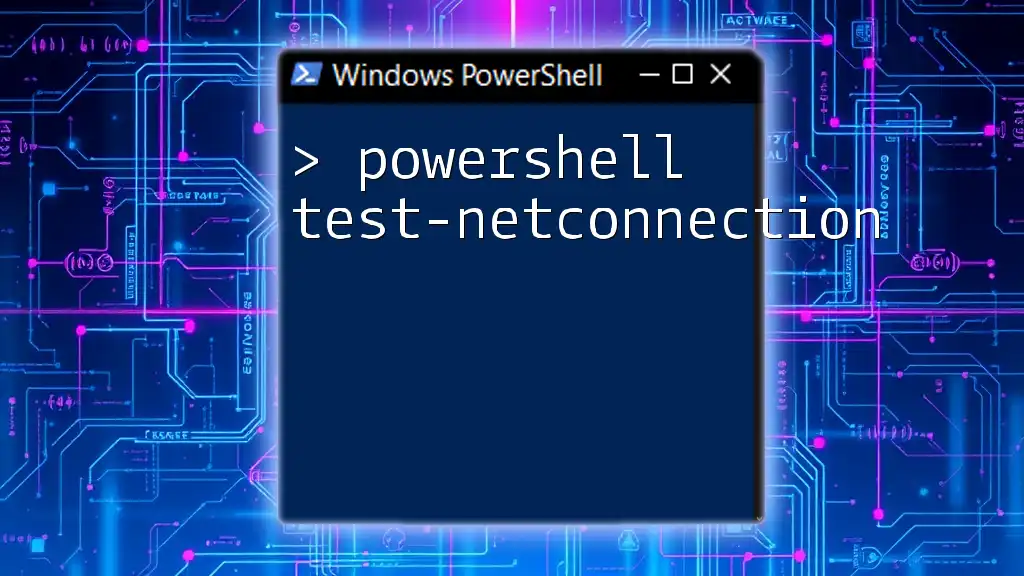
Best Practices for Writing PowerShell Functions
Naming Conventions
Choosing effective names for your functions contributes greatly to readability. Functions should use clear, descriptive names that indicate their purpose, such as `Get-UserInfo` or `Remove-File`.
Documentation and Comments
Proper documentation is vital for maintaining and understanding scripts. Use comment-based help to describe your function’s purpose, parameters, and examples. Here’s an example:
function Get-UserInfo {
<#
.SYNOPSIS
Retrieves user information based on user ID.
.PARAMETER UserID
The ID of the user to retrieve information for.
#>
param ([string]$UserID)
# Code implementation goes here.
}
With this format, anyone using your function can easily understand its functionality and how to use it.
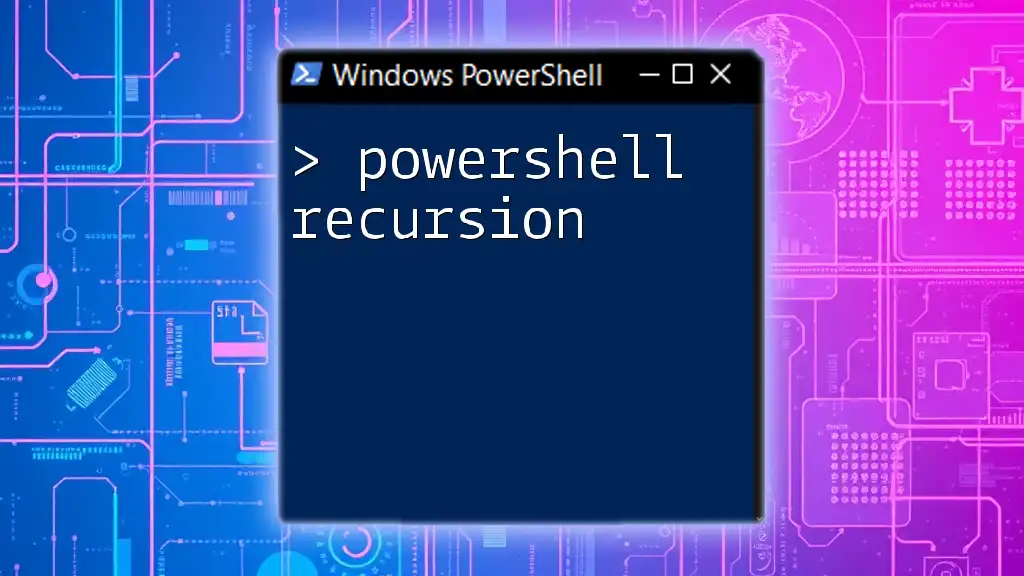
Common PowerShell Function Scenarios
Scripts with Multiple Functions
Creating scripts with several interconnected functions can enhance functionality and efficiency. Consider this script managing user records:
function Create-User {
param ([string]$UserName)
# Implementation for creating a user
}
function Remove-User {
param ([string]$UserName)
# Implementation for removing a user
}
function List-Users {
# Implementation for listing users
}
# Example usage
Create-User -UserName "Mike"
List-Users
This structure provides modularity, allowing you to manage user data through individual, reusable functions.
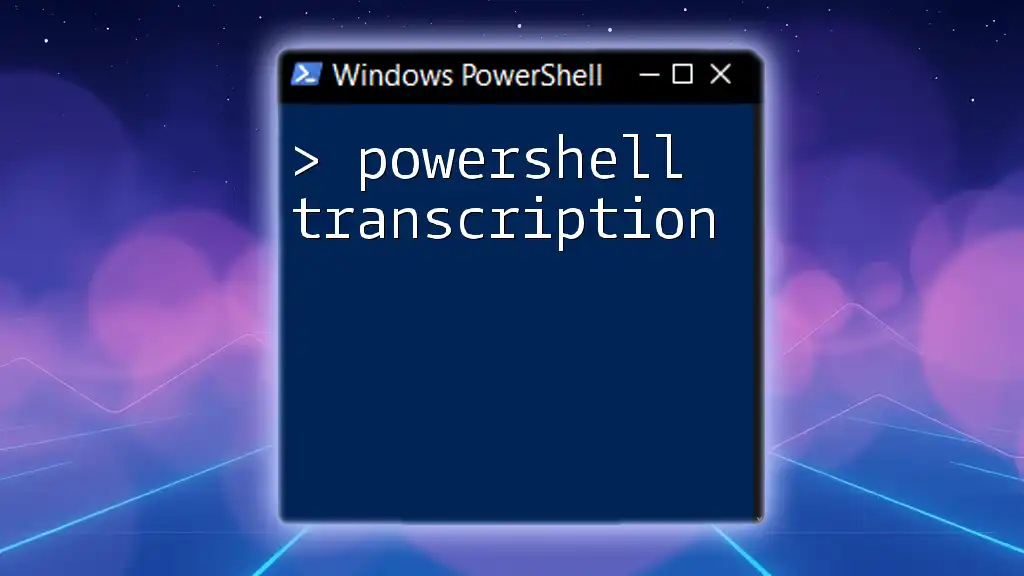
Troubleshooting PowerShell Functions
Common Errors and How to Resolve Them
While working with functions, you may encounter errors. Common symptoms include incorrect parameter usage or scope-related issues. PowerShell provides informative error messages that can help diagnose problems.
For instance, if you forget to specify a required parameter when calling a function, PowerShell will alert you:
Greet-User
This command will prompt an error, indicating that the parameter `$UserName` is missing. Always double-check parameters and variable scopes when troubleshooting.
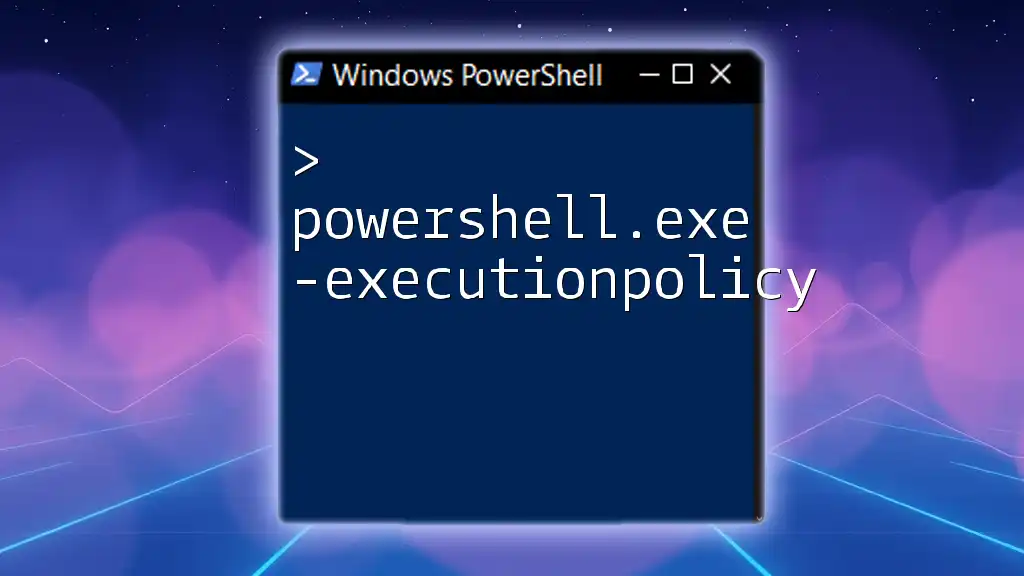
Conclusion
In summary, knowing how to create functions in PowerShell is a foundational skill that empowers you to write cleaner, more efficient scripts. From defining parameters to understanding scope, mastering the nuances of functions will greatly enhance your PowerShell scripting abilities. With practice, you can develop scripts that are not only powerful but also easy to read and maintain.
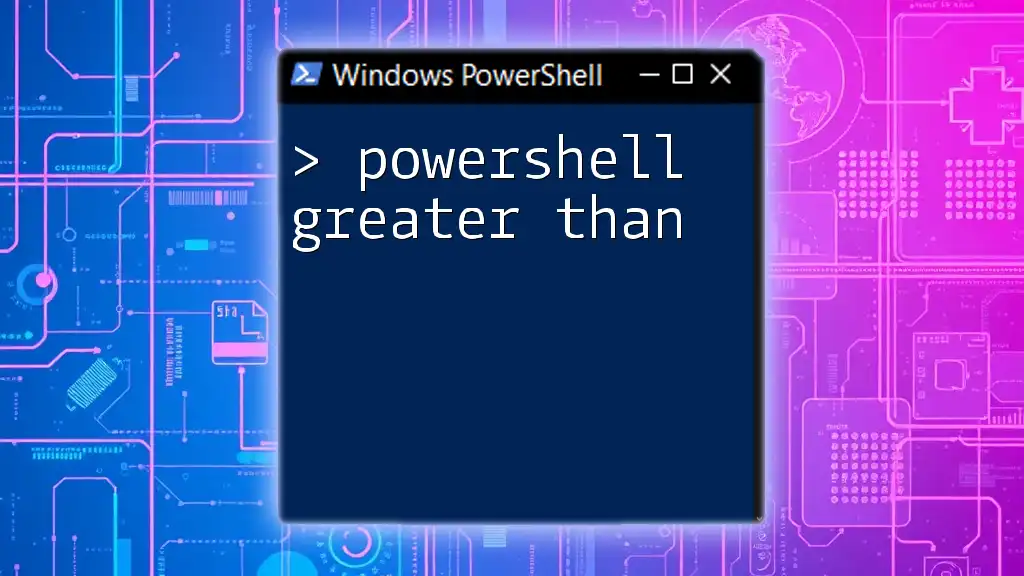
Additional Resources
For those looking to deepen their understanding, exploring the official Microsoft PowerShell documentation and engaging with online communities can provide valuable insights and support. Additionally, consider reading books focused on advanced PowerShell scripting techniques to elevate your skills further.