A PowerShell beautifier is a tool or script that formats and organizes PowerShell code for better readability and structure, ensuring that scripts are visually appealing and easier to understand.
# Example PowerShell script beautifier
$script = @"
function Greet {
param([string]$name)
Write-Host "Hello, $name!"
}
Greet -name "World"
"@
$formattedScript = $script -replace "function", "`nfunction" -replace "\{", "`n{`n" -replace "\}", "`n}"
$formattedScript
What is a PowerShell Beautifier?
A PowerShell beautifier is a tool or technique designed to improve the readability and organization of PowerShell scripts. By formatting code in a clear, consistent manner, a beautifier enhances both the aesthetic appeal of the script and its maintainability. As the complexity of PowerShell scripts increases, so does the importance of readability.
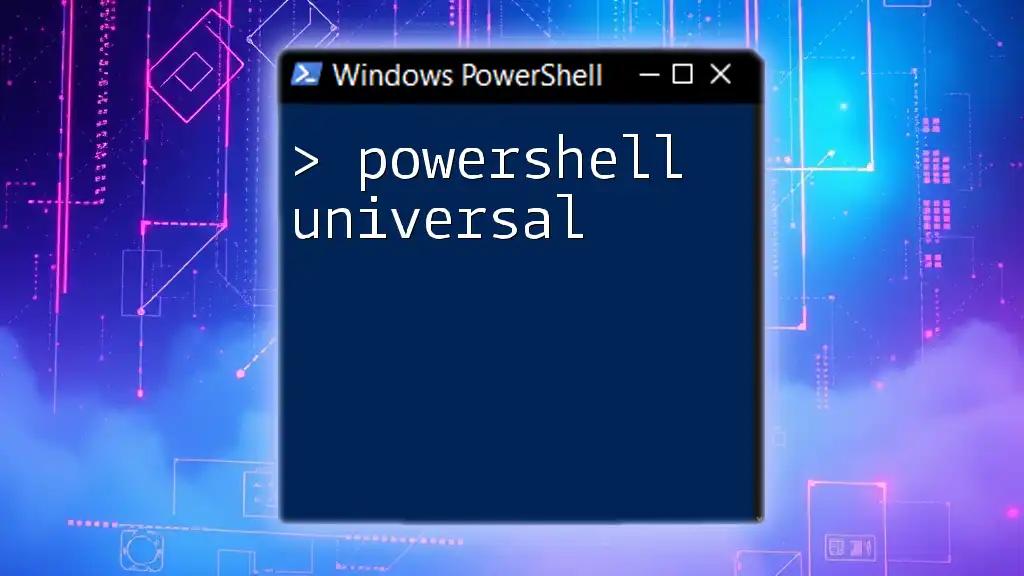
Why You Should Use a PowerShell Beautifier
Enhances Code Readability
Readable code is fundamental for effective collaboration among team members. When scripts are well-structured, they convey their purpose more clearly, making it easier for others (or even the original author at a later date) to understand the logic behind the commands. Poorly formatted code can appear daunting and obscure, leading to misinterpretations.
Example of before and after beautification:
Before beautification:
if($a -eq 1){Write-Host 'A is one'}else{Write-Host 'A is not one'}
After beautification:
if ($a -eq 1) {
Write-Host 'A is one'
} else {
Write-Host 'A is not one'
}
Increases Efficiency
Investing time in beautifying PowerShell scripts can significantly enhance development efficiency. When code is properly formatted, it allows for quicker navigation and understanding, speeding up both coding and debugging processes.
Consider this scenario: A developer is handed a 100-line PowerShell script. If that script is poorly formatted, it may take longer to identify errors, understand logic, or implement changes. With a beautified version, the developer can easily pinpoint issues and make adjustments rapidly.
Error Reduction
A well-organized script reduces the chances of introducing bugs. Many common mistakes, like missing brackets or incorrect command placements, can be easily spotted in a neatly formatted codebase.
For instance:
# Poorly formatted:
foreach($item in $collection){Process-Item $item -Verbose; if($item -eq $null){Write-Host 'Item is null'}}
# Beautified:
foreach ($item in $collection) {
Process-Item $item -Verbose
if ($item -eq $null) {
Write-Host 'Item is null'
}
}
In the beautified version, it's straightforward to see the structure of the blocks and to identify any potential issues.
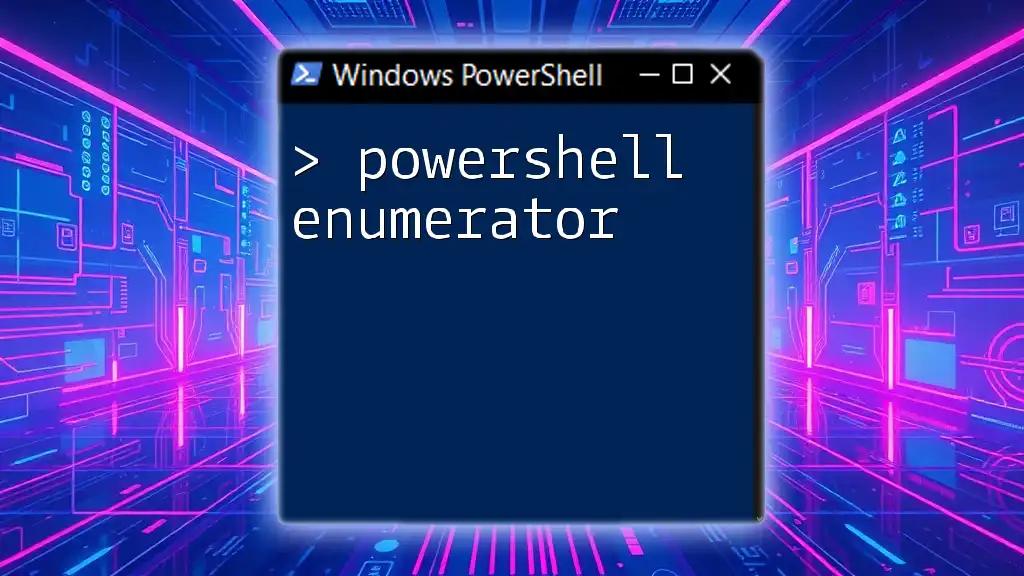
Key Features of PowerShell Beautifiers
Formatting Options
PowerShell beautifiers provide various formatting features that help maintain consistency within your scripts. Common features include:
- Indentation: Proper indentation aligns code blocks visually, indicating hierarchy and flow.
- Line breaks: Inserting line breaks can prevent lengthy lines, allowing scripts to be scanned easily without scrolling.
Example of indentation improvement:
# Unindented:
if($x -gt 10){Write-Host "Greater"; for($i=0;$i -lt 5;$i++){Write-Host $i}}
# Indented properly:
if ($x -gt 10) {
Write-Host "Greater"
for ($i = 0; $i -lt 5; $i++) {
Write-Host $i
}
}
Syntax Highlighting
Syntax highlighting can drastically improve focus and reduce cognitive load by visually distinguishing commands, variables, and strings. If a function name stands out in a bright color, for example, it allows the developer to quickly differentiate between different components of the script.
Comment Management
Incorporating comments into scripts is essential for providing context, especially in collaborative environments. A beautifier often helps format comments for clarity, ensuring they are easily distinguishable from code.
Good commenting practices would look like:
# This function processes input and logs the output
function Process-Data {
param (
[string]$InputData
)
# Logging the action
Write-Host "Processing data..."
}

Popular PowerShell Beautifiers
Overview of Available Tools
Various tools can serve as effective PowerShell beautifiers, notably:
- PowerShell ISE: Comes with built-in beautification features, providing an interactive approach.
- Visual Studio Code: An incredibly popular editor with a PowerShell extension that supports many features, including auto-formatting.
- Online Beautifier Tools: These are excellent for quick beautification without installing software. Websites like "Code Beautify" offer easy-to-use interfaces.
- Third-Party Software: Tools like Notepad++ also offer plugins that can beautify PowerShell scripts.
Comparison of Features
Each tool comes with its strengths. Here's a quick comparison to help you choose:
- PowerShell ISE: Great for beginners, built-in support, and easy-to-access.
- Visual Studio Code: Highly customizable, supports extensions, and offers advanced functionalities.
- Online Tools: Good for quick fixes and adaptable for any system.
- Third-Party Software: Great for users already familiar with other coding activities outside PowerShell.
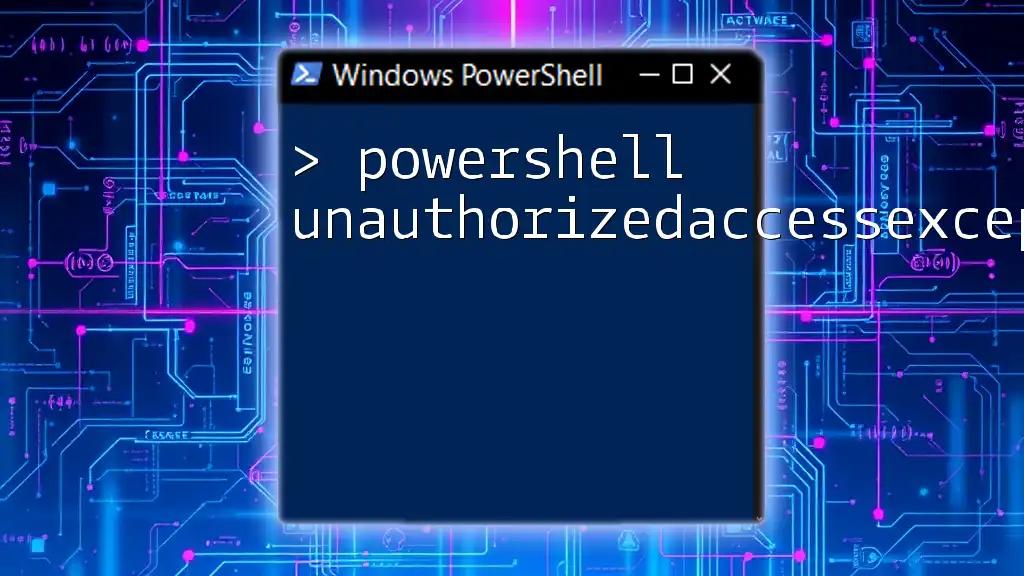
How to Use a PowerShell Beautifier
Using Visual Studio Code
To get started with beautifying PowerShell code in Visual Studio Code:
- Install Visual Studio Code and the PowerShell extension from the marketplace.
- Open your PowerShell script in the editor.
- Use the shortcut Shift + Alt + F to beautify your code.
- Customize settings in the settings JSON to adjust your preferred formatting.
Online Beautifier Tools
You can quickly beautify PowerShell scripts using online tools with these steps:
- Navigate to a tool like "Code Beautify".
- Copy and paste your script into the designated text area.
- Click the "Beautify" button.
- Copy the beautified script back to your workspace.
Using PowerShell ISE
PowerShell ISE also provides simple beautification options:
- Open your PowerShell script in ISE.
- Use Edit → Format Script from the menu.
- The script will be automatically formatted, improving its readability.
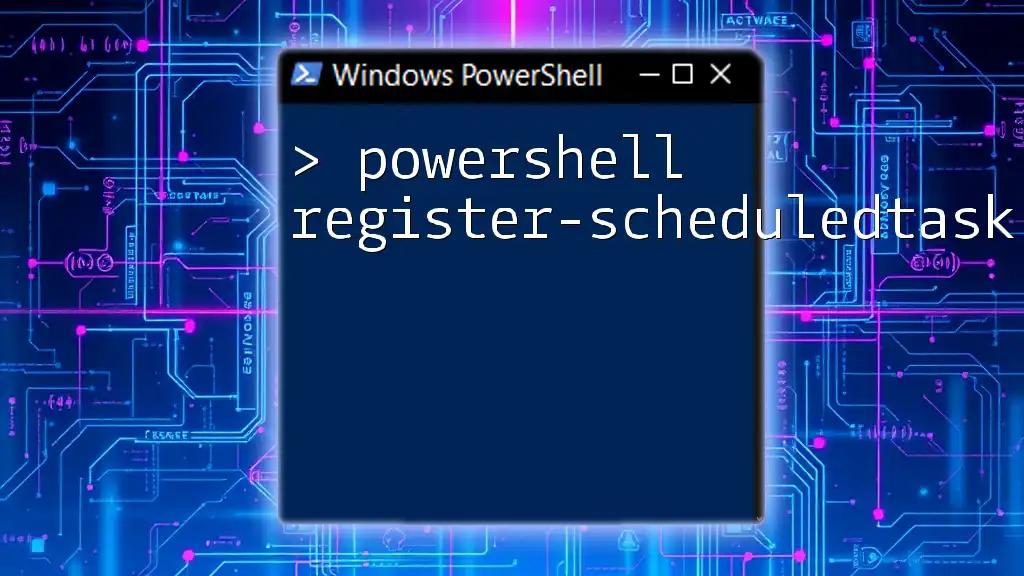
Best Practices for Beautifying PowerShell Code
Consistent Indentation and Line Breaks
Consistency is crucial for readability. Establishing a coding style guide for your team ensures that everyone follows the same rules, making collaboration seamless. Adopting a standardized indentation format, such as two spaces, helps maintain uniformity throughout your scripts.
Commenting Wisely
Utilize comments effectively by providing context without overwhelming the reader. Use comments to explain the "why" behind complex logic, and keep them brief and to the point.
Refactoring Dirty Code
Regular code refactoring is essential. Review your scripts periodically to enhance their structure:
- Identify common patterns and create functions.
- Remove redundant code.
- Break large scripts into modular sections.
Example of refactoring: Before:
if ($temp -gt 100) { Write-Host "Too High"; } else { Write-Host "Temperature is fine"; }
After:
function Check-Temperature {
param ($temp)
if ($temp -gt 100) {
Write-Host "Too High"
} else {
Write-Host "Temperature is fine"
}
}
Check-Temperature -temp $temp
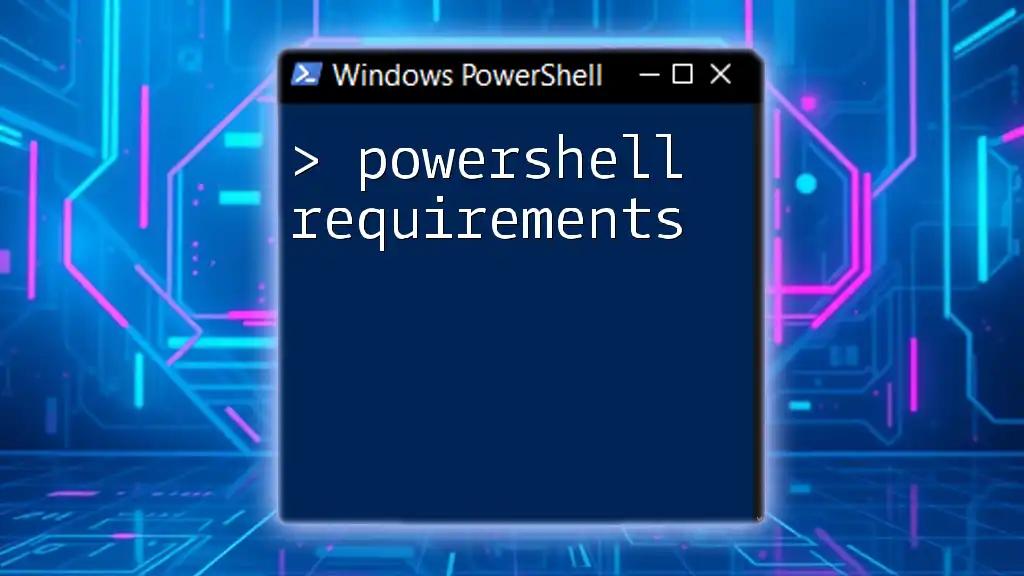
Common Challenges and Solutions
Dealing with Large Scripts
When working with extensive codebases, it's easy to lose track of structure. A beautifier helps to visualize control structures clearly. In some cases, chunking code into functions can enhance readability and functionality.
Case Study: Consider a 500-line script that processes various data entries. By utilizing a beautifier, you can identify modular components and refactor into functions, allowing for easier testing and debugging.
Integrating Beautifiers in CI/CD Pipelines
Automating beautification in CI/CD pipelines ensures that all submitted code adheres to style guidelines. Tools such as Prettier can be integrated into your pipeline, running formatting checks on each commit, immediately highlighting any unknown formatting issues.
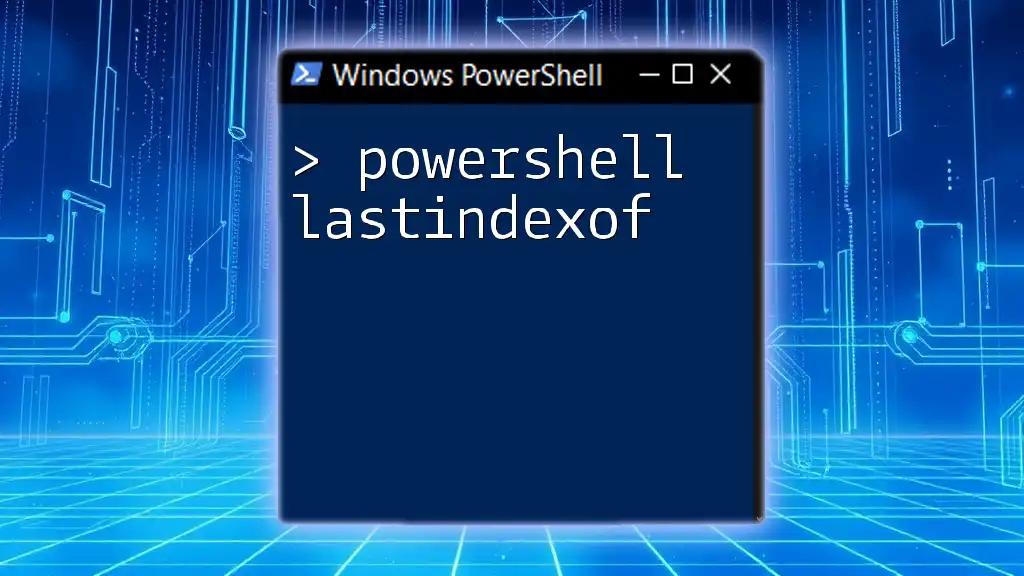
Conclusion
Understanding and utilizing a PowerShell beautifier is invaluable for creating clean, effective scripts. By enhancing readability, increasing efficiency, and reducing errors, a beautifier transforms coding—the benefits are immense. Whether you're a solo developer or part of a larger team, adopting beautification practices will contribute significantly to the success of your PowerShell projects.
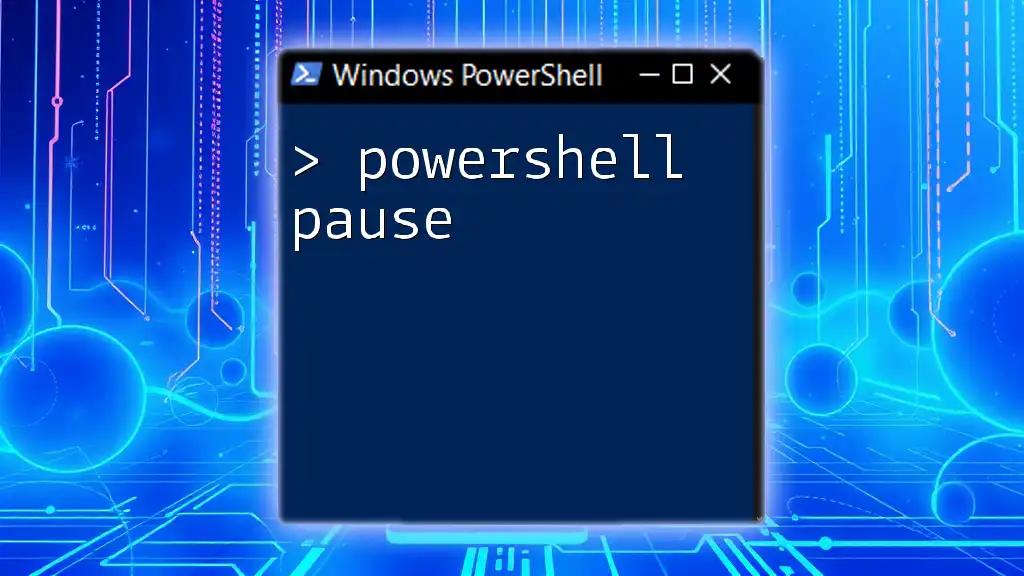
Additional Resources
For further exploration, consider delving into:
- Online tutorials focused on PowerShell scripting.
- PowerShell forums and communities for peer support.
- Official documentation from Microsoft regarding PowerShell best practices and tools.