In PowerShell, a carriage return is represented by the escape character `` `r ``, which moves the cursor to the beginning of the line without advancing to the next line, allowing subsequent output to overwrite previous output. Here’s a code snippet demonstrating its use:
Write-Host "Hello, World!`rOverwritten Text"
Understanding Carriage Return in PowerShell
What is a Carriage Return?
A carriage return refers to a control character that returns the cursor to the beginning of the line without advancing to the next line. In the context of text display, it can be crucial for formatting outputs correctly. In PowerShell, the carriage return is represented by the character `\r`. This character can be instrumental for creating cleaner outputs in scripts or when generating reports.
Importance of Carriage Return in Scripting
The carriage return character plays a significant role in how data is displayed in PowerShell scripts. It’s especially useful when you want to overwrite a line of text in the console output or create controlled, formatted outputs. For instance, when executing scripts that log status updates, using a carriage return allows you to refresh the existing line instead of creating clutter with multiple lines of output. This aids in making your scripts more readable and professional-looking.
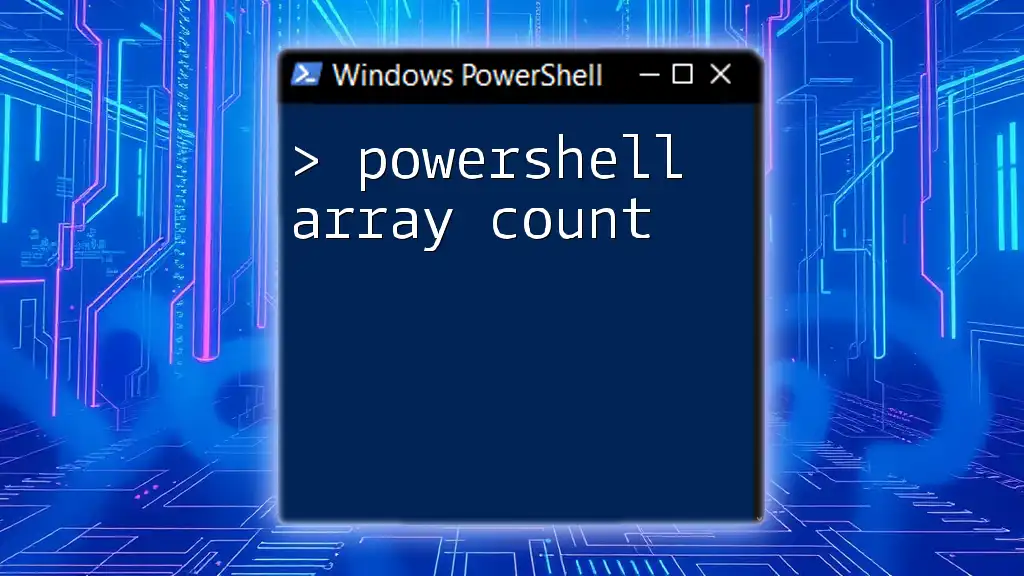
The Carriage Return Character in PowerShell
Representing Carriage Return
To represent a carriage return in PowerShell, you can use the escape sequence `\r`. This can be used in various contexts, most commonly in output commands. For example, using the `Write-Host` cmdlet with a carriage return signifies that subsequent text should overwrite the current line.
# Example of using carriage return
Write-Host "Hello World`rPowerShell"
When executed, this command outputs "PowerShell" on the same line where "Hello World" was initially displayed, effectively demonstrating how carriage return can modify outputs.
Combining Carriage Return with Other Characters
You can effectively combine the carriage return with the new line character `\n` to create a more complex output formatting structure. This enables you to move the cursor to the beginning of the line while also moving to the next line.
# Example of combining carriage return and new line
Write-Host "First Line`r`nSecond Line"
Here, "First Line" will be cleared and replaced by "Second Line", which appears on a new line due to the new line character.
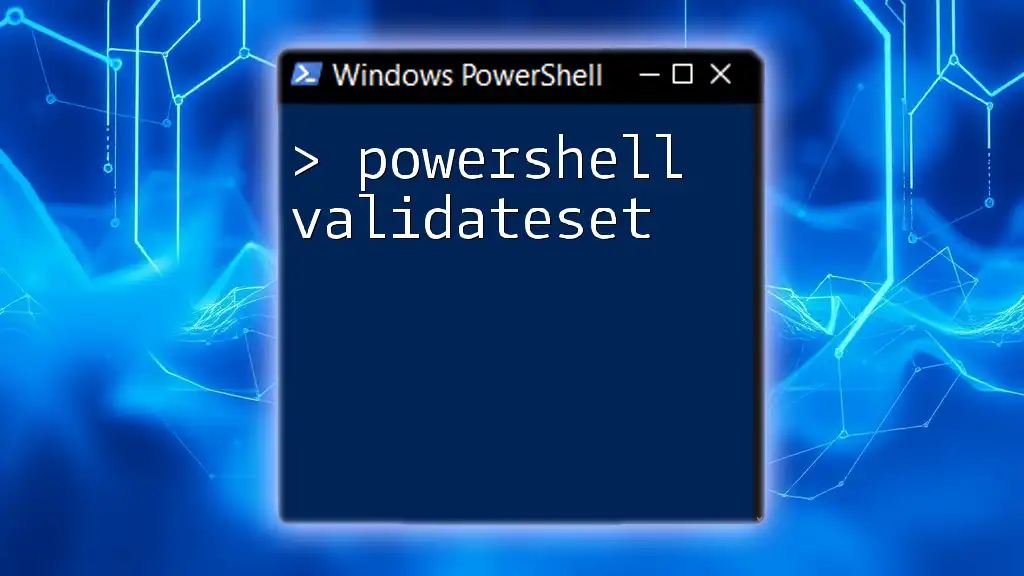
Creating New Lines in PowerShell
Introduction to New Line Character
The new line character in PowerShell is represented by `\n`. Unlike the carriage return, which merely brings the cursor back to the start of the same line, the new line character appends content below the current line. This distinction is critical when formatting output for readability.
Using New Line in PowerShell
To create a new line in PowerShell, you can insert the new line character directly into strings. This allows you to manage output formats effectively.
# Example of new line character
Write-Host "This is line one`nThis is line two"
In this instance, when the code runs, "This is line one" appears on one line, followed by "This is line two" on the next line, thanks to the new line character.
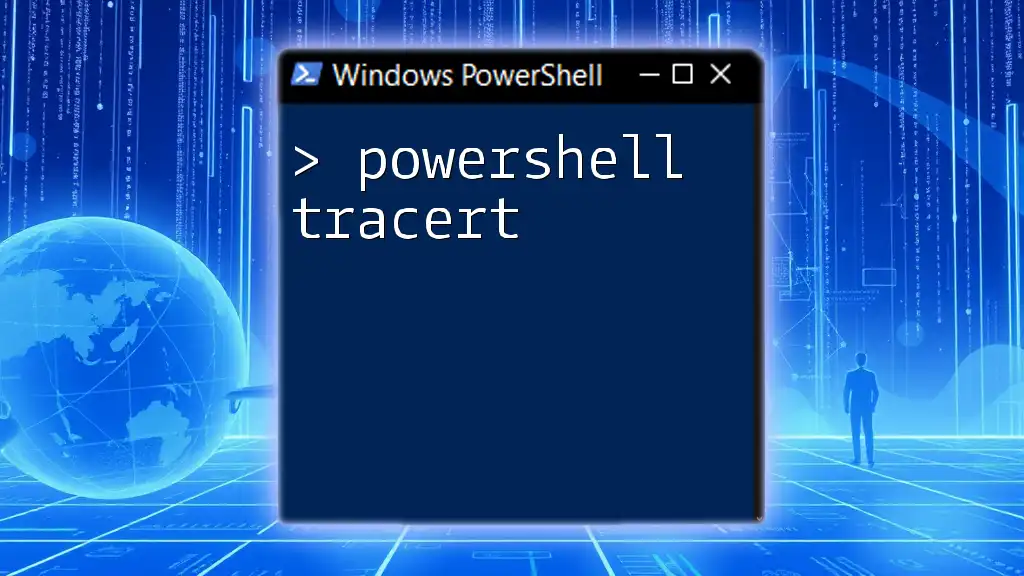
Adding New Lines to Strings in PowerShell
Using Backticks for New Line in Strings
PowerShell employs the backtick (`) as its escape character. You can use it to insert new lines within strings effortlessly, thus improving overall readability and organization.
# Example of adding new line to string using backticks
$myString = "Hello`nWorld"
Write-Host $myString
This code outputs "Hello" on the first line and "World" on the second line.
Using Double Quotes for New Line
In addition to backticks, double quotes allow you to create new lines in strings without much complication. However, ensure you handle quotes correctly, especially when strings contain quotes.
# Example of adding new line with double quotes
$myString = "Hello`n`nWorld" # Two new lines
Write-Host $myString
Here, you notice a double newline between "Hello" and "World", creating more space for clarity in output.
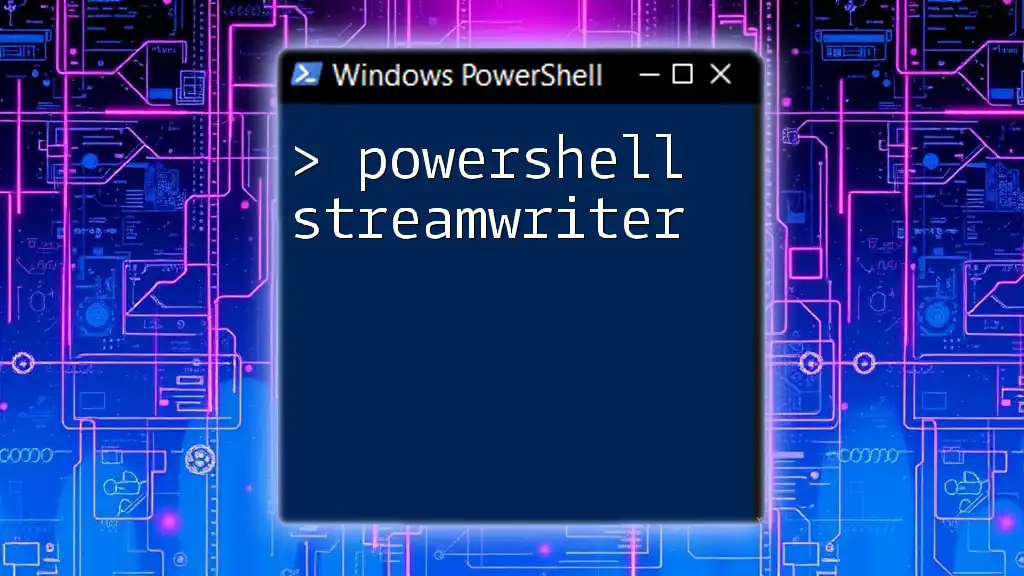
Adding Carriage Return to Strings in PowerShell
Using Carriage Return with Strings
You can incorporate a carriage return directly within strings to manage how outputs are structured. Doing this can create significant visual effects in the console.
# Example of adding carriage return to a string
$myString = "Before Carriage Return`rAfter Carriage Return"
Write-Host $myString
On execution, the text produces "After Carriage Return" because the carriage return causes the cursor to move back to the beginning of the line, effectively replacing the first segment.
Combination: Carriage Return and New Line
For the best output formatting, using both carriage returns and new lines together proves to be highly effective. This technique is particularly important when you have multiple lines of data that need structured representation.
# Example of combining carriage return and newline
$myString = "Line 1`r`nLine 2"
Write-Host $myString
When this runs, "Line 1" is replaced by "Line 2", which appears on a new line, demonstrating efficient line management using both characters.
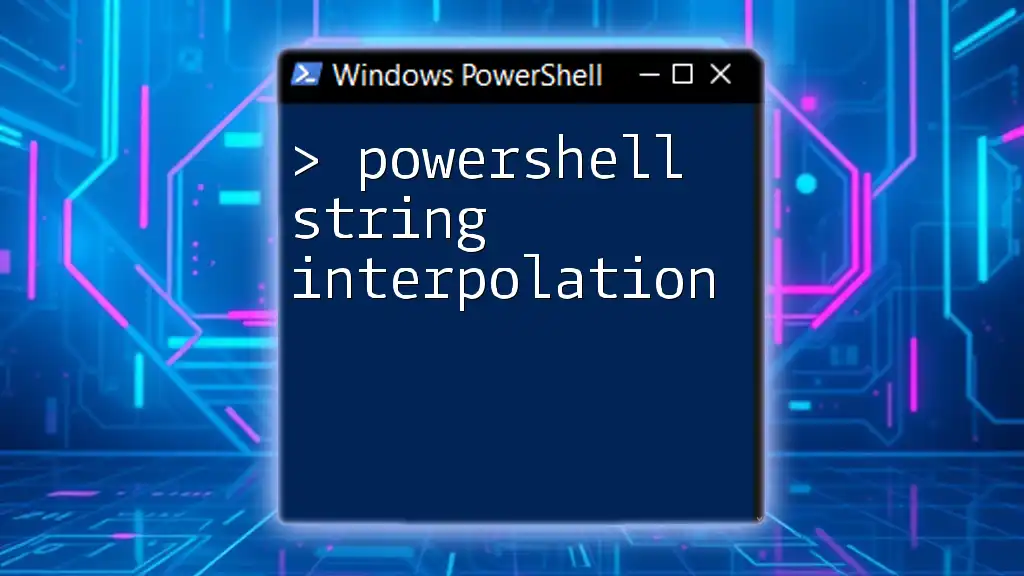
Practical Applications of Carriage Return and New Line
Logging and Output Formatting
When dealing with logs, using carriage returns and new lines can make your outputs more informative and organized. By arranging information efficiently, you can improve the readability of your logs significantly.
# Example logging with formatted output
$logMessage = "Error occurred:`r`nCheck the log files."
Write-Host $logMessage
This structure clearly separates log entries and makes it simpler for users to parse information.
Dynamically Generating Content
Generating content dynamically, such as email bodies or reports, also benefits from the use of carriage returns and new lines. It allows you to maintain a structured format, ensuring clarity in your messaging.
# Example of creating an email body
$emailBody = "Hello Team,`nThis is a report of the last execution:`r`nDetails: ..."
Write-Host $emailBody
This formatting makes sure that your email flows logically, enhancing the professional appearance of your communications.
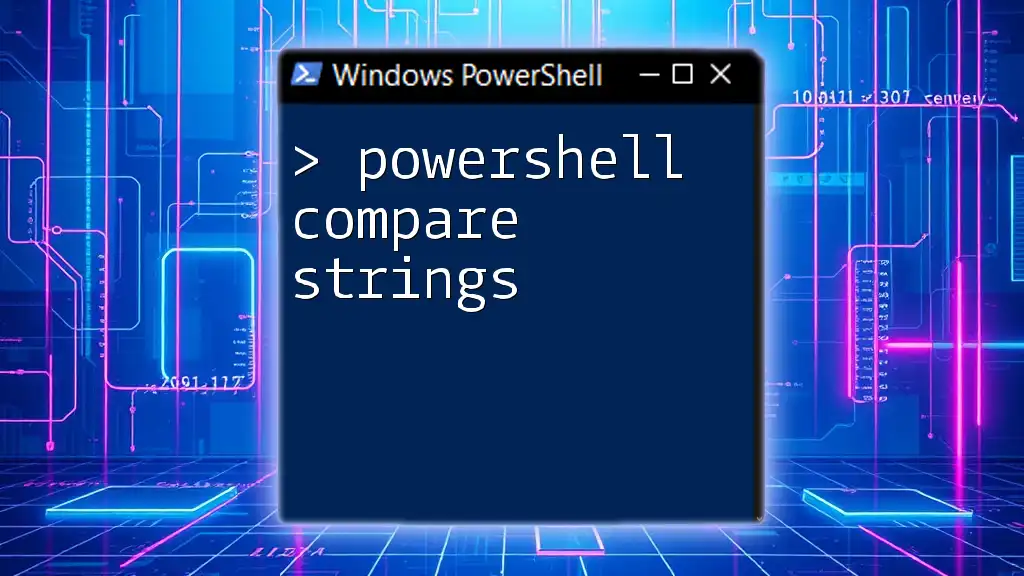
Conclusion
Summary of Key Concepts
In summary, understanding how to manipulate carriage return and new line characters in PowerShell is essential for effective scripting. By applying these concepts, you can greatly enhance the clarity and aesthetics of your outputs.
Encouragement to Practice
Let this guide encourage you to experiment with these characters in your PowerShell scripts. Learning to utilize these tools can drastically improve not only the readability but also the professionalism of your scripting endeavors.
Further Resources
To deepen your understanding of PowerShell string manipulation, consult additional resources, participate in community forums, or explore further tutorials that address real-world use cases and advanced methods. The more you practice, the more proficient you will become in using PowerShell effectively.