A PowerShell array parameter allows a function to accept multiple values of the same type, enabling users to pass in an array without needing to specify each element individually.
function Show-Array {
param (
[string[]]$Items
)
foreach ($item in $Items) {
Write-Host $item
}
}
Show-Array -Items "Apple", "Banana", "Cherry"
What is a PowerShell Array Parameter?
Understanding Parameters in PowerShell
In PowerShell, parameters are essential building blocks that define the input values for a function or script. They allow you to customize the behavior of your commands by accepting user input. Parameters can be mandatory, requiring user input, or optional, providing default behaviors if no input is given.
What is an Array?
An array is a data structure that holds multiple values, organized in a single variable. In PowerShell, arrays can store different data types, such as strings, integers, or objects. This flexibility allows for the easy management and manipulation of collections of data. You can create an array with multiple elements, and PowerShell provides various methods to access and work with these elements efficiently.
PowerShell Array Parameters Explained
A PowerShell array parameter refers specifically to a parameter that accepts an array as its input. This is particularly useful for scenarios where you need to work with collections of data, such as lists of names or sets of numbers.
The main advantage of using array parameters is that they allow you to pass multiple values into a function or script in a single command, streamlining the input process and improving the flexibility of your code. Unlike single-value parameters, which only accept one input, array parameters can expand the possibilities of your scripts by enabling operations on multiple items at once.
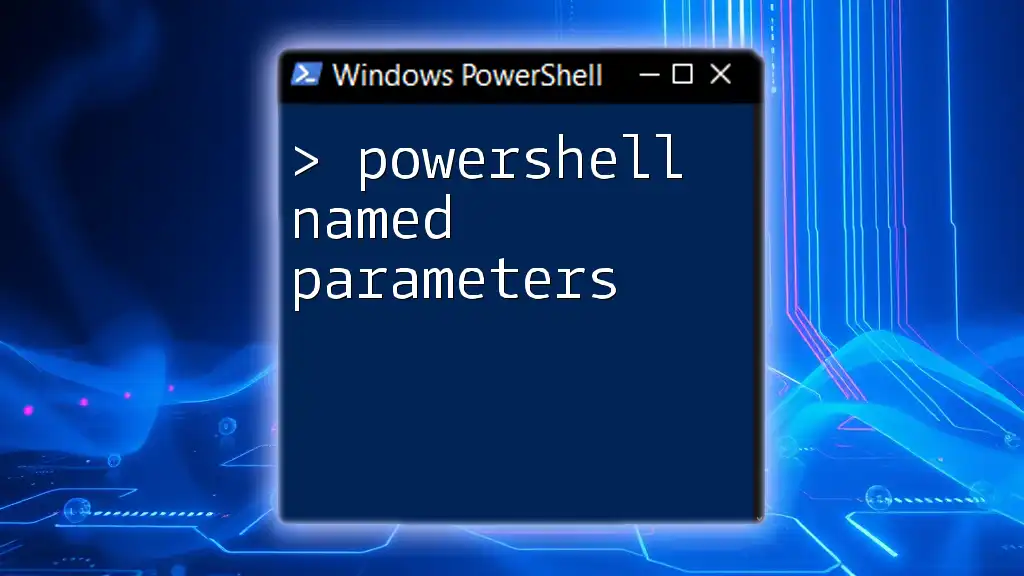
Creating an Array Parameter in PowerShell
Using Param Keyword
To create an array parameter in PowerShell, you typically employ the `param` block. This is a designated section within your function or script where you define the parameters your script will accept. Here’s how you can declare an array parameter:
param (
[string[]]$Names
)
Explanation of Syntax
In the above declaration, `[string[]]` specifies that the parameter will accept an array of strings. The `$Names` identifier is how you’ll reference this parameter within your script. The array marker (`[]`) indicates to PowerShell that multiple values can be assigned to this parameter.
Accepting Array Input
Passing multiple values into your function using an array parameter is straightforward. You simply separate each string with a comma when calling the function. For example, consider the following script that processes an array of names:
function Greet-Users {
param (
[string[]]$UserNames
)
foreach ($name in $UserNames) {
"Hello, $name!"
}
}
Greet-Users -UserNames "Alice", "Bob", "Charlie"
In this example, the function `Greet-Users` takes an array parameter `$UserNames`, iterates through it with a `foreach` loop, and produces a greeting for each name.
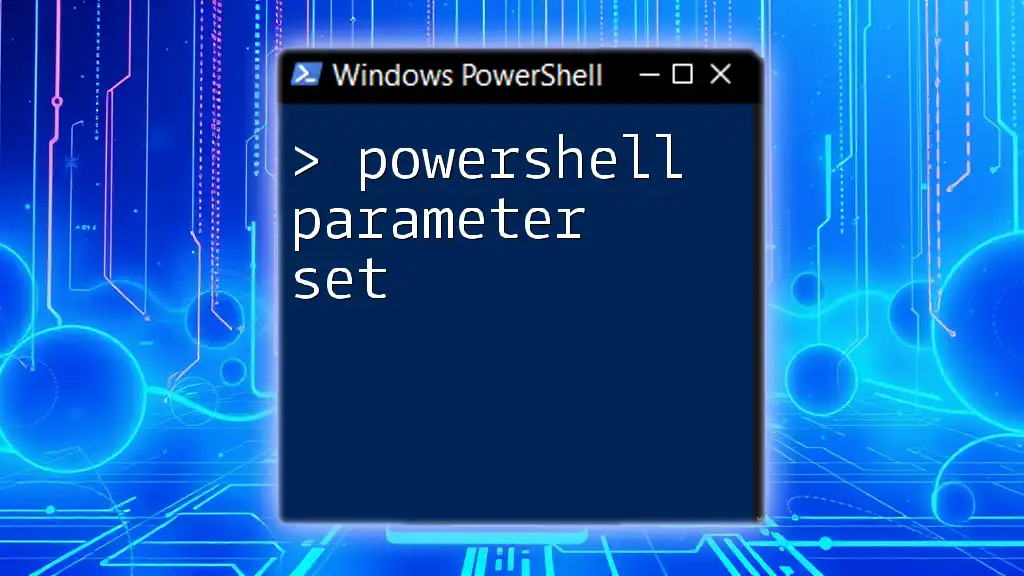
Accessing and Manipulating Array Parameters
Accessing Array Elements
Once you receive input through an array parameter, you can easily access specific elements. PowerShell uses zero-based indexing, meaning the first element of the array can be accessed with index `0`. For example:
$firstUser = $UserNames[0] # Accessing the first element
Looping Through Array Parameters
Looping through your array parameters is straightforward using the `foreach` loop. This approach allows you to perform actions on each element without needing to manage indexing manually. Here’s a code example:
foreach ($item in $UserNames) {
Write-Host $item
}
This will print each name contained in the array to the console.
Modifying Array Elements
PowerShell also enables you to modify elements within an array parameter. For example, if you want to change the second element of the array, you can easily do so:
$UserNames[1] = "Robby" # Changing Bob to Robby
After executing this modification, `$UserNames` would contain "Alice", "Robby", and "Charlie".
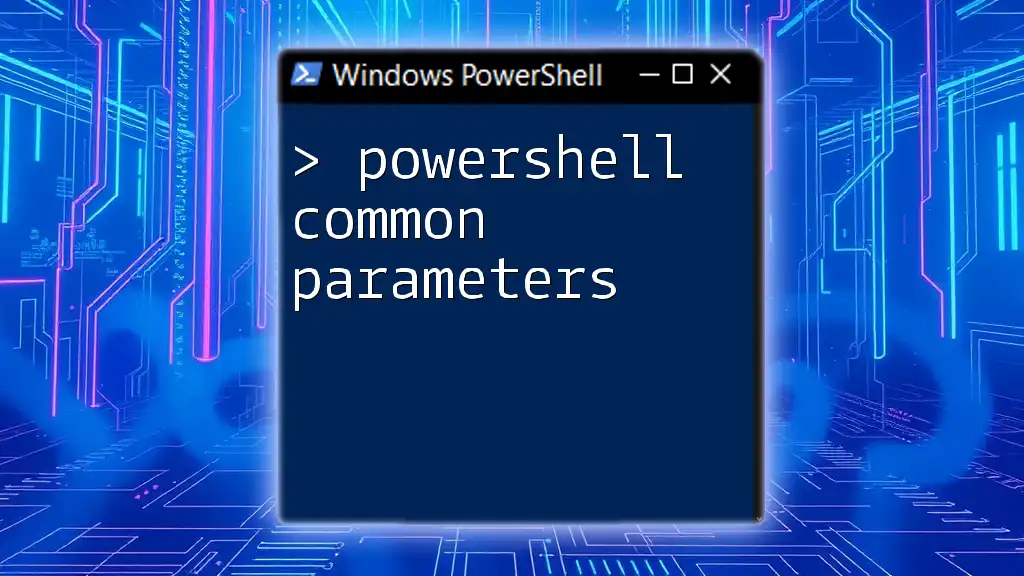
Advanced Techniques with PowerShell Array Parameters
Default Values for Array Parameters
You can define default values for an array parameter, making your scripts more user-friendly. For instance, if a user doesn't provide any input, you can set the function to return a predefined list:
param (
[string[]]$UserNames = @("Guest")
)
If no names are passed, the function will default to greeting "Guest".
Using “Param Array” Syntax
PowerShell also provides a `param array` syntax that allows for even more flexible input handling. This syntax can accept any number of parameters without explicitly specifying an array. Here's how to use it:
function Add-Numbers {
param (
[int[]]$Numbers
)
return ($Numbers | Measure-Object -Sum).Sum
}
Add-Numbers -Numbers 1, 2, 3, 4
In this function, `Add-Numbers` can accept several integer parameters and calculate their sum using the `Measure-Object` cmdlet.
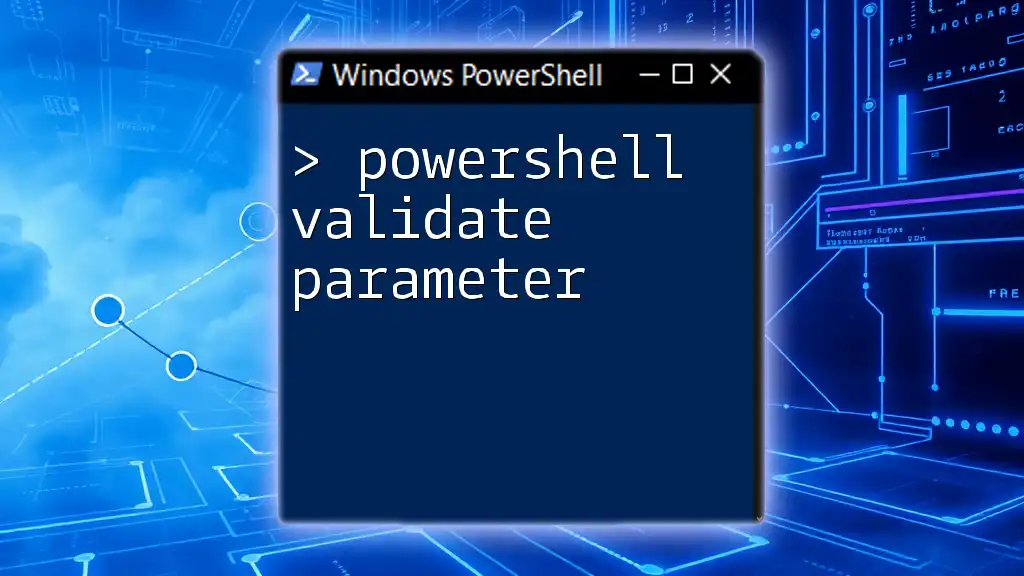
Common Use Cases for Array Parameters
Scripting Contexts
PowerShell array parameters find their utility in numerous scripting contexts. They are especially beneficial when dealing with collections, such as processing multiple files in bulk, managing user accounts, or performing batch operations on datasets.
Performance Considerations
Utilizing array parameters can lead to performance optimizations in your scripts, particularly when handling large datasets. They enable you to manage collections of information efficiently, reducing redundancy and improving execution speed.
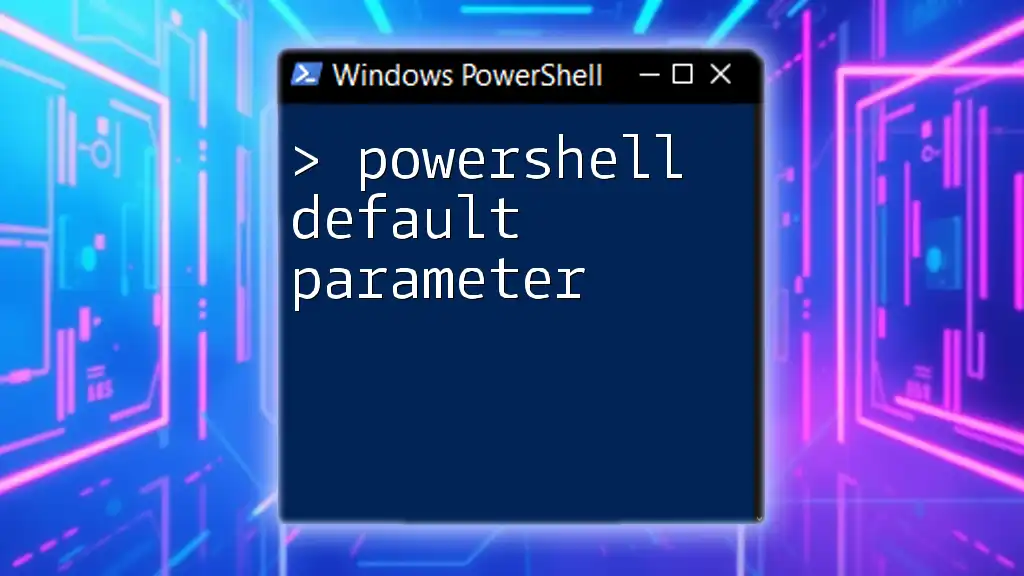
Troubleshooting Array Parameters in PowerShell
Common Errors
While working with array parameters, you may encounter common pitfalls. These include passing a single value when an array is expected or forgetting to use the array syntax. Make sure you always pass values in an array-friendly format.
Debugging Techniques
To debug array parameters effectively, utilize PowerShell's built-in debugging tools. Techniques such as using the `Write-Debug` cmdlet can provide insight into how your array parameters are being executed within your script. Monitoring the output at various stages will help you understand the behavior of your arrays and identify any issues.
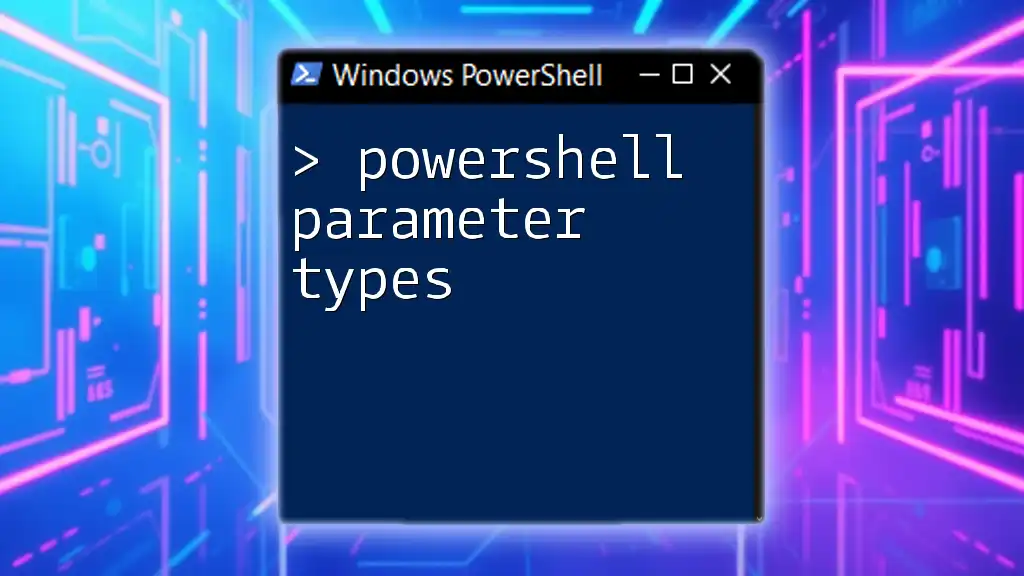
Conclusion
Understanding and effectively utilizing PowerShell array parameters opens new doors for automation and scripting. They simplify the handling of multiple data inputs, enhance the flexibility of your functions, and contribute to the overall efficiency of your scripts. As you grow more comfortable, experiment with these parameters to see how they can be integrated into your workflows.
Engage with the PowerShell community to share experiences and discover new techniques, and don't hesitate to explore further resources to enhance your understanding. Happy scripting!