In PowerShell, an optional parameter allows a function to be called without providing a value for that parameter, enabling flexibility and simplicity in command usage.
function Greet-User {
param (
[string]$Name = "Guest" # Optional parameter with a default value
)
Write-Host "Hello, $Name!"
}
# Calling the function without an argument
Greet-User # Outputs: Hello, Guest!
# Calling the function with an argument
Greet-User -Name "Alice" # Outputs: Hello, Alice!
Understanding Parameters in PowerShell
What are Parameters?
In PowerShell, parameters are a method for passing data into functions, scripts, or cmdlets. They allow users to customize the behavior of a function by providing specific input values. Parameters can be categorized as either required or optional, with required parameters needing to be supplied when the function is called, while optional parameters do not.
When a parameter is defined as optional, the script will execute even if that parameter is not included in the call. This flexibility allows for more dynamic and user-friendly scripts.
Role of Optional Parameters
Optional parameters enhance the versatility of PowerShell scripts. They allow you to create more complex functionalities without overwhelming users who may not be familiar with all options. This flexibility means that users can execute basic commands quickly while still having the option to alter their functionality as needed.
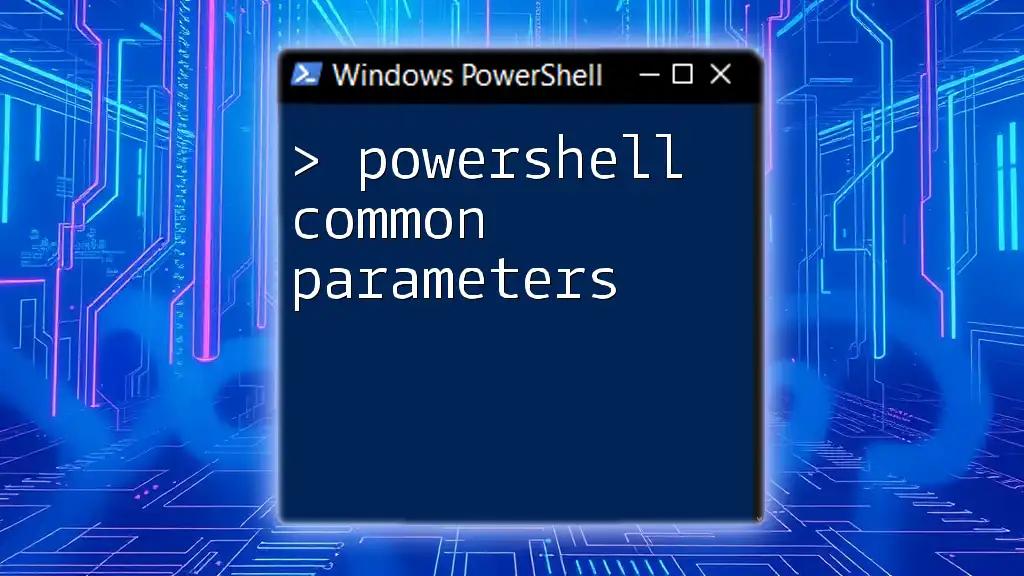
Defining Optional Parameters in PowerShell Functions
Syntax for Declaring Optional Parameters
To declare an optional parameter in a PowerShell function, you use the `param` block. The syntax for defining a parameter is straightforward:
function My-Function {
param(
[string]$RequiredParam,
[string]$OptionalParam = "DefaultValue"
)
Write-Output "Required: $RequiredParam, Optional: $OptionalParam"
}
In this example, `RequiredParam` must always be supplied, while `OptionalParam` will default to "DefaultValue" if not specified. This provides convenience while ensuring necessary input is always received.
Importance of Default Values
Default values play a critical role in making optional parameters user-friendly. They prevent errors that might occur if the user forgets to specify an optional value when calling the function.
For instance, if a user calls `My-Function -RequiredParam "Hello"`, the script would output:
Required: Hello, Optional: DefaultValue
However, if they wanted to customize the output, they could specify the optional parameter as well:
My-Function -RequiredParam "Hello" -OptionalParam "World"
This flexibility allows users to enjoy both simplicity and customization.
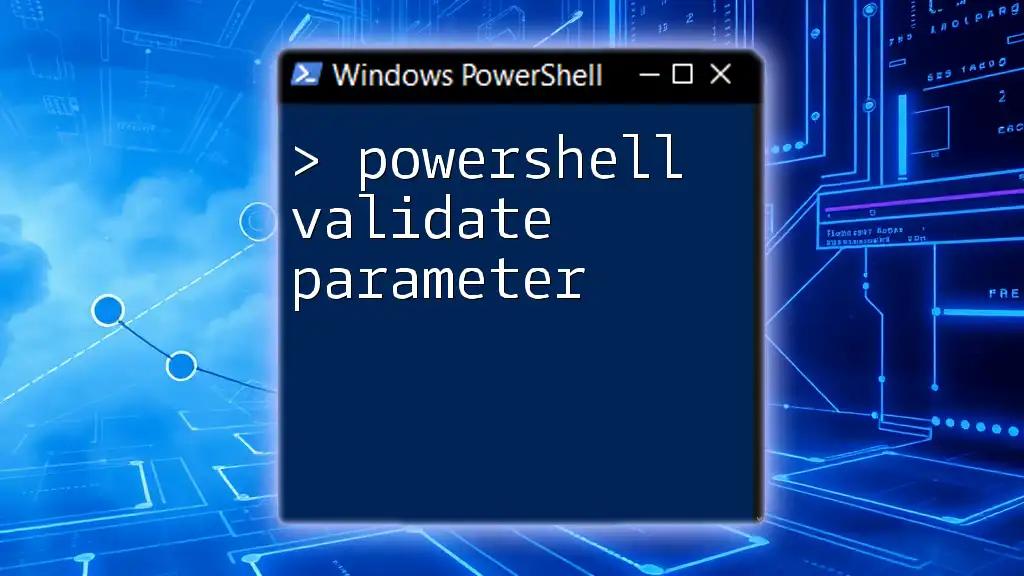
Using Optional Parameters in PowerShell Scripts
Calling a Function with Optional Parameters
One of the key advantages of optional parameters is the ability to call a function with fewer arguments than it accepts. Consider the previous example:
My-Function -RequiredParam "Hello" # Using the optional parameter's default value
My-Function -RequiredParam "Hello" -OptionalParam "World" # Specifying the optional parameter
In the first call, the output would indicate the use of the default value, while the second call showcases customization. This capability significantly simplifies the user experience.
Handling Parameter Values
When working with optional parameters, it’s essential to think about how those values are handled within your scripts. Good practices include checking for potential null values and validating types where necessary.
For example, you could improve the previous function to handle errors like this:
function My-Function {
param(
[string]$RequiredParam,
[string]$OptionalParam = "Default"
)
if (-not $RequiredParam) {
throw "Required parameter cannot be null."
}
Write-Output "Param: $RequiredParam; Optional: $OptionalParam"
}
This example ensures that the function won’t run unless the required parameter is present, increasing robustness and reliability.
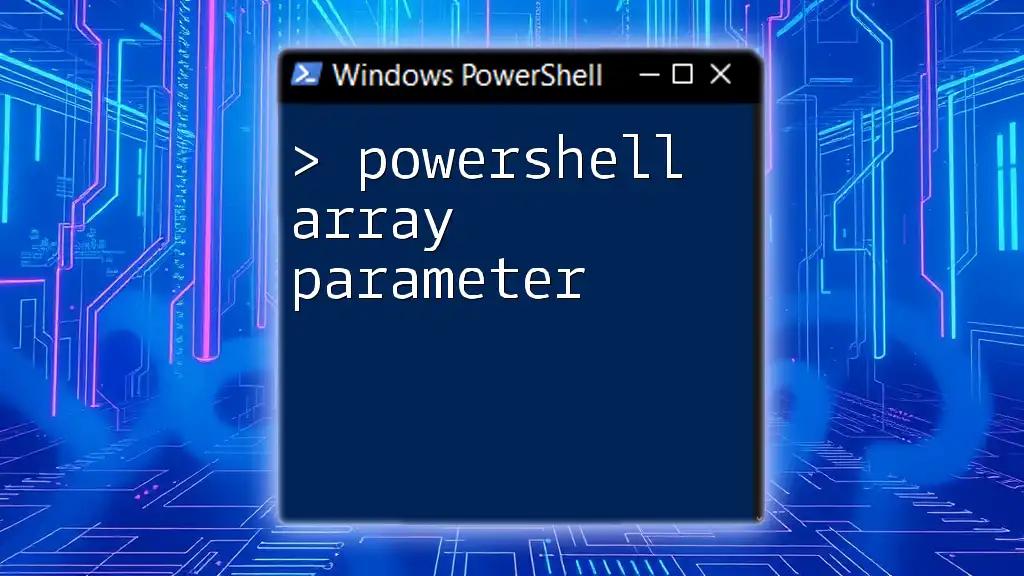
Working with Multiple Optional Parameters
Defining Multiple Optional Parameters
PowerShell allows developers to define multiple optional parameters in a function. This can enhance flexibility further, depending on the scenario:
function My-MultipleParams {
param(
[string]$Param1,
[string]$Param2 = "Optional1",
[string]$Param3 = "Optional2"
)
Write-Output "Param1: $Param1, Param2: $Param2, Param3: $Param3"
}
In this function, `Param1` is required, while `Param2` and `Param3` are optional with their own default values.
Best Practices for Using Multiple Optional Parameters
When defining multiple optional parameters, it’s vital to maintain clarity. Organizing your parameters logically helps users understand how to call your function. You should also consider the order of parameters; typically, required parameters are listed first, followed by optional ones.
For example, the following call shows how one might call `My-MultipleParams` with just the required parameter:
My-MultipleParams -Param1 "Hello"

Advanced Usage of Optional Parameters
Using Parameter Sets
For more complex scenarios, PowerShell supports parameter sets. Parameter sets define groups of parameters — allowing only the parameters from one set to be used at a time.
Here's a simple example:
function My-ComplexFunction {
[CmdletBinding()]
param(
[Parameter(Mandatory = $true, ParameterSetName = "Set1")]
[string]$ParamA,
[Parameter(Mandatory = $false, ParameterSetName = "Set1")]
[string]$ParamB = "DefaultB",
[Parameter(Mandatory = $true, ParameterSetName = "Set2")]
[string]$ParamC
)
# Function logic here
}
In this function, a user calling `My-ComplexFunction` can either use parameters from Set1 or Set2, but not both at once. This method increases flexibility while reducing potential configuration errors.
Dynamic Parameter Creation
In addition to standard optional parameters, PowerShell also allows for dynamic parameters. These are parameters added to a function at runtime based on specific criteria. Although a more advanced feature, dynamic parameters can be beneficial for creating highly adaptable scripts.
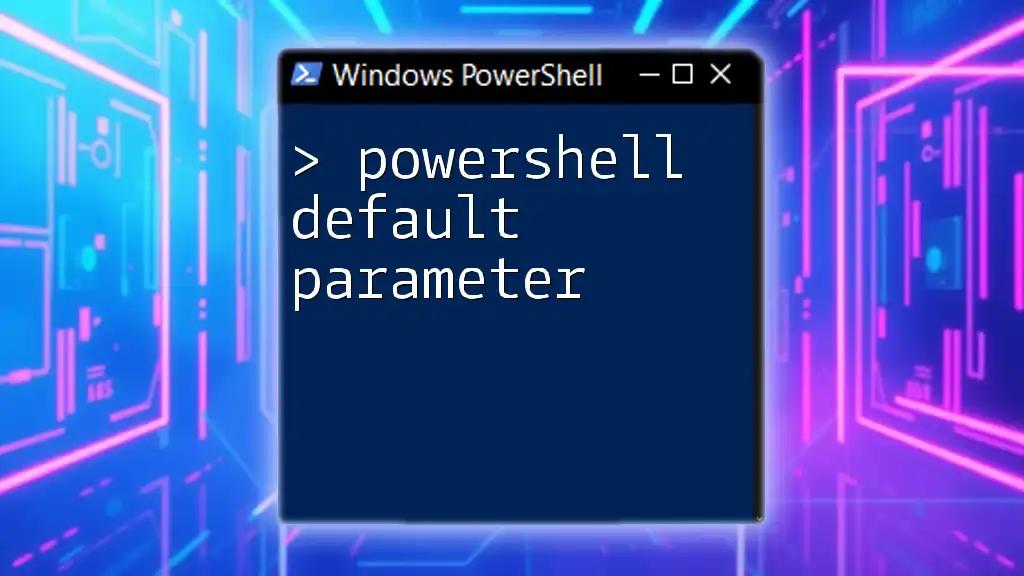
Conclusion
PowerShell optional parameters greatly enhance the functionality and usability of your scripts. By allowing functions to accept varying numbers of arguments, you can create flexible, user-friendly tools that can adapt to different scenarios.
Implementing both required and optional parameters enables powerful scripting capabilities while ensuring that you maintain clarity and control.
As you explore the world of PowerShell scripting, consider experimenting with optional parameters to deepen your understanding and improve your scripting efficiency. Happy scripting!

Additional Resources
For further learning about PowerShell optional parameters, consider checking official Microsoft documentation, community tutorials, and forums. Engaging with the PowerShell community can provide deeper insights and practical experiences that can elevate your scripting skills.