PowerShell named parameters allow you to specify parameters by their names when calling a command, making your scripts more readable and easier to maintain.
Here's an example of using named parameters in a command:
Write-Host -Message 'Hello, World!' -NoNewline $false
What are Named Parameters?
Named parameters are a powerful feature in PowerShell that enhance script clarity and usability. They allow you to specify the value for a parameter by name, rather than by position. This distinction is vital as it prevents errors and improves the readability of scripts, especially when dealing with functions or cmdlets that accept multiple parameters.
Conversely, positional parameters rely on the order in which you provide values. If the order isn't followed correctly, it can lead to unexpected behaviors or results.
Benefits of Using Named Parameters
Using named parameters in PowerShell scripting offers several key advantages:
-
Improved Readability: Scripts with named parameters are easier to understand at a glance, allowing others (and yourself in the future) to quickly grasp the purpose and function of each argument.
-
Flexibility: You can specify only the parameters you care about while skipping others. This feature is particularly useful when a cmdlet has many optional parameters.
-
Error Reduction: Named parameters help eliminate mistakes that can occur when relying solely on positional input, which can become confusing in complex scripts.
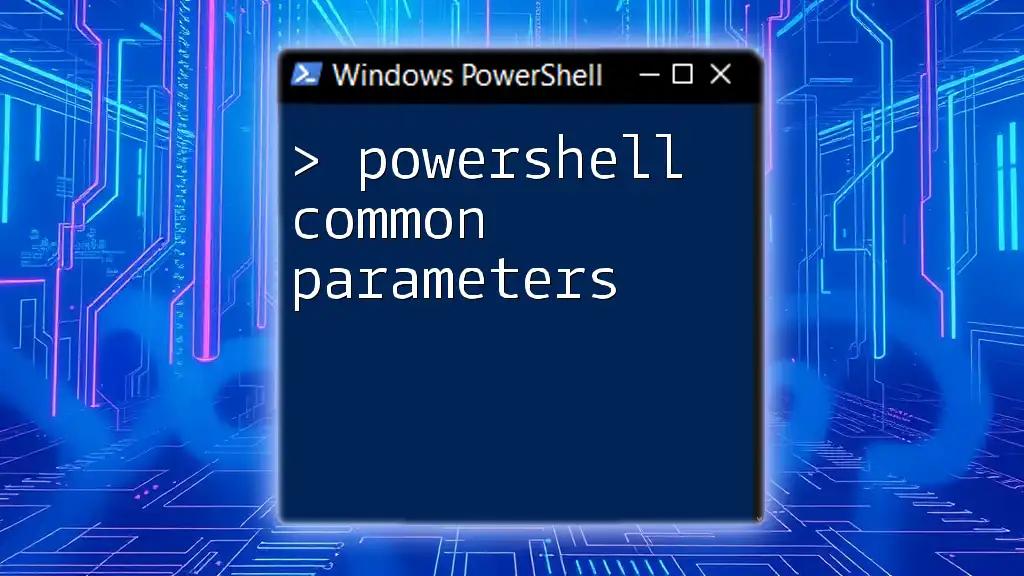
How to Use Named Parameters
Basic Syntax of Named Parameters
To utilize named parameters in PowerShell, the general syntax is as follows:
Cmdlet-Name -ParameterName Value
This simple format allows for more intuitive command construction, leading to cleaner and more maintainable scripts.
Examples of Using Named Parameters
Example 1: Using Named Parameters in Cmdlets
An excellent demonstration of named parameters can be seen with the `Get-Process` cmdlet. You can easily request information about a specific process by using its name:
Get-Process -Name "powershell"
Here, `-Name` is the named parameter, and "powershell" is the value provided for that parameter.
Example 2: Common Cmdlets with Named Parameters
Using named parameters can also be extended to other cmdlets like `Set-Location` or `Copy-Item`:
Set-Location -Path "C:\Users\Documents"
Copy-Item -Path "C:\file.txt" -Destination "C:\backup\file.txt"
These examples illustrate how easily named parameters can be applied, allowing for clear specification of where operations are directed.
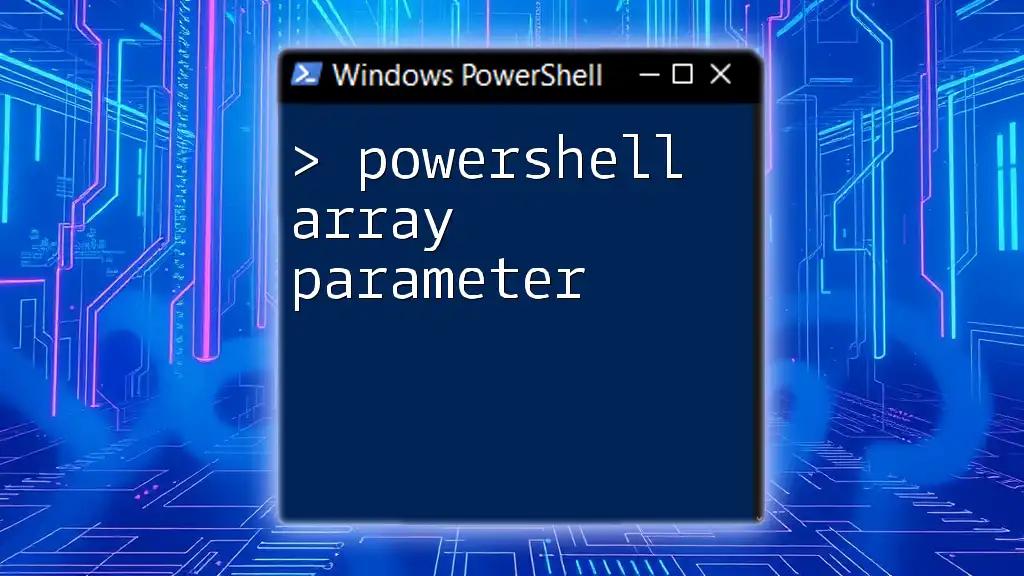
Understanding Positional vs. Named Parameters
What are Positional Parameters?
Positional parameters are those that are defined by their position in the command rather than by name. This can lead to confusion, especially when multiple parameters are involved. If the order is incorrect, you risk passing values to the wrong parameters, causing unexpected results and increasing difficulty in debugging.
Comparison: Named Parameters vs. Positional Parameters
When scripting, consider the following:
- Simplicity: Named parameters can significantly simplify the process. For instance:
Get-Process "powershell" "Notepad"
This command relies on order. If you mistakenly switch the arguments, you might inadvertently cause confusion during the script execution.
- Clarity: When using named parameters, you can explicitly specify which parameter receives which value:
Get-Process -Name "powershell" -Id 1234
This clarity comes at no cost to performance and greatly aids in code maintenance.

Advanced Uses of Named Parameters
Using Default Values with Named Parameters
Defining default values for named parameters in functions can make your scripts more robust and user-friendly.
Take the following example:
function Get-Demo {
param (
[string]$Name = "DefaultName"
)
Write-Host "Name is $Name"
}
Get-Demo
In this case, if you don't provide a name, "DefaultName" will be used. This reduces the need to specify every parameter each time, enhancing efficiency.
Validating Named Parameters
Validation attributes such as `ValidateSet` and `ValidateRange` can also be applied to named parameters, providing stricter control over input values. For instance:
function Get-Pet {
param (
[ValidateSet("Dog", "Cat", "Fish")]
[string]$Animal
)
Write-Host "You selected a $Animal"
}
Get-Pet -Animal "Dog"
Here, the function restricts options to a specified set, ensuring that only valid values are accepted, which reduces errors in parameter input.

Handling Named Parameters in Functions
Creating Functions with Named Parameters
Creating functions that utilize named parameters is straightforward. By establishing the parameter with clear declarations, you enhance the function's usability:
function Send-Email {
param (
[string]$To,
[string]$Subject,
[string]$Body
)
# Function logic here
}
This style not only makes the function easier to call but also allows for improved documentation, helping future users understand how to use it effectively.
Using Named Parameters in Custom Functions
When calling the defined function, named parameters can be specified for clarity:
Send-Email -To "example@example.com" -Subject "Hello" -Body "Test message."
In this case, each value is explicitly associated with its parameter, which helps minimize errors and enhances readability.

Common Mistakes with Named Parameters
Forgetting Parameter Names
One common mistake when using named parameters in PowerShell is neglecting to specify the parameter names entirely. An approach to mitigate this issue is to develop a habit of using named parameters consistently in all scripts, elevating code clarity and reducing confusion.
Mixing Positional and Named Parameters
Mixing positional and named parameters can lead to ambiguities and errors, particularly in more complex commands. To maintain clarity, it's best practice to choose one style and consistently apply it throughout your script.
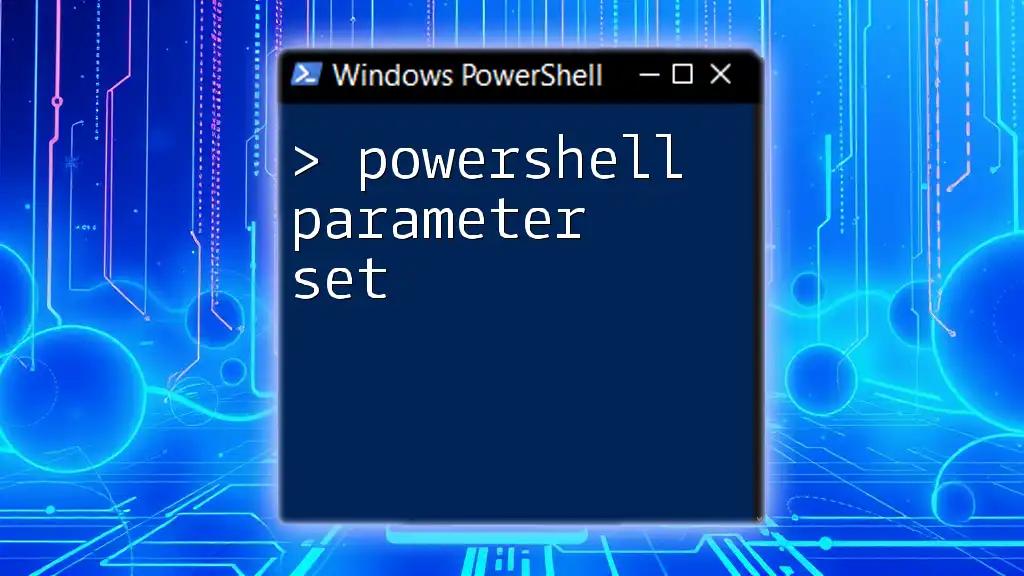
Conclusion
In summary, PowerShell named parameters are an essential tool for anyone looking to write efficient, readable, and maintainable scripts. Emphasizing named parameters helps not only in the clarity of commands but also in reducing errors that can arise from misinterpretation of positional inputs. As you continue to explore the PowerShell landscape, embracing named parameters will significantly enhance your scripting capabilities. Consider practicing with different cmdlets and functions to solidify your understanding and improve your proficiency.