In PowerShell, you can check if an array is empty by evaluating its length, which will return `0` if it contains no elements.
$array = @()
if ($array.Length -eq 0) {
Write-Host 'The array is empty.'
} else {
Write-Host 'The array contains elements.'
}
Understanding Empty Arrays
A PowerShell array is a collection of items that can store multiple values in a single variable. When discussing PowerShell array empty, it's essential to comprehend the distinction between different states of arrays.
Definition of an Empty Array
An empty array in PowerShell is an array that currently has no elements. This means its length is zero, and it contains no data. Importantly, an uninitialized array is different; it simply lacks an assignment, not necessarily indicating that it's empty.
Use Cases of Empty Arrays
Empty arrays are particularly useful in scripts where you may not yet know the values you will populate. They allow for dynamic data management, preserving clarity in your code. However, working with empty arrays also comes with challenges. For instance, trying to access or manipulate elements in an uninitialized vs. an empty array can lead to runtime errors.
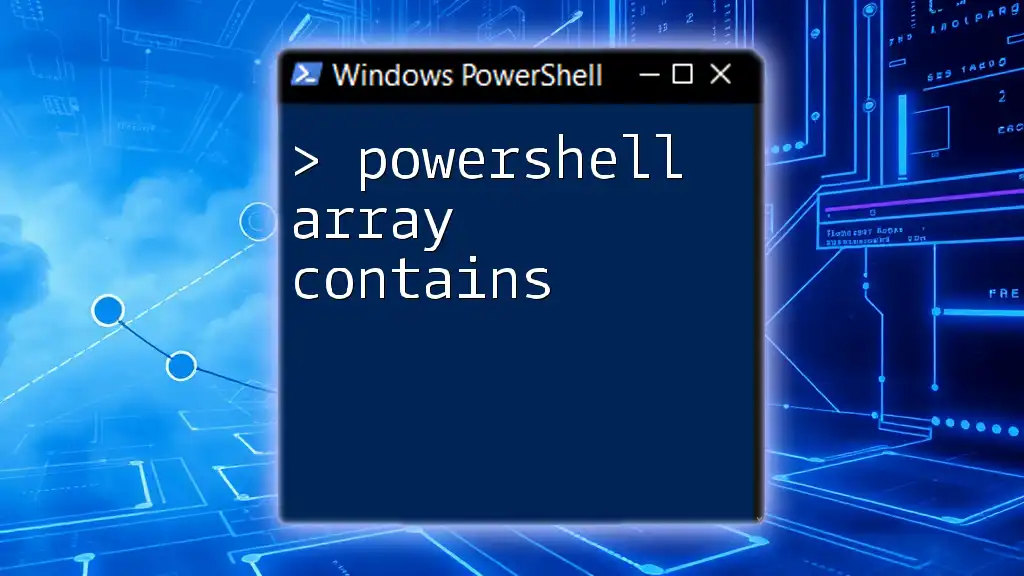
Creating Empty Arrays
Creating empty arrays in PowerShell is straightforward. There are various syntax options available, each serving its purpose depending on your needs.
How to Create an Empty Array
You can create an empty array using the following syntax:
$emptyArray = @()
This line initializes an empty array, allowing you to later add elements or manipulate it as needed.
Different Methods to Initialize Empty Arrays
In addition to the basic syntax, PowerShell provides alternative methods:
-
Using the array sub-expression operator: The above method is commonly used but can be expressed through more technical methods as shown below.
-
Using `New-Object`: Another effective way to define an empty array is by using `New-Object`:
$emptyArray = New-Object -TypeName System.Object[] -ArgumentList 0
This explicitly creates an array object, ensuring clarity in your script.
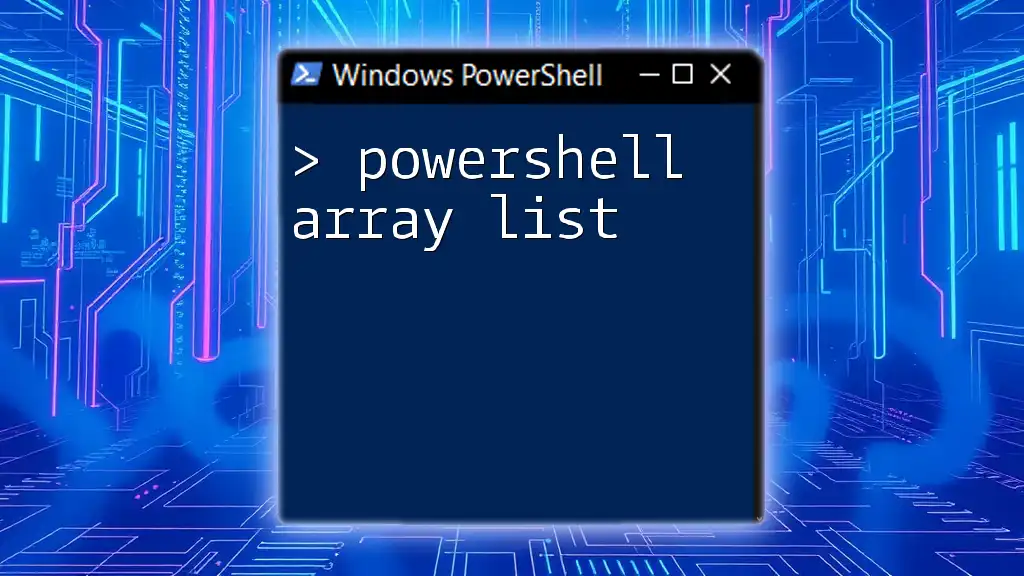
Checking if an Array is Empty
Determining if an array is empty is crucial, especially when processing data dynamically. You need to ensure that operations involving the array do not lead to errors.
How to Determine if an Array is Empty
One straightforward way to check if an array is empty is by examining its length property. Here’s an example:
if ($emptyArray.Length -eq 0) {
"Array is empty"
}
This code checks the `Length` of the array and returns a message confirming its emptiness.
Alternative Methods for Checking Emptiness
Additionally, you can check for emptiness by comparing the array to `$null` or by using conditional statements:
if (-not $emptyArray) {
"Array is empty"
}
This method leverages PowerShell's evaluation of `$null` to derive the same conclusion regarding the array's state.
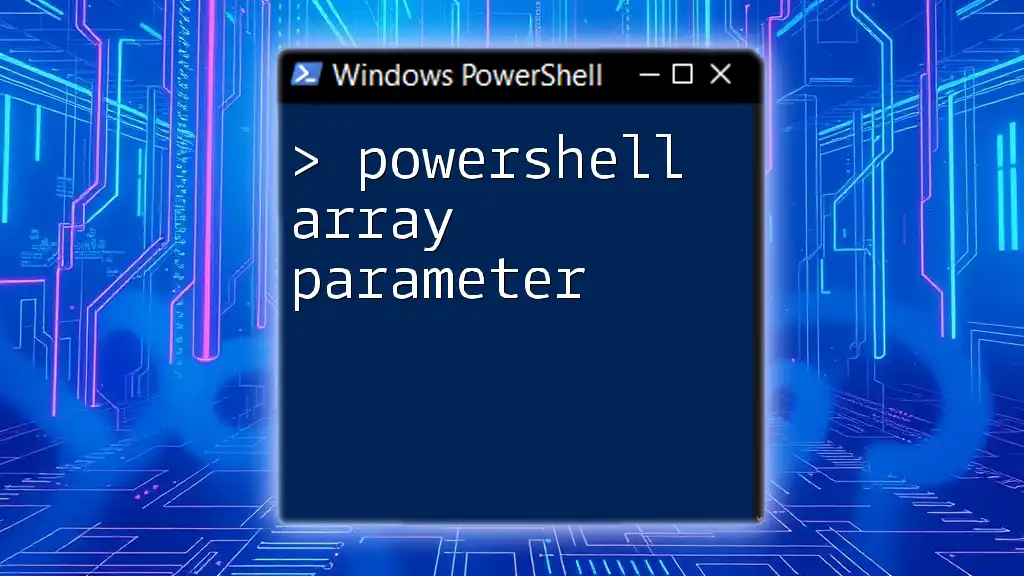
Adding Elements to an Array
Once an empty array is created, you may want to add items to it, which is a straightforward process in PowerShell.
How to Add Elements to an Empty Array
You can add elements using the `+=` operator, which appends items to the array:
$array = @()
$array += "Item 1"
$array += "Item 2"
This will result in an array containing two elements: "Item 1" and "Item 2".
Considerations When Adding Elements
While the `+=` operator is convenient, it’s important to note that it can have performance implications, particularly for larger arrays where adding elements repeatedly can be inefficient.
Alternative Methods to Expand Arrays Efficiently
For a more optimized process, especially with frequent additions, consider using `ArrayList`:
$arrayList = New-Object System.Collections.ArrayList
$arrayList.Add("Item 1") | Out-Null
An `ArrayList` allows for more efficient addition and manipulation of elements without the overhead of creating new array instances.
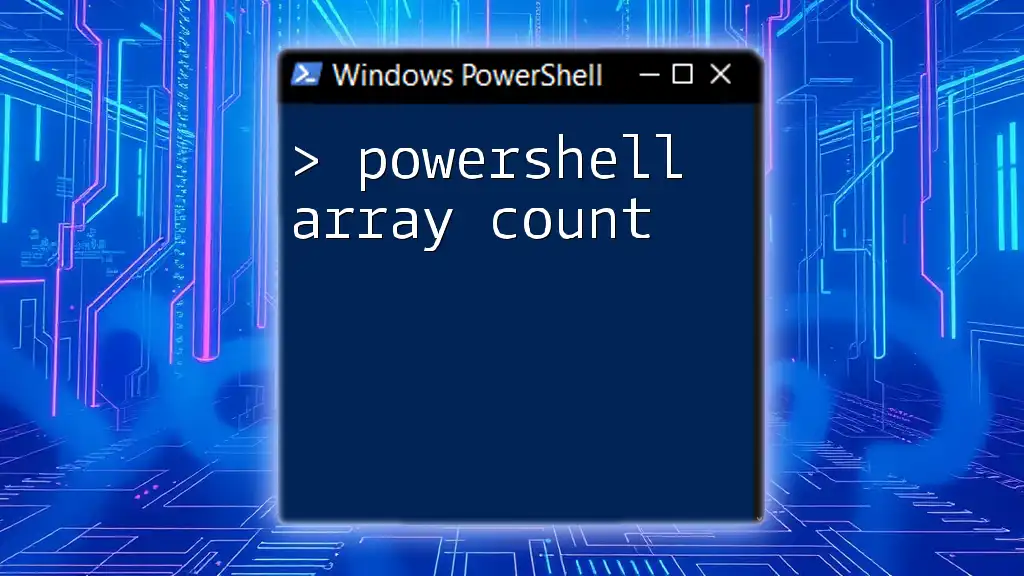
Manipulating and Using Empty Arrays
Empty arrays are versatile, and you can perform a variety of operations, even when they are empty.
Common Operations with Empty Arrays
Often, it’s necessary to process arrays, even if they contain no data. Using a `foreach` loop on an empty array won’t conflict but will simply execute its body without iterating:
foreach ($item in $emptyArray) {
"Processing $item"
}
In this case, no output occurs, as there are no items, but it’s a safe operation, avoiding any errors.
Combining Empty Arrays with Other Operations
Merging arrays can also be conveniently handled. You can merge arrays, regardless of whether one is empty:
$array1 = @()
$array2 = @("Item 1", "Item 2")
$mergedArray = $array1 + $array2
The resulting `$mergedArray` will simply contain "Item 1" and "Item 2".
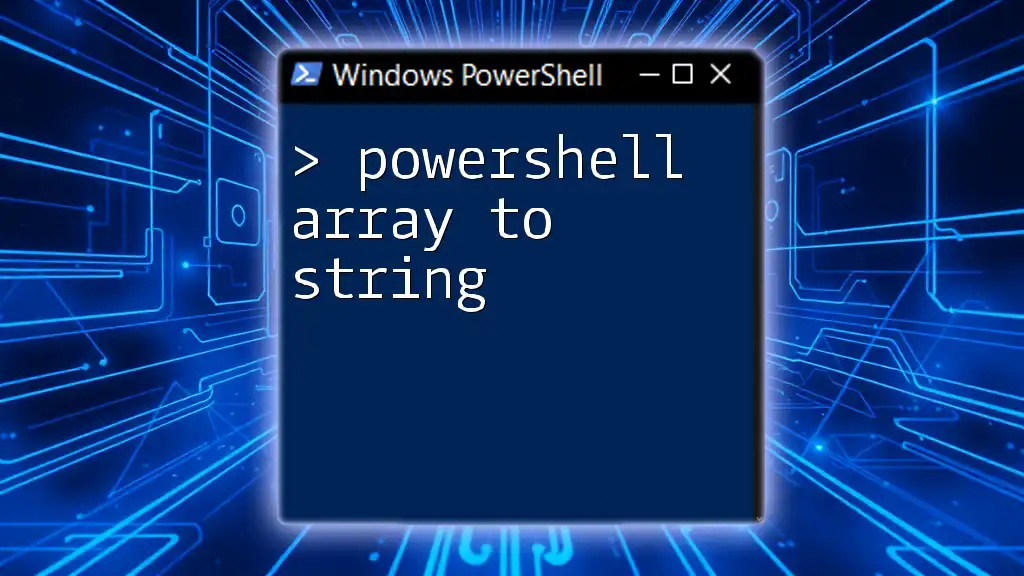
Best Practices when Working with Empty Arrays
It’s essential to adopt best practices with arrays, primarily focusing on clarity and performance.
Avoiding Common Mistakes
One common mistake is confusing null references with empty arrays. An empty array is initialized but contains no elements, whereas a `$null` reference indicates the absence of the object itself.
Performance Considerations
When working with larger datasets, consider data structures such as hash tables or lists depending on your needs. Arrays are useful for fixed data and sequential access, while other structures may enhance performance with dynamic datasets.
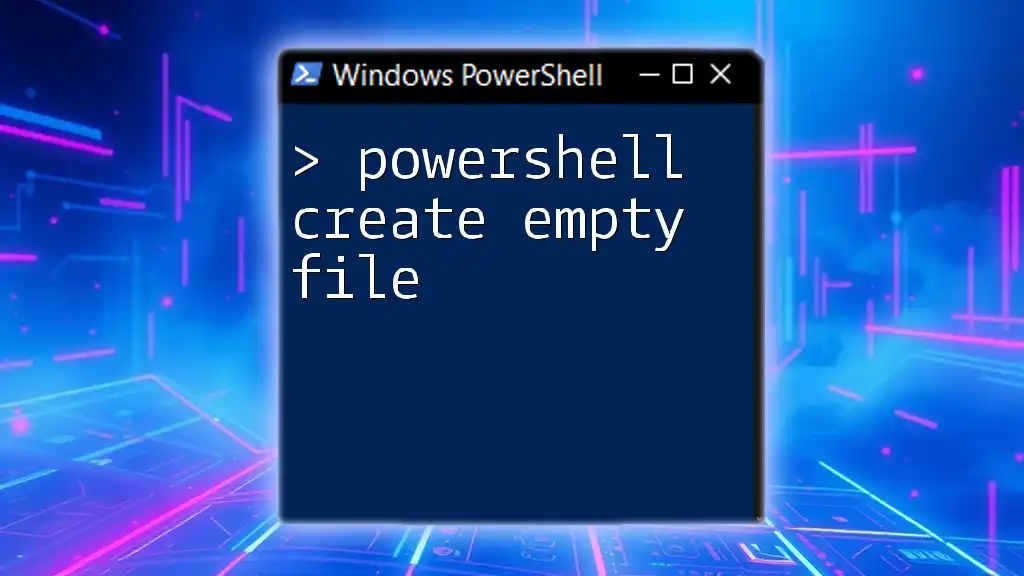
Conclusion
Understanding PowerShell array empty is integral in efficient scripting. Recognizing its uses from creation through manipulation allows for more dynamic and robust code. By mastering these concepts, you lay a foundation for enhancing your PowerShell scripting skills.
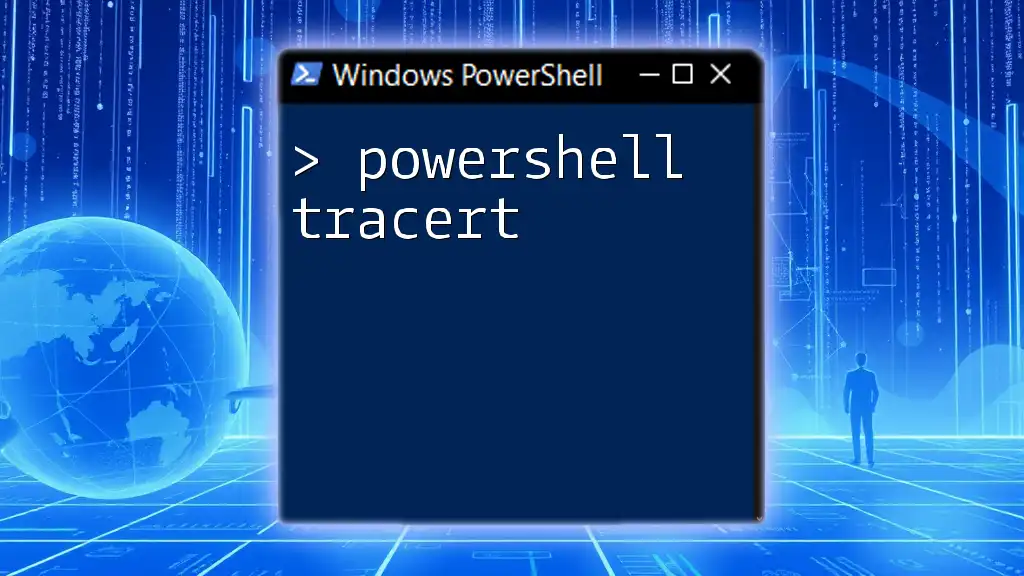
Call to Action
We encourage you to share your experiences with PowerShell arrays and how you navigate the complexities of managing empty arrays in your scripts. What challenges have you faced, and what techniques have you found helpful? Let's continue the conversation and explore the depths of PowerShell together!