To create an empty file using PowerShell, you can use the `New-Item` cmdlet as shown in the following code snippet:
New-Item -Path "C:\Path\To\Your\Directory\EmptyFile.txt" -ItemType File
Understanding Empty Files
An empty file is defined as a file that has no content and a size of zero bytes. Despite their apparent lack of utility, empty files can play a significant role in scripting and automation. They often serve as placeholders, signaling a specific state in workflows, or as a way to clear previous content without deleting the file itself.
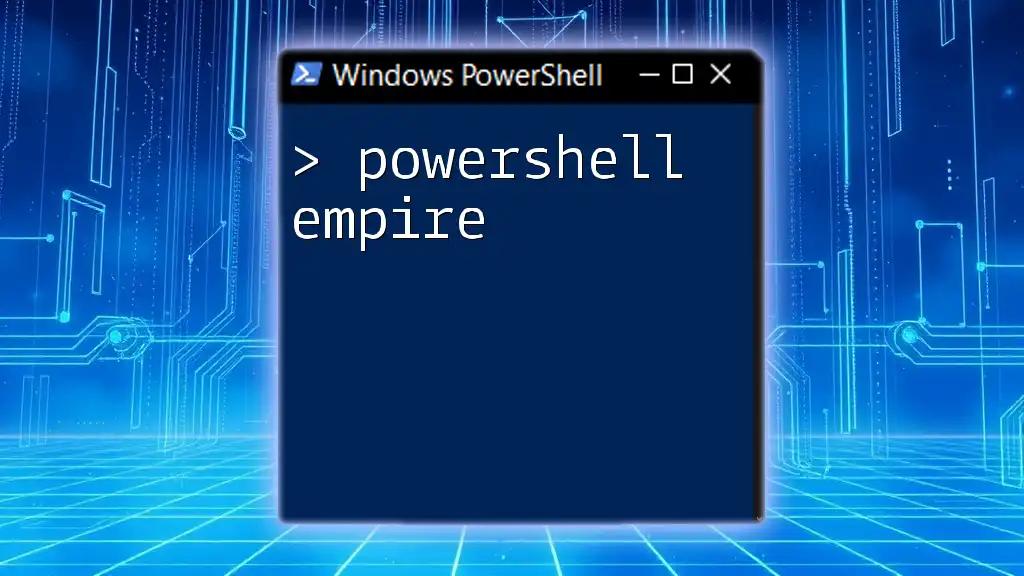
Creating an Empty File
Using `New-Item` Command
The simplest method for creating an empty file in PowerShell is with the `New-Item` cmdlet. This command allows you to specify the path where the file will be created and the file type.
Syntax:
New-Item -Path "filepath" -ItemType "File"
Example:
New-Item -Path "C:\temp\emptyfile.txt" -ItemType "File"
In this example, an empty text file named `emptyfile.txt` is created in the `C:\temp` directory. This straightforward command provides a quick and efficient way to establish an empty file when needed.
Using `Out-File` Command
Another way to create an empty file is by utilizing the `Out-File` command, which can effectively redirect output to a file. Even if there is no output to redirect, an empty string can suffice.
Syntax:
Out-File -FilePath "filepath"
Example:
"" | Out-File -FilePath "C:\temp\emptyfile2.txt"
In this code snippet, the empty string `""` is piped into `Out-File`, resulting in the creation of `emptyfile2.txt` in the `C:\temp` directory. This method is particularly useful when integrating into a script that is producing output dynamically.
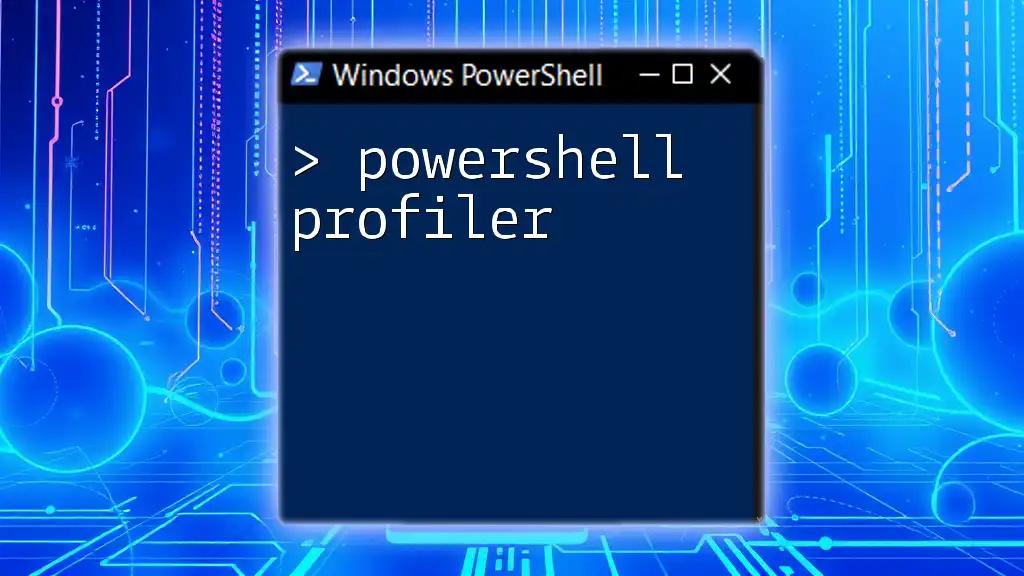
Verifying the Creation of an Empty File
Using `Test-Path` Command
Once the file is created, it's essential to verify its existence. You can accomplish this using the `Test-Path` cmdlet, which checks whether a specified path exists.
Example:
Test-Path "C:\temp\emptyfile.txt"
This command returns `True` if the file exists, allowing you to confirm successful creation.
Checking File Size with `Get-Item`
Additionally, you can determine if a file is, in fact, empty by checking its size. The `Get-Item` command retrieves the file's properties, including its length.
Example:
(Get-Item "C:\temp\emptyfile.txt").length
The output will be `0` if the file is empty. This is a great way to ensure that your operations in PowerShell are succeeding as intended.
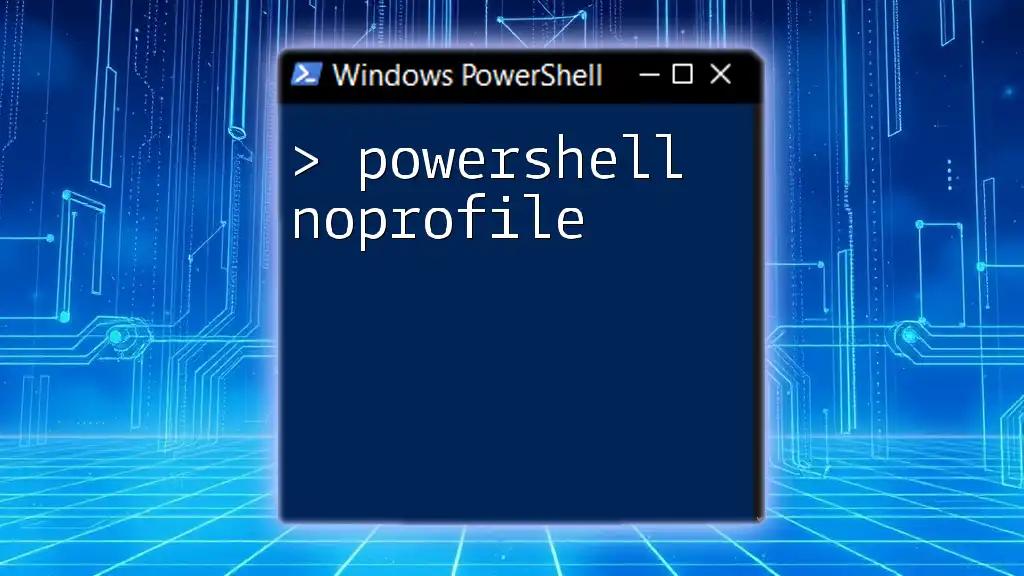
Writing to an Empty File
Appending Text to an Empty File
Once an empty file has been created, you may want to add content to it. The `Add-Content` cmdlet is designed for this purpose, allowing you to append text to an existing file.
Example:
Add-Content -Path "C:\temp\emptyfile.txt" -Value "Hello, World!"
After executing this command, the text `Hello, World!` will be added to `emptyfile.txt`. If you check the file now, it will no longer be empty, showcasing the ease with which you can modify file content.
Overwriting Text in a File
If your goal is to replace any existing content in a file (even if it’s empty), the `Set-Content` cmdlet is what you need. It will overwrite any existing data, including an empty file.
Example:
Set-Content -Path "C:\temp\emptyfile.txt" -Value "New Content"
Executing this command would completely replace any content in `emptyfile.txt` with `New Content`. It’s essential to keep in mind that this action cannot be undone, so it should be used cautiously.
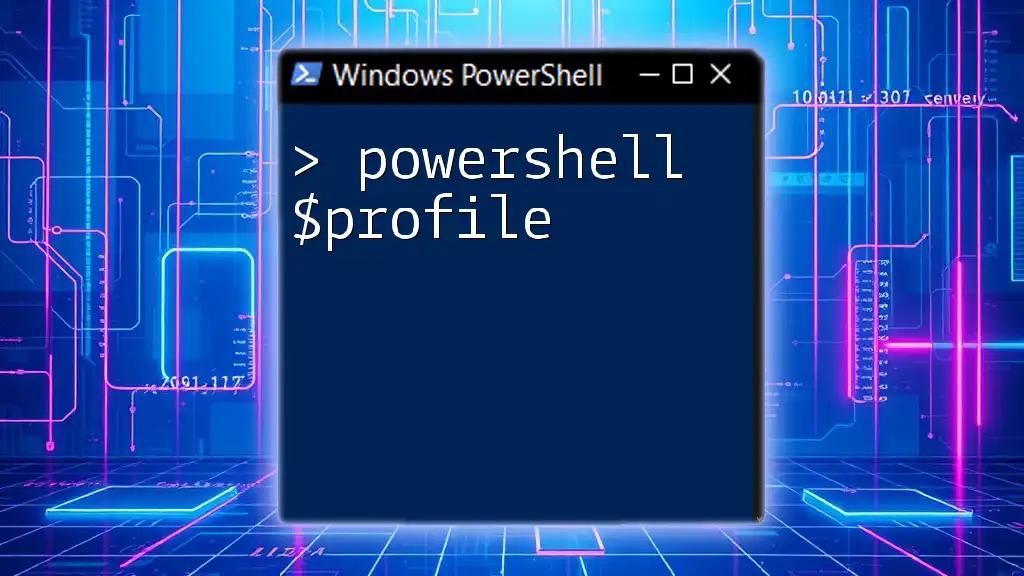
Deleting an Empty File
If you determine that you no longer need the empty file, it can be easily removed using the `Remove-Item` cmdlet.
Syntax:
Remove-Item -Path "filepath"
Example:
Remove-Item -Path "C:\temp\emptyfile.txt"
Upon executing this command, the file `emptyfile.txt` will be deleted. You can use `Test-Path` again to confirm that the file no longer exists, ensuring it has been successfully removed.
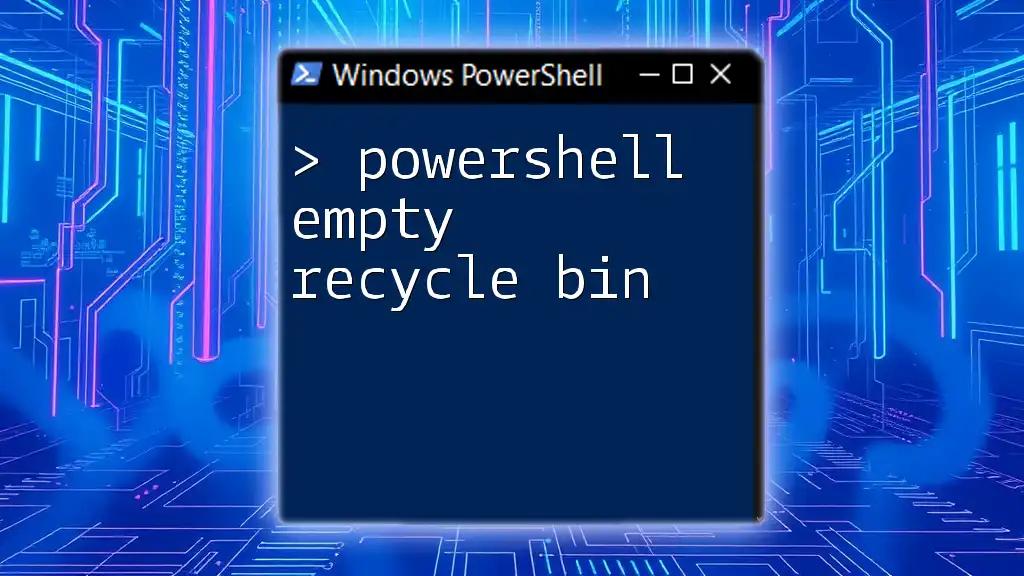
Common Pitfalls
While working with PowerShell empty files, there are several common pitfalls to be aware of:
-
Accidentally Creating Duplicate Empty Files: Always double-check the file paths and names to prevent creating multiple empty files unnecessarily. Keeping to naming conventions and using structured storage can help mitigate this.
-
Permissions Issues: It's crucial to run PowerShell with the appropriate permissions. Certain directories may require elevated permissions to create or delete files.
-
File System Limits and Performance: If you create many empty files in a directory, it may lead to performance degradation. Establish best practices, such as organizing files into dedicated folders and regular cleanup processes to ensure efficiency.
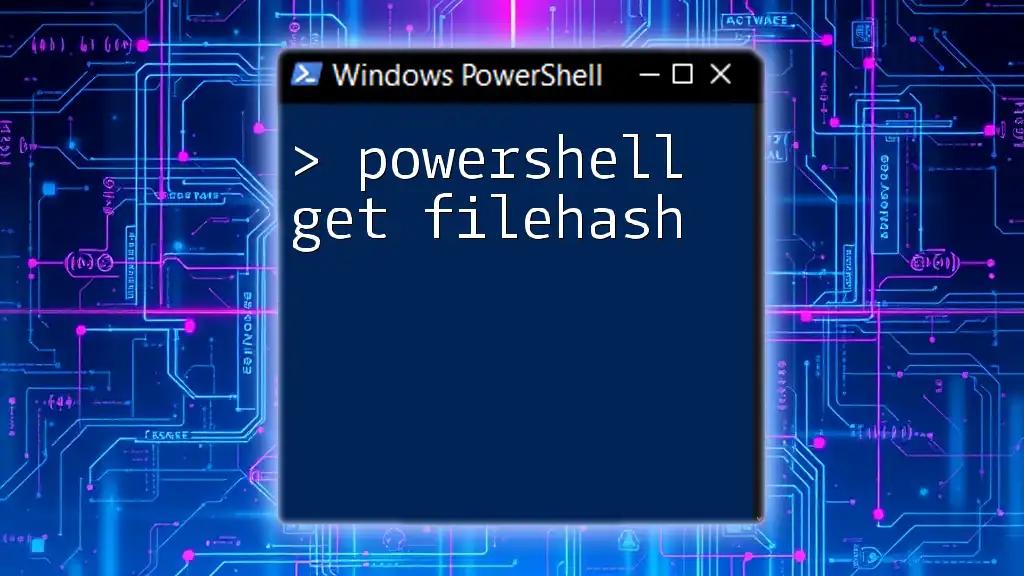
Tips and Best Practices
-
Use meaningful filenames to describe the purpose of your empty files. This makes it easier to identify their purpose later.
-
Consider storing empty files in a designated directory to avoid cluttering your main folders and to streamline maintenance.
-
Regularly clean up unnecessary empty files to keep your file system organized and efficient. Utilizing scheduled scripts to automate this cleanup can be a great practice.
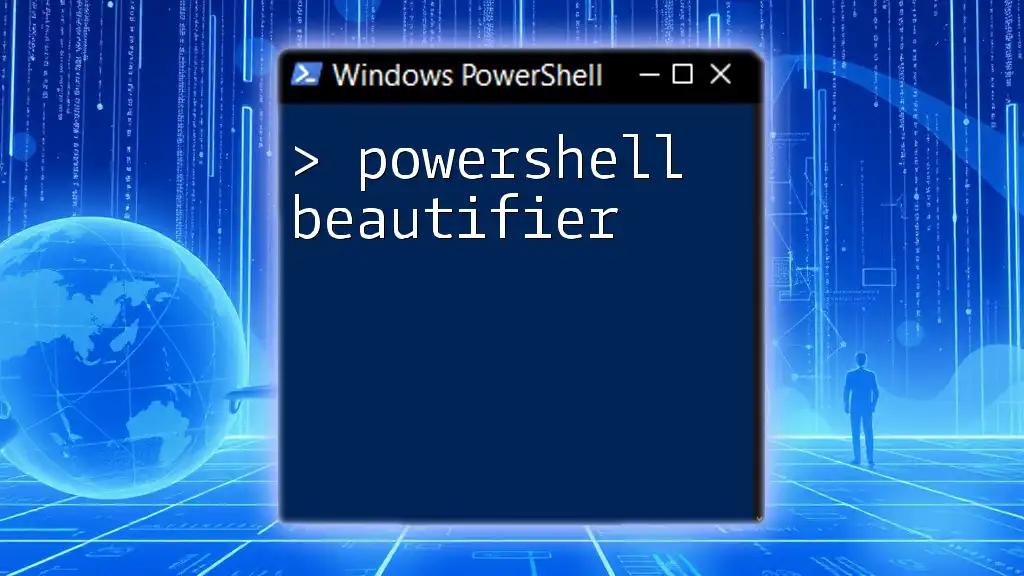
Conclusion
Working with PowerShell empty files allows for versatile file management and scripting capabilities. By mastering the commands and techniques outlined in this article, you can enhance your scripting efficiency and handle files with confidence. Experiment with these commands to develop your PowerShell expertise, and see how they can streamline your workflow.
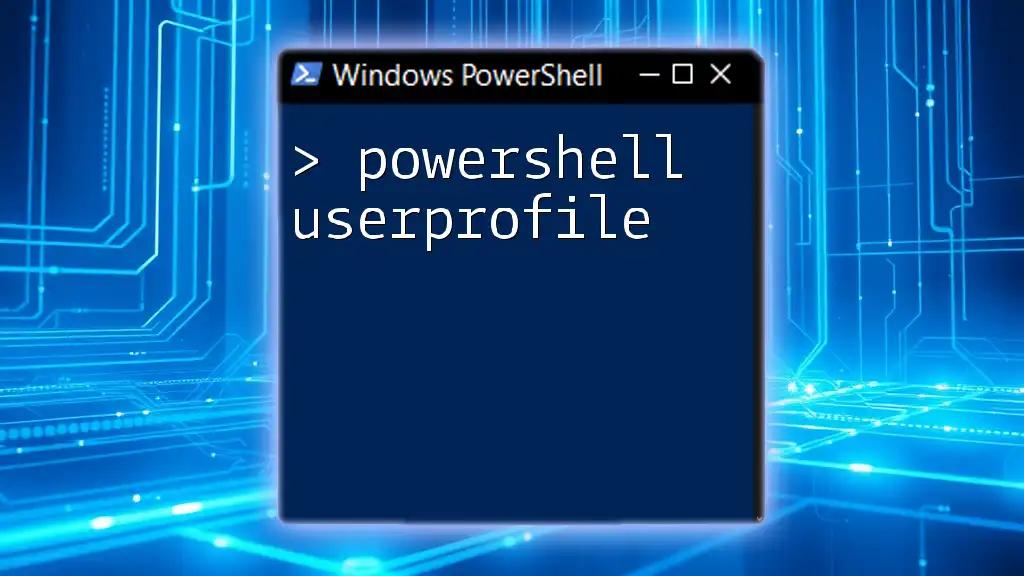
Additional Resources
For further reading, explore PowerShell documentation and online resources, and consider joining community forums to gain more insights and support as you delve deeper into PowerShell scripting.