In PowerShell, a pipeline variable allows you to pass data through the pipeline and access it later in the same command chain using the `$` notation, which can enhance the efficiency and readability of your scripts.
Here’s an example of using a pipeline variable:
Get-Process | ForEach-Object { $process = $_; Write-Host "Process Name: $($process.Name), ID: $($process.Id)" }
Understanding PowerShell Pipelines
What are PowerShell Pipelines?
PowerShell pipelines are a powerful feature that allows you to chain commands together. Instead of running a command and processing results separately, pipelines enable you to send the output of one command directly into the next. This efficient method creates a seamless flow of data, increasing productivity and simplifying tasks.
How Pipelines Work
In PowerShell, commands are executed from left to right. When using pipelines, the output of each command can be passed as input to the next command without intermediate variables. The basic syntax involves using the pipe symbol `|` to connect commands. For example:
Get-Process | Where-Object { $_.CPU -gt 100 }
This command retrieves all current processes and filters them to display only those using more than 100 CPU time.
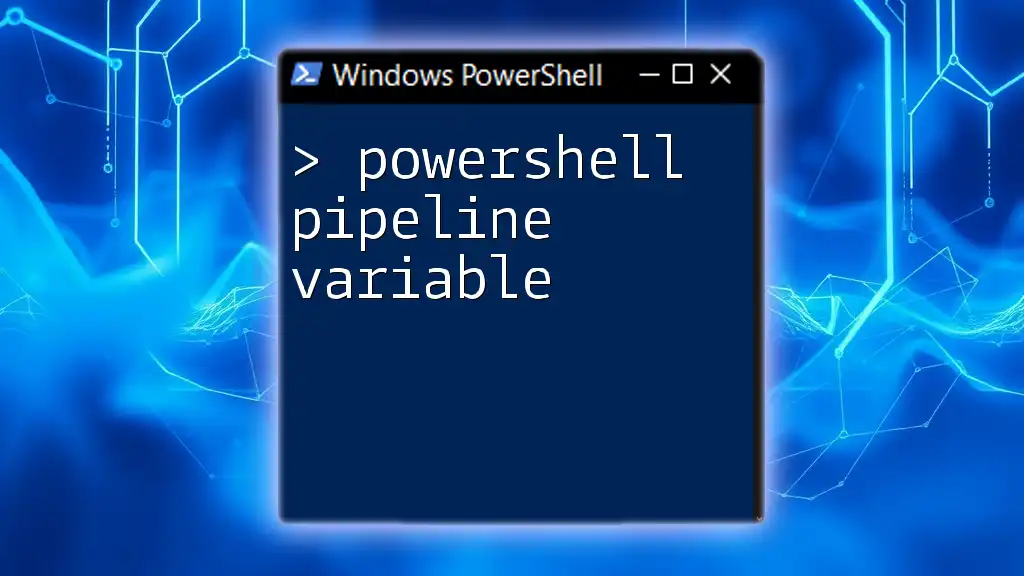
Introduction to PipelineVariable
Definition of PipelineVariable
A PowerShell `PipelineVariable` is a variable that can hold data and be shared across pipeline commands. Unlike traditional variables, which require explicit definitions and may exist outside the pipeline's context, `PipelineVariables` are designed to enhance readability and efficiency during data manipulation within a pipeline.
Benefits of Using PipelineVariable
- Enhanced Readability: Code utilizing `PipelineVariable` is often clearer and more concise, making it easier for others (or you in the future) to understand the workflow.
- Simplified Command Usage: You can effectively utilize the data flowing through the pipeline without needing to manually assign values or manage scope.
- Efficiency in Processing Data: When processing multiple commands in a sequence, `PipelineVariables` allow you to handle the output without the overhead of additional variable assignments.
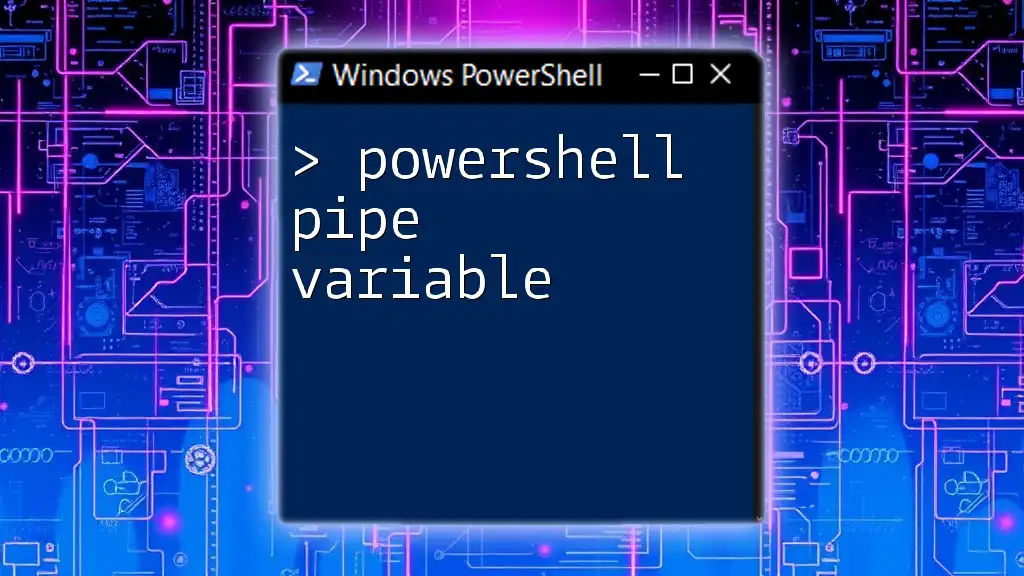
Syntax and Usage of PipelineVariable
Basic Syntax
Declaring a `PipelineVariable` in PowerShell is straightforward. You add the `-PipelineVariable` parameter to the command followed by a variable name. This variable will hold the entire result set of that command.
For example:
Get-Process -PipelineVariable myProcess
In this example, `myProcess` will hold all the process objects retrieved by `Get-Process`.
Common Use Cases
Pipeline variables can be employed in various scenarios, from loops to data filtering. Here’s an example showcasing their utility. Suppose you want to list all files in a directory that exceed 1MB in size:
Get-ChildItem -Path C:\Temp -PipelineVariable tempFiles |
Where-Object { $_.Length -gt 1MB } |
ForEach-Object { $tempFiles += $_.Name }
In this case, `tempFiles` stores the list of files while still allowing you to manipulate or display them easily.
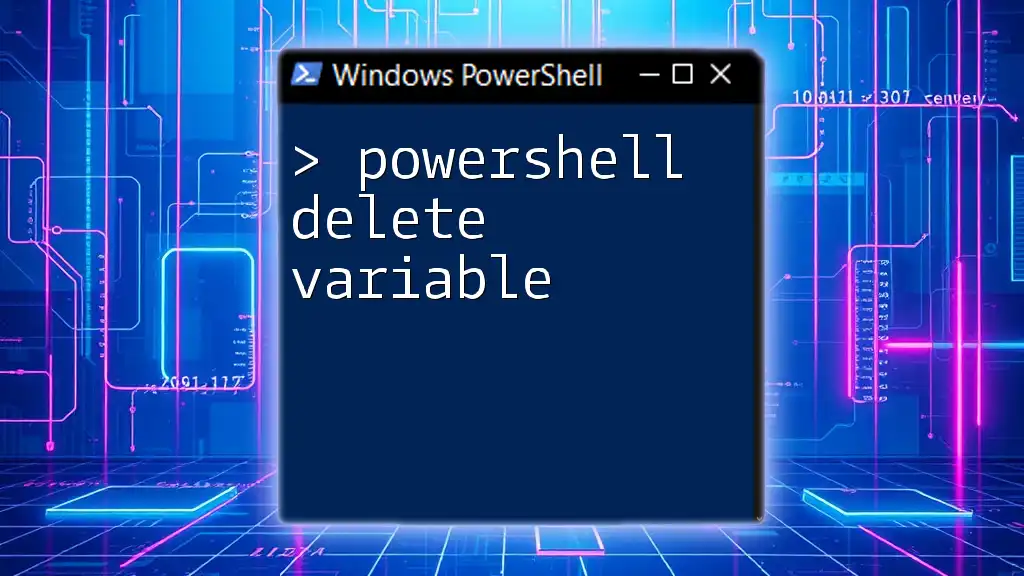
Practical Examples of PipelineVariable
Example 1: Chaining Commands with PipelineVariable
Consider a scenario where you need to display all running services. You can streamline the process significantly by leveraging `PipelineVariable`:
Get-Service -PipelineVariable svc |
Where-Object { $_.Status -eq 'Running' } |
Select-Object DisplayName, Status
This command retrieves services, filters those that are running, and then selects the display name and status, all while keeping `svc` available for further use or logging.
Example 2: Using PipelineVariable in Scripts
Imagine you want to create a script that reports the status of non-stopped services. Here’s how you can achieve this using `PipelineVariable`:
$services = Get-Service -PipelineVariable svc |
Where-Object { $_.Status -ne 'Stopped' }
foreach ($service in $services) {
Write-Host "$($service.DisplayName) is $($service.Status)"
}
In this script, `svc` serves as a temporary holder for running services, enabling monitoring without excessive variable management.
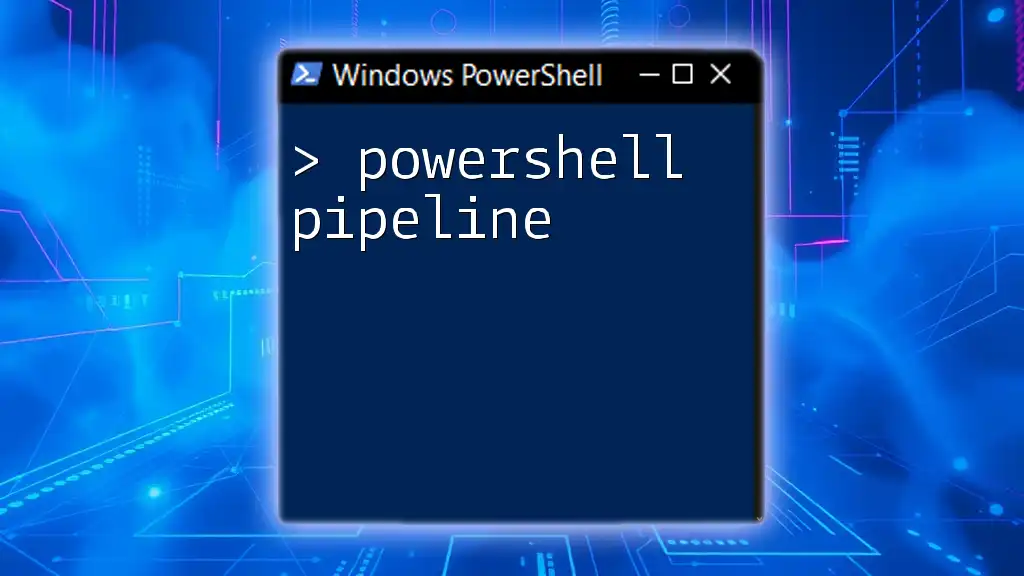
Comparing PipelineVariable with Regular Variables
Key Differences
The main distinction between `PipelineVariable` and regular variables lies in their scope and lifetime. `PipelineVariable`s exist only within the command scope and are automatically cleaned up after the command completes, promoting a more efficient resource usage pattern. In contrast, traditional variables persist in the session or script until manually cleared.
When to Use PipelineVariable vs Traditional Variables
- Choose `PipelineVariable` when working within pipelines where values need to be shared seamlessly across commands.
- Regular variables are appropriate when data needs to be accessed globally or manipulated extensively beyond a single command flow.
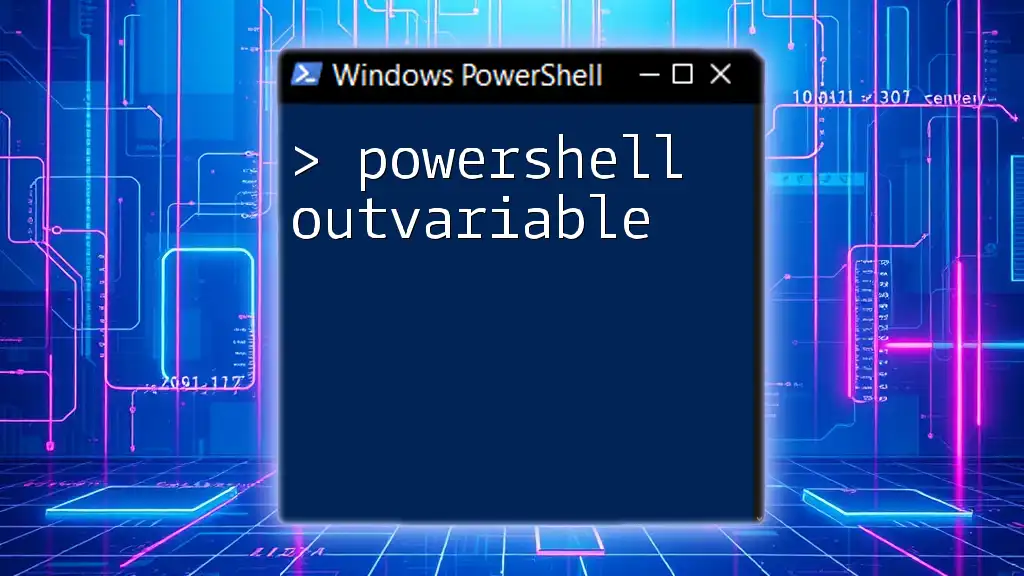
Tips and Best Practices
Best Practices for Using PipelineVariable
Adhering to certain best practices can optimize your use of `PipelineVariable`:
- Use descriptive names for your pipeline variables to clarify their purpose.
- Incorporate error handling to ensure your scripts can manage unexpected situations without failing silently.
Common Pitfalls and How to Avoid Them
While powerful, `PipelineVariable` misuse can lead to confusion:
- Ensure you understand variable scope and lifecycle; mismanaging these can lead to unexpected results.
- Always clear variables if they are no longer needed to avoid memory bloat.
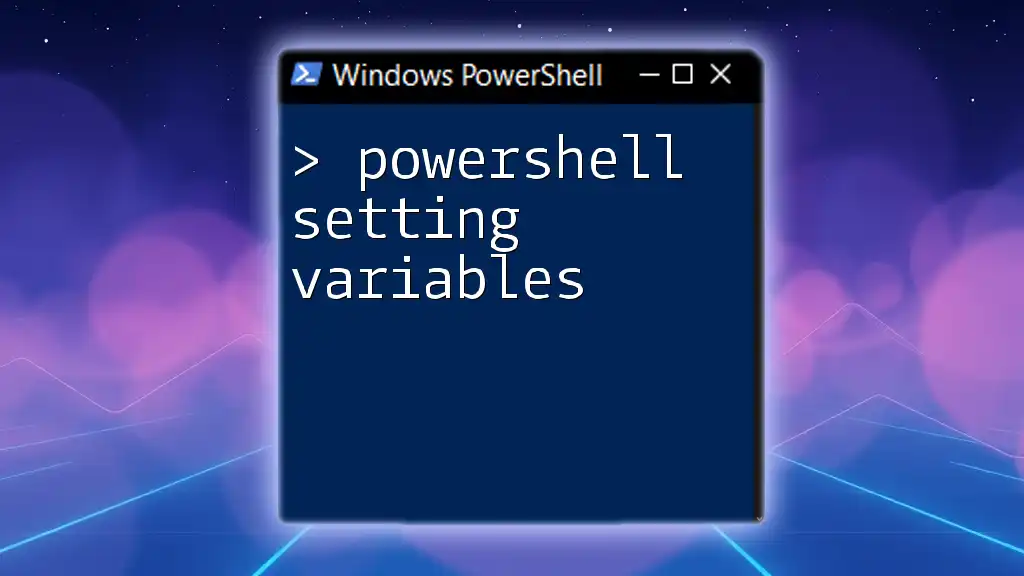
Conclusion
The `PowerShell PipelineVariable` is a potent tool that enhances scripting efficiency and readability. By utilizing `PipelineVariables`, you can streamline your PowerShell workflows, making them more effective and easier to understand. Practicing with sample commands and integrating these concepts into your daily scripting tasks will solidify your understanding and skill.
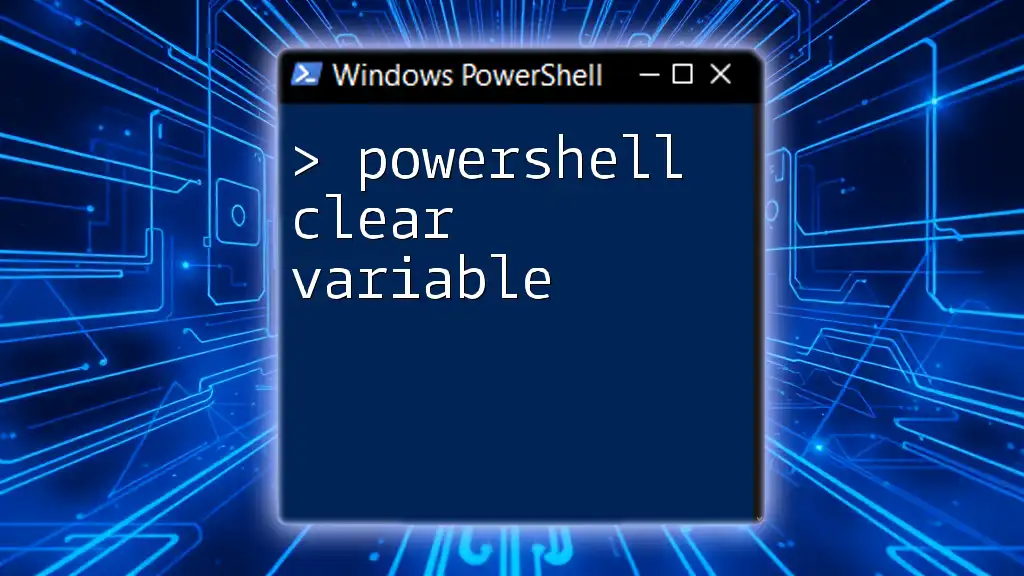
Frequently Asked Questions
-
What is the main advantage of using PipelineVariable?
The primary advantage is its ability to simplify the management of data within a pipeline, improving code readability and reducing complexity. -
Can PipelineVariables hold multiple values?
Yes, PipelineVariables can store collections of objects, allowing you to work with multiple items seamlessly. -
Are there performance differences with PipelineVariables?
In most cases, using PipelineVariables can improve performance by reducing the overhead associated with creating and managing traditional variables. However, the actual performance gain will depend on the specific use cases and complexity of the commands used.