In PowerShell, you can check if a variable is not null using an `if` statement, as demonstrated in the following code snippet:
if ($myVariable -ne $null) { Write-Host 'The variable is not null.' }
Understanding Variables in PowerShell
What is a Variable?
In PowerShell, a variable is a storage location identified by a name that holds data. Programmers can assign values to these variables, manipulate them, and retrieve their values whenever needed in scripts. This flexibility makes variables a fundamental aspect of any scripting language, including PowerShell.
Types of Variables in PowerShell
PowerShell supports various types of variables, each serving different purposes:
-
Scalar Variables: Simple variables containing a single value. Example:
$myVar = "Hello"
-
Array Variables: Variables that can store multiple values. Example:
$myArray = @("Value1", "Value2", "Value3")
-
Hash Tables: Key-value pairs, useful for organizing decisions or configurations. Example:
$myHashTable = @{ Key1 = "Value1" Key2 = "Value2" }
All these variable types can be examined for null values, which is crucial for maintaining error-free scripts.
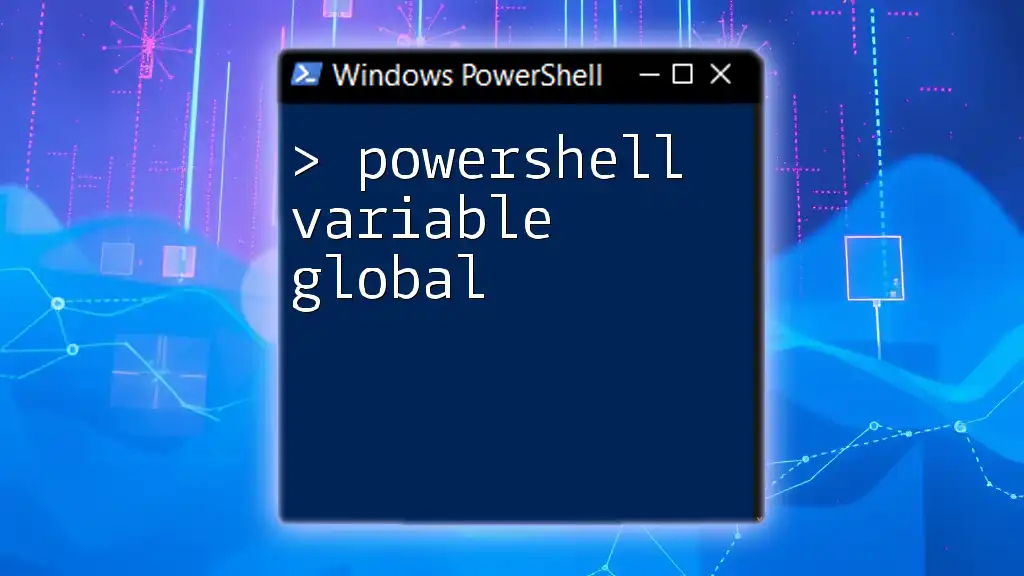
The Concept of Null in PowerShell
What Does Null Mean?
In programming, null represents an absence of value or object. In PowerShell, a null variable means it has not been assigned a value. Understanding null is vital for effective scripting, as nullable variables can lead to runtime errors if not handled properly.
Identifying Null Values
PowerShell allows you to check if a variable is null using straightforward syntax. For instance, if you simply display a variable, and it returns nothing or an error, it might be null. You can also explicitly check it using:
if ($myVar -eq $null) { "Variable is null" }
This kind of check is crucial in avoiding unexpected behaviors in your scripts.
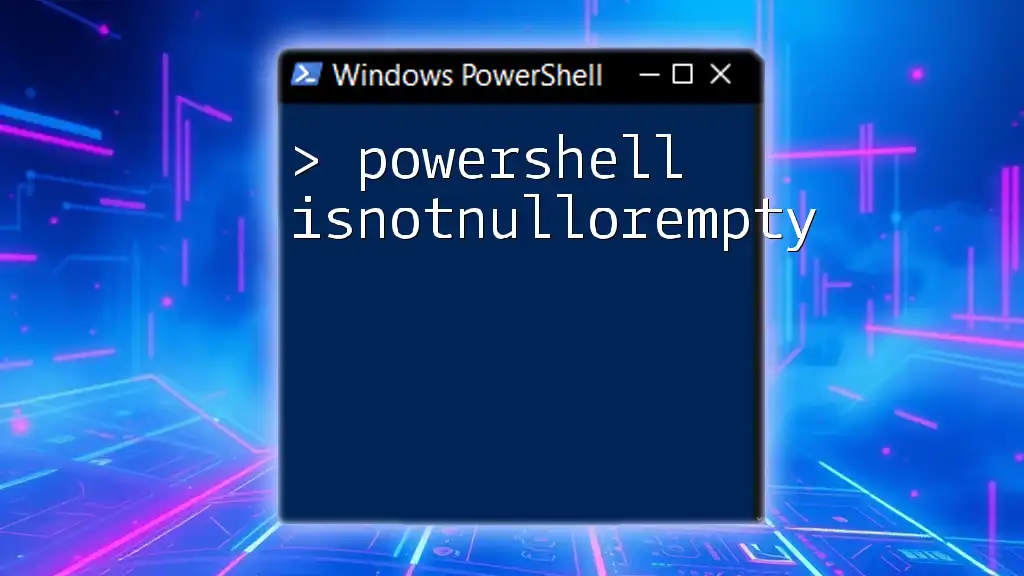
PowerShell: If Variable is Not Null
Syntax for Null Check
To determine if a variable is not null in PowerShell, you can utilize the if statement. Here’s the simple syntax:
if ($myVar -ne $null) { "Variable is not null" }
This line checks if `myVar` does not equal null and executes the subsequent code block if true.
Working with Conditional Statements
Single Variable Check
You can check a single variable against null. For example:
$myVar = "Hello"
if ($myVar -ne $null) {
Write-Output "The variable contains: $myVar"
}
In this snippet, if `$myVar` holds a non-null value, the script outputs the content of the variable.
Multiple Variable Checks
When working with multiple variables, it’s often necessary to check them collectively. Here’s how to do that:
$var1 = "Test"
$var2 = $null
if ($var1 -ne $null -and $var2 -ne $null) {
Write-Output "Both variables are not null"
} else {
Write-Output "At least one variable is null"
}
The above statement demonstrates how logical operators can help you validate several variables in a single conditional check.
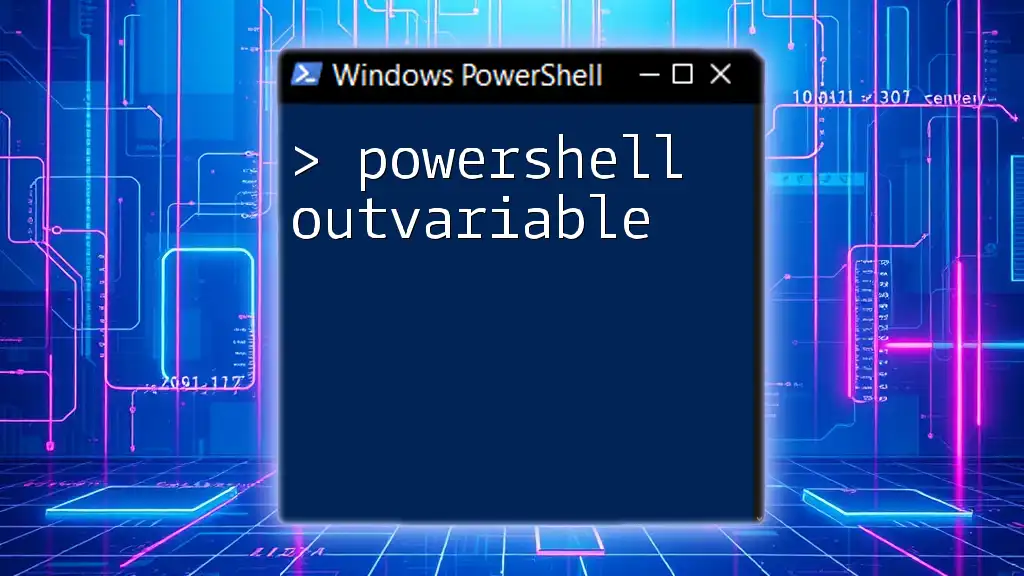
Checking for Empty Variables
Understanding Empty Values
It is crucial to distinguish between `$null` and `""` (an empty string). While null indicates an absence of value, an empty string means there is a variable assigned but contains no data. Understanding this distinction helps you avoid errors and misunderstandings.
Conditional Checking for Empty Variables
To check if a variable is empty, you can use:
$emptyVar = ""
if (-not $emptyVar) {
Write-Output "The variable is empty"
}
In this check, using `-not` helps identify if the variable has any content.
Combining Checks for Null and Empty
You can combine checks for both null and empty values for robust scripting. Consider the following example:
if ($myVar -ne $null -and $myVar -ne "") {
Write-Output "Variable is neither null nor empty."
}
This line ensures that the variable contains valid data before proceeding with further logic.
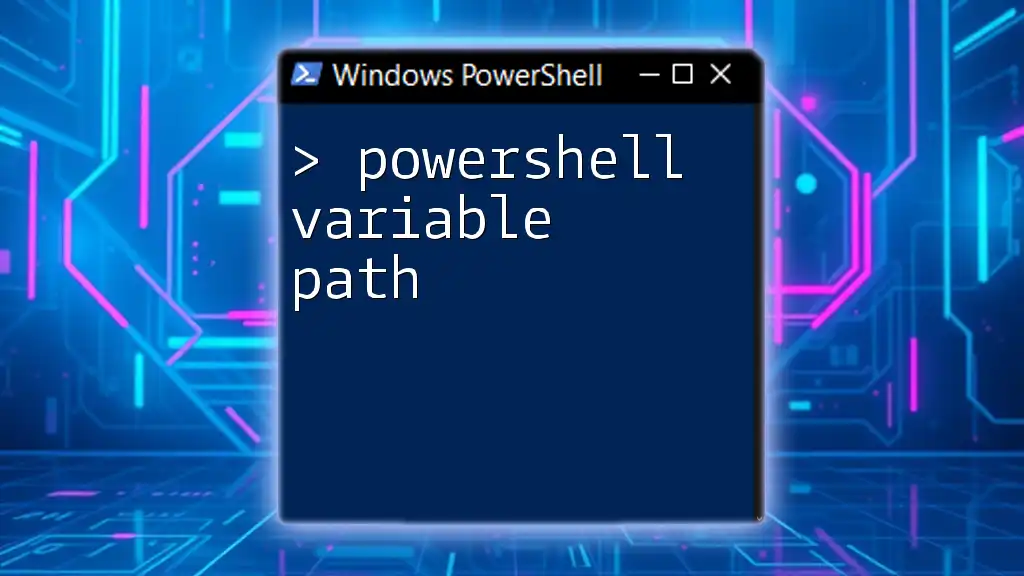
Practical Examples
Common Use Cases
User Input Validation
One of the most common scenarios in scripts is validating user inputs. For instance, when prompting for a username, you might want to ensure the user provides an actual value:
$userInput = Read-Host "Please enter your name"
if (-not $userInput) {
Write-Output "No input provided!"
} else {
Write-Output "Hello, $userInput!"
}
This example will check if the user input is non-null and not empty before proceeding.
Configuration File Checks
When loading settings from a configuration file, it’s crucial to ensure that all necessary variables are defined. If a variable is null, you can handle it gracefully within your script rather than failing unexpectedly.
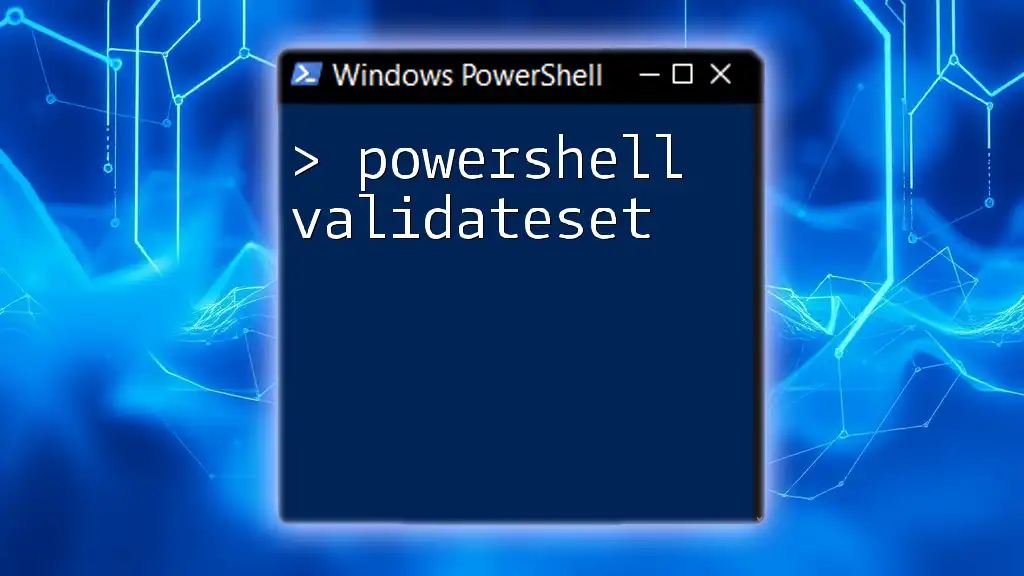
Best Practices
Using IsNullOrEmpty Method
To streamline your checks, consider using the `IsNullOrEmpty()` method. This method is cleaner and can simplify your logic:
if ([string]::IsNullOrEmpty($myVar)) {
Write-Output "Variable is either null or empty."
}
Using this method can enhance your code's readability and reduce potential errors caused by misidentifying null or empty variables.
Debugging Null-Related Errors
Debugging is a critical aspect of script writing. If you encounter issues related to null values, consider enabling debugging and verbose messaging. This approach allows you to track the flow of the script and understand where and why a variable might be null.
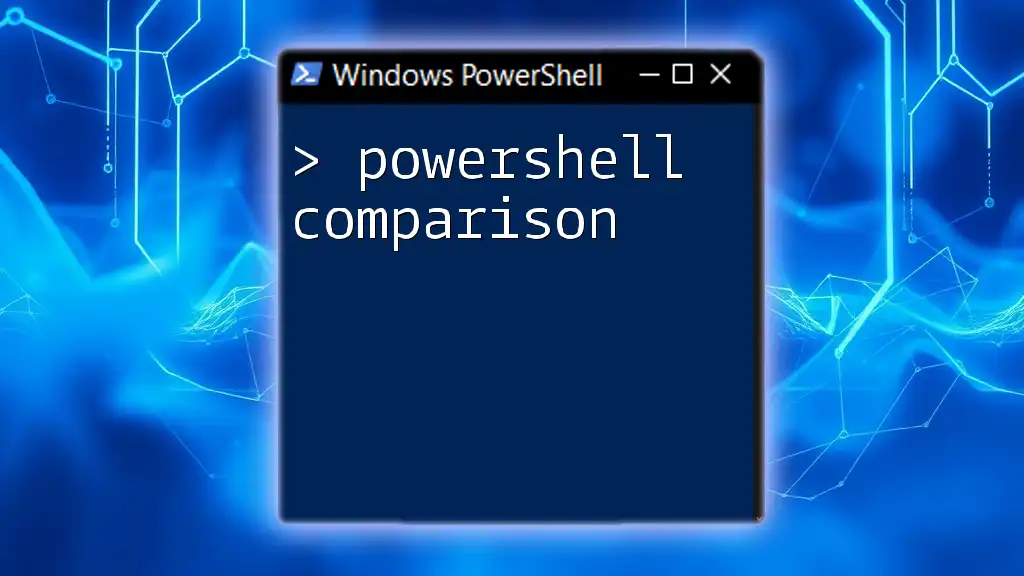
Conclusion
Validating whether variables are not null in PowerShell is a vital skill for every scriptwriter. By mastering these checks, you can enhance the robustness of your scripts, avoid unnecessary errors, and improve overall script performance. As you practice these techniques, you will become more proficient in creating reliable scripts that handle a multitude of scenarios seamlessly.
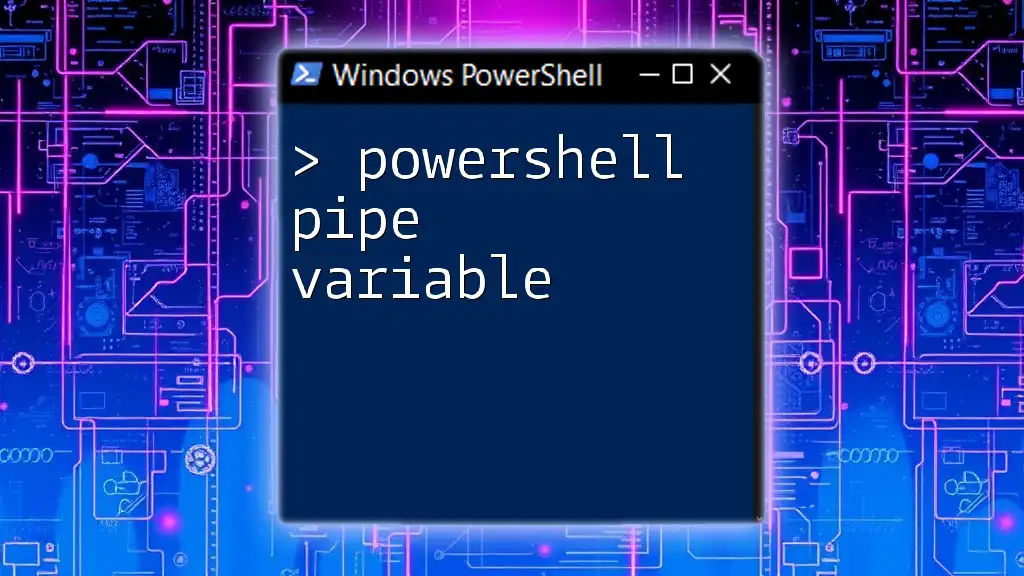
Call to Action
To further enhance your understanding of PowerShell scripting, consider exploring advanced topics and engaging in practical exercises. Join our community or subscribe to our courses for a deeper dive into PowerShell!
Additional Resources
For more information, check the official PowerShell documentation or look for further reading on conditional statements and error handling in scripts. This knowledge will significantly elevate your scripting skillset.