In PowerShell, you can split a string into variables by using the `-split` operator to separate the string based on a specified delimiter.
Here's a code snippet that demonstrates this:
$string = "apple,banana,cherry"
$variables = $string -split ','
$fruit1 = $variables[0]
$fruit2 = $variables[1]
$fruit3 = $variables[2]
Write-Host "Fruit 1: $fruit1, Fruit 2: $fruit2, Fruit 3: $fruit3"
Understanding Strings in PowerShell
What is a String?
A string is a sequence of characters used to represent text in programming. In PowerShell, strings are versatile and can store any collection of characters, including letters, numbers, and symbols. They are crucial for data manipulation and output formatting in scripts.
String Syntax and Examples
In PowerShell, strings can be created using either single quotes or double quotes. The two types of quotes influence how the strings are processed:
- Single Quotes (`'`): The text is treated literally. Variables inside single quotes will not be expanded.
- Double Quotes (`"`): The text is processed, and any variables within will be expanded to their values.
Here are some examples of string creation:
$singleQuotedString = 'Hello, World!'
$doubleQuotedString = "Hello, PowerShell!"
Using the double quotes allows for variable expansion and expressions to be included directly in the string.

Methods to Split Strings in PowerShell
Using the `-split` Operator
The `-split` operator is a powerful tool in PowerShell for breaking strings into an array based on specified delimiters. The basic syntax to use this operator is as follows:
$result = $string -split $delimiter
Example: Basic Usage of the `-split` Operator
Consider the string below, where fruits are separated by commas:
$inputString = "apple,banana,cherry"
$fruits = $inputString -split ","
After executing this code, the `$fruits` variable will contain an array with three elements: `"apple"`, `"banana"`, and `"cherry"`.
This method is efficient for breaking strings where a consistent delimiter exists.
Using the `Split()` Method
Another way to split strings in PowerShell is by using the `.Split()` method. This method is part of the string object's functionality in .NET and is called as follows:
$result = $string.Split($delimiter)
Example: Using the `Split()` Method
For example, to separate personal information in a string:
$inputString = "John;Doe;35"
$personData = $inputString.Split(";")
In this case, `$personData` will contain an array including `"John"`, `"Doe"`, and `"35"`. The method is intuitive and can be tailored according to specific needs, making it a popular choice for string manipulation.

Assigning Split Results to Variables
Direct Assignment to Variables
One of the great features of PowerShell is the ability to assign array elements directly to variables. This is how you can efficiently capture multiple values within a single line of code.
$inputString = "one,two,three"
$var1, $var2, $var3 = $inputString -split ","
After running this, `$var1` will hold `"one"`, `$var2` will have `"two"`, and `$var3` will contain `"three"`. This approach is not just clean, but also concise, facilitating easy assignment for multiple values.
Using Arrays to Capture Split Values
In instances where the number of elements is undetermined, using arrays is advantageous. When splitting a string into an array, you can capture all results efficiently:
$inputString = "dog;cat;fish"
$animals = $inputString -split ";"
To access individual elements within the array, use the index, as shown below:
$firstAnimal = $animals[0] # dog
This approach provides flexibility and ease when working with varying string lengths.

Advanced Techniques for String Splitting
Handling Multiple Delimiters
When strings contain multiple delimiters, you can still use the `-split` operator effectively. For example, consider a string with mixed delimiters:
$inputString = "apple, orange;banana:grape"
$result = $inputString -split "[,;:]"
In this scenario, the output will be an array with elements: `"apple"`, `" orange"`, `"banana"`, and `"grape"`. Using regex inside square brackets lets you specify multiple characters as delimiters, enhancing the splitting capability.
Trimming White Spaces
Often, strings can have undesired whitespace, which can disrupt processing. Trimming spaces is crucial for data integrity. Here’s how to handle this:
$inputString = " apple, orange ,banana "
$result = $inputString.Trim() -split ","
The `Trim()` method removes leading and trailing spaces. Thus, if you print `$result`, it will contain properly formatted elements, free of extra spaces.
Using Regex for Complex Patterns
For even more complex splitting scenarios, regular expressions (regex) are incredibly useful. Regex allows for sophisticated string manipulation based on patterns rather than single characters.
Here’s an example that utilizes regex to split based on multiple delimiters:
$inputString = "Item1-Item2|Item3.Item4"
$result = $inputString -split "[-|.|]"
This results in an array with elements reflecting the split values according to your specified patterns.

Common Pitfalls and Troubleshooting
Common Errors When Splitting Strings
When working with the `-split` operator or `.Split()` method, users may encounter issues if delimiters are incorrect or when trying to split non-string values. Common mistakes involve assuming a string has a certain format that it does not.
Debugging Tips
If you run into issues, consider using `Write-Host` to display intermediate results, helping you understand what's happening in your code.
For example:
Write-Host "Fruits Array: $fruits"
This outputs the current state of the `$fruits` array, helping to pinpoint errors effectively.
Using PowerShell’s debugging techniques, like `Set-PSBreakpoint`, can further aid in fine-tuning your scripts.
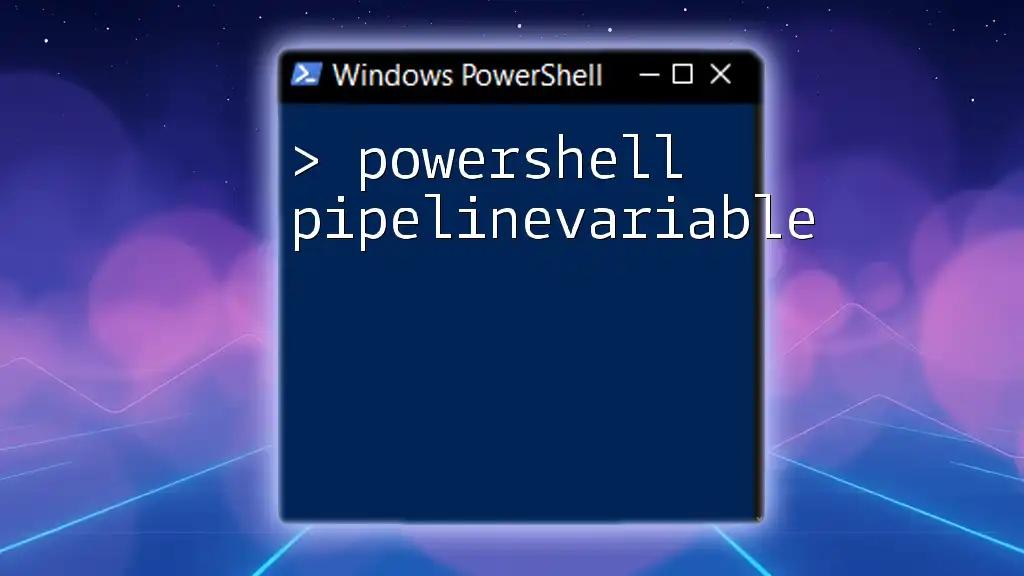
Conclusion
Mastering how to PowerShell split string into variables is an essential skill that enhances your ability to process and manipulate data effectively. By becoming proficient in using both the `-split` operator and the `Split()` method, along with advanced techniques such as regex, you can efficiently handle strings in complex scenarios. Regularly practicing these methods will deepen your understanding and broaden your scripting proficiency.