In PowerShell, you can find a substring within a string using the `.Contains()` method to return a boolean value indicating whether the substring exists.
$string = "Hello, World!"
$substring = "World"
$result = $string.Contains($substring)
Write-Host "Substring found: $result"
Understanding Strings in PowerShell
What is a String?
A string is a sequence of characters that can represent text in programming. In PowerShell, strings can be enclosed in single quotes (`'`) or double quotes (`"`).
-
Single-quoted strings are treated literally; any variable within them won't be expanded. For example:
$name = 'World' Write-Host 'Hello, $name' # Outputs: Hello, $name
-
Double-quoted strings allow for variable expansion:
$name = 'World' Write-Host "Hello, $name" # Outputs: Hello, World
Common Use Cases for String Searching
Understanding how to efficiently find a string in another string is essential for several tasks. Common use cases include:
- Data Extraction: Rapidly locate specific information in text files, such as logs or reports.
- Validation: Ensure that user input conforms to expected formats.
- Text Processing: Manipulate and format data for reporting purposes.

Methods to Find a String Within Another String
Using the `-like` Operator
The `-like` operator enables wildcard matching in PowerShell, allowing you to search for substrings with simple patterns.
How it works:
- The asterisk (`*`) acts as a wildcard, representing any sequence of characters.
Code Example:
$string = "Hello, World!"
if ($string -like "*World*") {
Write-Host "Found 'World' in the string."
}
In this example, the condition evaluates to true since "World" is indeed part of the string.
Explanation: The `-like` operator is particularly useful for partial matches when you're not concerned about the exact placement of text or case sensitivity.
Using the `-match` Operator
The `-match` operator utilizes regular expressions to find patterns within strings. This offers more powerful searching capabilities.
Code Example:
$string = "PowerShell is awesome!"
if ($string -match "awesome") {
Write-Host "Found 'awesome' in the string."
}
In this scenario, the code checks for the presence of "awesome" in the string.
Explanation: The `-match` operator is beneficial when needing to find more complex patterns or when case sensitivity matters.
Using `Contains` Method
The `.Contains()` method is a straightforward way to check if a string contains a specific substring.
How it works: This method returns a boolean value indicating whether the substring exists.
Code Example:
$string = "Learn PowerShell"
if ($string.Contains("PowerShell")) {
Write-Host "Found 'PowerShell' in the string."
}
In this example, the method returns `true`, confirming that "PowerShell" is part of the original string.
Explanation: It's important to note that the `.Contains()` method is case-sensitive, which means "powershell" would not match "PowerShell".
Using `IndexOf` Method
The `.IndexOf()` method returns the zero-based index of the first occurrence of a substring.
How it works: If the substring is not found, it returns `-1`.
Code Example:
$string = "Find the position of PowerShell"
$index = $string.IndexOf("PowerShell")
if ($index -ne -1) {
Write-Host "'PowerShell' found at index $index."
}
Here, the index will give you the position of "PowerShell" in the string, which can be useful for further manipulations.
Explanation: This method can be particularly useful when you need to know the exact location of a substring for additional processing.

Advanced Techniques for String Searching
Case Sensitivity Considerations
It's crucial to understand how case sensitivity impacts string searches in PowerShell.
- The `-like` operator is case-insensitive, while the `.Contains()` method is case-sensitive.
Code Example:
$string = "Hello, World!"
if ($string -like "*world*") {
Write-Host "Found 'world' (case insensitive)."
}
This would output: `Found 'world' (case insensitive).`
Conversely:
if ($string.Contains("world")) {
Write-Host "Found 'world' (case sensitive)."
} else {
Write-Host "'world' not found."
}
This would result in: `'world' not found.`
Using Regular Expressions for Complex Searches
Regular expressions provide a sophisticated method for identifying complex patterns within strings.
How to use regex: The `-match` operator can accept regex, making it powerful for tasks like validating formats.
Code Example:
$string = "Email: test@example.com"
if ($string -match "\w+@\w+\.\w+") {
Write-Host "Found an email format in the string."
}
Here, the regex pattern `\w+@\w+\.\w+` is used to match a typical email structure.
Explanation: Using regular expressions allows for greater flexibility in string searching, enabling checks for specific formats, such as emails or phone numbers.

Practical Applications
Real-World Scenarios
PowerShell's ability to find strings within strings can be immensely beneficial in real-world applications:
-
Example 1: Searching through log files to find specific error messages helps in diagnosing issues quickly and efficiently.
-
Example 2: Validating user input in scripts, such as checking for a valid IP address or a specific keyword.
Performance Considerations
When working with large datasets or logs, consider the performance of string searching methods. Some approaches, especially those using regex, can be slower than others.
Example: When searching a massive log file for patterns, using the `-match` operator could be inefficient if not optimized properly.
Understanding when to apply various string searching methods is key to maintaining performance and efficiency in your scripts.

Conclusion
In summary, knowing how to find strings within strings in PowerShell can significantly enhance your scripting capabilities. By leveraging methods such as `-like`, `-match`, `.Contains()`, and `.IndexOf()`, you can perform complex data manipulation and validation tasks with ease.
As you continue to hone your PowerShell skills, practice implementing these techniques in various scenarios to become more proficient.
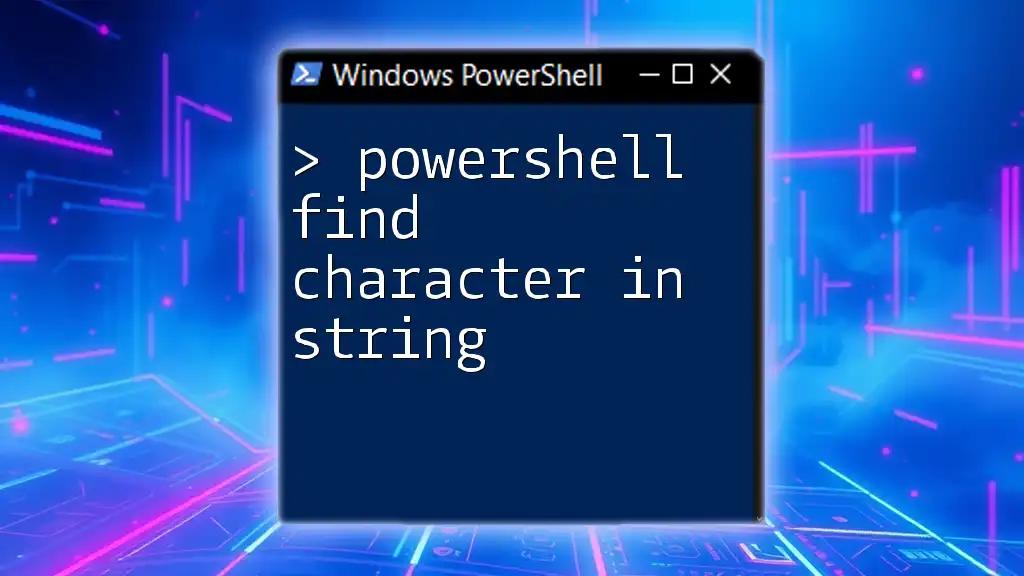
Additional Resources
Refer to the official [PowerShell documentation](https://docs.microsoft.com/en-us/powershell/) for more advanced topics and community discussions. Join forums and groups to connect with fellow PowerShell enthusiasts and further your learning.

Call to Action
Don’t stop here! Sign up for more concise PowerShell tutorials and empower your scripting proficiency today.