PowerShell string substitution allows you to dynamically replace placeholders in a string with specified values, enhancing code readability and maintainability.
Here's a simple code snippet demonstrating string substitution in PowerShell:
$greeting = "Hello, $name!"
$name = "World"
Write-Host $greeting
Understanding Strings in PowerShell
Definition of Strings
In PowerShell, a string is a sequence of characters that can encompass letters, numbers, and symbols. Strings are a vital part of any scripting language, and PowerShell is no exception. When creating scripts, understanding how to manipulate and substitute strings effectively can enhance the automation experience and improve code readability.
Strings in PowerShell can be encapsulated in single quotes (`'`) or double quotes (`"`). The key difference is that single quotes create a literal string, while double quotes allow for variable substitution and expression evaluation within the string.
String Data Types
All strings in PowerShell are of the String data type. It’s important to note that strings in PowerShell are immutable, meaning once a string is created, it cannot be changed. Instead, any manipulation creates a new string.
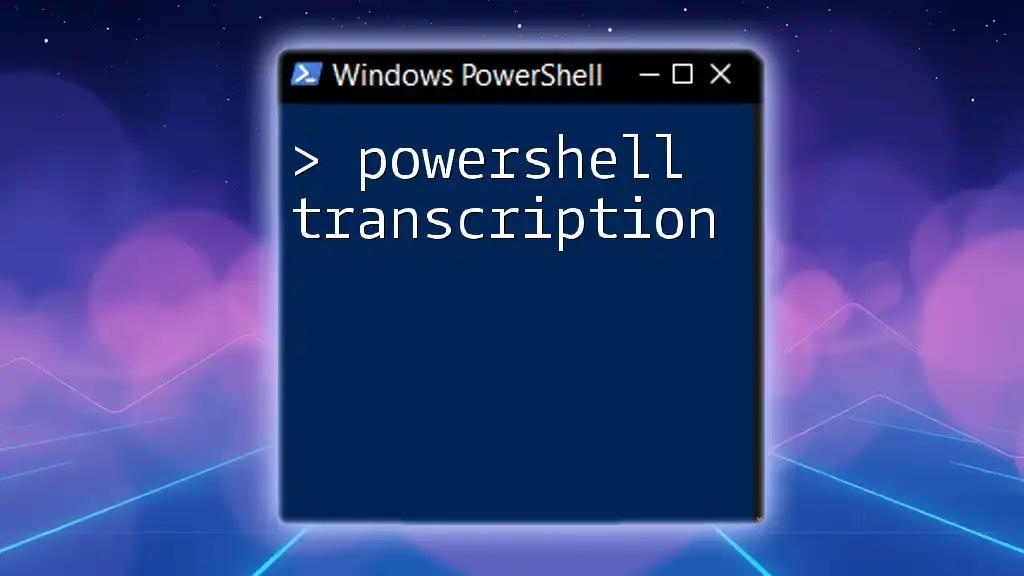
Fundamentals of String Substitution
What is String Substitution?
String substitution in PowerShell refers to the process of dynamically inserting variables or expressions into a string, allowing for greater flexibility and power in creating outputs. This becomes particularly useful when formatting messages or generating content based on variable values, enhancing both efficiency and readability.
The Role of Variables in String Substitution
Variables serve as containers for strings and other data types. In PowerShell, variables are declared using the `$` symbol, and values can be assigned easily. For instance:
$name = "John Doe"
Here, `$name` holds the string `"John Doe"`, which can be substituted into other strings.
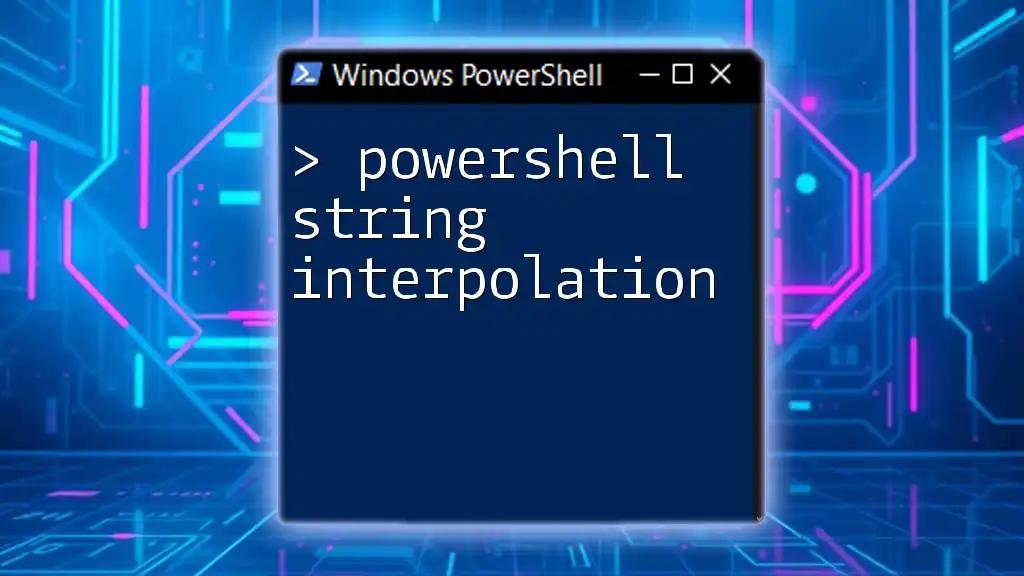
Methods of String Substitution in PowerShell
Using Double Quotes for Substitution
Double quotes in PowerShell allow for straightforward variable substitution. When a variable is included within double quotes, PowerShell evaluates it and replaces it with its current value. For example:
$name = "World"
"Hello, $name!"
This code snippet outputs: `Hello, World!`
If the variable is undefined, PowerShell will not throw an error but will return an empty space.
Substituting Expressions
More complex substitutions can also be executed within double quotes. PowerShell allows expressions to be evaluated within the string by enclosing them in `$(` and `)`. For example:
$a = 5
$b = 10
"The sum is: $($a + $b)"
The output here would be `The sum is: 15`. Substituting expressions can make debugging and displaying calculations more intuitive.
The Substring Method
The `.Substring()` method is useful for extracting parts of a string. It allows specifying a starting index and the length of the substring needed. Here’s how it works:
$text = "Hello, PowerShell"
$text.Substring(7, 10) # Output: PowerShell
In this case, starting from index `7`, a substring of length `10` is extracted, resulting in `PowerShell`. This method is particularly helpful when processing strings where specific information needs to be isolated.
String Replacement with `-replace`
PowerShell includes a flexible `-replace` operator for substituting parts of strings. Here’s a practical example:
$str = "The cat sat on the mat"
$str -replace "cat", "dog"
This results in `The dog sat on the mat`. The `-replace` operator employs regular expressions, providing powerful capabilities for more complex substitutions.
Using String Interpolation
String interpolation refers to the process of incorporating variables into strings seamlessly. By simply placing a variable within double quotes, its value will be embedded in the resulting string. For example:
$firstName = "Alice"
$lastName = "Doe"
"My name is $firstName $lastName"
The output would be `My name is Alice Doe`. This feature promotes clean and readable code, making it clear what the final output will contain.

Advanced Techniques in String Substitution
Using the Format Operator
The `-f` format operator allows for sophisticated string formatting, making it easy to construct strings with dynamic data. Here’s an example:
$name = "Alice"
$age = 30
"Name: {0}, Age: {1}" -f $name, $age
This would produce: `Name: Alice, Age: 30`. The format operator accepts placeholders indicated by `{n}` where `n` corresponds to the position of the variable in the `-f` argument list.
String Builder for Performance Optimization
For scenarios where performance is critical, especially when manipulating large strings or concatenating many values, it is recommended to use the `StringBuilder` class. This class allows for more efficient string handling and avoids the overhead of immutable strings. Here’s how to implement it:
$sb = New-Object System.Text.StringBuilder
[void]$sb.Append("Hello ")
[void]$sb.Append("World")
$sb.ToString() # Output: Hello World
Using `StringBuilder`, you can construct strings incrementally without the typical performance penalties.
Handling Escaped Characters
When working with strings, you may encounter situations that require escaping certain characters. For instance, to include a backslash in a string, you’ll need to escape it by typing it twice:
"This is a backslash: \\"
This results in `This is a backslash: \`. Understanding how to handle escaped characters is crucial for managing strings effectively.
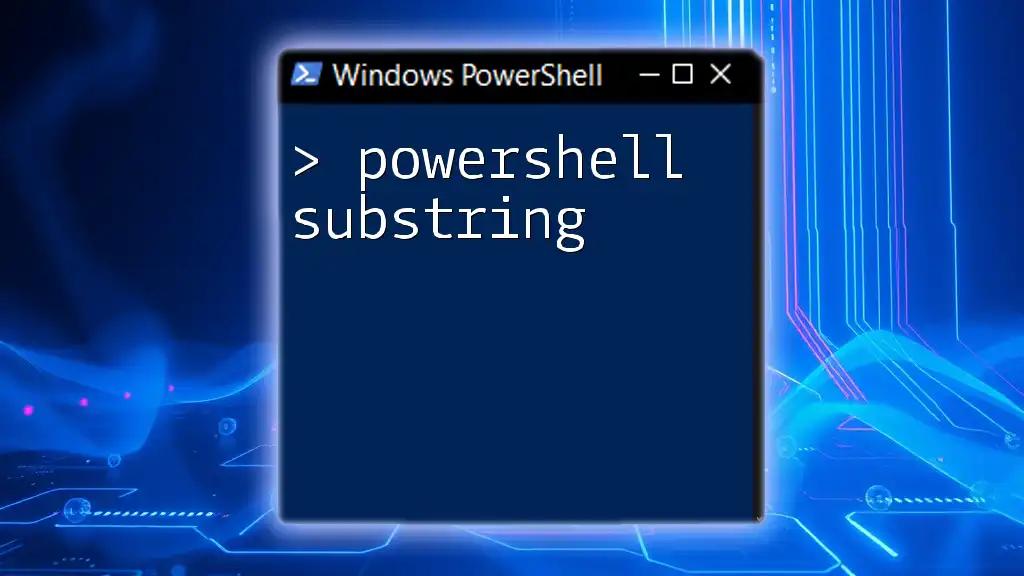
Best Practices for String Substitution
Consistency in Quotation Usage
Consistency in using single and double quotes is paramount. For instance, prefer double quotes when you need variable substitution and use single quotes when you want a literal string without evaluation. This approach reduces errors and improves code maintainability.
Performance Considerations
When managing large strings, consider performance implications. The use of `StringBuilder` can save time and resources, especially in scripts involving extensive string manipulation.
Debugging Substitution Issues
Common pitfalls in string substitution may include misplaced brackets or incorrect variable names. If the output doesn't appear as expected, verify the variable's scope and context. Using the `Write-Host` command can help to debug outputs easily:
Write-Host "Current Value: $name"
This will print the current value of `$name`, assisting in troubleshooting.
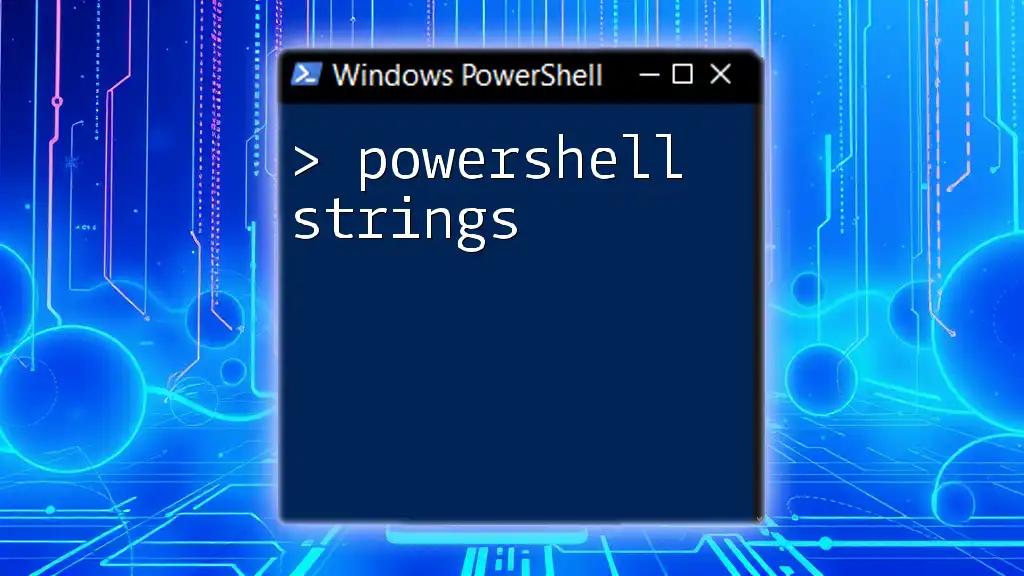
Conclusion
String substitution is a powerful technique in PowerShell that enables dynamically generating strings with embedded variables and expressions. By mastering these methods—using double quotes, the `-replace` operator, interpolation, and more—you can significantly increase the efficiency and readability of your PowerShell scripts. Embrace these techniques to enhance your scripting mastery and streamline automation tasks.

Additional Resources
For further exploration of PowerShell string substitution, consider visiting the official PowerShell documentation or engaging with online communities dedicated to scripting and automation.

Call to Action
We invite you to share your experiences with string substitution in PowerShell. Have questions? Feel free to ask in the comments, and don’t forget to subscribe for more insightful PowerShell tips and guides!