PowerShell string methods allow users to manipulate and interact with string data effectively, enhancing their scripting capabilities.
Here's a simple example demonstrating how to use the `ToUpper()` method to convert a string to uppercase:
$originalString = "Hello, World!"
$upperCaseString = $originalString.ToUpper()
Write-Host $upperCaseString # Outputs: HELLO, WORLD!
Understanding Strings in PowerShell
Definition of Strings
In PowerShell, a string is a sequence of characters used to represent text. These strings are widely used in scripts and commands for data manipulation, user output, and parameter passing.
Key Characteristics of Strings
One crucial aspect of strings in PowerShell is immutability. This means that once a string is created, it cannot be modified directly. Any operation that appears to change a string will actually create a new string without altering the original. Understanding this concept is vital for managing memory and optimizing performance in your scripts.

Common String Methods in PowerShell
Getting Started with String Methods
PowerShell provides a variety of methods to manipulate strings. To invoke a string method, simply use the dot notation followed by the method name. For example:
$myString = "Hello"
$length = $myString.Length
This will give you the length of the string stored in `$myString`.
Overview of Essential String Methods
Length Method
The `Length` method returns the total number of characters in a string, including spaces and punctuation.
Usage:
$sampleString = "Hello, PowerShell!"
$length = $sampleString.Length
Write-Output $length # Output: 17
In this example, `17` indicates that there are 17 characters in the string.
Substring Method
The `Substring` method allows you to extract a portion of a string by specifying a starting index and an optional length.
Usage:
$sampleString = "PowerShell"
$subString = $sampleString.Substring(0, 5)
Write-Output $subString # Output: Power
This method is particularly useful for parsing or extracting information from strings.
ToUpper and ToLower Methods
These methods are used to convert the entire string to either uppercase or lowercase.
Usage:
$sampleString = "Hello World"
Write-Output $sampleString.ToUpper() # Output: HELLO WORLD
By changing the case of strings, you can standardize input data or prepare it for comparisons.
Trim Method
The `Trim` method removes any leading and trailing whitespace characters from a string.
Usage:
$sampleString = " Trim this string "
$trimmedString = $sampleString.Trim()
Write-Output $trimmedString # Output: "Trim this string"
This method is excellent for cleaning up user input data before processing.
Advanced String Methods
Replace Method
The `Replace` method substitutes all occurrences of a specified substring in the original string with a new substring.
Usage:
$sampleString = "Hello World"
$newString = $sampleString.Replace("World", "PowerShell")
Write-Output $newString # Output: Hello PowerShell
This method is useful for updating strings dynamically or altering content based on conditions.
Split Method
The `Split` method divides a string into an array of substrings based on a delimiter.
Usage:
$sampleString = "apple,banana,cherry"
$fruits = $sampleString.Split(',')
Write-Output $fruits # Output: apple banana cherry
This method can help in scenarios where you need to process individual items from a comma-separated list or similar formats.
Join Method
The `Join` method provides a convenient way to concatenate elements of an array into a single string, with a specified character or string separating each element.
Usage:
$fruits = @('apple', 'banana', 'cherry')
$joinedString = [String]::Join(',', $fruits)
Write-Output $joinedString # Output: apple,banana,cherry
This method is particularly beneficial when you need to convert arrays back into a single formatted string.
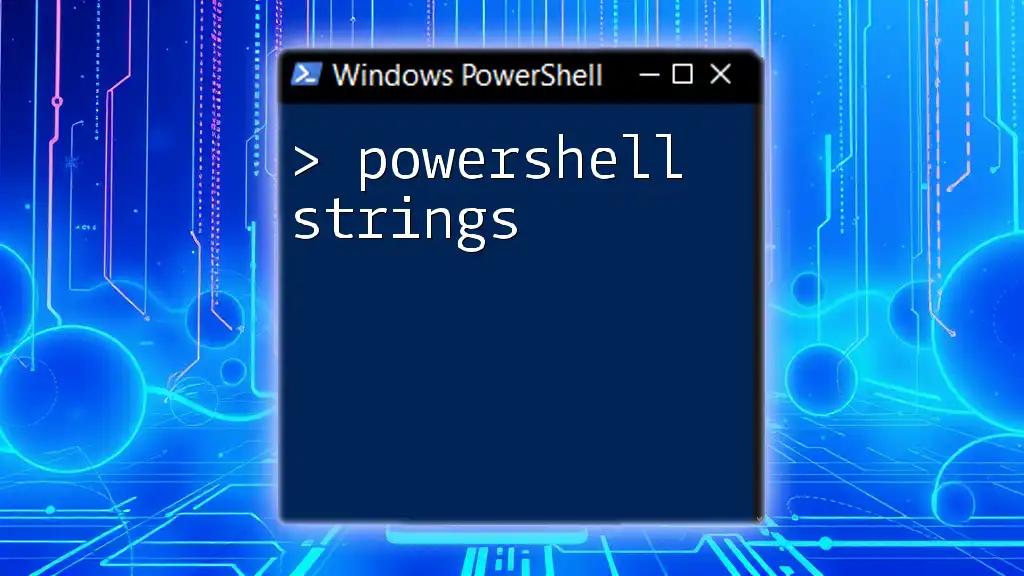
Working with String Formatting
Format Method
The `Format` method allows you to create a formatted string, which is particularly useful for generating user-friendly outputs.
Usage:
$name = "World"
$greeting = "Hello {0}" -f $name
Write-Output $greeting # Output: Hello World
This approach enables you to insert variables into strings dynamically while maintaining control over the format.
Interpolation Techniques
PowerShell supports string interpolation, allowing you to embed variables directly within string literals by enclosing them in double quotes. You can also use the `$()` format to evaluate complex expressions within strings.
Usage:
$name = "PowerShell"
Write-Output "Welcome to $($name)!" # Output: Welcome to PowerShell!
This technique simplifies string building and is especially useful for generating dynamic outputs.
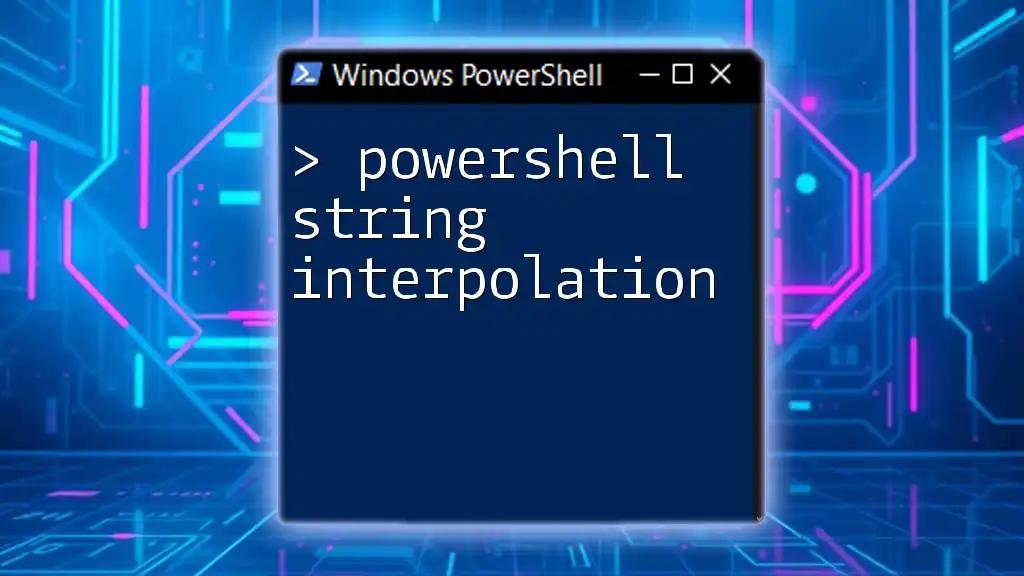
Practical Applications of String Methods
Data Manipulation Scenarios
String methods are indispensable when cleaning and managing data. For example, using `Trim`, `Replace`, and `Split` lets you preprocess user input, ensuring that your applications only deal with clean, well-structured data.
Dynamic Content Creation
String methods also play a crucial role in generating reports or logs, where readability is paramount. Utilizing methods like `Join`, `Format`, and interpolation creates clear and organized output from your scripts, making it easier for users to understand the results.

Conclusion
Mastering PowerShell string methods is essential for effective scripting and automation. These methods provide a powerful toolkit for handling text in diverse scenarios, from cleaning data to generating dynamic outputs. Practice using these methods in real-world scenarios to reinforce your learning and expand your PowerShell proficiency.

Additional Resources
For further exploration, refer to the official PowerShell documentation and participate in online communities and forums to connect with other PowerShell enthusiasts, where you can share insights and best practices in leveraging string methods effectively.