Powershell's `TimeSpan` represents a duration of time and can be used to calculate the difference between two dates or define a specific period of time.
$startTime = Get-Date
# Simulate some code execution with a 2-second pause
Start-Sleep -Seconds 2
$endTime = Get-Date
$duration = $endTime - $startTime
Write-Host "Execution time: $($duration.TotalSeconds) seconds"
What is a Timespan in PowerShell?
In PowerShell, a Timespan object represents a duration of time, allowing users to calculate, manipulate, and display time intervals conveniently. It is crucial for tasks that require timing calculations, such as tracking execution durations or scheduling events. Understanding how to utilize the Timespan object can simplify many scripting tasks and enhance overall productivity.
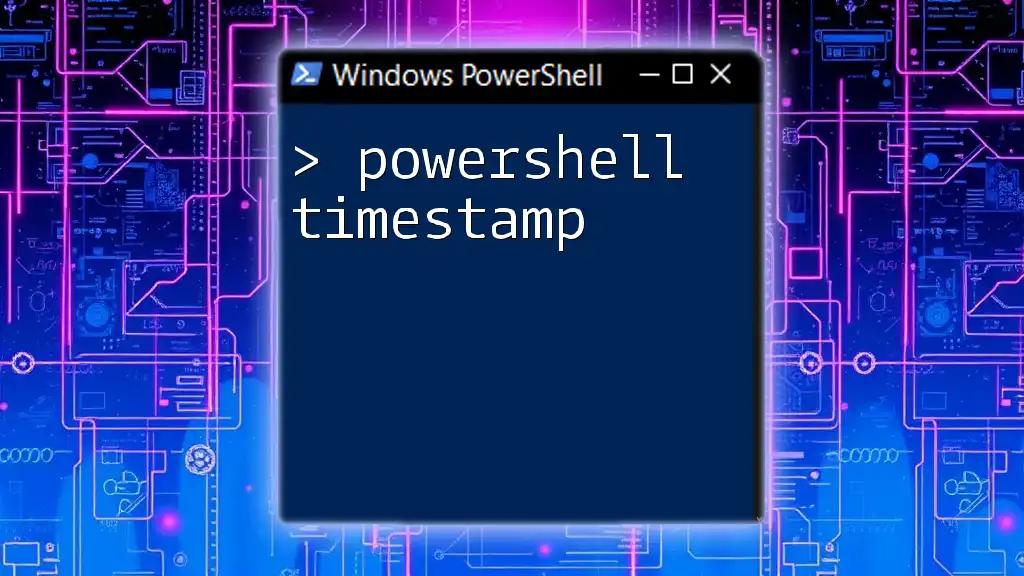
Understanding the Timespan Object
Definition and Purpose
The Timespan object encapsulates a range of time expressed in terms of days, hours, minutes, seconds, and fractions of a second. This functionality is particularly useful in scripting for:
- Calculating differences between dates.
- Performing timing operations in automation scripts.
- Handling scheduled tasks and events efficiently.
Key Properties of the Timespan Object
Days, Hours, Minutes, and Seconds
Every Timespan object consists of distinct properties that define its components:
- Days: Represents the total number of days in the Timespan.
- Hours: Indicates the total hours beyond the complete days.
- Minutes: Shows how many minutes remain in the Timespan.
- Seconds: Tells how many seconds are left after accounting for the other components.
For example, to create a Timespan and display its components, you can use:
$timeSpan = New-TimeSpan -Days 2 -Hours 5
Write-Output "Days: $($timeSpan.Days), Hours: $($timeSpan.Hours)"
Total Days, Total Hours, Total Minutes
In addition to standard components, the Timespan object provides Total Days, Total Hours, and Total Minutes properties. These properties represent the entire duration expressed in their respective units, making it easier to conduct calculations.
For instance, to retrieve the total hours from a Timespan, you can run:
$totalHours = $timeSpan.TotalHours
Write-Output "Total Hours: $totalHours"
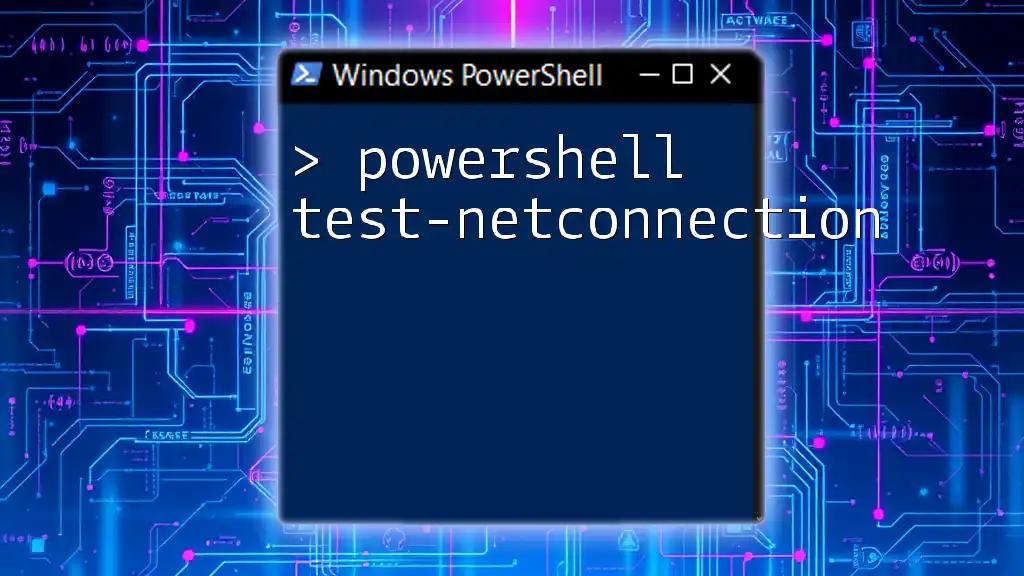
Creating a New Timespan
Using the New-TimeSpan Cmdlet
The primary method of creating a Timespan in PowerShell is through the `New-TimeSpan` cmdlet. This cmdlet is highly versatile and can accept various parameters, such as start and end dates or duration components.
An example of utilizing the `New-TimeSpan` cmdlet is as follows:
$myTimeSpan = New-TimeSpan -Start "2023-01-01" -End "2023-12-31"
Write-Output "My Time Span: $($myTimeSpan)"
In this case, the Timespan object stores the duration between January 1, 2023, and December 31, 2023.
Common Scenarios for New Timespan
Creating Timespans is particularly valuable for calculating time intervals, such as project timelines or task durations. For example, you can determine the duration of events scheduled in a calendar or calculate the time taken for various operations.
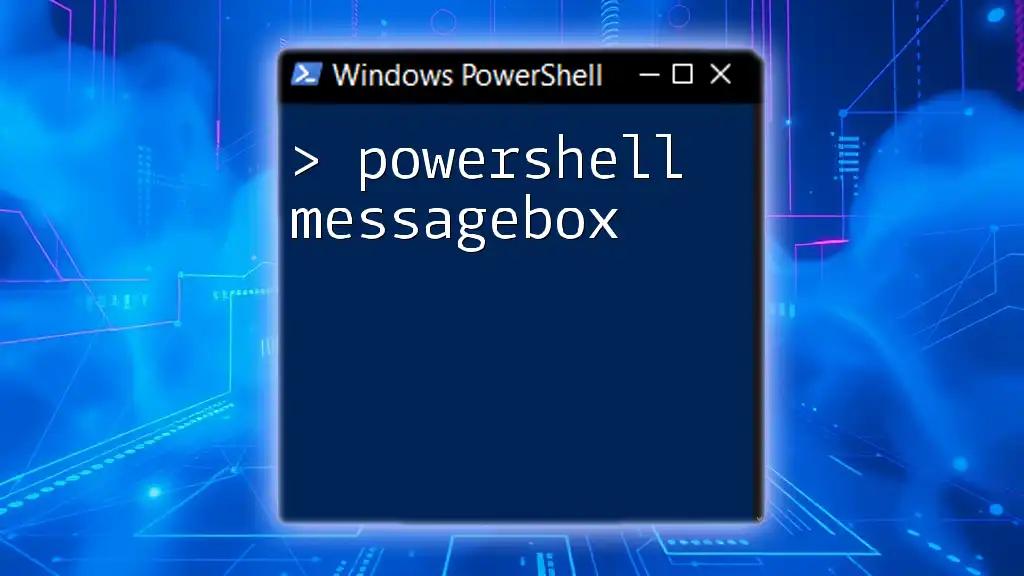
Manipulating Timespan
Adding and Subtracting Time
PowerShell enables users to manipulate Timespan objects seamlessly, allowing for addition and subtraction of time intervals. Using the `.Add()` method on a Timespan instance enables you to extend the duration as needed.
Here’s an example of how to add hours to an existing Timespan:
$extendedTimeSpan = $myTimeSpan.Add((New-TimeSpan -Hours 3))
Write-Output "Extended Time Span: $($extendedTimeSpan)"
This operation results in a new Timespan that includes an additional 3 hours.
Comparing TimeSpan Objects
PowerShell supports comparison between Timespan objects using standard comparison operators. This functionality is useful when you need to determine the order of events or validate time intervals.
Here is an example comparing two Timespan objects:
$timeSpan1 = New-TimeSpan -Hours 10
$timeSpan2 = New-TimeSpan -Hours 5
if ($timeSpan1 -gt $timeSpan2) {
Write-Output "TimeSpan 1 is greater than TimeSpan 2"
}
In this example, the result illustrates whether the first Timespan is longer than the second.
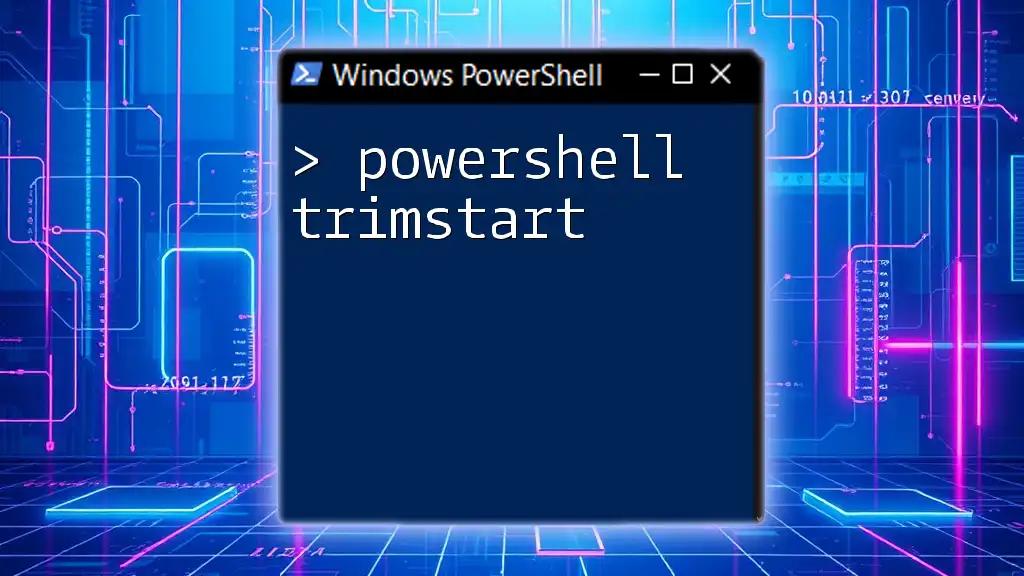
Practical Applications of Timespan
Scripting Tasks with Timespan
Timespan objects can greatly enhance script automation by managing scheduling effectively. For example, scheduling background jobs or determining when to execute specific tasks can be done using Timespan calculations.
Logging Execution Time with Timespan
Another common usage of Timespan is tracking the execution time of a script. This is pivotal for optimizing performance and ensuring tasks are completed within expected time frames.
Here’s how you can implement logging for script execution time:
$start = Get-Date
# Your script logic here
Start-Sleep -Seconds 5 # Simulating script work
$duration = New-TimeSpan -Start $start -End (Get-Date)
Write-Output "Script Execution Time: $duration"
In this example, the script sleeps for 5 seconds, simulating processing time, and logs the duration using a Timespan object.

Conclusion
Understanding various aspects of PowerShell Timespan equips users with the tools needed for effective and efficient time management in their scripts. From creating new Timespan objects to manipulating and comparing them, there are numerous applications that can enhance your scripting capabilities.
Practicing these concepts in your day-to-day PowerShell scripts will not only make your work easier but also significantly improve your productivity. Dive into experimenting with Timespan, and witness how it can transform your automation tasks!

Additional Resources
For further learning about Timespan in PowerShell, consider exploring:
- The official PowerShell documentation on Timespan.
- Community forums and blogs for hands-on examples and discussions.
Feel free to reach out for more insights or if you have questions about specific use cases!