In PowerShell, the script path refers to the location of a PowerShell script file on the filesystem, which can be used to execute the script in the PowerShell environment.
Here's how to get the current script path:
$scriptPath = $PSScriptRoot
Write-Host "The script is located at: $scriptPath"
Understanding PowerShell Script Path
What is a Script Path in PowerShell?
A PowerShell script path refers to the full location of a script file within the file system. It is vital for executing scripts, as PowerShell needs to know where the script resides to run it correctly. Understanding script paths is essential for automating tasks, managing files, and organizing scripts efficiently.
Components of a PowerShell Script Path
The script path is comprised of several elements:
- Drive Letter: This indicates the storage drive (e.g., `C:`).
- Folder Structure: The hierarchy of folders leading to your script.
- Script Name and Extension: The file name and its extension (most often `.ps1` for PowerShell scripts).
Example: A typical PowerShell script path could look like this: `C:\Scripts\MyScript.ps1`. Here, `C:` is the drive, `Scripts` is the folder, and `MyScript.ps1` is the script file.
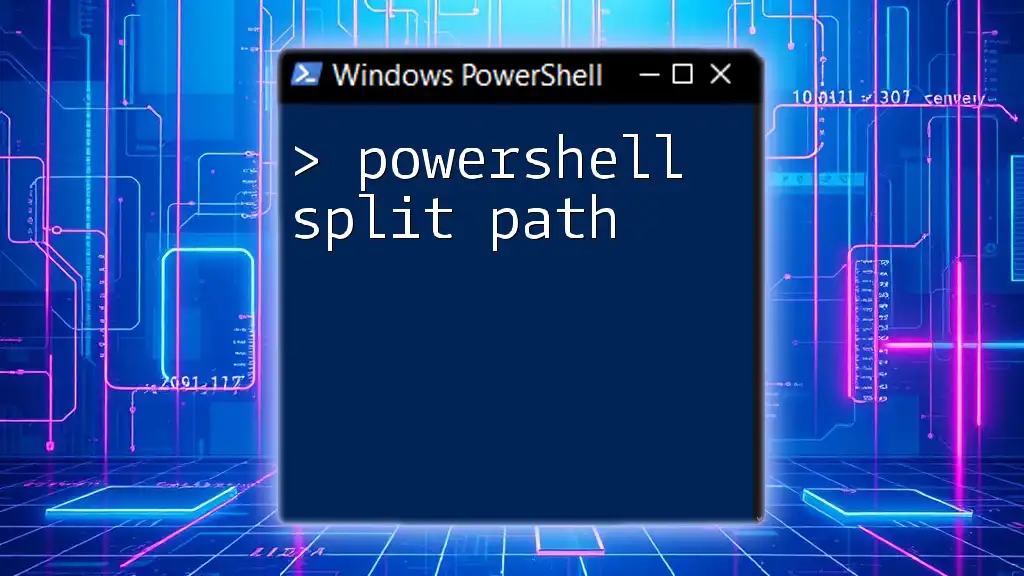
PowerShell Script File Path
How to Define a Script File Path
When defining a script file path, it's crucial to understand the difference between absolute and relative paths. An absolute path specifies the complete location from the root of the file system, while a relative path defines the location in relation to the current directory.
For example, an absolute path might look like this:
$scriptPath = "C:\Scripts\MyScript.ps1"
Conversely, a relative path, if your current directory is `C:\Scripts`, could simply be:
$scriptPath = "MyScript.ps1"
Accessing Script File Paths
To work with script file paths, you need to access them within your PowerShell scripts. Here’s how you can declare and use the path of a script.
Example Code Snippet:
$scriptPath = "C:\Scripts\MyScript.ps1"
Write-Host "The script is located at: $scriptPath"
This snippet displays the absolute path of the script, which can be helpful for debugging or logging purposes.
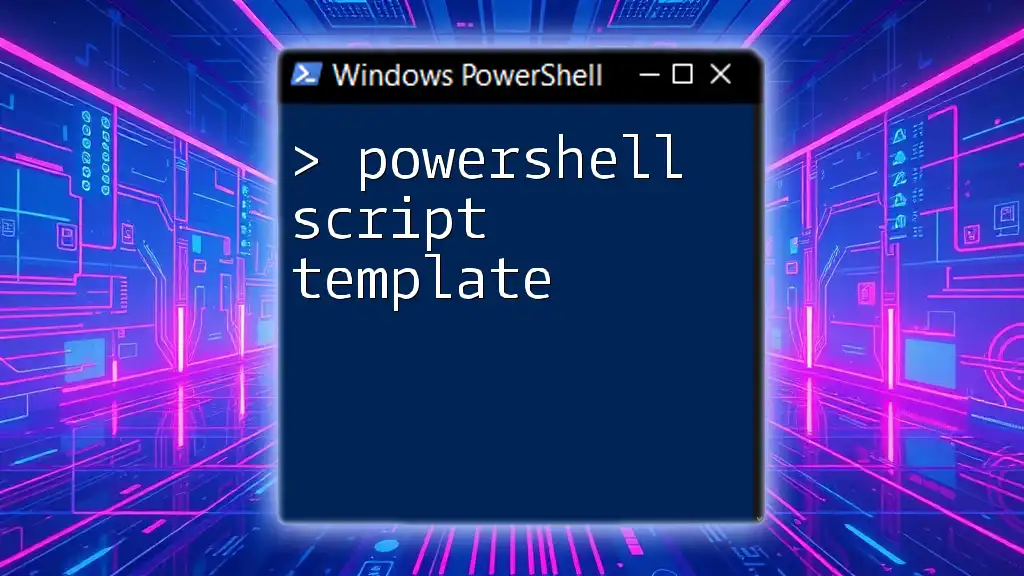
How to Get the Script Path in PowerShell
Using `$PSScriptRoot`
One of the most useful automatic variables in PowerShell is `$PSScriptRoot`. This variable holds the directory from which a script is being executed, allowing for dynamic path resolution.
Example Code Snippet:
Write-Host "The script is located at: $PSScriptRoot"
This means that if you move your script around, it will still always reference the directory where it resides, making your scripts more portable and robust.
Using `Get-Location`
Another way to get the current directory in PowerShell is by utilizing the `Get-Location` cmdlet. This method can differentiate between where the script is located and where you are executing it from.
Example Code Snippet:
$currentLocation = Get-Location
Write-Host "Current directory: $currentLocation"
This is particularly useful for debugging when you're not sure which directory your script is executing from.
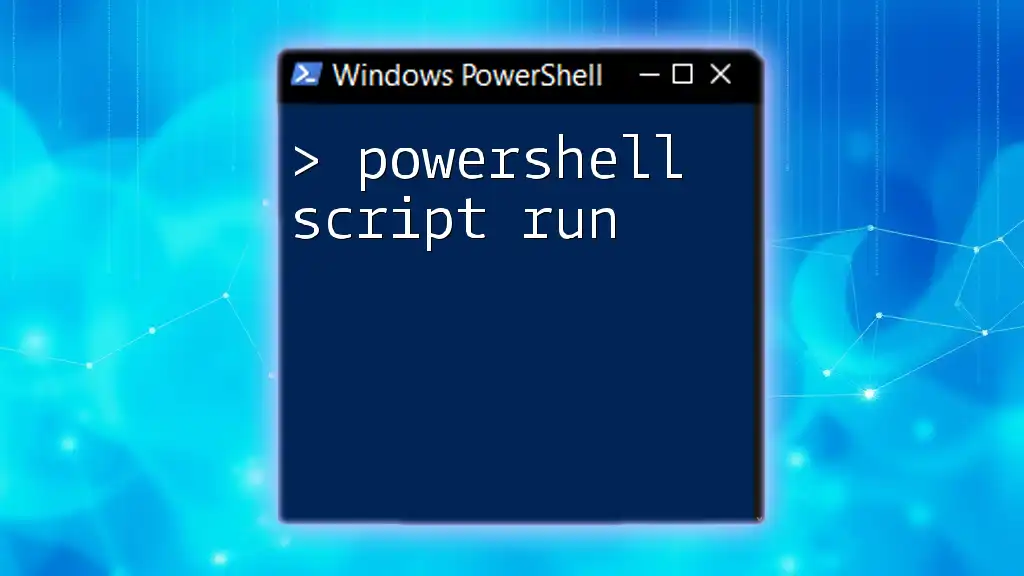
Get Current Script Path PowerShell
Utilizing `MyInvocation.MyCommand.Path`
To retrieve the actual path of the currently executing script, you can utilize the `MyInvocation` automatic variable. It provides a wealth of information about the running script, including its full path.
Example Code Snippet:
$currentScriptPath = $MyInvocation.MyCommand.Path
Write-Output "Current script path: $currentScriptPath"
This method is immensely practical, especially when your script needs to access other files or resources located relative to its own location.
Practical Usage
Understanding how to get the current script path is essential in various scenarios. For instance, if your script needs to read configuration files stored in the same directory or log output to a file, knowing its exact path becomes vital. It eliminates hardcoding paths, which can lead to broken scripts when files are moved.
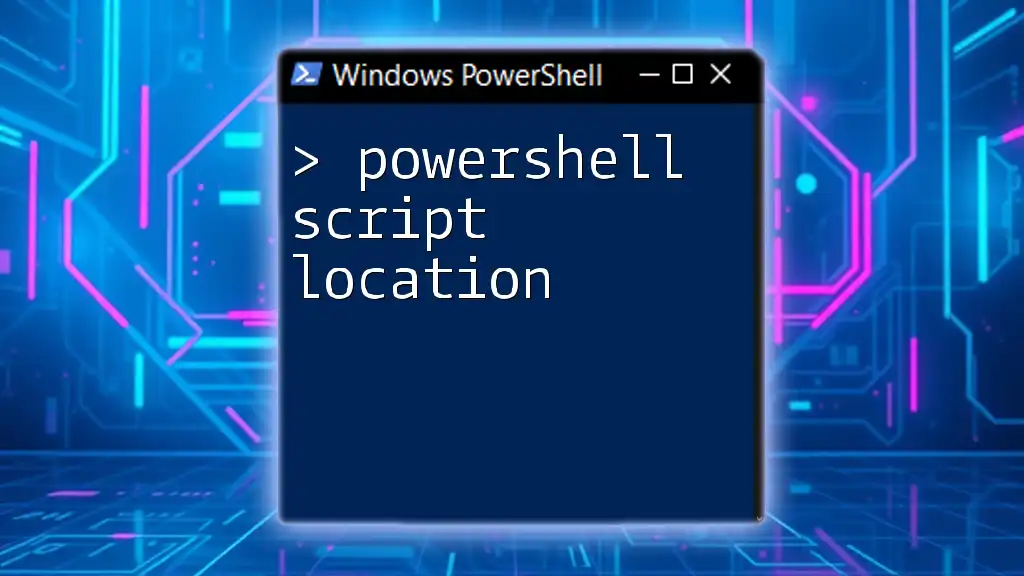
Best Practices for Managing PowerShell Script Paths
Consistency in Path Definitions
Using consistent formats for defining script paths is vital to ensure your scripts are maintainable and less prone to errors. Always use either absolute or relative paths but remain consistent throughout the script.
Handling Paths in Different Contexts
When running scripts in diverse environments (like different servers or development machines), hardcoded paths can cause failures. To mitigate this, employ dynamic path resolution methods discussed earlier and utilize environment variables where appropriate.
Security Considerations
Securing your scripts and their locations is crucial. Avoid hardcoded paths, especially if they lead to sensitive data. Instead, use environment variables to keep sensitive information hidden. This practice not only enhances security but also makes it easier to deploy scripts across multiple environments without changing code.
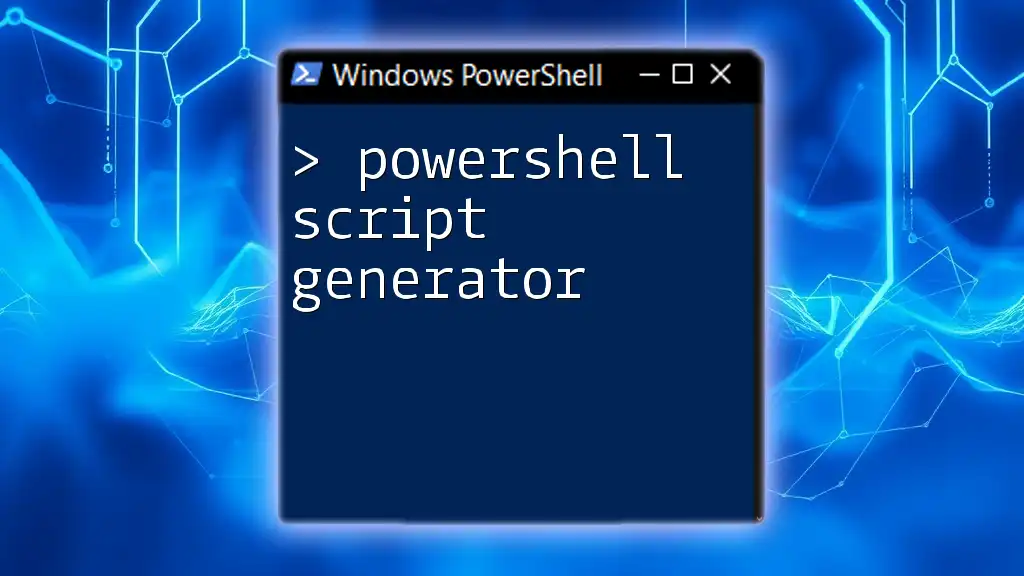
Conclusion
Understanding the concept of PowerShell script paths is essential for efficient scripting and automation. Whether you are defining, accessing, or retrieving script paths, mastering these concepts will significantly enhance your PowerShell capabilities.
By familiarizing yourself with the available tools and best practices for managing script paths, you can ensure your scripts are robust, secure, and easy to maintain. Now, you’re encouraged to put this knowledge into practice and elevate your PowerShell scripting skills!