In PowerShell, you can execute an executable file (`.exe`) by simply calling its path, as shown in the following example:
Start-Process "C:\Path\To\Your\File.exe"
Understanding EXE Files
What is an EXE File?
An EXE file (short for "executable") is a compiled program in Windows that the operating system can directly run. These files usually contain instructions for a computer to perform specific tasks, such as installing software, launching applications, or executing system commands.
Common use cases for EXE files include:
- Installing software packages
- Running system utilities
- Managing configurations and settings
When to Use PowerShell to Execute EXE Files
Using PowerShell to run EXE files can significantly enhance your productivity. Here are some scenarios where running an EXE with PowerShell is particularly advantageous:
- Automation Tasks: When you need to execute the same program multiple times or on a schedule.
- Batch Processing: If you need to run multiple EXE files sequentially or concurrently in a single script.
- Remote Execution: PowerShell allows for running commands on remote systems, which can be essential for network administrators.
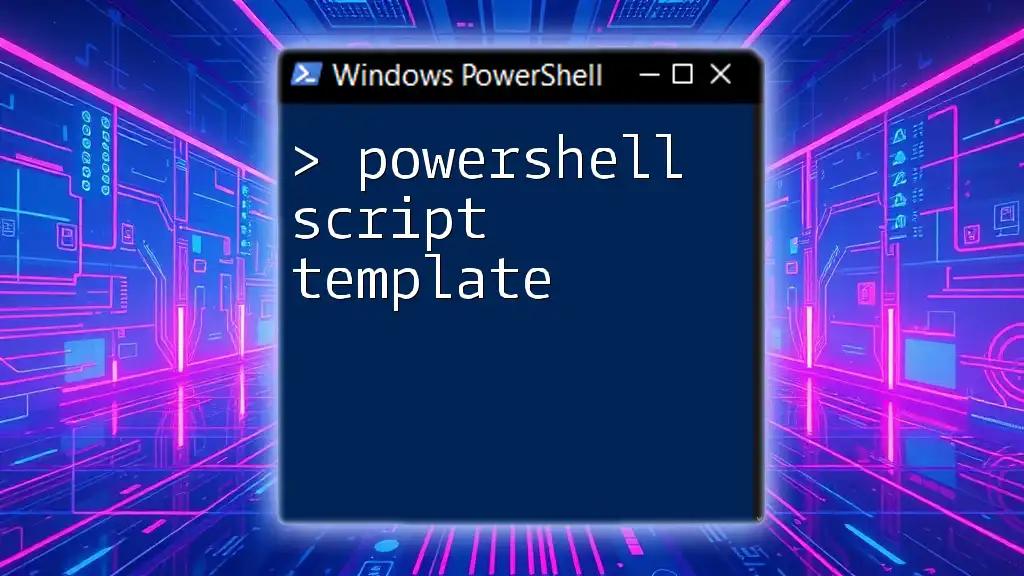
How to Run EXE with PowerShell
The Basic Command
The most straightforward way to execute an EXE file in PowerShell is to use the call operator `&`. This operator allows you to run the executable directly. The basic syntax is as follows:
& "C:\Path\To\YourExecutable.exe"
This command will execute the specified EXE file. Be sure to enclose the path in double quotes if it includes spaces.
Using Start-Process
Another powerful way to run EXE files is by using the `Start-Process` cmdlet. This cmdlet provides more features, such as starting a process in a new window or redirecting output. Here's how to use it:
Start-Process -FilePath "C:\Path\To\YourExecutable.exe"
This command starts the specified executable in a new process.
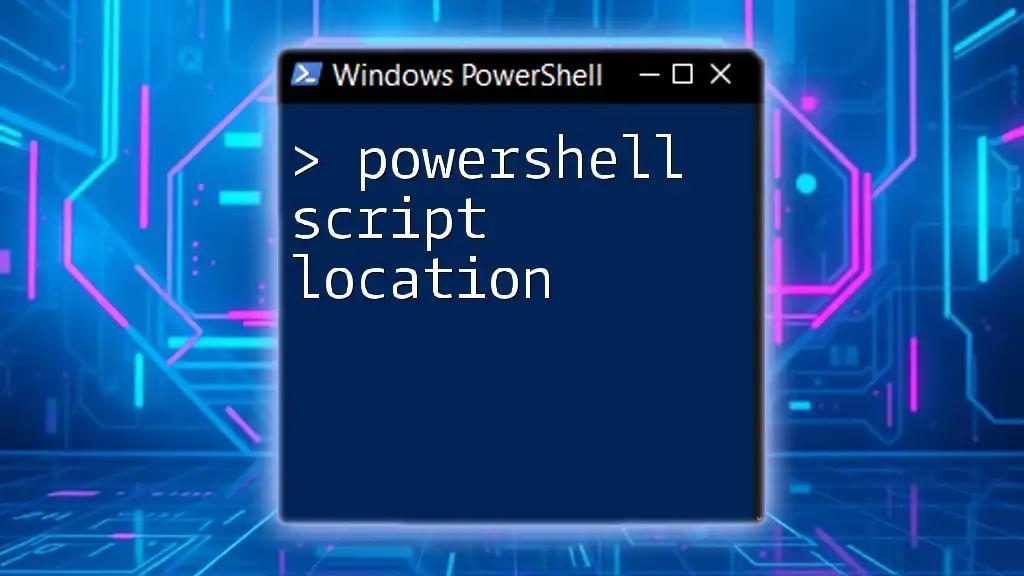
How to Run an Executable in PowerShell with Arguments
Passing Arguments to EXE Files
Many executable programs accept command-line arguments that perform specific actions or modify behavior. You can pass these arguments to an EXE file using PowerShell. Here’s an example:
Start-Process -FilePath "C:\Path\To\YourExecutable.exe" -ArgumentList "arg1", "arg2"
In this example, replace `"arg1"` and `"arg2"` with the actual arguments required by your EXE file.
Using StdOut and StdErr
Standard output (StdOut) and standard error (StdErr) are crucial for managing output from an EXE file. You can capture or redirect this output easily using PowerShell. Here’s an example of redirecting output to a file:
Start-Process -FilePath "C:\Path\To\YourExecutable.exe" -RedirectStandardOutput "output.txt" -NoNewWindow
This command runs the EXE and writes the output to `output.txt`, allowing you to easily review the results later.
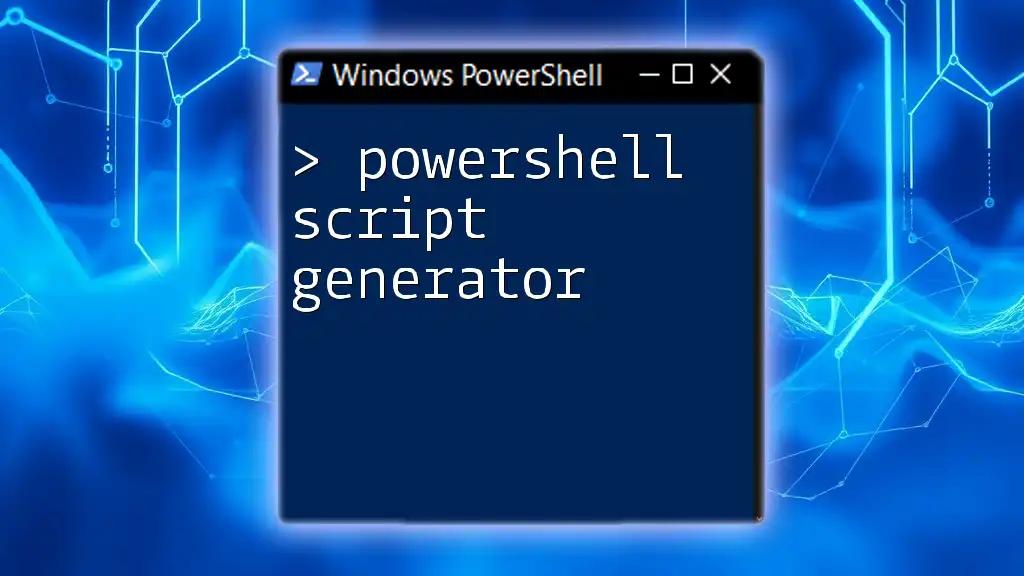
Running EXE Files in PowerShell Scripts
Creating a PowerShell Script
Creating a PowerShell script that executes an EXE file is straightforward. Begin by opening a text editor and writing your script. Here’s a simple example:
# MyScript.ps1
& "C:\Path\To\YourExecutable.exe" -ArgumentList "arg1", "arg2"
Save this file with a `.ps1` extension. Your script is now ready to be executed.
Executing the Script
To run your PowerShell script, use the following command in the PowerShell console:
powershell -ExecutionPolicy Bypass -File "C:\Path\To\MyScript.ps1"
The `ExecutionPolicy Bypass` parameter allows the script to run without restrictions imposed by your system's execution policy.

Run EXE from PowerShell Script: Best Practices
Error Handling in PowerShell Scripts
Implementing robust error handling is essential for any PowerShell script. This practice ensures that your script can handle unexpected situations without crashing. Here’s an example using try/catch/finally blocks:
try {
Start-Process -FilePath "C:\Path\To\YourExecutable.exe"
} catch {
Write-Host "An error occurred: $_"
}
In this snippet, if an error occurs while attempting to run the EXE, the catch block will execute, providing you with valuable feedback about the issue.
Logging and Output Management
Maintaining logs of your program's executions can help troubleshoot issues down the road. You can easily log output and errors to separate files. For example:
Start-Process -FilePath "C:\Path\To\YourExecutable.exe" -NoNewWindow -RedirectStandardOutput "log.txt" -RedirectStandardError "error.txt"
This command captures both standard output and errors, writing them to `log.txt` and `error.txt` respectively.
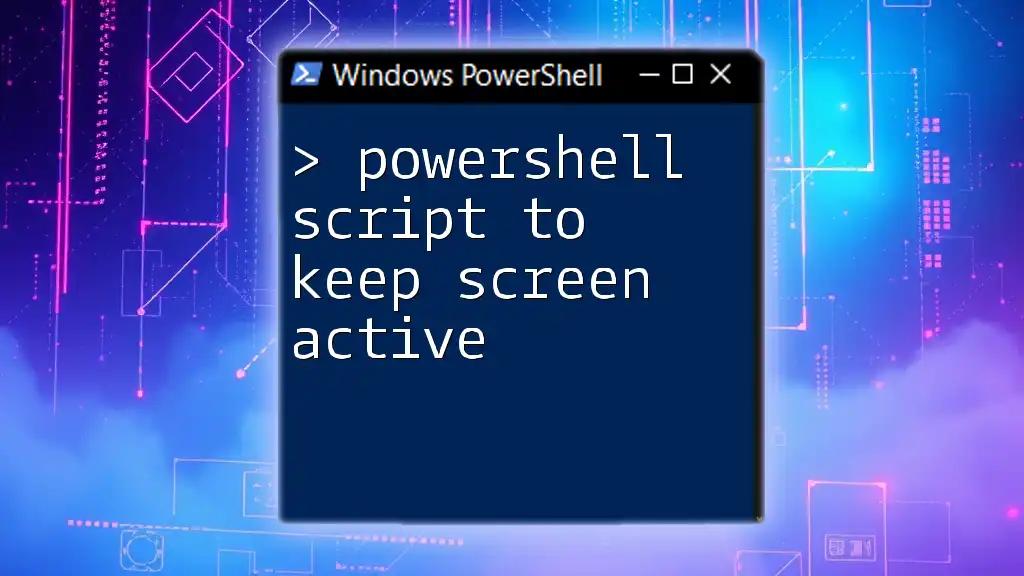
Troubleshooting Common Issues
Common Errors in Executing EXE Files
While executing EXE files using PowerShell, you may encounter several common errors such as:
- Permissions Issues: Make sure you have the appropriate permissions to execute the EXE file.
- Path Not Found: Verify that the path to the EXE is correct. If it contains spaces, ensure it is wrapped in quotes.
Testing and Debugging PowerShell Scripts
Testing your PowerShell scripts is crucial for ensuring that your EXE files execute correctly. When debugging, consider using the `-WhatIf` parameter or write verbose outputs to help you track the execution flow. Collecting feedback along the way will guide you to identify and fix potential problems quicker.

Conclusion
Using a PowerShell script to execute EXE files can greatly enhance your scripting capabilities, automate tedious tasks, and streamline system management. By understanding how to run executable files efficiently, you can leverage PowerShell to boost your productivity and deepen your command over IT automation.
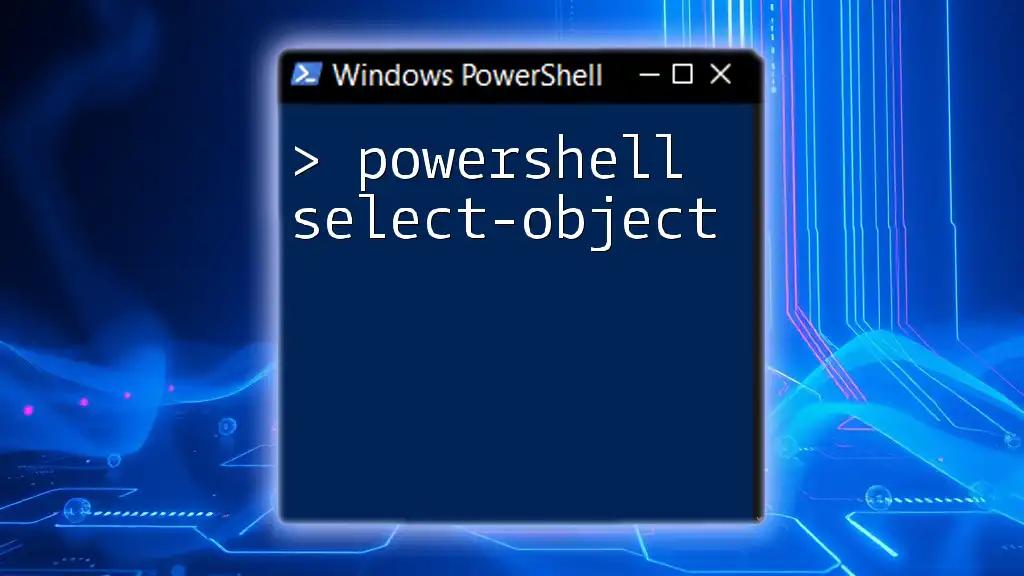
Call to Action
We invite you to share your experiences with executing EXEs using PowerShell and how it has improved your workflows. If you found this article helpful, consider subscribing for more insights and tips on mastering PowerShell!
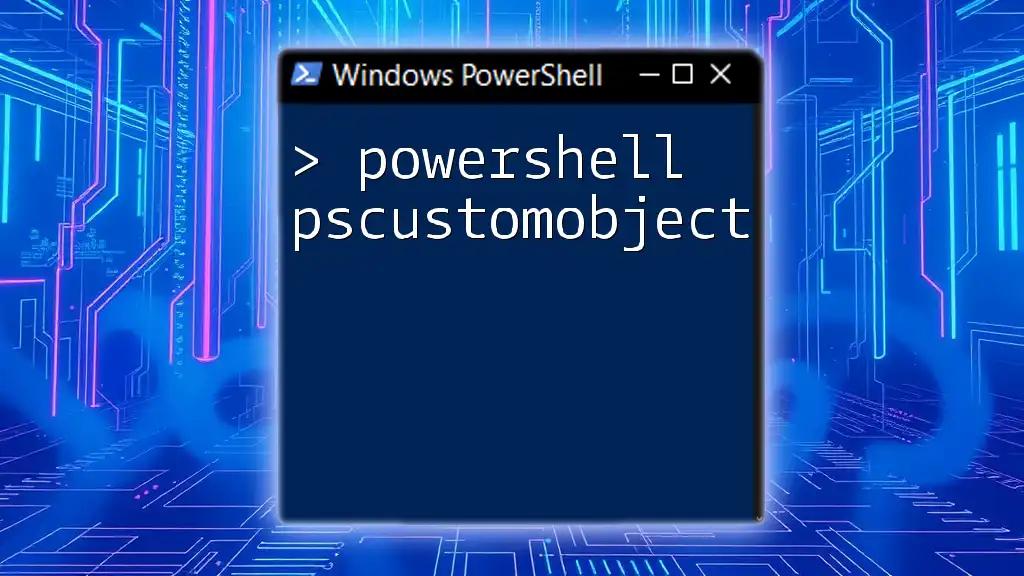
Additional Resources
For further exploration, consider checking out the official PowerShell documentation and a selection of books or online courses designed for advanced PowerShell users.