You can use the following PowerShell script to keep your screen active by simulating key presses at regular intervals:
while ($true) {
[System.Windows.Forms.SendKeys]::SendWait("{F15}")
Start-Sleep -Seconds 300
}
This script will send a non-intrusive function key press every 5 minutes to prevent your screen from sleeping.
Understanding Power Settings
The Importance of Power Settings
Power settings in Windows play a crucial role in managing how your computer behaves when it's not actively in use. Understanding these settings is essential for improving productivity, particularly for individuals who may need their screens unfettered by automatic sleep or timeout periods. Specifically, when the system enters sleep mode, it can interrupt workflows and lead to loss of concentration, making it vital to find ways to keep the computer awake.
How PowerShell Interacts with Power Settings
PowerShell serves as a powerful tool for interacting with and adjusting system settings, including power configurations. With just a few commands, users can tweak power options to create a conducive work environment that fits their needs. Commands in PowerShell allow you to query and modify the power settings programmatically, making it easier to keep your screen active without the need for continual manual adjustments.
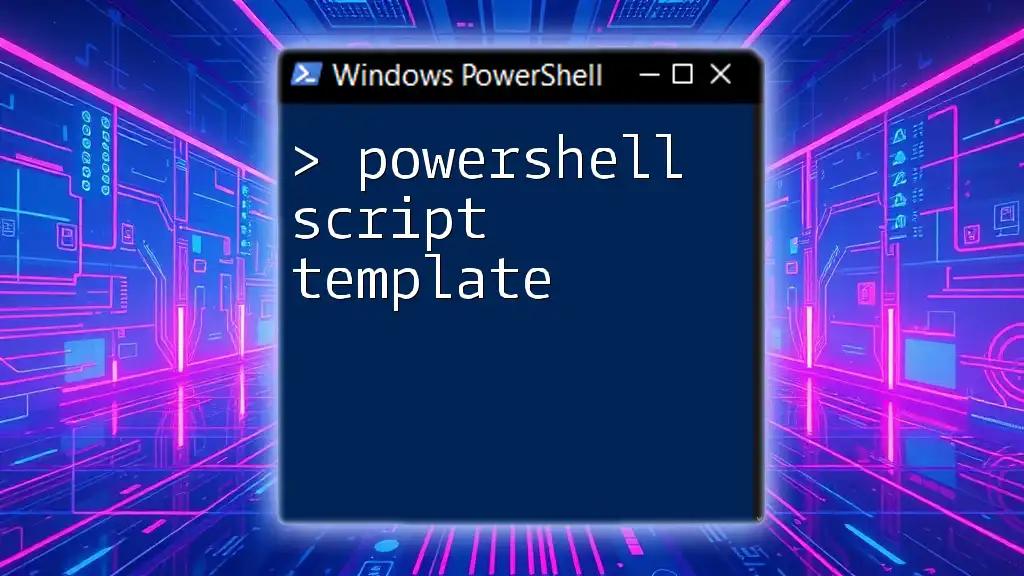
Basics of Writing a PowerShell Script
What is a PowerShell Script?
A PowerShell script is a file containing a series of commands that the PowerShell interpreter can execute. These scripts enable users to automate repetitive tasks, streamline workflows, and manage system configurations efficiently. The key components generally include cmdlets, functions, variables, and, most importantly, control structures that dictate how and when commands are executed.
Setting Up Your PowerShell Environment
To start creating and executing PowerShell scripts, ensure that you are running an appropriate version of PowerShell. Typically, it is recommended to use the Windows PowerShell or PowerShell Core—the latter being cross-platform. You can easily open PowerShell as an administrator by searching for it in the Start menu, right-clicking it, and selecting "Run as administrator," which may be necessary for certain commands.
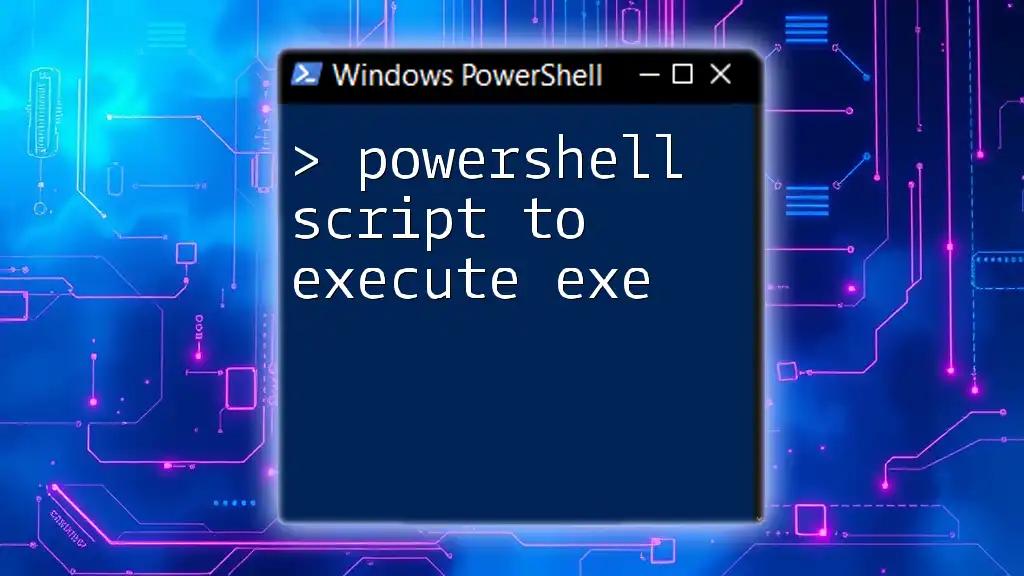
Creating a PowerShell Script to Keep the Screen Active
Overview of the Concept
Keeping the screen active can be particularly beneficial during long presentations, video calls, or coding sessions where you don't want your monitor to dim or lock automatically. This is where a PowerShell script to keep the screen active comes into play, allowing for prolonged computer use without interruptions.
Example Command to Keep the Screen Awake
Here is a sample PowerShell script that can keep your computer's screen active:
Add-Type -AssemblyName System.Windows.Forms
[System.Windows.Forms.Application]::SetCompatibleTextRenderingDefault($false)
while ($true) {
# Move the mouse cursor slightly to keep the screen awake
[System.Windows.Forms.Cursor]::Position = New-Object System.Drawing.Point(0, 0)
Start-Sleep -Seconds 300 # Sleep for 5 minutes
}
Explanation of Each Line:
- The first line loads the System.Windows.Forms assembly, which provides the necessary classes for UI manipulation, including cursor control.
- The second line ensures that text rendering is set correctly to avoid compatibility issues.
- The `while` loop runs indefinitely, moving the cursor slightly at the specified interval to prevent screen timeout.
- The `Start-Sleep` command pauses the script for 300 seconds (or 5 minutes) between movements. You can change the duration to your preference.
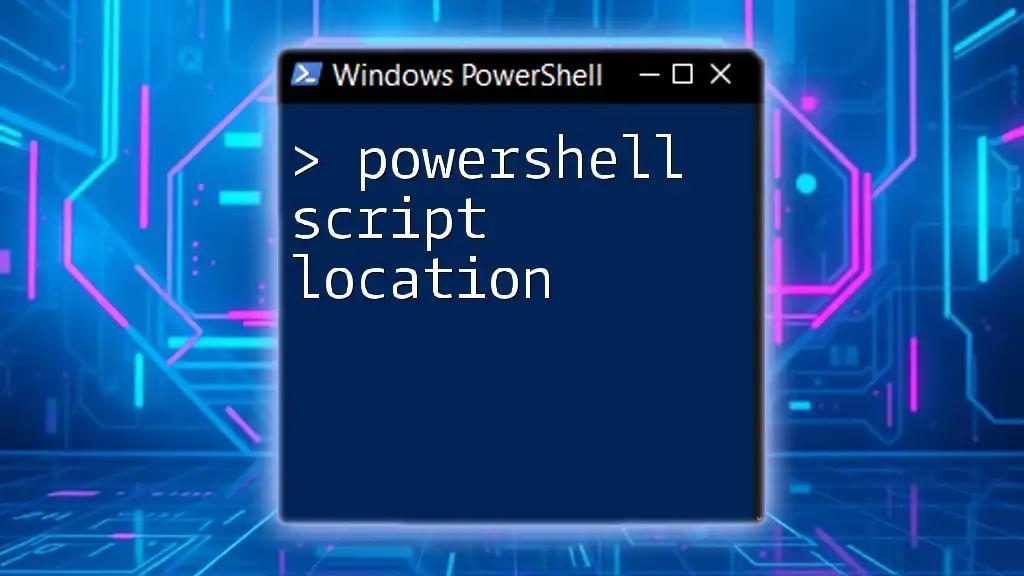
Scheduling the Script
Using Task Scheduler to Run the Script
To ensure that your PowerShell script runs at specific intervals or times, you can utilize the Windows Task Scheduler. Here’s how:
- Open Task Scheduler by searching for it in the Start Menu.
- Click on “Create Basic Task” to start the wizard.
- Name your task and provide a description, then click Next.
- Choose the trigger (daily, weekly, etc.) and click Next.
- Select Start a Program and then browse to select your PowerShell script.
- Finish the wizard, and your script will now run according to the schedule you set.
Running the Script at Startup
For convenience, you might want to configure your script to run automatically when you start your computer:
- Press `Win + R` to open the Run dialog.
- Type `shell:startup` to open the startup folder.
- Create a shortcut of your PowerShell script in this folder.
This way, every time you log in, the PowerShell script will start running, ensuring your screen remains active without any additional effort.
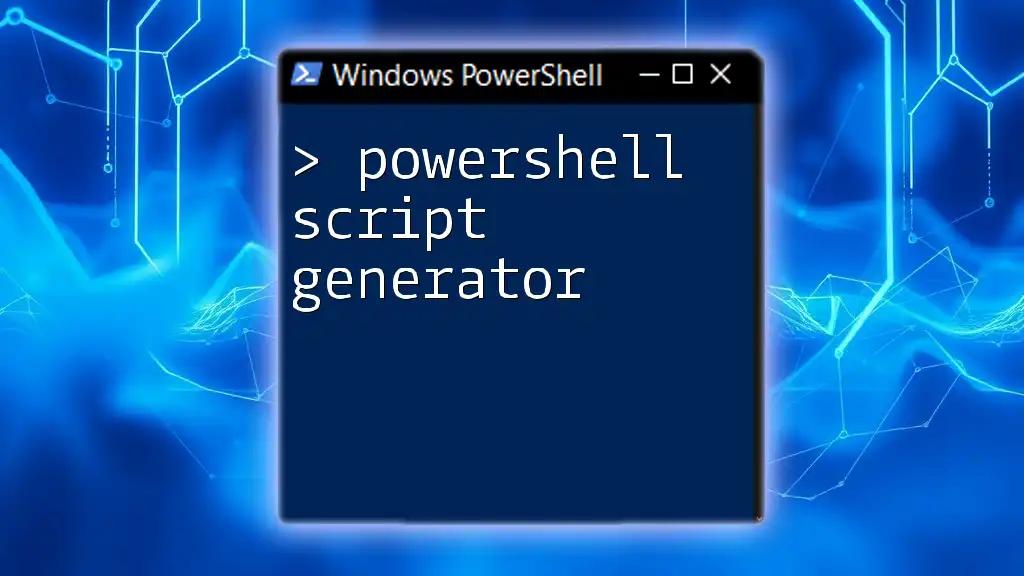
Alternative Methods to Keep the Computer Awake
Using PowerShell to Adjust Power Plan Settings
Instead of running a script continuously, you can also adjust the power settings through PowerShell commands. The following are commands that set your monitor timeout to never, thus keeping your screen active:
powercfg -change -monitor-timeout-ac 0
powercfg -change -monitor-timeout-dc 0
Explanation:
- The first command applies to when the computer is plugged into a power source (AC).
- The second command applies to when the computer is running on battery (DC).
- Setting the timeout to `0` means that the monitor will never turn off due to inactivity.
Third-party Tools
For users looking for a more user-friendly solution, third-party applications are available that can perform similar tasks with a graphical user interface. Tools like Caffeine or Mouse Jiggler simulate mouse movement without continuous scripting. However, these applications might come with their own costs and limitations, making PowerShell scripting a more flexible solution for those comfortable with command lines.

Troubleshooting Common Issues
Script Doesn’t Work
If your script fails to execute correctly, consider these common issues:
- Ensure PowerShell is running with administrative privileges; otherwise, some commands may be blocked.
- Check for typographical errors in the script which might prevent it from running properly.
- Use `Write-Host` statements for debugging by inserting them to see how far the script executes before encountering an error.
Power Settings Not Applying
Sometimes adjustments to power settings may not apply correctly. Here’s what can be checked:
- Reboot your system after making changes, as some settings may require a restart to take effect.
- Verify if any group policies in a corporate environment are overriding personal settings.
- Run `powercfg -query` in PowerShell to display current settings and ensure they’re set as intended.
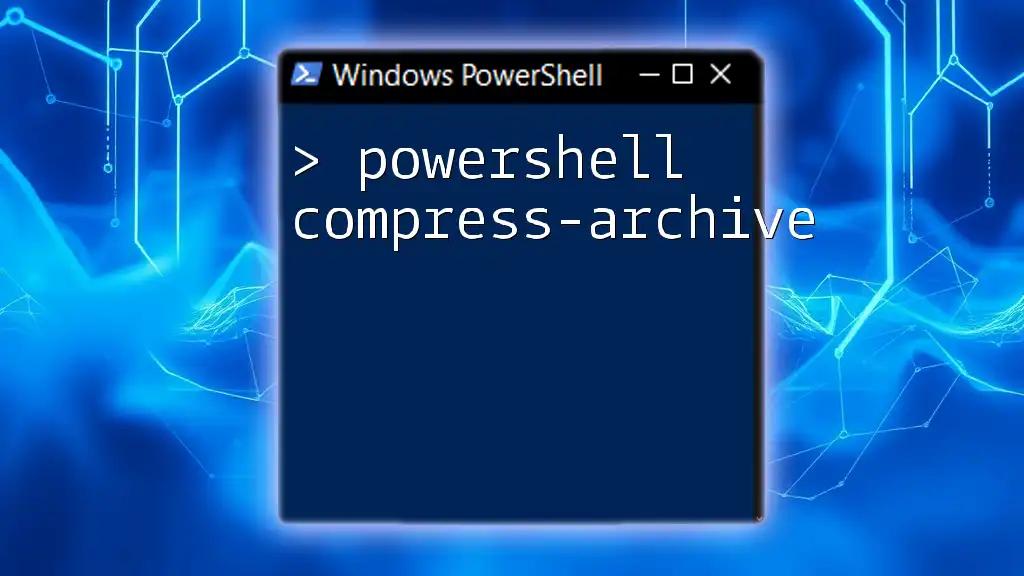
Conclusion
Using a PowerShell script to keep the screen active is an effective way to ensure your productivity remains uninterrupted. With just a few lines of code, you can automate a process that significantly enhances your working environment. By leveraging tools such as Task Scheduler and adjusting power settings, you can create a seamless workflow that suits your needs.
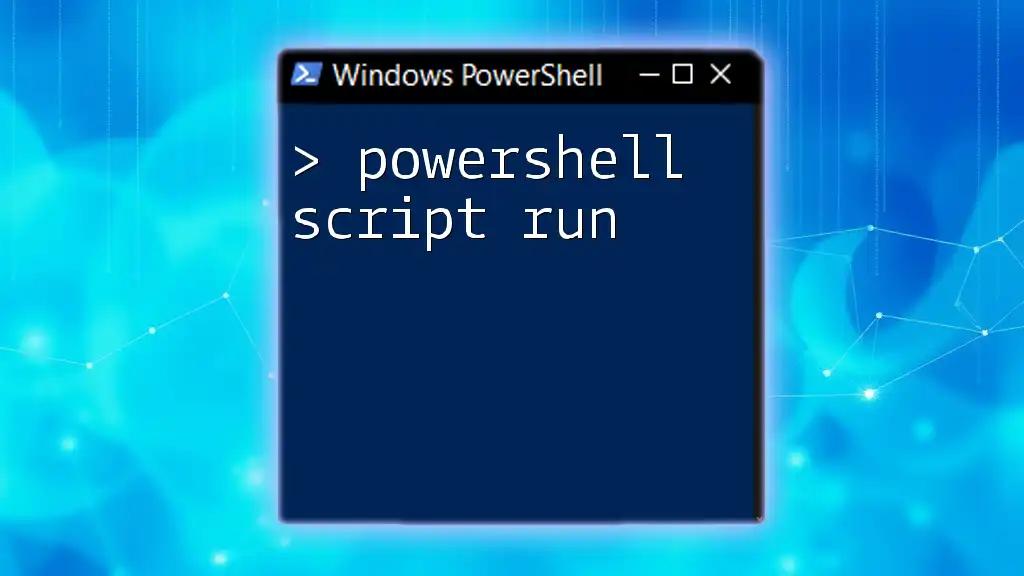
Call to Action
If you found this guide helpful, don't hesitate to reach out for more PowerShell tips and scripting ideas. Join our community for ongoing learning and to share your experiences with automation.
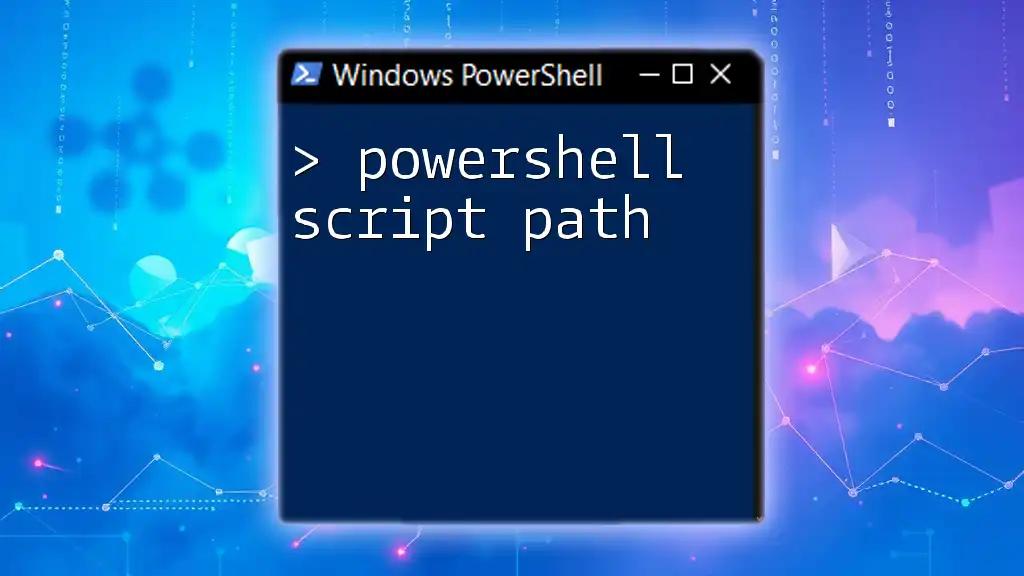
Additional Resources
For further reading and advanced PowerShell scripting techniques, consider visiting reputable websites and forums dedicated to scripting and Windows management. Resources like the Microsoft PowerShell documentation or PowerShell.org can be invaluable for both beginners and advanced users.
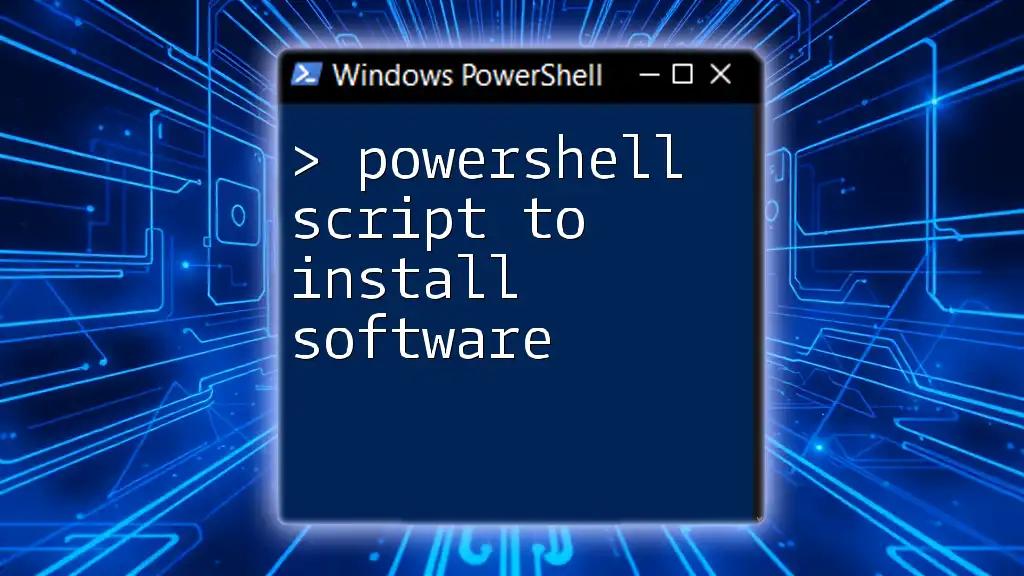
FAQs
Is it safe to keep the computer awake for long periods?
Keeping your computer awake for extended periods is generally safe but may lead to increased power consumption and potentially harm the display in the long run.
Can I customize the interval of the script to keep the screen active?
Yes, you can easily change the `Start-Sleep -Seconds 300` command in the script to any duration you prefer.
What are some other scripts I can use to enhance productivity?
Many scripts can automate routine tasks, such as regular backups, software installations, or system monitoring. Let us know what specific tasks you want to automate, and we can provide scripts tailored to those needs!